A project to implement a console using the Mbed using VGA for video output and a PS/2 keyboard for the input. The eventual goal is to also include tools for managing SD cards, and a semi-self-hosting programming environment.
Dependencies: PS2_MbedConsole fastlib SDFileSystem vga640x480g_mbedconsole lightvm mbed
shell.cpp
00001 /* 00002 <Copyright Header> 00003 Copyright (c) 2012 Jordan "Earlz" Earls <http://lastyearswishes.com> 00004 All rights reserved. 00005 00006 Redistribution and use in source and binary forms, with or without 00007 modification, are permitted provided that the following conditions 00008 are met: 00009 00010 1. Redistributions of source code must retain the above copyright 00011 notice, this list of conditions and the following disclaimer. 00012 2. Redistributions in binary form must reproduce the above copyright 00013 notice, this list of conditions and the following disclaimer in the 00014 documentation and/or other materials provided with the distribution. 00015 3. The name of the author may not be used to endorse or promote products 00016 derived from this software without specific prior written permission. 00017 00018 THIS SOFTWARE IS PROVIDED ``AS IS'' AND ANY EXPRESS OR IMPLIED WARRANTIES, 00019 INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY 00020 AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL 00021 THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00022 EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, 00023 PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; 00024 OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 00025 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR 00026 OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF 00027 ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00028 00029 This file is part of the MbedConsole project 00030 */ 00031 00032 #include "mbedconsole.h" 00033 #include "plEarlz.h" 00034 #include "PS2Keyboard.h" 00035 00036 #include "SDFileSystem.h" 00037 00038 char kbd_GetKey(); 00039 00040 LocalFileSystem local("local"); 00041 00042 /* 00043 void do_hackaday() 00044 { 00045 // int y=150; 00046 int tmp=0; 00047 for(int y=0;y<HACKADAY_HEIGHT;y++) 00048 { 00049 for(int x=0;x<HACKADAY_WIDTH;x++) 00050 { 00051 int byte=tmp/8; 00052 int bit=tmp%8; 00053 bit=bit-7; //bits are reversed! Those bastards! 00054 bit=-bit; 00055 vga_plot(x,y, (hackadaylogo[byte]&(1<<bit))==0); 00056 tmp++; 00057 } 00058 } 00059 } 00060 */ 00061 void shell_begin(){ 00062 //do_hackaday(); 00063 vputs(">>Micro eMBEDded Shell v0.1<<\n"); 00064 char *cmd=(char*)malloc(128); 00065 bool valid=false; 00066 while(1){ 00067 vputs("> "); 00068 vgetsl(cmd, 128); 00069 vputc('\n'); 00070 valid=false; 00071 if(strlcmp(cmd, "help", 5)==0){ 00072 valid=true; 00073 vputs("Command list:\n"); 00074 vputs("help -- this text \n"); 00075 vputs("cls -- clear the screen\n"); 00076 vputs("testX -- test (where X is number) performs tests\n"); 00077 vputs("reboot -- resets the processor\n"); 00078 vputs("about -- prints text about how we got to here\n"); 00079 vputs("plearlz -- enter the PLEarlz Forth shell\n"); 00080 }else if(strlcmp(cmd,"cls",4)==0){ 00081 valid=true; 00082 vga_cls(); 00083 vsetcursor(0,0); 00084 }else if(strlcmp(cmd,"test", 5)==0){ 00085 valid=true; 00086 vputs("Opening File...Screen may flicker!\n"); // Drive should be marked as removed 00087 wait(5); 00088 FILE *fp = fopen("/local/test.txt", "w"); 00089 if(!fp) { 00090 vputs("File /local/test.txt could not be opened!\n"); 00091 exit(1); 00092 } 00093 00094 wait(5.0); 00095 00096 vputs("Writing Data...\n"); 00097 fprintf(fp, "Hello World!"); 00098 00099 wait(5.0); 00100 00101 vputs("Closing File...\n"); 00102 fclose(fp); 00103 00104 // Drive should be restored. this is the same as just returning from main 00105 wait(5); 00106 }else if(strlcmp(cmd, "test2", 6)==0){ 00107 while(1){ 00108 vputc(kbd_GetKey()); 00109 } 00110 }else if(strlcmp(cmd, "test3", 6) == 0) 00111 { 00112 //SDFileSystem sdcard(p11, p12, p13, p20, "sd"); 00113 FILE *fp = fopen("/sd/sdtest.txt", "w"); 00114 if(fp == NULL) { 00115 vputs("Could not open file for write\n"); 00116 } 00117 fprintf(fp, "Hello fun SD Card World!"); 00118 fclose(fp); 00119 }else if(strlcmp(cmd, "format", 7) == 0) 00120 { 00121 if(sdcard->format()!=0) 00122 { 00123 vputs("Error formatting :("); 00124 } 00125 }else if(strlcmp(cmd, "plearlz", 8)==0){ 00126 valid=1; 00127 pl_shell(); 00128 }else if(strlcmp(cmd,"reboot", 7)==0){ 00129 valid=1; 00130 NVIC_SystemReset(); 00131 }else if(strlcmp(cmd, "about", 6)==0){ 00132 valid=1; 00133 vputs("I am Jack's broken monolog\n"); 00134 }else if(strlcmp(cmd, "scheme", 7)==0){ 00135 valid=1; 00136 //scheme_main(); 00137 } 00138 if(!valid){ 00139 vputs("Invalid Command! Try `help` if you need it\n"); 00140 } 00141 } 00142 } 00143 00144 00145
Generated on Sun Jul 17 2022 09:10:09 by
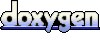