GUI parts for DISCO-F746NG. GuiBase, Button, ButtonGroup, Label, BlinkLabel, NumericLabel, SeekBar, SeekbarGroup
Dependents: F746_SD_GraphicEqualizer_ren0620
Fork of F746_GUI by
ButtonGroup.hpp
00001 //----------------------------------------------------------- 00002 // ButtonGroup class -- Header 00003 // 00004 // 2016/04/21, Copyright (c) 2016 MIKAMI, Naoki 00005 //----------------------------------------------------------- 00006 00007 #ifndef F746_BUTTON_GROUP_HPP 00008 #define F746_BUTTON_GROUP_HPP 00009 00010 #include "Button.hpp" 00011 00012 namespace Mikami 00013 { 00014 class ButtonGroup : public GuiBase 00015 { 00016 public: 00017 // Constructor 00018 ButtonGroup(uint16_t x0, uint16_t y0, 00019 uint16_t width, uint16_t height, 00020 uint16_t number, const string str[], 00021 uint16_t spaceX = 0, uint16_t spaceY = 0, 00022 uint16_t column = 1, 00023 int touched = -1, // number for button initially touched-color 00024 bool expanded = false, 00025 sFONT &fonts = Font12, 00026 uint32_t textColor = GuiBase::ENUM_TEXT, 00027 uint32_t backColor = GuiBase::ENUM_BACK, 00028 uint32_t createdColor = GuiBase::ENUM_CREATED, 00029 uint32_t touchedColor = GuiBase::ENUM_TOUCHED, 00030 uint32_t inactiveColor = GuiBase::ENUM_INACTIVE, 00031 uint32_t inactiveTextColor = GuiBase::ENUM_INACTIVE_TEXT); 00032 00033 // Destructor 00034 ~ButtonGroup(); 00035 00036 // Draw button 00037 bool Draw(int num, uint32_t color, uint32_t textColor); 00038 bool Draw(int num) { return Draw(num, CREATED_COLOR_, TEXT_COLOR_); } 00039 00040 // Change to touched color 00041 bool TouchedColor(int num); 00042 00043 // Draw all buttons 00044 void DrawAll(uint32_t color, uint32_t textColor) 00045 { 00046 for (int n=0; n<numberOfButtons_; n++) 00047 buttons_[n]->Draw(color, textColor); 00048 } 00049 void DrawAll() { DrawAll(CREATED_COLOR_, TEXT_COLOR_); } 00050 00051 // Erase button 00052 bool Erase(int num); 00053 00054 // Check touch detected for specified button 00055 bool Touched(int num); 00056 00057 // Get touched number 00058 bool GetTouchedNumber(int &num); 00059 00060 // Activate and inactivate 00061 bool Activate(int num); 00062 bool Inactivate(int num); 00063 void ActivateAll() 00064 { 00065 for (int n=0; n<numberOfButtons_; n++) 00066 buttons_[n]->Activate(); 00067 } 00068 void InactivateAll() 00069 { 00070 for (int n=0; n<numberOfButtons_; n++) 00071 buttons_[n]->Inactivate(); 00072 } 00073 00074 private: 00075 Button **buttons_; 00076 int numberOfButtons_; 00077 __IO int prevNum_; 00078 00079 // Check range of argument 00080 bool Range(int n) 00081 { return ((n >= 0) && (n < numberOfButtons_)); } 00082 00083 // disallow copy constructor and assignment operator 00084 ButtonGroup(const ButtonGroup&); 00085 ButtonGroup& operator=(const ButtonGroup&); 00086 }; 00087 } 00088 #endif // F746_BUTTON_GROUP_HPP
Generated on Fri Jul 22 2022 12:03:18 by
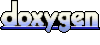