My controller identifies as an ILI9328, but only works if initialised as an ILI9325. This fork includes a fix to force 9325 initialization when a 9328 is detected.
Dependents: TouchScreenCalibrate TouchScreenGUIDemo
Fork of UniGraphic by
TextDisplay.h
00001 /* mbed TextDisplay Library Base Class 00002 * Copyright (c) 2007-2009 sford 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * A common base class for Text displays 00006 * To port a new display, derive from this class and implement 00007 * the constructor (setup the display), character (put a character 00008 * at a location), rows and columns (number of rows/cols) functions. 00009 * Everything else (locate, printf, putc, cls) will come for free 00010 * 00011 * The model is the display will wrap at the right and bottom, so you can 00012 * keep writing and will always get valid characters. The location is 00013 * maintained internally to the class to make this easy 00014 */ 00015 00016 #ifndef MBED_TEXTDISPLAY_H 00017 #define MBED_TEXTDISPLAY_H 00018 00019 #include "mbed.h" 00020 #include "Stream.h" 00021 00022 /** A common base class for Text displays 00023 */ 00024 class TextDisplay : public Stream { 00025 public: 00026 00027 // functions needing implementation in derived implementation class 00028 // ---------------------------------------------------------------- 00029 /** Create a TextDisplay interface 00030 * @param name The name used in the path to access the strean through the filesystem 00031 */ 00032 TextDisplay(const char *name = NULL); 00033 00034 /** output a character at the given position 00035 * 00036 * @param column column where charater must be written 00037 * @param row where character must be written 00038 * @param c the character to be written to the TextDisplay 00039 * @note this method may be overridden in a derived class. 00040 */ 00041 virtual void character(int column, int row, int c) = 0; 00042 00043 /** return number of rows on TextDisplay 00044 * @result number of rows 00045 * @note this method must be supported in the derived class. 00046 */ 00047 virtual int rows() = 0; 00048 00049 /** return number of columns on TextDisplay 00050 * @result number of columns 00051 * @note this method must be supported in the derived class. 00052 */ 00053 virtual int columns() = 0; 00054 00055 // functions that come for free, but can be overwritten 00056 // ---------------------------------------------------- 00057 /** redirect output from a stream (stoud, sterr) to display 00058 * @param stream stream that shall be redirected to the TextDisplay 00059 * @note this method may be overridden in a derived class. 00060 * @returns true if the claim succeeded. 00061 */ 00062 virtual bool claim (FILE *stream); 00063 00064 /** clear the entire screen 00065 * @note this method may be overridden in a derived class. 00066 */ 00067 virtual void cls(); 00068 00069 /** locate the cursor at a character position. 00070 * Based on the currently active font, locate the cursor on screen. 00071 * @note this method may be overridden in a derived class. 00072 * @param column is the horizontal offset from the left side. 00073 * @param row is the vertical offset from the top. 00074 */ 00075 virtual void locate(int column, int row); 00076 00077 /** set the foreground color 00078 * @note this method may be overridden in a derived class. 00079 * @param color is color to use for foreground drawing. 00080 */ 00081 virtual void setForeground(uint16_t colour); 00082 00083 /** set the background color 00084 * @note this method may be overridden in a derived class. 00085 * @param color is color to use for background drawing. 00086 */ 00087 virtual void setBackground(uint16_t colour); 00088 virtual uint16_t getForeground(); 00089 virtual uint16_t getBackground(); 00090 00091 // putc (from Stream) 00092 // printf (from Stream) 00093 00094 protected: 00095 00096 virtual int _putc(int value); 00097 virtual int _getc(); 00098 00099 // character location 00100 int _column; 00101 int _row; 00102 00103 // colours 00104 volatile uint16_t _foreground; 00105 volatile uint16_t _background; 00106 char *_path; 00107 }; 00108 00109 #endif
Generated on Wed Jul 13 2022 01:53:44 by
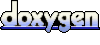