
test sending sensor results over lora radio. Accelerometer and temp/pressure.
Embed:
(wiki syntax)
Show/hide line numbers
LPS22HH_Driver.h
00001 /* 00002 ****************************************************************************** 00003 * @file lps22hh_reg.h 00004 * @author MEMS Software Solution Team 00005 * @date 14-December-2017 00006 * @brief This file contains all the functions prototypes for the 00007 * lps22hh_reg.c driver. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __LPS22HH_DRIVER__H 00039 #define __LPS22HH_DRIVER__H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include <stdint.h> 00047 00048 /** @addtogroup lps22hh 00049 * @{ 00050 */ 00051 00052 #ifndef __MEMS_SHARED__TYPES 00053 #define __MEMS_SHARED__TYPES 00054 00055 /** @defgroup ST_MEMS_common_types 00056 * @{ 00057 */ 00058 00059 typedef union 00060 { 00061 int16_t i16bit[3]; 00062 uint8_t u8bit[6]; 00063 } axis3bit16_t; 00064 00065 typedef union 00066 { 00067 int16_t i16bit; 00068 uint8_t u8bit[2]; 00069 } axis1bit16_t; 00070 00071 typedef union 00072 { 00073 int32_t i32bit[3]; 00074 uint8_t u8bit[12]; 00075 } axis3bit32_t; 00076 00077 typedef union 00078 { 00079 int32_t i32bit; 00080 uint8_t u8bit[4]; 00081 } axis1bit32_t; 00082 00083 typedef struct 00084 { 00085 uint8_t bit0 : 1; 00086 uint8_t bit1 : 1; 00087 uint8_t bit2 : 1; 00088 uint8_t bit3 : 1; 00089 uint8_t bit4 : 1; 00090 uint8_t bit5 : 1; 00091 uint8_t bit6 : 1; 00092 uint8_t bit7 : 1; 00093 } bitwise_t; 00094 00095 #define PROPERTY_DISABLE (0) 00096 #define PROPERTY_ENABLE (1) 00097 00098 #endif /*__MEMS_SHARED__TYPES*/ 00099 00100 /** 00101 * @} 00102 */ 00103 00104 /** @defgroup lps22hh_interface 00105 * @{ 00106 */ 00107 00108 typedef uint8_t (*lps22hh_write_ptr)(void *, uint8_t, uint8_t *, uint16_t); 00109 typedef uint8_t (*lps22hh_read_ptr)(void *, uint8_t, uint8_t *, uint16_t); 00110 00111 typedef struct 00112 { 00113 /** Component mandatory fields **/ 00114 lps22hh_write_ptr write_reg; 00115 lps22hh_read_ptr read_reg; 00116 /** Customizable optional pointer **/ 00117 void *handle; 00118 } lps22hh_ctx_t; 00119 00120 /** 00121 * @} 00122 */ 00123 00124 00125 /** @defgroup lps22hh_Infos 00126 * @{ 00127 */ 00128 /** I2C Device Address 8 bit format if SA0=0 -> B9 if SA0=1 -> BB **/ 00129 #define LPS22HH_I2C_ADD_H 0xBB 00130 #define LPS22HH_I2C_ADD_L 0xB9 00131 00132 /** Device Identification (Who am I) **/ 00133 #define LPS22HH_ID 0xB3 00134 00135 /** 00136 * @} 00137 */ 00138 00139 /** 00140 * @defgroup lps22hh_Sensitivity 00141 * @{ 00142 */ 00143 00144 #define LPS22HH_FROM_LSB_TO_hPa(lsb) (float)( lsb / 4096.0f ) 00145 #define LPS22HH_FROM_LSB_TO_degC(lsb) (float)( lsb / 100.0f ) 00146 00147 /** 00148 * @} 00149 */ 00150 00151 #define LPS22HH_INTERRUPT_CFG 0x0B 00152 typedef struct 00153 { 00154 uint8_t pe : 2; /* ple + phe */ 00155 uint8_t lir : 1; 00156 uint8_t diff_en : 1; 00157 uint8_t reset_az : 1; 00158 uint8_t autozero : 1; 00159 uint8_t reset_arp : 1; 00160 uint8_t autorifp : 1; 00161 } lps22hh_interrupt_cfg_t; 00162 00163 #define LPS22HH_THS_P_L 0x0C 00164 #define LPS22HH_THS_P_H 0x0D 00165 #define LPS22HH_IF_CTRL 0x0E 00166 typedef struct 00167 { 00168 uint8_t i2c_disable : 1; 00169 uint8_t i3c_disable : 1; 00170 uint8_t pd_dis_int1 : 1; 00171 uint8_t sdo_pu_en : 1; 00172 uint8_t sda_pu_en : 1; 00173 uint8_t not_used_01 : 2; 00174 uint8_t int_en_i3c : 1; 00175 } lps22hh_if_ctrl_t; 00176 00177 #define LPS22HH_WHO_AM_I 0x0F 00178 #define LPS22HH_CTRL_REG1 0x10 00179 typedef struct 00180 { 00181 uint8_t sim : 1; 00182 uint8_t bdu : 1; 00183 uint8_t lpfp_cfg : 2; /* en_lpfp + lpfp_cfg */ 00184 uint8_t odr : 3; 00185 uint8_t not_used_01 : 1; 00186 } lps22hh_ctrl_reg1_t; 00187 00188 #define LPS22HH_CTRL_REG2 0x11 00189 typedef struct 00190 { 00191 uint8_t one_shot : 1; 00192 uint8_t low_noise_en : 1; 00193 uint8_t swreset : 1; 00194 uint8_t not_used_01 : 1; 00195 uint8_t if_add_inc : 1; 00196 uint8_t pp_od : 1; 00197 uint8_t int_h_l : 1; 00198 uint8_t boot : 1; 00199 } lps22hh_ctrl_reg2_t; 00200 00201 #define LPS22HH_CTRL_REG3 0x12 00202 typedef struct 00203 { 00204 uint8_t int_s : 2; 00205 uint8_t drdy : 1; 00206 uint8_t f_ovr : 1; 00207 uint8_t f_fth : 1; 00208 uint8_t f_full : 1; 00209 uint8_t not_used_01 : 2; 00210 } lps22hh_ctrl_reg3_t; 00211 00212 #define LPS22HH_FIFO_CTRL 0x13 00213 typedef struct 00214 { 00215 uint8_t f_mode : 3; /* f_mode + trig_modes */ 00216 uint8_t stop_on_fth : 1; 00217 uint8_t not_used_01 : 4; 00218 } lps22hh_fifo_ctrl_t; 00219 00220 #define LPS22HH_FIFO_WTM 0x14 00221 typedef struct 00222 { 00223 uint8_t wtm : 7; 00224 uint8_t not_used_01 : 1; 00225 } lps22hh_fifo_wtm_t; 00226 00227 #define LPS22HH_REF_P_XL 0x15 00228 #define LPS22HH_REF_P_L 0x16 00229 #define LPS22HH_RPDS_L 0x18 00230 #define LPS22HH_RPDS_H 0x19 00231 #define LPS22HH_INT_SOURCE 0x24 00232 typedef struct 00233 { 00234 uint8_t ph : 1; 00235 uint8_t pl : 1; 00236 uint8_t ia : 1; 00237 uint8_t not_used_01 : 5; 00238 } lps22hh_int_source_t; 00239 00240 #define LPS22HH_FIFO_STATUS1 0x25 00241 #define LPS22HH_FIFO_STATUS2 0x26 00242 typedef struct 00243 { 00244 uint8_t not_used_01 : 5; 00245 uint8_t f_full : 1; 00246 uint8_t ovr : 1; 00247 uint8_t fth_fifo : 1; 00248 } lps22hh_fifo_status2_t; 00249 00250 #define LPS22HH_STATUS 0x27 00251 typedef struct 00252 { 00253 uint8_t p_da : 1; 00254 uint8_t t_da : 1; 00255 uint8_t not_used_01 : 2; 00256 uint8_t p_or : 1; 00257 uint8_t t_or : 1; 00258 uint8_t not_used_02 : 2; 00259 } lps22hh_status_t; 00260 00261 #define LPS22HH_PRESSURE_OUT_XL 0x28 00262 #define LPS22HH_PRESSURE_OUT_L 0x29 00263 #define LPS22HH_PRESSURE_OUT_H 0x2A 00264 #define LPS22HH_TEMP_OUT_L 0x2B 00265 #define LPS22HH_TEMP_OUT_H 0x2C 00266 #define LPS22HH_FIFO_DATA_OUT_PRESS_XL 0x78 00267 #define LPS22HH_FIFO_DATA_OUT_PRESS_L 0x79 00268 #define LPS22HH_FIFO_DATA_OUT_PRESS_H 0x7A 00269 #define LPS22HH_FIFO_DATA_OUT_TEMP_L 0x7B 00270 #define LPS22HH_FIFO_DATA_OUT_TEMP_H 0x7C 00271 00272 typedef union 00273 { 00274 lps22hh_interrupt_cfg_t interrupt_cfg; 00275 lps22hh_if_ctrl_t if_ctrl; 00276 lps22hh_ctrl_reg1_t ctrl_reg1; 00277 lps22hh_ctrl_reg2_t ctrl_reg2; 00278 lps22hh_ctrl_reg3_t ctrl_reg3; 00279 lps22hh_fifo_ctrl_t fifo_ctrl; 00280 lps22hh_fifo_wtm_t fifo_wtm; 00281 lps22hh_int_source_t int_source; 00282 lps22hh_fifo_status2_t fifo_status2; 00283 lps22hh_status_t status; 00284 bitwise_t bitwise; 00285 uint8_t byte; 00286 } lps22hh_reg_t; 00287 int32_t lps22hh_read_reg(lps22hh_ctx_t *ctx, uint8_t reg, uint8_t *data, 00288 uint16_t len); 00289 int32_t lps22hh_write_reg(lps22hh_ctx_t *ctx, uint8_t reg, uint8_t *data, 00290 uint16_t len); 00291 00292 int32_t lps22hh_autozero_rst_set(lps22hh_ctx_t *ctx, uint8_t val); 00293 int32_t lps22hh_autozero_rst_get(lps22hh_ctx_t *ctx, uint8_t *val); 00294 00295 int32_t lps22hh_autozero_set(lps22hh_ctx_t *ctx, uint8_t val); 00296 int32_t lps22hh_autozero_get(lps22hh_ctx_t *ctx, uint8_t *val); 00297 00298 int32_t lps22hh_pressure_snap_rst_set(lps22hh_ctx_t *ctx, uint8_t val); 00299 int32_t lps22hh_pressure_snap_rst_get(lps22hh_ctx_t *ctx, uint8_t *val); 00300 00301 int32_t lps22hh_pressure_snap_set(lps22hh_ctx_t *ctx, uint8_t val); 00302 int32_t lps22hh_pressure_snap_get(lps22hh_ctx_t *ctx, uint8_t *val); 00303 00304 int32_t lps22hh_block_data_update_set(lps22hh_ctx_t *ctx, uint8_t val); 00305 int32_t lps22hh_block_data_update_get(lps22hh_ctx_t *ctx, uint8_t *val); 00306 00307 typedef enum 00308 { 00309 LPS22HH_POWER_DOWN = 0x00, 00310 LPS22HH_ONE_SHOOT = 0x08, 00311 LPS22HH_1_Hz = 0x01, 00312 LPS22HH_10_Hz = 0x02, 00313 LPS22HH_25_Hz = 0x03, 00314 LPS22HH_50_Hz = 0x04, 00315 LPS22HH_75_Hz = 0x05, 00316 LPS22HH_1_Hz_LOW_NOISE = 0x11, 00317 LPS22HH_10_Hz_LOW_NOISE = 0x12, 00318 LPS22HH_25_Hz_LOW_NOISE = 0x13, 00319 LPS22HH_50_Hz_LOW_NOISE = 0x14, 00320 LPS22HH_75_Hz_LOW_NOISE = 0x15, 00321 LPS22HH_100_Hz = 0x06, 00322 LPS22HH_200_Hz = 0x07, 00323 } lps22hh_odr_t; 00324 int32_t lps22hh_data_rate_set(lps22hh_ctx_t *ctx, lps22hh_odr_t val); 00325 int32_t lps22hh_data_rate_get(lps22hh_ctx_t *ctx, lps22hh_odr_t *val); 00326 00327 int32_t lps22hh_pressure_ref_set(lps22hh_ctx_t *ctx, uint8_t *buff); 00328 int32_t lps22hh_pressure_ref_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00329 00330 int32_t lps22hh_pressure_offset_set(lps22hh_ctx_t *ctx, uint8_t *buff); 00331 int32_t lps22hh_pressure_offset_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00332 00333 typedef struct 00334 { 00335 lps22hh_int_source_t int_source; 00336 lps22hh_fifo_status2_t fifo_status2; 00337 lps22hh_status_t status; 00338 } lps22hh_all_sources_t; 00339 int32_t lps22hh_all_sources_get(lps22hh_ctx_t *ctx, 00340 lps22hh_all_sources_t *val); 00341 00342 int32_t lps22hh_status_reg_get(lps22hh_ctx_t *ctx, lps22hh_status_t *val); 00343 00344 int32_t lps22hh_press_flag_data_ready_get(lps22hh_ctx_t *ctx, uint8_t *val); 00345 00346 int32_t lps22hh_temp_flag_data_ready_get(lps22hh_ctx_t *ctx, uint8_t *val); 00347 00348 int32_t lps22hh_pressure_raw_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00349 00350 int32_t lps22hh_temperature_raw_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00351 00352 int32_t lps22hh_fifo_pressure_raw_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00353 00354 int32_t lps22hh_fifo_temperature_raw_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00355 00356 int32_t lps22hh_device_id_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00357 00358 int32_t lps22hh_reset_set(lps22hh_ctx_t *ctx, uint8_t val); 00359 int32_t lps22hh_reset_get(lps22hh_ctx_t *ctx, uint8_t *val); 00360 00361 int32_t lps22hh_auto_increment_set(lps22hh_ctx_t *ctx, uint8_t val); 00362 int32_t lps22hh_auto_increment_get(lps22hh_ctx_t *ctx, uint8_t *val); 00363 00364 int32_t lps22hh_boot_set(lps22hh_ctx_t *ctx, uint8_t val); 00365 int32_t lps22hh_boot_get(lps22hh_ctx_t *ctx, uint8_t *val); 00366 00367 typedef enum 00368 { 00369 LPS22HH_LPF_ODR_DIV_2 = 0, 00370 LPS22HH_LPF_ODR_DIV_9 = 2, 00371 LPS22HH_LPF_ODR_DIV_20 = 3, 00372 } lps22hh_lpfp_cfg_t; 00373 int32_t lps22hh_lp_bandwidth_set(lps22hh_ctx_t *ctx, lps22hh_lpfp_cfg_t val); 00374 int32_t lps22hh_lp_bandwidth_get(lps22hh_ctx_t *ctx, lps22hh_lpfp_cfg_t *val); 00375 00376 typedef enum 00377 { 00378 LPS22HH_I2C_ENABLE = 0, 00379 LPS22HH_I2C_DISABLE = 1, 00380 } lps22hh_i2c_disable_t; 00381 int32_t lps22hh_i2c_interface_set(lps22hh_ctx_t *ctx, 00382 lps22hh_i2c_disable_t val); 00383 int32_t lps22hh_i2c_interface_get(lps22hh_ctx_t *ctx, 00384 lps22hh_i2c_disable_t *val); 00385 00386 typedef enum 00387 { 00388 LPS22HH_I3C_ENABLE = 0x00, 00389 LPS22HH_I3C_ENABLE_INT_PIN_ENABLE = 0x10, 00390 LPS22HH_I3C_DISABLE = 0x11, 00391 } lps22hh_i3c_disable_t; 00392 int32_t lps22hh_i3c_interface_set(lps22hh_ctx_t *ctx, 00393 lps22hh_i3c_disable_t val); 00394 int32_t lps22hh_i3c_interface_get(lps22hh_ctx_t *ctx, 00395 lps22hh_i3c_disable_t *val); 00396 00397 typedef enum 00398 { 00399 LPS22HH_PULL_UP_DISCONNECT = 0, 00400 LPS22HH_PULL_UP_CONNECT = 1, 00401 } lps22hh_pu_en_t; 00402 int32_t lps22hh_sdo_sa0_mode_set(lps22hh_ctx_t *ctx, lps22hh_pu_en_t val); 00403 int32_t lps22hh_sdo_sa0_mode_get(lps22hh_ctx_t *ctx, lps22hh_pu_en_t *val); 00404 int32_t lps22hh_sda_mode_set(lps22hh_ctx_t *ctx, lps22hh_pu_en_t val); 00405 int32_t lps22hh_sda_mode_get(lps22hh_ctx_t *ctx, lps22hh_pu_en_t *val); 00406 00407 typedef enum 00408 { 00409 LPS22HH_SPI_4_WIRE = 0, 00410 LPS22HH_SPI_3_WIRE = 1, 00411 } lps22hh_sim_t; 00412 int32_t lps22hh_spi_mode_set(lps22hh_ctx_t *ctx, lps22hh_sim_t val); 00413 int32_t lps22hh_spi_mode_get(lps22hh_ctx_t *ctx, lps22hh_sim_t *val); 00414 00415 typedef enum 00416 { 00417 LPS22HH_INT_PULSED = 0, 00418 LPS22HH_INT_LATCHED = 1, 00419 } lps22hh_lir_t; 00420 int32_t lps22hh_int_notification_set(lps22hh_ctx_t *ctx, lps22hh_lir_t val); 00421 int32_t lps22hh_int_notification_get(lps22hh_ctx_t *ctx, lps22hh_lir_t *val); 00422 00423 typedef enum 00424 { 00425 LPS22HH_PUSH_PULL = 0, 00426 LPS22HH_OPEN_DRAIN = 1, 00427 } lps22hh_pp_od_t; 00428 int32_t lps22hh_pin_mode_set(lps22hh_ctx_t *ctx, lps22hh_pp_od_t val); 00429 int32_t lps22hh_pin_mode_get(lps22hh_ctx_t *ctx, lps22hh_pp_od_t *val); 00430 00431 typedef enum 00432 { 00433 LPS22HH_ACTIVE_HIGH = 0, 00434 LPS22HH_ACTIVE_LOW = 1, 00435 } lps22hh_int_h_l_t; 00436 int32_t lps22hh_pin_polarity_set(lps22hh_ctx_t *ctx, lps22hh_int_h_l_t val); 00437 int32_t lps22hh_pin_polarity_get(lps22hh_ctx_t *ctx, lps22hh_int_h_l_t *val); 00438 00439 int32_t lps22hh_pin_int_route_set(lps22hh_ctx_t *ctx, 00440 lps22hh_ctrl_reg3_t *val); 00441 int32_t lps22hh_pin_int_route_get(lps22hh_ctx_t *ctx, 00442 lps22hh_ctrl_reg3_t *val); 00443 00444 typedef enum 00445 { 00446 LPS22HH_NO_THRESHOLD = 0, 00447 LPS22HH_POSITIVE = 1, 00448 LPS22HH_NEGATIVE = 2, 00449 LPS22HH_BOTH = 3, 00450 } lps22hh_pe_t; 00451 int32_t lps22hh_int_on_threshold_set(lps22hh_ctx_t *ctx, lps22hh_pe_t val); 00452 int32_t lps22hh_int_on_threshold_get(lps22hh_ctx_t *ctx, lps22hh_pe_t *val); 00453 00454 00455 int32_t lps22hh_int_treshold_set(lps22hh_ctx_t *ctx, uint8_t *buff); 00456 int32_t lps22hh_int_treshold_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00457 typedef enum 00458 { 00459 LPS22HH_BYPASS_MODE = 0, 00460 LPS22HH_FIFO_MODE = 1, 00461 LPS22HH_STREAM_MODE = 2, 00462 LPS22HH_DYNAMIC_STREAM_MODE = 3, 00463 LPS22HH_BYPASS_TO_FIFO_MODE = 5, 00464 LPS22HH_BYPASS_TO_STREAM_MODE = 6, 00465 LPS22HH_STREAM_TO_FIFO_MODE = 7, 00466 } lps22hh_f_mode_t; 00467 int32_t lps22hh_fifo_mode_set(lps22hh_ctx_t *ctx, lps22hh_f_mode_t val); 00468 int32_t lps22hh_fifo_mode_get(lps22hh_ctx_t *ctx, lps22hh_f_mode_t *val); 00469 00470 int32_t lps22hh_fifo_stop_on_wtm_set(lps22hh_ctx_t *ctx, uint8_t val); 00471 int32_t lps22hh_fifo_stop_on_wtm_get(lps22hh_ctx_t *ctx, uint8_t *val); 00472 00473 int32_t lps22hh_fifo_watermark_set(lps22hh_ctx_t *ctx, uint8_t val); 00474 int32_t lps22hh_fifo_watermark_get(lps22hh_ctx_t *ctx, uint8_t *val); 00475 00476 int32_t lps22hh_fifo_data_level_get(lps22hh_ctx_t *ctx, uint8_t *buff); 00477 00478 int32_t lps22hh_fifo_src_get(lps22hh_ctx_t *ctx, lps22hh_fifo_status2_t *val); 00479 00480 int32_t lps22hh_fifo_full_flag_get(lps22hh_ctx_t *ctx, uint8_t *val); 00481 00482 int32_t lps22hh_fifo_ovr_flag_get(lps22hh_ctx_t *ctx, uint8_t *val); 00483 00484 int32_t lps22hh_fifo_wtm_flag_get(lps22hh_ctx_t *ctx, uint8_t *val); 00485 00486 /** 00487 * @} 00488 */ 00489 00490 #ifdef __cplusplus 00491 } 00492 #endif 00493 00494 #endif /*__LPS22HH_DRIVER__H */ 00495 00496 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 16:29:49 by
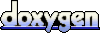