
Mbed Clock application using an NTP connection to get internet time and a terminal interface to send commands
Dependencies: 4DGL-uLCD-SE EthernetInterface NTPClient mbed-rtos mbed SDFileSystem wavfile
Clock.cpp
00001 #include "Clock.h" 00002 00003 00004 Clock::Clock() : timezone(UTC) { 00005 set_time(1388534400); 00006 } 00007 00008 void Clock::setAlarmThread(Thread *aThread) { 00009 alarmThread = aThread; 00010 } 00011 00012 /** 00013 * Sets the time and stores it in UTC time 00014 */ 00015 void Clock::setTime(int hour, int minute, int period) { 00016 time_t rawtime = time(NULL); 00017 struct tm *timeinfo = localtime(&rawtime); 00018 if (hour == 12) 00019 hour = 0; 00020 timeinfo->tm_hour = (((period == AM) ? hour : (hour + 12)) - timezone) % 24; 00021 timeinfo->tm_min = minute; 00022 timeinfo->tm_sec = 0; 00023 set_time(mktime(timeinfo)); 00024 } 00025 00026 /** 00027 * Sets the timezone. Since the time is stored in UTC, the system time 00028 * is not modified 00029 */ 00030 void Clock::setTimezone(int timezone) { 00031 00032 this->timezone = timezone; 00033 } 00034 00035 /** 00036 * Uses an NTP Client to set the time to UTC 00037 */ 00038 int Clock::syncTime() { 00039 NTPClient ntp; 00040 return ntp.setTime("0.pool.ntp.org"); 00041 } 00042 00043 void Clock::setAlarm(int hour, int minute, int period) { 00044 time_t currentTime = getTime(); 00045 struct tm *timeinfo = localtime(¤tTime); 00046 if (hour == 12) 00047 hour = 0; 00048 hour = ((period == AM) ? hour : (hour + 12)) % 24; 00049 if (hour <= timeinfo->tm_hour && minute <= timeinfo->tm_hour) { 00050 (timeinfo->tm_mday)++; 00051 } 00052 timeinfo->tm_hour = hour; 00053 timeinfo->tm_min = minute; 00054 timeinfo->tm_sec = 0; 00055 time_t alarmTime = mktime(timeinfo); 00056 alarmTicker.attach(this, &Clock::signalAlarm, difftime(alarmTime, currentTime)); 00057 aSet = true; 00058 } 00059 00060 bool Clock::alarmSet() { 00061 return aSet; 00062 } 00063 00064 void Clock::setTimer(int hours, int minutes) { 00065 alarmTicker.attach(this, &Clock::signalAlarm, hours * 3600 + minutes * 60); 00066 aSet = true; 00067 } 00068 00069 void Clock::deleteAlarm() { 00070 alarmTicker.detach(); 00071 aSet = false; 00072 } 00073 00074 /** 00075 * Gets the system time in UTC and converts it according to the given timezone 00076 */ 00077 time_t Clock::getTime() { 00078 time_t rawtime = time(NULL); 00079 struct tm *timeinfo = localtime(&rawtime); 00080 timeinfo->tm_hour = (timeinfo->tm_hour + timezone) % 24; 00081 return mktime(timeinfo); 00082 } 00083 00084 int Clock::getTimezone() { 00085 return timezone; 00086 } 00087 00088 void Clock::signalAlarm() { 00089 alarmThread->signal_set(0x1); 00090 alarmTicker.detach(); 00091 aSet = false; 00092 } 00093 00094
Generated on Sat Jul 16 2022 17:26:22 by
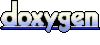