
UART console application for testing SX1272/SX1276
Embed:
(wiki syntax)
Show/hide line numbers
kermit.h
00001 #if 0 00002 #include "mbed.h" 00003 00004 #define RADIO_FILE_XFER 00005 00006 #ifdef RADIO_FILE_XFER 00007 #include "sx127x_lora.h" 00008 #endif /* RADIO_FILE_XFER */ 00009 00010 00011 #define SOH 0x01 00012 #define NAK 0x15 00013 #define CAN 0x18 00014 00015 typedef enum { 00016 KERMIT_STATE_OFF = 0, 00017 KERMIT_STATE_WAIT_SOH, 00018 KERMIT_STATE_WAIT_LEN, 00019 KERMIT_STATE_WAIT_SEQ, 00020 KERMIT_STATE_WAIT_TYPE, 00021 KERMIT_STATE_DATA, 00022 KERMIT_STATE_GET_EOL, 00023 } kermit_state_e; 00024 00025 00026 class Kermit 00027 { 00028 public: 00029 Kermit(SX127x_lora& _lora); 00030 ~Kermit(); 00031 00032 bool uart_rx_enabled; 00033 bool got_send_init; 00034 bool end; 00035 uint8_t end_cause; 00036 uint32_t total_file_bytes; 00037 SX127x_lora& lora; 00038 char filename[128]; 00039 00040 uint32_t bin_data_u32[128]; 00041 uint8_t* bin_data; 00042 uint32_t bin_data_idx; 00043 00044 uint8_t maxl; 00045 uint8_t time; 00046 uint8_t npad; 00047 char padc; 00048 char eol; 00049 char qctl; // verbatim quote char 00050 char qbin; 00051 char chkt; 00052 char rept; 00053 00054 void rx_callback(uint8_t); 00055 void uart_rx_enable(void); 00056 void service(void); 00057 uint32_t _HAL_CRC_Calculate(uint32_t u32_buf[], uint32_t Size); 00058 void test_crc(void); 00059 00060 protected: 00061 bool show_error; 00062 bool end_after_tx; 00063 kermit_state_e state; 00064 void kermit_uart_tx(void); 00065 int parse_rx(void); 00066 void radio_xfer_rx(void); 00067 00068 uint8_t tochar(uint8_t c); 00069 uint8_t unchar(uint8_t c); 00070 uint8_t ctl(uint8_t c); 00071 00072 uint32_t uart_rx_sum; 00073 uint8_t uart_rx_data[128]; 00074 uint8_t uart_rx_data_idx; 00075 uint8_t uart_rx_length; 00076 char uart_rx_seq; 00077 char uart_rx_type; 00078 00079 float uart_tx_sleep; 00080 uint8_t uart_tx_data[128]; 00081 uint8_t uart_tx_data_idx; 00082 bool uart_do_tx; 00083 }; 00084 #endif /* #if 0 */
Generated on Sat Jul 16 2022 01:04:54 by
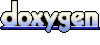