
test sending sensor results over lora radio. Accelerometer and temp/pressure.
Embed:
(wiki syntax)
Show/hide line numbers
LIS2DH12_ACC_driver.h
00001 /******************** (C) COPYRIGHT 2018 STMicroelectronics ******************** 00002 * File Name : LIS2DH12_ACC_driver.h 00003 * Author : MEMS Application Team 00004 * Description : LIS2DH12 Platform Independent Driver - header file 00005 * 00006 ******************************************************************************** 00007 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00008 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE TIME. 00009 * AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY DIRECT, 00010 * INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING FROM THE 00011 * CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE CODING 00012 * INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00013 * 00014 * THIS SOFTWARE IS SPECIFICALLY DESIGNED FOR EXCLUSIVE USE WITH ST PARTS. 00015 *******************************************************************************/ 00016 00017 /* Define to prevent recursive inclusion -------------------------------------*/ 00018 #ifndef __LIS2DH12_DRIVER__H 00019 #define __LIS2DH12_DRIVER__H 00020 00021 /* Includes ------------------------------------------------------------------*/ 00022 #include <stdint.h> 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /* Exported types ------------------------------------------------------------*/ 00029 00030 00031 //these could change accordingly with the architecture 00032 00033 #ifndef __ARCHDEP__TYPES 00034 #define __ARCHDEP__TYPES 00035 00036 typedef unsigned char u8_t; 00037 typedef unsigned short int u16_t; 00038 typedef unsigned int u32_t; 00039 typedef int i32_t; 00040 typedef short int i16_t; 00041 typedef signed char i8_t; 00042 00043 #endif /*__ARCHDEP__TYPES*/ 00044 00045 //define structure 00046 #ifndef __SHARED__TYPES 00047 #define __SHARED__TYPES 00048 00049 typedef union 00050 { 00051 i16_t i16bit[3]; 00052 u8_t u8bit[6]; 00053 } Type3Axis16bit_U; 00054 00055 typedef union 00056 { 00057 i16_t i16bit; 00058 u8_t u8bit[2]; 00059 } Type1Axis16bit_U; 00060 00061 typedef union 00062 { 00063 i32_t i32bit; 00064 u8_t u8bit[4]; 00065 } Type1Axis32bit_U; 00066 00067 typedef enum 00068 { 00069 MEMS_SUCCESS = 0x01, 00070 MEMS_ERROR = 0x00 00071 } status_t; 00072 00073 #endif /*__SHARED__TYPES*/ 00074 00075 typedef u8_t LIS2DH12_IntPinConf_t; 00076 typedef u8_t LIS2DH12_AxesEnabled_t; 00077 typedef u8_t LIS2DH12_Int1Conf_t; 00078 typedef u8_t LIS2DH12_Int2Conf_t; 00079 00080 typedef enum 00081 { 00082 LIS2DH12_ENABLE = 0x01, 00083 LIS2DH12_DISABLE = 0x00 00084 } LIS2DH12_ACC_State_t; 00085 00086 typedef enum 00087 { 00088 LIS2DH12_ODR_POWER_DOWN = 0x00, 00089 LIS2DH12_ODR_1Hz = 0x01, 00090 LIS2DH12_ODR_10Hz = 0x02, 00091 LIS2DH12_ODR_25Hz = 0x03, 00092 LIS2DH12_ODR_50Hz = 0x04, 00093 LIS2DH12_ODR_100Hz = 0x05, 00094 LIS2DH12_ODR_200Hz = 0x06, 00095 LIS2DH12_ODR_400Hz = 0x07, 00096 LIS2DH12_ODR_1620Hz_LP = 0x08, 00097 LIS2DH12_ODR_1344Hz_NP_5367HZ_LP = 0x09 00098 } LIS2DH12_ODR_t; 00099 00100 typedef enum 00101 { 00102 LIS2DH12_HIGH_RES = 0x00, 00103 LIS2DH12_LOW_POWER = 0x01, 00104 LIS2DH12_NORMAL = 0x02 00105 } LIS2DH12_Mode_t; 00106 00107 typedef enum 00108 { 00109 LIS2DH12_HPM_NORMAL_MODE_RES = 0x00, 00110 LIS2DH12_HPM_REF_SIGNAL = 0x01, 00111 LIS2DH12_HPM_NORMAL_MODE = 0x02, 00112 LIS2DH12_HPM_AUTORESET_INT = 0x03 00113 } LIS2DH12_HPFMode_t; 00114 00115 typedef enum 00116 { 00117 LIS2DH12_HPFCF_0 = 0x00, 00118 LIS2DH12_HPFCF_1 = 0x01, 00119 LIS2DH12_HPFCF_2 = 0x02, 00120 LIS2DH12_HPFCF_3 = 0x03 00121 } LIS2DH12_HPFCutOffFreq_t; 00122 00123 typedef enum 00124 { 00125 LIS2DH12_FULLSCALE_2 = 0x00, 00126 LIS2DH12_FULLSCALE_4 = 0x01, 00127 LIS2DH12_FULLSCALE_8 = 0x02, 00128 LIS2DH12_FULLSCALE_16 = 0x03 00129 } LIS2DH12_Fullscale_t; 00130 00131 typedef enum 00132 { 00133 LIS2DH12_BLE_LSB = 0x00, 00134 LIS2DH12_BLE_MSB = 0x01 00135 } LIS2DH12_Endianess_t; 00136 00137 typedef enum 00138 { 00139 LIS2DH12_TEMP_ENABLE = 0x00, 00140 LIS2DH12_TEMP_DISABLE = 0x03 00141 } LIS2DH12_TempMode_t; 00142 00143 typedef enum 00144 { 00145 LIS2DH12_SELF_TEST_DISABLE = 0x00, 00146 LIS2DH12_SELF_TEST_0 = 0x01, 00147 LIS2DH12_SELF_TEST_1 = 0x02 00148 } LIS2DH12_SelfTest_t; 00149 00150 typedef enum 00151 { 00152 LIS2DH12_FIFO_BYPASS_MODE = 0x00, 00153 LIS2DH12_FIFO_MODE = 0x01, 00154 LIS2DH12_FIFO_STREAM_MODE = 0x02, 00155 LIS2DH12_FIFO_TRIGGER_MODE = 0x03, 00156 LIS2DH12_FIFO_DISABLE = 0x04 00157 } LIS2DH12_FifoMode_t; 00158 00159 typedef enum 00160 { 00161 LIS2DH12_TRIG_INT1 = 0x00, 00162 LIS2DH12_TRIG_INT2 = 0x01 00163 } LIS2DH12_TrigInt_t; 00164 00165 typedef enum 00166 { 00167 LIS2DH12_SPI_4_WIRE = 0x00, 00168 LIS2DH12_SPI_3_WIRE = 0x01 00169 } LIS2DH12_SPIMode_t; 00170 00171 typedef enum 00172 { 00173 LIS2DH12_X_ENABLE = 0x01, 00174 LIS2DH12_X_DISABLE = 0x00, 00175 LIS2DH12_Y_ENABLE = 0x02, 00176 LIS2DH12_Y_DISABLE = 0x00, 00177 LIS2DH12_Z_ENABLE = 0x04, 00178 LIS2DH12_Z_DISABLE = 0x00 00179 } LIS2DH12_AXISenable_t; 00180 00181 typedef enum 00182 { 00183 LIS2DH12_INT1_6D_4D_DISABLE = 0x00, 00184 LIS2DH12_INT1_6D_ENABLE = 0x01, 00185 LIS2DH12_INT1_4D_ENABLE = 0x02 00186 } LIS2DH12_INT1_6D_4D_t; 00187 00188 typedef enum 00189 { 00190 LIS2DH12_INT2_6D_4D_DISABLE = 0x00, 00191 LIS2DH12_INT2_6D_ENABLE = 0x01, 00192 LIS2DH12_INT2_4D_ENABLE = 0x02 00193 } LIS2DH12_INT2_6D_4D_t; 00194 00195 typedef enum 00196 { 00197 LIS2DH12_UP_SX = 0x44, 00198 LIS2DH12_UP_DX = 0x42, 00199 LIS2DH12_DW_SX = 0x41, 00200 LIS2DH12_DW_DX = 0x48, 00201 LIS2DH12_TOP = 0x60, 00202 LIS2DH12_BOTTOM = 0x50 00203 } LIS2DH12_POSITION_6D_t; 00204 00205 typedef enum 00206 { 00207 LIS2DH12_INT_MODE_OR = 0x00, 00208 LIS2DH12_INT_MODE_6D_MOVEMENT = 0x01, 00209 LIS2DH12_INT_MODE_AND = 0x02, 00210 LIS2DH12_INT_MODE_6D_POSITION = 0x03 00211 } LIS2DH12_IntMode_t; 00212 00213 //interrupt click response 00214 // b7 = don't care b6 = IA b5 = DClick b4 = Sclick b3 = Sign 00215 // b2 = z b1 = y b0 = x 00216 typedef enum 00217 { 00218 LIS2DH12_DCLICK_Z_P = 0x24, 00219 LIS2DH12_DCLICK_Z_N = 0x2C, 00220 LIS2DH12_SCLICK_Z_P = 0x14, 00221 LIS2DH12_SCLICK_Z_N = 0x1C, 00222 LIS2DH12_DCLICK_Y_P = 0x22, 00223 LIS2DH12_DCLICK_Y_N = 0x2A, 00224 LIS2DH12_SCLICK_Y_P = 0x12, 00225 LIS2DH12_SCLICK_Y_N = 0x1A, 00226 LIS2DH12_DCLICK_X_P = 0x21, 00227 LIS2DH12_DCLICK_X_N = 0x29, 00228 LIS2DH12_SCLICK_X_P = 0x11, 00229 LIS2DH12_SCLICK_X_N = 0x19, 00230 LIS2DH12_NO_CLICK = 0x00 00231 } LIS2DH12_Click_Response; 00232 00233 00234 /* Exported constants --------------------------------------------------------*/ 00235 00236 #ifndef __SHARED__CONSTANTS 00237 #define __SHARED__CONSTANTS 00238 00239 #define MEMS_SET 0x01 00240 #define MEMS_RESET 0x00 00241 00242 #endif /*__SHARED__CONSTANTS*/ 00243 00244 // WHO_AM_I value 00245 #define LIS2DH12_WHO_AM_I 0x33 00246 00247 //Register Definition 00248 // WHO_AM_I register 00249 #define LIS2DH12_WHO_AM_I_REG 0x0F // device identification register 00250 00251 // CONTROL REGISTER 1 00252 #define LIS2DH12_CTRL_REG1 0x20 00253 #define LIS2DH12_ODR_BIT BIT(4) 00254 #define LIS2DH12_LPEN BIT(3) 00255 #define LIS2DH12_ZEN BIT(2) 00256 #define LIS2DH12_YEN BIT(1) 00257 #define LIS2DH12_XEN BIT(0) 00258 00259 //CONTROL REGISTER 2 00260 #define LIS2DH12_CTRL_REG2 0x21 00261 #define LIS2DH12_HPM BIT(6) 00262 #define LIS2DH12_HPCF BIT(4) 00263 #define LIS2DH12_FDS BIT(3) 00264 #define LIS2DH12_HPCLICK BIT(2) 00265 #define LIS2DH12_HPIS2 BIT(1) 00266 #define LIS2DH12_HPIS1 BIT(0) 00267 00268 //CONTROL REGISTER 3 00269 #define LIS2DH12_CTRL_REG3 0x22 00270 #define LIS2DH12_I1_CLICK BIT(7) 00271 #define LIS2DH12_I1_AOI1 BIT(6) 00272 #define LIS2DH12_I1_AOI2 BIT(5) 00273 #define LIS2DH12_I1_DRDY1 BIT(4) 00274 #define LIS2DH12_I1_DRDY2 BIT(3) 00275 #define LIS2DH12_I1_WTM BIT(2) 00276 #define LIS2DH12_I1_ORUN BIT(1) 00277 00278 //CONTROL REGISTER 6 00279 #define LIS2DH12_CTRL_REG6 0x25 00280 #define LIS2DH12_I2_CLICK BIT(7) 00281 #define LIS2DH12_I2_INT1 BIT(6) 00282 #define LIS2DH12_I2_INT2 BIT(5) 00283 #define LIS2DH12_I2_BOOT BIT(4) 00284 #define LIS2DH12_P2_ACT BIT(3) 00285 #define LIS2DH12_H_LACTIVE BIT(1) 00286 00287 //TEMPERATURE CONFIG REGISTER 00288 #define LIS2DH12_TEMP_CFG_REG 0x1F 00289 #define LIS2DH12_TEMP_EN BIT(6) 00290 00291 //CONTROL REGISTER 4 00292 #define LIS2DH12_CTRL_REG4 0x23 00293 #define LIS2DH12_BDU BIT(7) 00294 #define LIS2DH12_BLE BIT(6) 00295 #define LIS2DH12_FS BIT(4) 00296 #define LIS2DH12_HR BIT(3) 00297 #define LIS2DH12_ST BIT(1) 00298 #define LIS2DH12_SIM BIT(0) 00299 00300 //CONTROL REGISTER 5 00301 #define LIS2DH12_CTRL_REG5 0x24 00302 #define LIS2DH12_BOOT BIT(7) 00303 #define LIS2DH12_FIFO_EN BIT(6) 00304 #define LIS2DH12_LIR_INT1 BIT(3) 00305 #define LIS2DH12_D4D_INT1 BIT(2) 00306 #define LIS2DH12_LIR_INT2 BIT(1) 00307 #define LIS2DH12_D4D_INT2 BIT(0) 00308 00309 //REFERENCE/DATA_CAPTURE 00310 #define LIS2DH12_REFERENCE_REG 0x26 00311 #define LIS2DH12_REF BIT(0) 00312 00313 //STATUS_REG_AXIES 00314 #define LIS2DH12_STATUS_REG 0x27 00315 #define LIS2DH12_ZYXOR BIT(7) 00316 #define LIS2DH12_ZOR BIT(6) 00317 #define LIS2DH12_YOR BIT(5) 00318 #define LIS2DH12_XOR BIT(4) 00319 #define LIS2DH12_ZYXDA BIT(3) 00320 #define LIS2DH12_ZDA BIT(2) 00321 #define LIS2DH12_YDA BIT(1) 00322 #define LIS2DH12_XDA BIT(0) 00323 00324 //STATUS_REG_AUX 00325 #define LIS2DH12_STATUS_AUX 0x07 00326 00327 //INTERRUPT 1 CONFIGURATION 00328 #define LIS2DH12_INT1_CFG 0x30 00329 #define LIS2DH12_ANDOR BIT(7) 00330 #define LIS2DH12_INT_6D BIT(6) 00331 #define LIS2DH12_ZHIE BIT(5) 00332 #define LIS2DH12_ZLIE BIT(4) 00333 #define LIS2DH12_YHIE BIT(3) 00334 #define LIS2DH12_YLIE BIT(2) 00335 #define LIS2DH12_XHIE BIT(1) 00336 #define LIS2DH12_XLIE BIT(0) 00337 00338 //INTERRUPT 2 CONFIGURATION 00339 #define LIS2DH12_INT2_CFG 0x34 00340 00341 //FIFO CONTROL REGISTER 00342 #define LIS2DH12_FIFO_CTRL_REG 0x2E 00343 #define LIS2DH12_FM BIT(6) 00344 #define LIS2DH12_TR BIT(5) 00345 #define LIS2DH12_FTH BIT(0) 00346 00347 //CONTROL REG3 bit mask 00348 #define LIS2DH12_CLICK_ON_PIN_INT1_ENABLE 0x80 00349 #define LIS2DH12_CLICK_ON_PIN_INT1_DISABLE 0x00 00350 #define LIS2DH12_I1_INT1_ON_PIN_INT1_ENABLE 0x40 00351 #define LIS2DH12_I1_INT1_ON_PIN_INT1_DISABLE 0x00 00352 #define LIS2DH12_I1_INT2_ON_PIN_INT1_ENABLE 0x20 00353 #define LIS2DH12_I1_INT2_ON_PIN_INT1_DISABLE 0x00 00354 #define LIS2DH12_I1_DRDY1_ON_INT1_ENABLE 0x10 00355 #define LIS2DH12_I1_DRDY1_ON_INT1_DISABLE 0x00 00356 #define LIS2DH12_I1_DRDY2_ON_INT1_ENABLE 0x08 00357 #define LIS2DH12_I1_DRDY2_ON_INT1_DISABLE 0x00 00358 #define LIS2DH12_WTM_ON_INT1_ENABLE 0x04 00359 #define LIS2DH12_WTM_ON_INT1_DISABLE 0x00 00360 #define LIS2DH12_INT1_OVERRUN_ENABLE 0x02 00361 #define LIS2DH12_INT1_OVERRUN_DISABLE 0x00 00362 00363 //CONTROL REG6 bit mask 00364 #define LIS2DH12_CLICK_ON_PIN_INT2_ENABLE 0x80 00365 #define LIS2DH12_CLICK_ON_PIN_INT2_DISABLE 0x00 00366 #define LIS2DH12_I2_INT1_ON_PIN_INT2_ENABLE 0x40 00367 #define LIS2DH12_I2_INT1_ON_PIN_INT2_DISABLE 0x00 00368 #define LIS2DH12_I2_INT2_ON_PIN_INT2_ENABLE 0x20 00369 #define LIS2DH12_I2_INT2_ON_PIN_INT2_DISABLE 0x00 00370 #define LIS2DH12_I2_BOOT_ON_INT2_ENABLE 0x10 00371 #define LIS2DH12_I2_BOOT_ON_INT2_DISABLE 0x00 00372 #define LIS2DH12_I2_ACTIVITY_ON_INT2_ENABLE 0x08 00373 #define LIS2DH12_I2_ACTIVITY_ON_INT2_DISABLE 0x00 00374 #define LIS2DH12_INT_ACTIVE_HIGH 0x00 00375 #define LIS2DH12_INT_ACTIVE_LOW 0x02 00376 00377 //INT1_CFG bit mask 00378 #define LIS2DH12_INT1_AND 0x80 00379 #define LIS2DH12_INT1_OR 0x00 00380 #define LIS2DH12_INT1_ZHIE_ENABLE 0x20 00381 #define LIS2DH12_INT1_ZHIE_DISABLE 0x00 00382 #define LIS2DH12_INT1_ZLIE_ENABLE 0x10 00383 #define LIS2DH12_INT1_ZLIE_DISABLE 0x00 00384 #define LIS2DH12_INT1_YHIE_ENABLE 0x08 00385 #define LIS2DH12_INT1_YHIE_DISABLE 0x00 00386 #define LIS2DH12_INT1_YLIE_ENABLE 0x04 00387 #define LIS2DH12_INT1_YLIE_DISABLE 0x00 00388 #define LIS2DH12_INT1_XHIE_ENABLE 0x02 00389 #define LIS2DH12_INT1_XHIE_DISABLE 0x00 00390 #define LIS2DH12_INT1_XLIE_ENABLE 0x01 00391 #define LIS2DH12_INT1_XLIE_DISABLE 0x00 00392 00393 //INT2_CFG bit mask 00394 #define LIS2DH12_INT2_AND 0x80 00395 #define LIS2DH12_INT2_OR 0x00 00396 #define LIS2DH12_INT2_ZHIE_ENABLE 0x20 00397 #define LIS2DH12_INT2_ZHIE_DISABLE 0x00 00398 #define LIS2DH12_INT2_ZLIE_ENABLE 0x10 00399 #define LIS2DH12_INT2_ZLIE_DISABLE 0x00 00400 #define LIS2DH12_INT2_YHIE_ENABLE 0x08 00401 #define LIS2DH12_INT2_YHIE_DISABLE 0x00 00402 #define LIS2DH12_INT2_YLIE_ENABLE 0x04 00403 #define LIS2DH12_INT2_YLIE_DISABLE 0x00 00404 #define LIS2DH12_INT2_XHIE_ENABLE 0x02 00405 #define LIS2DH12_INT2_XHIE_DISABLE 0x00 00406 #define LIS2DH12_INT2_XLIE_ENABLE 0x01 00407 #define LIS2DH12_INT2_XLIE_DISABLE 0x00 00408 00409 //INT1_SRC bit mask 00410 #define LIS2DH12_INT1_SRC_IA 0x40 00411 #define LIS2DH12_INT1_SRC_ZH 0x20 00412 #define LIS2DH12_INT1_SRC_ZL 0x10 00413 #define LIS2DH12_INT1_SRC_YH 0x08 00414 #define LIS2DH12_INT1_SRC_YL 0x04 00415 #define LIS2DH12_INT1_SRC_XH 0x02 00416 #define LIS2DH12_INT1_SRC_XL 0x01 00417 00418 //INT2_SRC bit mask 00419 #define LIS2DH12_INT2_SRC_IA 0x40 00420 #define LIS2DH12_INT2_SRC_ZH 0x20 00421 #define LIS2DH12_INT2_SRC_ZL 0x10 00422 #define LIS2DH12_INT2_SRC_YH 0x08 00423 #define LIS2DH12_INT2_SRC_YL 0x04 00424 #define LIS2DH12_INT2_SRC_XH 0x02 00425 #define LIS2DH12_INT2_SRC_XL 0x01 00426 00427 //INT1 REGISTERS 00428 #define LIS2DH12_INT1_THS 0x32 00429 #define LIS2DH12_INT1_DURATION 0x33 00430 00431 //INT2 REGISTERS 00432 #define LIS2DH12_INT2_THS 0x36 00433 #define LIS2DH12_INT2_DURATION 0x37 00434 00435 //INTERRUPT 1 SOURCE REGISTER 00436 #define LIS2DH12_INT1_SRC 0x31 00437 #define INT1 0x31 00438 00439 //INTERRUPT 2 SOURCE REGISTER 00440 #define LIS2DH12_INT2_SRC 0x35 00441 #define INT2 0x35 00442 00443 //FIFO Source Register bit Mask 00444 #define LIS2DH12_FIFO_SRC_WTM 0x80 00445 #define LIS2DH12_FIFO_SRC_OVRUN 0x40 00446 #define LIS2DH12_FIFO_SRC_EMPTY 0x20 00447 00448 //INTERRUPT CLICK REGISTER 00449 #define LIS2DH12_CLICK_CFG 0x38 00450 //INTERRUPT CLICK CONFIGURATION bit mask 00451 #define LIS2DH12_ZD_ENABLE 0x20 00452 #define LIS2DH12_ZD_DISABLE 0x00 00453 #define LIS2DH12_ZS_ENABLE 0x10 00454 #define LIS2DH12_ZS_DISABLE 0x00 00455 #define LIS2DH12_YD_ENABLE 0x08 00456 #define LIS2DH12_YD_DISABLE 0x00 00457 #define LIS2DH12_YS_ENABLE 0x04 00458 #define LIS2DH12_YS_DISABLE 0x00 00459 #define LIS2DH12_XD_ENABLE 0x02 00460 #define LIS2DH12_XD_DISABLE 0x00 00461 #define LIS2DH12_XS_ENABLE 0x01 00462 #define LIS2DH12_XS_DISABLE 0x00 00463 00464 //INTERRUPT CLICK SOURCE REGISTER 00465 #define LIS2DH12_CLICK_SRC 0x39 00466 //INTERRUPT CLICK SOURCE REGISTER bit mask 00467 #define LIS2DH12_IA 0x40 00468 #define LIS2DH12_DCLICK 0x20 00469 #define LIS2DH12_SCLICK 0x10 00470 #define LIS2DH12_CLICK_SIGN 0x08 00471 #define LIS2DH12_CLICK_Z 0x04 00472 #define LIS2DH12_CLICK_Y 0x02 00473 #define LIS2DH12_CLICK_X 0x01 00474 00475 //Click-click Register 00476 #define LIS2DH12_CLICK_THS 0x3A 00477 #define LIS2DH12_TIME_LIMIT 0x3B 00478 #define LIS2DH12_TIME_LATENCY 0x3C 00479 #define LIS2DH12_TIME_WINDOW 0x3D 00480 00481 //OUTPUT REGISTER 00482 #define LIS2DH12_OUT_X_L 0x28 00483 #define LIS2DH12_OUT_X_H 0x29 00484 #define LIS2DH12_OUT_Y_L 0x2A 00485 #define LIS2DH12_OUT_Y_H 0x2B 00486 #define LIS2DH12_OUT_Z_L 0x2C 00487 #define LIS2DH12_OUT_Z_H 0x2D 00488 00489 //TEMP REGISTERS 00490 #define LIS2DH12_OUT_TEMP_L 0x0C 00491 #define LIS2DH12_OUT_TEMP_H 0x0D 00492 00493 //STATUS REGISTER bit mask 00494 #define LIS2DH12_STATUS_REG_ZYXOR 0x80 // 1 : new data set has over written the previous one 00495 // 0 : no overrun has occurred (default) 00496 #define LIS2DH12_STATUS_REG_ZOR 0x40 // 0 : no overrun has occurred (default) 00497 // 1 : new Z-axis data has over written the previous one 00498 #define LIS2DH12_STATUS_REG_YOR 0x20 // 0 : no overrun has occurred (default) 00499 // 1 : new Y-axis data has over written the previous one 00500 #define LIS2DH12_STATUS_REG_XOR 0x10 // 0 : no overrun has occurred (default) 00501 // 1 : new X-axis data has over written the previous one 00502 #define LIS2DH12_STATUS_REG_ZYXDA 0x08 // 0 : a new set of data is not yet avvious one 00503 // 1 : a new set of data is available 00504 #define LIS2DH12_STATUS_REG_ZDA 0x04 // 0 : a new data for the Z-Axis is not availvious one 00505 // 1 : a new data for the Z-Axis is available 00506 #define LIS2DH12_STATUS_REG_YDA 0x02 // 0 : a new data for the Y-Axis is not available 00507 // 1 : a new data for the Y-Axis is available 00508 #define LIS2DH12_STATUS_REG_XDA 0x01 // 0 : a new data for the X-Axis is not available 00509 00510 #define LIS2DH12_DATAREADY_BIT LIS2DH12_STATUS_REG_ZYXDA 00511 00512 00513 //STATUS AUX REGISTER bit mask 00514 #define LIS2DH12_STATUS_AUX_TOR 0x40 00515 #define LIS2DH12_STATUS_AUX_TDA 0x04 00516 00517 // I2C Address 00518 #define LIS2DH12_I2C_ADDRESS_LOW 0x30 // SAD[0] = 0 00519 #define LIS2DH12_I2C_ADDRESS_HIGH 0x32 // SAD[0] = 1 00520 00521 //FIFO REGISTERS 00522 #define LIS2DH12_FIFO_CTRL_REG 0x2E 00523 #define LIS2DH12_FIFO_SRC_REG 0x2F 00524 00525 //Sleep to Wake Registers 00526 #define LIS2DH12_ACT_THS 0x3E 00527 #define LIS2DH12_ACT_DUR 0x3F 00528 00529 /* Exported macro ------------------------------------------------------------*/ 00530 00531 #ifndef __SHARED__MACROS 00532 00533 #define __SHARED__MACROS 00534 #define ValBit(VAR,Place) (VAR & (1<<Place)) 00535 #define BIT(x) ( (x) ) 00536 00537 #endif /*__SHARED__MACROS*/ 00538 00539 /* Exported functions --------------------------------------------------------*/ 00540 //Generic 00541 status_t LIS2DH12_ACC_WriteReg(void *handle, u8_t Reg, u8_t *Bufp, u16_t len); 00542 status_t LIS2DH12_ACC_ReadReg(void *handle, u8_t Reg, u8_t *Bufp, u16_t len); 00543 00544 //Sensor Configuration Functions 00545 status_t LIS2DH12_GetODR(void *handle, LIS2DH12_ODR_t *ov); 00546 status_t LIS2DH12_SetODR(void *handle, LIS2DH12_ODR_t ov); 00547 status_t LIS2DH12_GetMode(void *handle, LIS2DH12_Mode_t *md); 00548 status_t LIS2DH12_SetMode(void *handle, LIS2DH12_Mode_t md); 00549 status_t LIS2DH12_GetAxesEnabled(void *handle, LIS2DH12_AxesEnabled_t *axes); 00550 status_t LIS2DH12_SetAxesEnabled(void *handle, LIS2DH12_AxesEnabled_t axes); 00551 status_t LIS2DH12_GetFullScale(void *handle, LIS2DH12_Fullscale_t *fs); 00552 status_t LIS2DH12_SetFullScale(void *handle, LIS2DH12_Fullscale_t fs); 00553 status_t LIS2DH12_SetBDU(void *handle, LIS2DH12_ACC_State_t bdu); 00554 status_t LIS2DH12_SetBLE(void *handle, LIS2DH12_Endianess_t ble); 00555 status_t LIS2DH12_SetSelfTest(void *handle, LIS2DH12_SelfTest_t st); 00556 status_t LIS2DH12_SetTemperature(void *handle, LIS2DH12_TempMode_t tempmode); 00557 00558 //Filtering Functions 00559 status_t LIS2DH12_HPFClickEnable(void *handle, LIS2DH12_ACC_State_t hpfe); 00560 status_t LIS2DH12_HPFAOI1Enable(void *handle, LIS2DH12_ACC_State_t hpfe); 00561 status_t LIS2DH12_HPFAOI2Enable(void *handle, LIS2DH12_ACC_State_t hpfe); 00562 status_t LIS2DH12_SetHPFMode(void *handle, LIS2DH12_HPFMode_t hpf); 00563 status_t LIS2DH12_SetHPFCutOFF(void *handle, LIS2DH12_HPFCutOffFreq_t hpf); 00564 status_t LIS2DH12_SetFilterDataSel(void *handle, LIS2DH12_ACC_State_t state); 00565 00566 //Interrupt Functions 00567 status_t LIS2DH12_SetInt1Pin(void *handle, LIS2DH12_IntPinConf_t pinConf); 00568 status_t LIS2DH12_SetInt2Pin(void *handle, LIS2DH12_IntPinConf_t pinConf); 00569 status_t LIS2DH12_Int1LatchEnable(void *handle, LIS2DH12_ACC_State_t latch); 00570 status_t LIS2DH12_ResetInt1Latch(void *handle); 00571 status_t LIS2DH12_Int2LatchEnable(void *handle, LIS2DH12_ACC_State_t latch); 00572 status_t LIS2DH12_ResetInt2Latch(void *handle); 00573 status_t LIS2DH12_SetInt1Configuration(void *handle, LIS2DH12_Int1Conf_t ic); 00574 status_t LIS2DH12_SetInt2Configuration(void *handle, LIS2DH12_Int2Conf_t ic); 00575 status_t LIS2DH12_SetInt1Threshold(void *handle, u8_t ths); 00576 status_t LIS2DH12_SetInt1Duration(void *handle, LIS2DH12_Int1Conf_t id); 00577 status_t LIS2DH12_SetInt2Threshold(void *handle, u8_t ths); 00578 status_t LIS2DH12_SetInt2Duration(void *handle, LIS2DH12_Int2Conf_t id); 00579 status_t LIS2DH12_SetInt1Mode(void *handle, LIS2DH12_IntMode_t ic); 00580 status_t LIS2DH12_SetInt2Mode(void *handle, LIS2DH12_IntMode_t ic); 00581 status_t LIS2DH12_SetClickCFG(void *handle, u8_t status); 00582 status_t LIS2DH12_SetClickTHS(void *handle, u8_t ths); 00583 status_t LIS2DH12_SetClickLIMIT(void *handle, u8_t t_limit); 00584 status_t LIS2DH12_SetClickLATENCY(void *handle, u8_t t_latency); 00585 status_t LIS2DH12_SetClickWINDOW(void *handle, u8_t t_window); 00586 status_t LIS2DH12_SetInt16D4DConfiguration(void *handle, LIS2DH12_INT1_6D_4D_t ic); 00587 status_t LIS2DH12_SetInt26D4DConfiguration(void *handle, LIS2DH12_INT2_6D_4D_t ic); 00588 status_t LIS2DH12_GetInt1Src(void *handle, u8_t *val); 00589 status_t LIS2DH12_GetInt1SrcBit(void *handle, u8_t statusBIT, u8_t *val); 00590 status_t LIS2DH12_GetInt2Src(void *handle, u8_t *val); 00591 status_t LIS2DH12_GetInt2SrcBit(void *handle, u8_t statusBIT, u8_t *val); 00592 00593 //FIFO Functions 00594 status_t LIS2DH12_FIFOModeEnable(void *handle, LIS2DH12_FifoMode_t fm); 00595 status_t LIS2DH12_SetWaterMark(void *handle, u8_t wtm); 00596 status_t LIS2DH12_SetTriggerInt(void *handle, LIS2DH12_TrigInt_t tr); 00597 status_t LIS2DH12_GetFifoSourceReg(void *handle, u8_t *val); 00598 status_t LIS2DH12_GetFifoSourceBit(void *handle, u8_t statusBIT, u8_t *val); 00599 status_t LIS2DH12_GetFifoSourceFSS(void *handle, u8_t *val); 00600 status_t LIS2DH12_SetSPIInterface(void *handle, LIS2DH12_SPIMode_t spi); 00601 00602 //Sleep to Wake Functions 00603 status_t LIS2DH12_SetActTHS(void *handle, u8_t val); 00604 status_t LIS2DH12_SetActDUR(void *handle, u8_t val); 00605 00606 //Other Reading Functions 00607 status_t LIS2DH12_GetStatusReg(void *handle, u8_t *val); 00608 status_t LIS2DH12_GetStatusBit(void *handle, u8_t statusBIT, u8_t *val); 00609 status_t LIS2DH12_GetStatusAUXBit(void *handle, u8_t statusBIT, u8_t *val); 00610 status_t LIS2DH12_GetStatusAUX(void *handle, u8_t *val); 00611 status_t LIS2DH12_GetAccAxesRaw(void *handle, u8_t *buff); 00612 status_t LIS2DH12_GetClickResponse(void *handle, u8_t *val); 00613 status_t LIS2DH12_GetTempRaw(void *handle, i8_t *val); 00614 status_t LIS2DH12_GetWHO_AM_I(void *handle, u8_t *val); 00615 status_t LIS2DH12_Get6DPosition(void *handle, u8_t *val, u8_t INT); 00616 00617 #ifdef __cplusplus 00618 } 00619 #endif 00620 00621 #endif /* __LIS2DH12_H */ 00622 00623 /******************* (C) COPYRIGHT 2018 STMicroelectronics *****END OF FILE****/ 00624 00625 00626
Generated on Tue Jul 12 2022 16:29:49 by
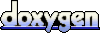