
simple typeABZ test with continuous carrier option
Dependencies: SX127x LPS22HB sx12xx_hal
main.cpp
00001 #include "radio.h" 00002 #include "LPS22HBSensor.h" 00003 00004 #ifndef TARGET_DISCO_L072CZ_LRWAN1 00005 #error only_for_L072CZ_discovery 00006 #endif 00007 00008 #define BW_KHZ 125 00009 #define SPREADING_FACTOR 7 00010 #define CF_HZ 915000000 00011 #define TX_DBM 20 00012 00013 /* 00014 DevI2C devI2c(I2C_SDA, I2C_SCL); 00015 LPS22HBSensor press_temp(&devI2c); 00016 */ 00017 AnalogIn pa0(PA_0); 00018 DigitalIn test_in_pin(PB_5, PullDown); 00019 DigitalOut ext_led(PB_2); 00020 #define EXT_LED_ON 0 00021 #define EXT_LED_OFF 1 00022 00023 00024 /**********************************************************************/ 00025 volatile bool txDone; 00026 00027 void txDoneCB() 00028 { 00029 txDone = true; 00030 } 00031 00032 void rxDoneCB(uint8_t size, float rssi, float snr) 00033 { 00034 } 00035 00036 const RadioEvents_t rev = { 00037 /* Dio0_top_half */ NULL, 00038 /* TxDone_topHalf */ NULL, 00039 /* TxDone_botHalf */ txDoneCB, 00040 /* TxTimeout */ NULL, 00041 /* RxDone */ rxDoneCB, 00042 /* RxTimeout */ NULL, 00043 /* RxError */ NULL, 00044 /* FhssChangeChannel */NULL, 00045 /* CadDone */ NULL 00046 }; 00047 00048 int main() 00049 { 00050 printf("\r\nreset-tx "); 00051 00052 Radio::Init(&rev); 00053 00054 Radio::Standby(); 00055 Radio::LoRaModemConfig(BW_KHZ, SPREADING_FACTOR, 1); 00056 Radio::SetChannel(CF_HZ); 00057 00058 Radio::set_tx_dbm(TX_DBM); 00059 00060 // preambleLen, fixLen, crcOn, invIQ 00061 Radio::LoRaPacketConfig(8, false, true, false); 00062 00063 for (;;) { 00064 00065 uint16_t samp = pa0.read_u16(); 00066 if (test_in_pin) { 00067 ext_led = EXT_LED_ON; 00068 printf("continuous-tx\r\n"); 00069 #ifdef SX127x_H 00070 Radio::radio.set_opmode(RF_OPMODE_SLEEP); 00071 Radio::fsk.enable(false); 00072 Radio::radio.write_u16(REG_FSK_FDEVMSB, 0); 00073 Radio::radio.write_u16(REG_FSK_PREAMBLEMSB, 0xffff); 00074 Radio::fsk.start_tx(8); 00075 #else 00076 #error tx_carrier 00077 #endif /* SX127x_H*/ 00078 } 00079 00080 ext_led = EXT_LED_ON; 00081 Radio::radio.tx_buf[0] = samp; 00082 samp >>= 8; 00083 Radio::radio.tx_buf[1] = samp; 00084 txDone = false; 00085 Radio::Send(2, 0, 0, 0); /* begin transmission */ 00086 00087 printf("Sent %04x\r\n", samp); 00088 while (!txDone) { 00089 Radio::service(); 00090 } 00091 printf("got-tx-done\r\n"); 00092 ext_led = EXT_LED_OFF; 00093 00094 wait(1.0); /* throttle sending rate */ 00095 } 00096 } 00097
Generated on Mon Aug 22 2022 12:34:00 by
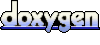