Serial output logger based on the LoggerInterface
Fork of LogIt by
null_logger.h
00001 #pragma once 00002 00003 #include "LoggerInterface.h" 00004 00005 // NullLogger logs nothing but it allows us to inject other logger when necessary. 00006 // With NullLogger we dont have to check if logger is set to actual logger. 00007 // Kudos to Sandi Metz and her seminar on how to avoid if statements 00008 00009 // Source: http://stackoverflow.com/questions/1008019/c-singleton-design-pattern 00010 namespace LogIt { 00011 00012 class NullLogger : public Log::LoggerInterface 00013 { 00014 public: 00015 static NullLogger * get_instance() 00016 { 00017 static NullLogger instance; // Guaranteed to be destroyed. 00018 // Instantiated on first use. 00019 return &instance; 00020 } 00021 00022 private: 00023 NullLogger() {} // Constructor? (the {} brackets) are needed here. 00024 00025 // C++ 03 00026 // ======== 00027 // Dont forget to declare these two. You want to make sure they 00028 // are unacceptable otherwise you may accidentally get copies of 00029 // your singleton appearing. 00030 NullLogger(NullLogger const&); // Don't Implement 00031 void operator=(NullLogger const&); // Don't implement 00032 00033 // C++ 11 00034 // ======= 00035 // We can use the better technique of deleting the methods 00036 // we don't want. 00037 public: 00038 // NullLogger(NullLogger const&) = delete; 00039 // void operator=(NullLogger const&) = delete; 00040 00041 // Note: Scott Meyers mentions in his Effective Modern 00042 // C++ book, that deleted functions should generally 00043 // be public as it results in better error messages 00044 // due to the compilers behavior to check accessibility 00045 // before deleted status 00046 00047 public: 00048 void emergency(const char * message, ...) {}; 00049 void alert(const char * message, ...) {}; 00050 void critical(const char * message, ...) {}; 00051 void error(const char * message, ...) {}; 00052 void warning(const char * message, ...) {}; 00053 void notice(const char * message, ...) {}; 00054 void info(const char * message, ...) {}; 00055 void debug(const char * message, ...) {}; 00056 void log(Level level, const char * message, ...) {}; 00057 }; 00058 00059 };
Generated on Thu Jul 14 2022 14:54:23 by
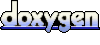