
point-2-point demo
Embed:
(wiki syntax)
Show/hide line numbers
trigger_digital.cpp
00001 #include "main.h" 00002 #ifdef DIGITAL_TRIGGER 00003 00004 Ticker ticker; 00005 InterruptIn user_button(USER_BUTTON); 00006 00007 DigitalOut jumper_out(PC_10); 00008 InterruptIn jumper_in(PC_12); 00009 volatile bool start_tx; 00010 00011 volatile bool jin; 00012 uint8_t out_pin_state; 00013 00014 void button_isr() 00015 { 00016 if (!jumper_in.read()) 00017 start_tx = true; 00018 } 00019 00020 void auto_tx() 00021 { 00022 if (jumper_in.read()) 00023 start_tx = true; 00024 else 00025 ticker.detach(); 00026 } 00027 00028 void trigger_init() 00029 { 00030 jin = false; 00031 00032 jumper_out = 1; 00033 jumper_in.mode(PullDown); 00034 00035 while (!user_button) { 00036 printf("button-lo\r\n"); 00037 wait(0.01); 00038 } 00039 user_button.fall(&button_isr); 00040 } 00041 00042 void trigger_mainloop() 00043 { 00044 if (jumper_in.read()) { 00045 if (!jin) { 00046 ticker.attach(auto_tx, 0.5); 00047 jin = true; 00048 } 00049 } else { 00050 jin = false; 00051 } 00052 00053 if (start_tx) { 00054 start_tx = false; 00055 00056 uint8_t buf[2]; 00057 out_pin_state ^= 1; 00058 buf[0] = CMD_OUT_PIN; 00059 buf[1] = out_pin_state; 00060 radio_tx(buf, 2); 00061 } 00062 } 00063 00064 #endif /* DIGITAL_TRIGGER */ 00065
Generated on Thu Jul 14 2022 21:36:36 by
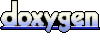