GUI parts for DISCO-F746NG. GuiBase, Button, ButtonGroup, Label, BlinkLabel, NumericLabel, SeekBar, SeekbarGroup
Dependents: F746_SD_GraphicEqualizer_ren0620
Fork of F746_GUI by
Button.hpp
00001 //----------------------------------------------------------- 00002 // Button class handling multi-touch -- Header 00003 // Multi-touch: Enabled (default) 00004 // 00005 // 2016/03/29, Copyright (c) 2016 MIKAMI, Naoki 00006 //----------------------------------------------------------- 00007 00008 #ifndef F746_BUTTON_HPP 00009 #define F746_BUTTON_HPP 00010 00011 #include "GuiBase.hpp" 00012 00013 namespace Mikami 00014 { 00015 class Button : public GuiBase 00016 { 00017 public: 00018 // Constructor 00019 Button(uint16_t x, uint16_t y, 00020 uint16_t width, uint16_t height, uint16_t x_Expand, 00021 const string str = "", sFONT &fonts = Font12, 00022 uint32_t textColor = GuiBase::ENUM_TEXT, 00023 uint32_t backColor = GuiBase::ENUM_BACK, 00024 uint32_t createdColor = GuiBase::ENUM_CREATED, 00025 uint32_t touchedColor = GuiBase::ENUM_TOUCHED, 00026 uint32_t inactiveColor = GuiBase::ENUM_INACTIVE, 00027 uint32_t inactiveTextColor = GuiBase::ENUM_INACTIVE_TEXT) 00028 : GuiBase(x, y, fonts, 00029 textColor, backColor, createdColor, 00030 touchedColor, inactiveColor, 00031 inactiveTextColor), 00032 W_(width), H_(height), X_expend(x_Expand), STR_(str), active_(true) 00033 { Draw(); } 00034 00035 // Draw button 00036 void Draw(uint32_t color, uint32_t textColor); 00037 void Draw(uint32_t color) { Draw(color, TEXT_COLOR_); } 00038 void Draw() { Draw(CREATED_COLOR_, TEXT_COLOR_); } 00039 00040 // Erase button 00041 void Erase() { Draw(BACK_COLOR_, BACK_COLOR_); } 00042 00043 // Check touch detected and redraw button 00044 bool Touched(); 00045 00046 bool IsOnButton(); 00047 00048 void Activate(); 00049 void Inactivate(); 00050 bool IsActive() { return active_; } 00051 00052 // Set or reset multi-touch 00053 static void SetMultiTouch(bool tf) { multiTouch_ = tf; } 00054 00055 private: 00056 const uint16_t W_, H_, X_expend; 00057 const string STR_; 00058 bool active_; 00059 00060 // disallow copy constructor and assignment operator 00061 Button(const Button&); 00062 Button& operator=(const Button&); 00063 }; 00064 } 00065 #endif // F746_BUTTON_HPP
Generated on Fri Jul 22 2022 12:03:18 by
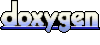