
This is an example of BLE GATT Client, which receives broadcast data from BLE_Server_BME280 ( a GATT server) , then transfers values up to mbed Device Connector (cloud).
test_m2mconnectionhandlerimpl_classic.cpp
00001 /* 00002 * Copyright (c) 2016 ARM Limited. All rights reserved. 00003 * SPDX-License-Identifier: Apache-2.0 00004 * Licensed under the Apache License, Version 2.0 (the License); you may 00005 * not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an AS IS BASIS, WITHOUT 00012 * WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "CppUTest/TestHarness.h" 00017 #include "test_m2mconnectionhandlerimpl_classic.h" 00018 #include "m2mconnectionhandlerpimpl_stub.h" 00019 #include "m2mconnectionobserver.h" 00020 00021 class M2MConnection_TestObserver : public M2MConnectionObserver { 00022 00023 public: 00024 M2MConnection_TestObserver(){} 00025 00026 virtual ~M2MConnection_TestObserver(){} 00027 00028 void set_class_object(M2MConnectionHandler *impl) {} 00029 void data_available(uint8_t*, 00030 uint16_t, 00031 const M2MConnectionObserver::SocketAddress &){ 00032 dataAvailable = true; 00033 } 00034 00035 void socket_error(uint8_t error_code, bool retry){error = true;} 00036 00037 void address_ready(const M2MConnectionObserver::SocketAddress &, 00038 M2MConnectionObserver::ServerType, 00039 const uint16_t){addressReady = true;} 00040 00041 void data_sent(){dataSent = true;} 00042 00043 bool dataAvailable; 00044 bool error; 00045 bool addressReady; 00046 bool dataSent; 00047 }; 00048 00049 Test_M2MConnectionHandler_classic::Test_M2MConnectionHandler_classic() 00050 { 00051 observer = new M2MConnection_TestObserver(); 00052 handler = new M2MConnectionHandler(*observer, NULL ,M2MInterface::NOT_SET,M2MInterface::Uninitialized); 00053 } 00054 00055 Test_M2MConnectionHandler_classic::~Test_M2MConnectionHandler_classic() 00056 { 00057 delete handler; 00058 delete observer; 00059 } 00060 00061 void Test_M2MConnectionHandler_classic::test_bind_connection() 00062 { 00063 m2mconnectionhandlerpimpl_stub::bool_value = true; 00064 CHECK( handler->bind_connection(7) == true); 00065 } 00066 00067 void Test_M2MConnectionHandler_classic::test_resolve_server_address() 00068 { 00069 m2mconnectionhandlerpimpl_stub::bool_value = true; 00070 CHECK(handler->resolve_server_address("10", 7, M2MConnectionObserver::LWM2MServer, NULL) == true); 00071 00072 CHECK(handler->resolve_server_address("10", 7, M2MConnectionObserver::LWM2MServer, NULL) == true); 00073 00074 m2mconnectionhandlerpimpl_stub::bool_value = false; 00075 CHECK(handler->resolve_server_address("10", 7, M2MConnectionObserver::LWM2MServer, NULL) == false); 00076 } 00077 00078 void Test_M2MConnectionHandler_classic::test_send_data() 00079 { 00080 sn_nsdl_addr_s* addr = (sn_nsdl_addr_s*)malloc(sizeof(sn_nsdl_addr_s)); 00081 00082 memset(addr, 0, sizeof(sn_nsdl_addr_s)); 00083 m2mconnectionhandlerpimpl_stub::bool_value = false; 00084 CHECK(false == handler->send_data(NULL, 0 , NULL)); 00085 00086 m2mconnectionhandlerpimpl_stub::bool_value = true; 00087 CHECK(true == handler->send_data(NULL, 0 , addr)); 00088 00089 m2mconnectionhandlerpimpl_stub::bool_value = false; 00090 CHECK(false == handler->send_data(NULL, 0 , addr)); 00091 00092 free(addr); 00093 } 00094 00095 void Test_M2MConnectionHandler_classic::test_start_listening_for_data() 00096 { 00097 handler->start_listening_for_data(); 00098 } 00099 00100 00101 00102 void Test_M2MConnectionHandler_classic::test_stop_listening() 00103 { 00104 00105 handler->stop_listening(); 00106 00107 } 00108 00109 void Test_M2MConnectionHandler_classic::test_send_to_socket() 00110 { 00111 const char buf[] = "hello"; 00112 handler->send_to_socket((unsigned char *)&buf, 5); 00113 } 00114 00115 void Test_M2MConnectionHandler_classic::test_receive_from_socket() 00116 { 00117 unsigned char *buf = (unsigned char *)malloc(6); 00118 handler->receive_from_socket(buf, 5); 00119 free(buf); 00120 } 00121 00122 void Test_M2MConnectionHandler_classic::test_handle_connection_error() 00123 { 00124 // To manage code coverage 00125 handler->handle_connection_error(5); 00126 } 00127 00128 void Test_M2MConnectionHandler_classic::test_set_platform_network_handler() 00129 { 00130 // To manage code coverage 00131 handler->set_platform_network_handler(NULL); 00132 } 00133 00134 void Test_M2MConnectionHandler_classic::test_claim_mutex() 00135 { 00136 // To manage code coverage 00137 handler->claim_mutex(); 00138 } 00139 00140 void Test_M2MConnectionHandler_classic::test_release_mutex() 00141 { 00142 // To manage code coverage 00143 handler->release_mutex(); 00144 }
Generated on Tue Jul 12 2022 19:07:00 by
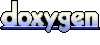