GUI parts for DISCO-F746NG. GuiBase, Button, ButtonGroup, Label, BlinkLabel, NumericLabel, SeekBar, SeekbarGroup
Dependents: F746_SD_GraphicEqualizer_ren0620
Fork of F746_GUI by
Label.cpp
00001 //----------------------------------------------------------- 00002 // Label class 00003 // 00004 // 2016/04/24, Copyright (c) 2016 MIKAMI, Naoki 00005 //----------------------------------------------------------- 00006 00007 #include "Label.hpp" 00008 00009 namespace Mikami 00010 { 00011 // Constructor 00012 Label::Label(uint16_t x, uint16_t y, 00013 const string str, TextAlignMode mode, sFONT &fonts, 00014 uint32_t textColor, uint32_t backColor) 00015 : GuiBase(x, y, fonts, textColor, backColor), 00016 MODE_(mode), STR_(str) 00017 { 00018 length_ = str.length(); 00019 lcd_.SetBackColor(backColor); 00020 lcd_.SetTextColor(textColor); 00021 lcd_.SetFont(&fonts); 00022 DrawString(PosX(x), y, str); 00023 } 00024 00025 void Label::Draw(const string str, uint32_t textColor) 00026 { 00027 // Erase previously-drawn string 00028 lcd_.SetTextColor(BACK_COLOR_); 00029 length_ = (length_ > str.length()) ? length_ : str.length(); 00030 lcd_.FillRect(PosX(X_), Y_, FONTS_->Width*length_+1, FONTS_->Height); 00031 00032 // Draw new string 00033 length_ = str.length(); 00034 lcd_.SetFont(FONTS_); 00035 lcd_.SetTextColor(textColor); 00036 DrawString(PosX(X_), Y_, str); 00037 } 00038 00039 uint16_t Label::PosX(uint16_t x) 00040 { 00041 if (MODE_ == LEFT) return x; 00042 else 00043 { 00044 if (MODE_ == CENTER) 00045 return x - length_*FONTS_->Width/2; 00046 else 00047 return x - length_*FONTS_->Width; 00048 } 00049 } 00050 }
Generated on Fri Jul 22 2022 12:03:18 by
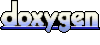