
This is an example of BLE GATT Client, which receives broadcast data from BLE_Server_BME280 ( a GATT server) , then transfers values up to mbed Device Connector (cloud).
test_m2mstring.cpp
00001 /* 00002 * Copyright (c) 2015 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "CppUTest/TestHarness.h" 00017 #include "test_m2mstring.h" 00018 #include <stdio.h> 00019 #include <string.h> 00020 00021 Test_M2MString::Test_M2MString() 00022 { 00023 str = new String("test"); 00024 } 00025 00026 Test_M2MString::~Test_M2MString() 00027 { 00028 delete str; 00029 } 00030 00031 void Test_M2MString::test_string_and_len_constructor() 00032 { 00033 // make sure the len parameter works as expected 00034 String s("hello_world", 5); 00035 CHECK(s == "hello"); 00036 CHECK(s.size() == 5); 00037 } 00038 00039 void Test_M2MString::test_copy_constructor() 00040 { 00041 String s("name"); 00042 String s1(s); 00043 CHECK(s1.p[1] == 'a'); 00044 CHECK(s.size() == s1.size()); 00045 } 00046 00047 void Test_M2MString::test_operator_assign() 00048 { 00049 String s("name"); 00050 String s1 = s; 00051 const String s2("yes"); 00052 CHECK(s1.p[1] == 'a'); 00053 00054 s1 = "yeb"; 00055 CHECK(s1.p[1] == 'e'); 00056 s = s2; 00057 CHECK(s.p[1] == 'e'); 00058 } 00059 00060 void Test_M2MString::test_operator_add() 00061 { 00062 String s("name"); 00063 String s1("yeb"); 00064 00065 s += 'r'; 00066 CHECK(s.p[4] == 'r'); 00067 00068 s += s1; 00069 CHECK(s.p[5] == 'y'); 00070 00071 s += "hoi"; 00072 CHECK(s.p[9] == 'o'); 00073 00074 s1 += "somethingverylongggggggg"; 00075 00076 } 00077 00078 void Test_M2MString::test_push_back() 00079 { 00080 String s("name"); 00081 00082 s.push_back('r'); 00083 CHECK(s.p[4] == 'r'); 00084 } 00085 00086 void Test_M2MString::test_operator_equals() 00087 { 00088 String s("name"); 00089 String s1("yeb"); 00090 00091 CHECK( (s == s1) == false); 00092 CHECK( (s == "name") == true); 00093 const char c = NULL; 00094 CHECK( (s == c) == false); 00095 free(s.p); 00096 s.p = NULL; 00097 CHECK( (s == c) == true); 00098 } 00099 00100 void Test_M2MString::test_clear() 00101 { 00102 String s("name"); 00103 00104 s.clear(); 00105 CHECK(s.size_ == 0); 00106 } 00107 00108 void Test_M2MString::test_size() 00109 { 00110 String s("name"); 00111 String s1("yeb"); 00112 00113 CHECK(s.size() == 4); 00114 } 00115 00116 void Test_M2MString::test_length() 00117 { 00118 String s("name"); 00119 String s1("yeb"); 00120 00121 CHECK(s.length() == 4); 00122 } 00123 00124 void Test_M2MString::test_capacity() 00125 { 00126 String s("name"); 00127 String s1("yeb"); 00128 00129 CHECK(s.capacity() == 4); 00130 } 00131 00132 void Test_M2MString::test_empty() 00133 { 00134 String s("name"); 00135 String s1("yeb"); 00136 00137 CHECK(s.empty() == false); 00138 } 00139 00140 void Test_M2MString::test_c_str() 00141 { 00142 String s("name"); 00143 String s1("yeb"); 00144 00145 CHECK( s.c_str() != NULL); 00146 } 00147 00148 void Test_M2MString::test_reserve() 00149 { 00150 String s("name"); 00151 String s1("yeb"); 00152 00153 s.reserve(12); 00154 CHECK(s.allocated_ == 13); 00155 } 00156 00157 void Test_M2MString::test_resize() 00158 { 00159 String s("name"); 00160 String s1("yeb"); 00161 00162 s.resize(2); 00163 CHECK( s.size() == 2); 00164 00165 s.resize(12); 00166 CHECK( s.size() == 12); 00167 } 00168 00169 void Test_M2MString::test_swap() 00170 { 00171 String s("name"); 00172 String s1("yeb"); 00173 00174 s.swap(s1); 00175 CHECK(s1.p[1] == 'a'); 00176 00177 CHECK(s.p[1] == 'e'); 00178 } 00179 00180 void Test_M2MString::test_substr() 00181 { 00182 String s("name"); 00183 String s1("yeb"); 00184 00185 CHECK(s.substr(1, 1) == "a"); 00186 CHECK(s.substr(3, 4) == "e"); 00187 } 00188 00189 void Test_M2MString::test_operator_get() 00190 { 00191 String s("name"); 00192 const String s1("yeb"); 00193 00194 CHECK(s[1] == 'a'); 00195 CHECK(s1[1] == 'e'); 00196 } 00197 00198 void Test_M2MString::test_at() 00199 { 00200 String s("name"); 00201 const String s1("yeb"); 00202 00203 CHECK(s.at(1) == 'a'); 00204 CHECK(s.at(14) == '\0'); 00205 CHECK(s1.at(1) == 'e'); 00206 CHECK(s1.at(31) == '\0'); 00207 } 00208 00209 void Test_M2MString::test_erase() 00210 { 00211 String s("name"); 00212 String s1("yeb"); 00213 00214 s.erase(1,1); 00215 CHECK(s[1] == 'm'); 00216 } 00217 00218 void Test_M2MString::test_append() 00219 { 00220 String s("name"); 00221 String s1("yeb"); 00222 00223 s.append( s1.c_str(), 1 ); 00224 CHECK(s.size() == 5); 00225 00226 s.append( s1.c_str(), 15 ); 00227 CHECK(s.size() == 8); 00228 } 00229 00230 void Test_M2MString::test_append_raw() 00231 { 00232 String s("name"); 00233 const char test_source[] = "something"; 00234 String expected("namesomething"); 00235 00236 s.append_raw(test_source, 1); 00237 CHECK(s.size() == 5); 00238 00239 s.append_raw(test_source + 1, 8); 00240 CHECK(s.size() == 13); 00241 00242 CHECK(s == expected); 00243 } 00244 00245 void Test_M2MString::test_append_int() 00246 { 00247 String s("source"); 00248 String expected("source1234"); 00249 String expected2("source12342147483647"); 00250 00251 s.append_int(1234); 00252 CHECK(s.size() == 10); 00253 00254 CHECK(s == expected); 00255 00256 s.append_int(INT32_MAX); 00257 00258 CHECK(s == expected2); 00259 } 00260 00261 void Test_M2MString::test_compare() 00262 { 00263 String s("name"); 00264 String s1("yeb"); 00265 String s2("name"); 00266 String s3("nam"); 00267 00268 CHECK(s.compare(1,5, s1) < 0); 00269 CHECK(s1.compare(0,5, s2) > 0); 00270 CHECK(s.compare(0,4, s2) == 0); 00271 CHECK(s.compare(0,4, s3) > 0); 00272 00273 CHECK(s.compare(1,5, "yeb") < 0); 00274 CHECK(s1.compare(0,5, "name") > 0); 00275 CHECK(s.compare(0,4, "name") == 0); 00276 CHECK(s.compare(0,4, "nam") > 0); 00277 } 00278 00279 void Test_M2MString::test_find_last_of() 00280 { 00281 String s("namenamename"); 00282 String s1("yeb"); 00283 00284 CHECK(s.find_last_of('n') == 8); 00285 } 00286 00287 void Test_M2MString::test_operator_lt() 00288 { 00289 String s("name"); 00290 String s1("yeb"); 00291 String s2("yea"); 00292 00293 CHECK( (s < s1 ) == true); 00294 CHECK( (s1 < s2 ) == false); 00295 } 00296 void Test_M2MString::test_reverse() 00297 { 00298 char string1[] = "123"; 00299 char string2[] = "321"; 00300 m2m::reverse(string1, strlen(string1)); 00301 char string3[] = "9223372036854775807"; 00302 char string4[] = "7085774586302733229"; 00303 m2m::reverse(string3, strlen(string3)); 00304 00305 CHECK(strcmp(string1, string2) == 0); 00306 CHECK(strcmp(string3, string4) == 0); 00307 } 00308 void Test_M2MString::test_itoa_c() 00309 { 00310 int64_t value1 = 0; 00311 char* string1 = "0"; 00312 int64_t value2 = -10; 00313 char* string2 = "-10"; 00314 int64_t value3 = 10000; 00315 char* string3 = "10000"; 00316 int64_t value4 = 9223372036854775807; 00317 char* string4 = "9223372036854775807"; 00318 int64_t value5 = -9223372036854775807; 00319 char* string5 = "-9223372036854775807"; 00320 00321 char *buffer = (char*)malloc(21); 00322 00323 if(buffer) { 00324 m2m::itoa_c(value1, buffer); 00325 CHECK(strcmp(string1, buffer) == 0); 00326 m2m::itoa_c(value2, buffer); 00327 CHECK(strcmp(string2, buffer) == 0); 00328 m2m::itoa_c(value3, buffer); 00329 CHECK(strcmp(string3, buffer) == 0); 00330 m2m::itoa_c(value4, buffer); 00331 CHECK(strcmp(string4, buffer) == 0); 00332 m2m::itoa_c(value5, buffer); 00333 CHECK(strcmp(string5, buffer) == 0); 00334 free(buffer); 00335 } 00336 } 00337 00338 void Test_M2MString::test_convert_integer_to_array() 00339 { 00340 uint8_t *max_age_ptr = NULL; 00341 uint8_t max_age_len = 0; 00342 uint8_t *temp = NULL; 00343 uint8_t temp_len = 0; 00344 00345 int64_t val = 0; 00346 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00347 CHECK(max_age_ptr != NULL); 00348 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00349 temp = m2m::String::convert_integer_to_array(0,temp_len, max_age_ptr, max_age_len); 00350 CHECK(temp != NULL); 00351 CHECK(val == m2m::String::convert_array_to_integer(temp, temp_len)); 00352 free(temp); 00353 free(max_age_ptr); 00354 max_age_ptr = NULL; 00355 00356 val = 0xff; 00357 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00358 CHECK(max_age_ptr != NULL); 00359 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00360 temp = m2m::String::convert_integer_to_array(0,temp_len, max_age_ptr, max_age_len); 00361 CHECK(temp != NULL); 00362 CHECK(val == m2m::String::convert_array_to_integer(temp, temp_len)); 00363 free(temp); 00364 free(max_age_ptr); 00365 max_age_ptr = NULL; 00366 00367 val = 0xffff; 00368 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00369 CHECK(max_age_ptr != NULL); 00370 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00371 free(max_age_ptr); 00372 max_age_ptr = NULL; 00373 00374 val = 0xffffff; 00375 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00376 CHECK(max_age_ptr != NULL); 00377 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00378 free(max_age_ptr); 00379 max_age_ptr = NULL; 00380 00381 val = 0xffffffff; 00382 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00383 CHECK(max_age_ptr != NULL); 00384 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00385 free(max_age_ptr); 00386 max_age_ptr = NULL; 00387 00388 val = 0xffffffffff; 00389 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00390 CHECK(max_age_ptr != NULL); 00391 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00392 free(max_age_ptr); 00393 max_age_ptr = NULL; 00394 00395 val = 0xffffffffffff; 00396 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00397 CHECK(max_age_ptr != NULL); 00398 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00399 free(max_age_ptr); 00400 max_age_ptr = NULL; 00401 00402 val = 0xffffffffffffff; 00403 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00404 CHECK(max_age_ptr != NULL); 00405 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00406 free(max_age_ptr); 00407 max_age_ptr = NULL; 00408 00409 val = 0xffff; 00410 max_age_ptr = m2m::String::convert_integer_to_array(val,max_age_len); 00411 CHECK(max_age_ptr != NULL); 00412 CHECK(val == m2m::String::convert_array_to_integer(max_age_ptr, max_age_len)); 00413 free(max_age_ptr); 00414 max_age_ptr = NULL; 00415 00416 00417 00418 } 00419 00420 00421
Generated on Tue Jul 12 2022 19:07:00 by
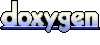