GUI parts for DISCO-F746NG. GuiBase, Button, ButtonGroup, Label, BlinkLabel, NumericLabel, SeekBar, SeekbarGroup
Dependents: F746_SD_GraphicEqualizer_ren0620
Fork of F746_GUI by
ButtonGroup.cpp
00001 //----------------------------------------------------------- 00002 // ButtonGroup class 00003 // 00004 // 2016/04/07, Copyright (c) 2016 MIKAMI, Naoki 00005 //----------------------------------------------------------- 00006 00007 #include "ButtonGroup.hpp" 00008 00009 namespace Mikami 00010 { 00011 // Constructor 00012 ButtonGroup::ButtonGroup( 00013 uint16_t x0, uint16_t y0, 00014 uint16_t width, uint16_t height, 00015 uint16_t number, const string str[], 00016 uint16_t spaceX, uint16_t spaceY, 00017 uint16_t column, int touched, 00018 bool expended, 00019 sFONT &fonts, 00020 uint32_t textColor, uint32_t backColor, 00021 uint32_t createdColor, uint32_t touchedColor, 00022 uint32_t inactiveColor, uint32_t inactiveTextColor) 00023 : GuiBase(x0, y0, fonts, textColor, backColor, 00024 createdColor, touchedColor, 00025 inactiveColor, inactiveTextColor), 00026 numberOfButtons_(number), prevNum_(touched) 00027 { 00028 buttons_ = new Button *[number]; 00029 for (int n=0; n<number; n++) 00030 { 00031 div_t u1 = div(n, column); 00032 uint16_t x = x0 + u1.rem*(width + spaceX); 00033 uint16_t y = y0 + u1.quot*(height + spaceY); 00034 uint16_t xpend_detectArea = (expended? width*6 : 0); 00035 buttons_[n] = 00036 new Button(x, y, width, height, xpend_detectArea, str[n], fonts, 00037 TEXT_COLOR_, BACK_COLOR_, 00038 CREATED_COLOR_, TOUCHED_COLOR_, 00039 INACTIVE_COLOR_, INACTIVE_TEXT_COLOR_); 00040 } 00041 // On created, set touched color as needed 00042 if (touched >= 0) TouchedColor(touched); 00043 } 00044 00045 // Destructor 00046 ButtonGroup::~ButtonGroup() 00047 { 00048 for (int n=0; n<numberOfButtons_; n++) delete buttons_[n]; 00049 delete[] *buttons_; 00050 } 00051 00052 // Draw button 00053 bool ButtonGroup::Draw(int num, uint32_t color, uint32_t textColor) 00054 { 00055 if (!Range(num)) return false; 00056 buttons_[num]->Draw(color, textColor); 00057 return true; 00058 } 00059 00060 // Change to touched color 00061 bool ButtonGroup::TouchedColor(int num) 00062 { 00063 if (prevNum_ != num) prevNum_ = num; 00064 return Draw(num, TOUCHED_COLOR_, TEXT_COLOR_); 00065 } 00066 00067 // Erase button 00068 bool ButtonGroup::Erase(int num) 00069 { 00070 if (!Range(num)) return false; 00071 buttons_[num]->Erase(); 00072 return true; 00073 } 00074 00075 // Check touch detected for specified button 00076 bool ButtonGroup::Touched(int num) 00077 { 00078 if (!Range(num)) return false; 00079 if (!buttons_[num]->IsActive()) return false; 00080 int touched; 00081 if (!GetTouchedNumber(touched)) return false; 00082 bool rtn = (touched == num) ? true : false; 00083 return rtn; 00084 } 00085 00086 // Get touched number 00087 bool ButtonGroup::GetTouchedNumber(int &num) 00088 { 00089 bool rtn = false; 00090 if (PanelTouched()) 00091 { 00092 for (int n=0; n<numberOfButtons_; n++) 00093 if (buttons_[n]->IsOnButton() && 00094 buttons_[n]->IsActive()) 00095 { 00096 num = n; 00097 rtn = true; 00098 } 00099 00100 if (!rtn) return false; 00101 } 00102 else 00103 return false; 00104 00105 buttons_[num]->Draw(TOUCHED_COLOR_); 00106 if ((prevNum_ >= 0) && (prevNum_ != num)) 00107 buttons_[prevNum_]->Draw(); 00108 if (prevNum_ != num) prevNum_ = num; 00109 return true; 00110 } 00111 00112 // Activate and inactivate 00113 bool ButtonGroup::Activate(int num) 00114 { 00115 if (!Range(num)) return false; 00116 buttons_[num]->Activate(); 00117 return true; 00118 } 00119 bool ButtonGroup::Inactivate(int num) 00120 { 00121 if (!Range(num)) return false; 00122 buttons_[num]->Inactivate(); 00123 return true; 00124 } 00125 }
Generated on Fri Jul 22 2022 12:03:18 by
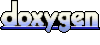