
operate LoRa radio over I2C
Dependencies: TimeoutAbs lib_i2c_slave_block sx12xx_hal
device_sx128x.cpp
00001 #include "radio_device.h" 00002 #ifdef SX128x_H 00003 void get_opmode() 00004 { 00005 status_t status; 00006 Radio::radio.xfer(OPCODE_GET_STATUS, 0, 1, &status.octet); 00007 00008 switch (status.bits.chipMode) { 00009 case 2: // STBY_RC 00010 case 3: // STBY_XOSC 00011 irq.buf[1] = OPMODE_STANDBY; 00012 break; 00013 case 4: // FS 00014 irq.buf[1] = OPMODE_FS; 00015 break; 00016 case 5: // RX 00017 irq.buf[1] = OPMODE_RX; 00018 break; 00019 case 6: // TX 00020 irq.buf[1] = OPMODE_TX; 00021 break; 00022 default: 00023 irq.buf[1] = OPMODE_FAIL; 00024 break; 00025 } 00026 00027 irq.fields.flags.irq_type = IRQ_TYPE_OPMODE; 00028 irqOutPin = 1; 00029 } 00030 00031 void get_lora_packet() 00032 { 00033 LoRaLrCtl_t LoRaLrCtl; 00034 LoRaPktPar1_t LoRaPktPar1; 00035 //LoRaPacketConfig(unsigned preambleLen, bool fixLen, bool crcOn, bool invIQ) 00036 LoRaPreambleReg_t LoRaPreambleReg; 00037 LoRaPreambleReg.octet = Radio::radio.readReg(REG_ADDR_LORA_PREAMBLE, 1); 00038 uint32_t val = (1 << LoRaPreambleReg.bits.preamble_symb_nb_exp) * LoRaPreambleReg.bits.preamble_symb1_nb; 00039 irq.buf[1] = val & 0xff; 00040 val >>= 8; 00041 irq.buf[2] = val & 0xff; 00042 00043 LoRaPktPar1.octet = Radio::radio.readReg(REG_ADDR_LORA_PKTPAR1, 1); 00044 irq.buf[3] = LoRaPktPar1.bits.implicit_header; 00045 irq.buf[4] = LoRaPktPar1.bits.rxinvert_iq ? 0 : 1; // std is 0 00046 00047 LoRaLrCtl.octet = Radio::radio.readReg(REG_ADDR_LORA_LRCTL, 1); 00048 irq.buf[5] = LoRaLrCtl.bits.crc_en; 00049 00050 irq.fields.flags.irq_type = IRQ_TYPE_LORA_PKT; 00051 irqOutPin = 1; 00052 } 00053 00054 static const unsigned lora_bws[] = { 00055 50, // 0 00056 100, // 1 00057 200, // 2 00058 400, // 3 00059 800, // 4 00060 1600 // 5 00061 }; 00062 00063 void get_lora_modem() 00064 { 00065 uint16_t khz; 00066 LoRaPktPar1_t LoRaPktPar1; 00067 LoRaPktPar0_t LoRaPktPar0; 00068 LoRaPktPar0.octet = Radio::radio.readReg(REG_ADDR_LORA_PKTPAR0, 1); 00069 00070 khz = lora_bws[LoRaPktPar0.bits.modem_bw]; 00071 irq.buf[1] = khz & 0xff; 00072 khz >>= 8; 00073 irq.buf[2] = khz & 0xff; 00074 00075 //LoRaModemConfig(unsigned KHz, unsigned sf, unsigned cr) 00076 irq.buf[3] = LoRaPktPar0.bits.modem_sf; 00077 00078 LoRaPktPar1.octet = Radio::radio.readReg(REG_ADDR_LORA_PKTPAR1, 1); 00079 irq.buf[4] = LoRaPktPar1.bits.coding_rate; 00080 00081 irq.fields.flags.irq_type = IRQ_TYPE_LORA_MODEM; 00082 irqOutPin = 1; 00083 } 00084 00085 #define TX_PWR_OFFSET 18 00086 void get_tx_dbm() 00087 { 00088 PaPwrCtrl_t PaPwrCtrl; 00089 00090 PaPwrCtrl.octet = Radio::radio.readReg(REG_ADDR_PA_PWR_CTRL, 1); 00091 irq.buf[1] = PaPwrCtrl.bits.tx_pwr - TX_PWR_OFFSET; 00092 irq.fields.flags.irq_type = IRQ_TYPE_TXDBM; 00093 irqOutPin = 1; 00094 } 00095 00096 void get_fsk_sync() 00097 { 00098 /* TODO 3 separate sync words */ 00099 00100 irq.fields.flags.irq_type = IRQ_TYPE_FSK_SYNC; 00101 irqOutPin = 1; 00102 } 00103 00104 void get_fsk_modem() 00105 { 00106 uint32_t u32; 00107 // GFSKModemConfig(unsigned bps, unsigned bwKHz, unsigned fdev_hz) 00108 00109 /* given in bitrate-bandwith combination */ 00110 u32 = 0; // TODO bitrate 00111 irq.buf[1] = u32 & 0xff; 00112 u32 >>= 8; 00113 irq.buf[2] = u32 & 0xff; 00114 u32 >>= 8; 00115 irq.buf[3] = u32 & 0xff; 00116 u32 >>= 8; 00117 irq.buf[4] = u32 & 0xff; 00118 00119 00120 u32 = 0; // TODO bandwidth 00121 irq.buf[5] = u32 & 0xff; 00122 u32 >>= 8; 00123 irq.buf[6] = u32 & 0xff; 00124 00125 u32 = 0; // TODO fdev mod index + bitrate 00126 irq.buf[7] = u32 & 0xff; 00127 u32 >>= 8; 00128 irq.buf[8] = u32 & 0xff; 00129 u32 >>= 8; 00130 irq.buf[9] = u32 & 0xff; 00131 u32 >>= 8; 00132 irq.buf[10] = u32 & 0xff; 00133 00134 irq.fields.flags.irq_type = IRQ_TYPE_FSK_MODEM; 00135 irqOutPin = 1; 00136 } 00137 00138 static const uint8_t fsk_pbl_lens[] = { 4, 8, 12, 16, 20, 24, 28, 32 }; 00139 00140 void get_fsk_packet() 00141 { 00142 PktBitStreamCtrl_t PktBitStreamCtrl; 00143 PktCtrl0_t PktCtrl0; 00144 // GFSKPacketConfig(unsigned preambleLen, bool fixLen, bool crcOn) 00145 PktCtrl1_t PktCtrl1; 00146 PktCtrl1.octet = Radio::radio.readReg(REG_ADDR_PKTCTRL1, 1); 00147 irq.buf[1] = fsk_pbl_lens[PktCtrl1.gfsk.preamble_len]; 00148 irq.buf[2] = 0; 00149 00150 PktCtrl0.octet = Radio::radio.readReg(REG_ADDR_PKTCTRL0, 1); 00151 irq.buf[3] = PktCtrl0.bits.pkt_len_format; // true = fixed 00152 00153 PktBitStreamCtrl.octet = Radio::radio.readReg(REG_ADDR_PKT_BITSTREAM_CTRL, 1); 00154 irq.buf[4] = PktBitStreamCtrl.bits.crc_mode; 00155 00156 irq.fields.flags.irq_type = IRQ_TYPE_FSK_PKT; 00157 irqOutPin = 1; 00158 } 00159 00160 void radio_reset() 00161 { 00162 Radio::radio.hw_reset(); 00163 } 00164 00165 void radio_device_init() 00166 { 00167 } 00168 00169 #endif /* ..SX128x_H */ 00170
Generated on Tue Jul 12 2022 23:07:48 by
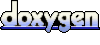