
Mbed Clock application using an NTP connection to get internet time and a terminal interface to send commands
Dependencies: 4DGL-uLCD-SE EthernetInterface NTPClient mbed-rtos mbed SDFileSystem wavfile
main.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "EthernetInterface.h" 00004 #include "uLCD_4DGL.h" 00005 #include "SDFileSystem.h" 00006 #include "Clock.h" 00007 #include "Recorder.h" 00008 #include "Speaker.h" 00009 #include <string> 00010 00011 //#define SERVER_IP "143.215.120.157" 00012 #define SERVER_IP "143.215.119.135" 00013 #define SERVER_PORT 13000 00014 #define REC_NAME "/sd/rec.wav" 00015 #define PLAY_NAME "/sd/play.wav" 00016 00017 #define BUF_SIZE 8192 00018 00019 // Big Components 00020 uLCD_4DGL uLCD(p28,p27,p30); 00021 Serial pc(USBTX, USBRX); 00022 Serial easyVR(p13, p14); 00023 SDFileSystem sdc(p5, p6, p7, p8, "sd"); 00024 EthernetInterface eth; 00025 TCPSocketConnection server; 00026 Clock clk; 00027 00028 // Small Components 00029 Speaker speaker(p21); 00030 DigitalOut sleepLED(LED4); 00031 Mutex cMutex; 00032 Mutex sMutex; 00033 char buf[BUF_SIZE]; 00034 // float array for recorder (they share the buffer) 00035 float *buffer = (float*)buf; 00036 00037 // Help with noise 00038 AnalogIn pin15(p15); 00039 AnalogIn pin16(p16); 00040 AnalogIn pin17(p17); 00041 AnalogIn pin19(p19); 00042 00043 void aThread(void const *args) { 00044 while (1) { 00045 Thread::signal_wait(0x1, osWaitForever); 00046 for (int i = 0; i < 5; i++) { 00047 speaker.playNote(969.0, 0.5, 0.1); 00048 Thread::wait(1000); 00049 } 00050 } 00051 } 00052 00053 void execute(char *command) { 00054 char buffer[12]; 00055 int hour, minute, period, zone; 00056 sscanf(command, "%s %d %d %d %d", buffer, &hour, &minute, &period, &zone); 00057 string operation(buffer); 00058 00059 if (operation == "setTime") { 00060 cMutex.lock(); 00061 clk.setTime(hour, minute, period); 00062 cMutex.unlock(); 00063 } else if (operation == "setTimezone") { 00064 cMutex.lock(); 00065 clk.setTimezone(zone); 00066 cMutex.unlock(); 00067 } else if (operation == "setAlarm") { 00068 cMutex.lock(); 00069 clk.setAlarm(hour, minute, period); 00070 cMutex.unlock(); 00071 } else if (operation == "setTimer") { 00072 cMutex.lock(); 00073 clk.setTimer(hour, minute); 00074 cMutex.unlock(); 00075 } else if (operation == "deleteAlarm") { 00076 cMutex.lock(); 00077 clk.deleteAlarm(); 00078 cMutex.unlock(); 00079 } else if (operation == "syncNow") { 00080 cMutex.lock(); 00081 if (clk.syncTime() != 0) { 00082 printf(" ERROR: failed to sync time\n\n\r"); 00083 } 00084 cMutex.unlock(); 00085 } else if (operation == "noCommand") { 00086 printf(" ERROR: speech not recognized\n\n\r"); 00087 } else { 00088 printf(" ERROR: not a valid command\n\n\r"); 00089 } 00090 } 00091 00092 00093 /** 00094 * Thread which updates the clock on the lcd while the main thread is waiting for 00095 * or executing a command. 00096 */ 00097 void lcdUpdateThread(void const *args) { 00098 time_t time; 00099 struct tm *timeinfo; 00100 char buffer[20]; 00101 00102 // set initial time 00103 cMutex.lock(); 00104 clk.syncTime(); 00105 clk.setTimezone(-5); 00106 cMutex.unlock(); 00107 00108 // set lcd format 00109 sMutex.lock(); 00110 uLCD.text_width(2); 00111 uLCD.text_height(2); 00112 uLCD.color(BLUE); 00113 sMutex.unlock(); 00114 00115 while (1) { 00116 cMutex.lock(); 00117 time = clk.getTime(); 00118 bool alarmSet = clk.alarmSet(); 00119 cMutex.unlock(); 00120 00121 timeinfo = localtime(&time); 00122 strftime(buffer, 20, "%I:%M:%S %p", timeinfo); 00123 00124 sMutex.lock(); 00125 uLCD.locate(0,3); 00126 uLCD.printf("%s", buffer); 00127 if (alarmSet) 00128 uLCD.printf("ALARM SET"); 00129 else 00130 uLCD.printf(" "); 00131 sMutex.unlock(); 00132 Thread::wait(200); 00133 } 00134 } 00135 00136 void init() { 00137 printf("\r\n\n--Starting MbedClock--\n\r"); 00138 00139 eth.init(); 00140 printf(" * Initialized Ethernet\n\r"); 00141 00142 eth.connect(); 00143 printf(" * Connected using DHCP\n\r"); 00144 wait(5); 00145 printf(" * Using IP: %s\n\r", eth.getIPAddress()); 00146 00147 easyVR.putc('b'); 00148 easyVR.getc(); 00149 printf(" * Initialized EasyVR\n\r"); 00150 00151 00152 } 00153 00154 void waitForTrigger() { 00155 // set EasyVR to 2 claps 00156 sMutex.lock(); 00157 easyVR.putc('s'); 00158 easyVR.putc('A' + 1); 00159 sMutex.unlock(); 00160 wait(0.2); 00161 00162 // Clear buffer and wait for awake 00163 printf("Waiting for trigger..."); 00164 sMutex.lock(); 00165 while (easyVR.readable()) 00166 easyVR.getc(); 00167 sMutex.unlock(); 00168 sleepLED = 1; 00169 while(!easyVR.readable()) { 00170 wait(0.2); 00171 } 00172 sleepLED = 0; 00173 printf("trigger received!\n\r"); 00174 } 00175 00176 void waitForTrigger2() { 00177 char rchar = 0; 00178 sleepLED = 1; 00179 printf("Waiting for trigger..."); 00180 while (rchar!='A') { 00181 wait(.001); 00182 sMutex.lock(); 00183 easyVR.putc('d'); 00184 // a small delay is needed when sending EasyVR several characters 00185 wait(.001); 00186 easyVR.putc('A'); 00187 sMutex.unlock(); 00188 while (!easyVR.readable()) { 00189 wait(0.2); 00190 } 00191 sMutex.lock(); 00192 rchar=easyVR.getc(); 00193 sMutex.unlock(); 00194 // word recognized 00195 if (rchar=='r') { 00196 wait(.001); 00197 sMutex.lock(); 00198 easyVR.putc(' '); 00199 rchar=easyVR.getc(); 00200 sMutex.unlock(); 00201 // error 00202 } else if (rchar=='e') { 00203 wait(.001); 00204 sMutex.lock(); 00205 easyVR.putc(' '); 00206 rchar=easyVR.getc(); 00207 easyVR.putc(' '); 00208 rchar=easyVR.getc(); 00209 sMutex.unlock(); 00210 } 00211 } 00212 sleepLED = 0; 00213 printf("trigger received!\n\r"); 00214 } 00215 00216 void sendFile() { 00217 printf(" Sending \"%s\"...", REC_NAME); 00218 FILE *fp = fopen(REC_NAME, "rb"); 00219 00220 int sum = 0; 00221 while (sum < 110296) 00222 { 00223 int i = fread(buf, 1, BUF_SIZE, fp); 00224 server.send(buf, i); 00225 sum += i; 00226 } 00227 printf("sent\n\r"); 00228 fclose(fp); 00229 00230 int n = server.receive(buf, BUF_SIZE); 00231 buf[n] = '\0'; 00232 printf(" Received \"%s\"\n\n\r", buf); 00233 } 00234 00235 int main() { 00236 init(); 00237 //RtosTimer alarmTimer(aThread, osTimerOnce, NULL); 00238 00239 00240 Thread alarmThread(aThread); 00241 Thread updateThread(lcdUpdateThread); 00242 printf(" * Started LCD and Alarm Threads\n\n\r"); 00243 00244 cMutex.lock(); 00245 clk.setAlarmThread(&alarmThread); 00246 cMutex.unlock(); 00247 while(1) { 00248 waitForTrigger(); 00249 00250 printf(" Recording audio file..."); 00251 rec(REC_NAME, 5); 00252 printf("complete\n\r"); 00253 //wait(0.1); 00254 //play(FILE_NAME); 00255 00256 if (!server.connect(SERVER_IP, SERVER_PORT)) { 00257 printf(" Connected to %s\n\r", SERVER_IP); 00258 sendFile(); 00259 execute(buf); 00260 server.close(); 00261 } else { 00262 printf(" Unable to connect to %s\n\n\r", SERVER_IP); 00263 } 00264 } 00265 }
Generated on Sat Jul 16 2022 17:26:22 by
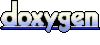