https://developer.mbed.org/users/ds4279/code/WiflySocket/
Embed:
(wiki syntax)
Show/hide line numbers
Wifly.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "mbed.h" 00020 00021 #include "headerStuff.h" 00022 #include "Wifly.h" 00023 #include <string> 00024 #include <algorithm> 00025 00026 //extern void sendToDisplay(int cmd,int canID,char *canMsg); 00027 extern Serial myDebug; 00028 00029 //Debug is disabled by default 00030 #if (0 && !defined(TARGET_LPC11U24)) 00031 #define DBG(x, ...) std::printf("[Wifly : DBG]"x"\r\n", ##__VA_ARGS__); 00032 #define WARN(x, ...) std::printf("[Wifly : WARN]"x"\r\n", ##__VA_ARGS__); 00033 #define ERR(x, ...) std::printf("[Wifly : ERR]"x"\r\n", ##__VA_ARGS__); 00034 #else 00035 #define DBG(x, ...) 00036 #define WARN(x, ...) 00037 #define ERR(x, ...) 00038 #endif 00039 00040 #if !defined(TARGET_LPC11U24) 00041 #define INFO(x, ...) printf("[Wifly : INFO]"x"\r\n", ##__VA_ARGS__); 00042 #else 00043 #define INFO(x, ...) 00044 #endif 00045 00046 #define MAX_TRY_JOIN 3 00047 00048 ///////////////////////////////////////// 00049 ///Make this object visible to the other 00050 ///classes. UDPSocket, etc... 00051 Wifly * Wifly::inst; 00052 00053 // PB_4/D5/GPIO6 short pad P6 on shield 00054 Wifly::Wifly( PinName tx, PinName rx, PinName _reset, PinName tcp_status, const char * ssid, const char * phrase, Security sec): 00055 wifiSer(tx, rx,512,2), reset_pin(_reset), tcp_status(tcp_status)//, buf_wifly(256) 00056 //PinName tx, PinName rx, uint32_t buf_size = 512, uint32_t tx_multiple = 4 00057 { 00058 memset(&state, 0, sizeof(state)); 00059 state.sec = sec; 00060 00061 /////////////////////////////////////////// 00062 ///The default baud rate 9600. Once change 00063 ///It will never have to be changed again. 00064 wifiSer.baud(19200); 00065 wifiSer.format(8,Serial::None,1); 00066 00067 // change all ' ' in '$' in the ssid and the passphrase 00068 strcpy(this->ssid, ssid); 00069 for (int i = 0; i < strlen(ssid); i++) 00070 { 00071 if (this->ssid[i] == ' ') 00072 this->ssid[i] = '$'; 00073 } 00074 00075 strcpy(this->phrase, phrase); 00076 for (int i = 0; i < strlen(phrase); i++) 00077 { 00078 if (this->phrase[i] == ' ') 00079 this->phrase[i] = '$'; 00080 } 00081 00082 strcpy(this->staticIP,"0.0.0.0"); 00083 inst = this; //make this class visible to other classes 00084 // attach_rx(false); 00085 state.cmd_mode = false; 00086 state.dhcp = true; //assume initial connection DHCP 00087 } 00088 00089 void Wifly::getDHCPhostInfo(char *SSID, char *passPhrase, int &auth) 00090 { 00091 auth = state.sec; 00092 strcpy(SSID,this->ssid); 00093 strcpy(passPhrase,this->phrase); 00094 } 00095 00096 bool Wifly::init() 00097 { 00098 00099 //set uart mode <value> 00100 //Bit Position Function 00101 //0 NOECHO - disables echo of RX data while in 00102 // command mode 00103 //1 DATA TRIGGER makes connection on RX data 00104 //2 Reserved for future use 00105 //3 Enable Sleep on RX BREAK signal 00106 //4 UART RX data buffer. See note below for details* 00107 00108 //no echo: set uart mode <value> 00109 //myDebug.printf("set u m 1\r"); 00110 if (!sendCommand("set u m 1\r", "AOK",NULL,1000)) 00111 { 00112 myDebug.printf("Error: set u m 1\n"); 00113 } // 00114 //continue; 00115 wait_ms(10); 00116 //myDebug.printf("set c t 30\r"); 00117 // set time: set comm timer <30ms> 00118 if (!sendCommand("set c t 30\r", "AOK",NULL,1000)) // 00119 { 00120 myDebug.printf("Error: set c t 30\n"); 00121 } 00122 00123 wait_ms(10); 00124 //myDebug.printf("set c s 1024\r"); 00125 // set size 00126 if (!sendCommand("set c s 1024\r", "AOK",NULL,1000)) 00127 { 00128 myDebug.printf("Error: set c s 1024\n"); 00129 } 00130 00131 00132 wait_ms(10); 00133 //myDebug.printf("set s i 0x40\r"); 00134 // red led on when tcp connection active 00135 if (!sendCommand("set s i 0x40\r", "AOK",NULL,1000)) 00136 { 00137 myDebug.printf("Error: set s i 0x40\n"); 00138 } 00139 00140 wait_ms(100); 00141 // Do not auto auto 00142 00143 if (!sendCommand("set w j 0\r", "AOK",NULL,500) ) 00144 { 00145 myDebug.printf("Error: set w j 0\n"); 00146 } 00147 //continue; 00148 wait_ms(10); 00149 //For the RN module to accept both TCP and UDP, set bits 1 and 2 (value = 3). 00150 if (!sendCommand("set ip p 3\r", "AOK",NULL,500) ) 00151 { 00152 myDebug.printf("Error: set w j 0\n"); 00153 } 00154 00155 /* 00156 wait_ms(10); 00157 //uart baudrate 00158 if (!sendCommand("set uart baud 19200\r", "AOK",NULL,500) ) 00159 { 00160 myDebug.printf("Error: set w j 0\n"); 00161 } 00162 */ 00163 if (!sendCommand("save\r", "Stor")) 00164 { 00165 // sendToDisplay(RS485Cmd,0,"Error: save, Stor\n"); 00166 } 00167 00168 00169 wait_ms(10); 00170 00171 00172 // sendCommand("reboot\r", "ree") ; 00173 // exitCmdMode(); //leave command mode 00174 00175 myDebug.printf("sending Reboot\n"); 00176 //cmd, ack, res, int timeout 00177 if(sendCommand("reboot\r", "Reboot",NULL,5000,false)) 00178 { 00179 //myDebug.printf("DHCP=ON\n"); 00180 } 00181 else 00182 { 00183 //myDebug.printf("DHCP Error\n"); 00184 00185 } 00186 state.cmd_mode = false; 00187 return true; 00188 } 00189 00190 void Wifly::setConnectionType(bool DHCP_STATIC) 00191 { 00192 state.dhcp = DHCP_STATIC; 00193 } 00194 00195 bool Wifly::join (const char *ssid, const char *phrase, int auth) 00196 { 00197 char cmd[200]; 00198 unsigned char retries = 3; 00199 00200 ////////////////////////////////////// 00201 ///auth set wlan auth <value> 00202 sprintf(cmd, "set w a %d\r", auth); 00203 sendCommand(cmd, "AOK"); 00204 00205 00206 wait_ms(10); 00207 ////////////////////////////////////// 00208 ///Pass Phrase 00209 sprintf(cmd, "set w p %s\r", phrase); //Returns 450ms 00210 sendCommand(cmd, "AOK"); 00211 00212 00213 00214 00215 //key step 00216 /* if (state.sec != NONE) 00217 { 00218 myDebug.printf("state.sec != NONE\n"); 00219 //Create command string 00220 if (state.sec == WPA) 00221 { 00222 /////////////////////////////////////////// 00223 ///set wlan phrase <string> 00224 myDebug.printf("set WPA\n"); 00225 sprintf(cmd, "set w p %s\r", phrase); 00226 } 00227 else if (state.sec == WEP_128) 00228 { 00229 myDebug.printf("set WEP\n"); 00230 sprintf(cmd, "set w k %s\r", phrase); 00231 } 00232 00233 if (!sendCommand(cmd, "AOK")) //returns 500ms 00234 { 00235 } 00236 } 00237 */ 00238 00239 wait_ms(10); 00240 00241 if(state.dhcp) 00242 { 00243 state.dhcp = true; 00244 myDebug.printf("Connect DHCP...\n"); 00245 sendCommand("set ip dhcp 1", "AOK",NULL,1000); 00246 wait_ms(10); 00247 sprintf(cmd, "join %s\r", ssid); //join the SSID network 00248 00249 while(retries) 00250 { 00251 if( sendCommand(cmd, "DHCP=ON",NULL,10000,true) ) 00252 { 00253 exitCmdMode(); //exit command mode 00254 return true; 00255 } 00256 else{ 00257 retries--; 00258 //myDebug.printf("Trying to JOIN...\n"); 00259 } 00260 00261 }; 00262 } 00263 else //setup for static IP 00264 { 00265 //myDebug.printf("Connect Static IP\n"); 00266 sprintf(cmd, "set ip a %s\r", this->staticIP); 00267 sendCommand(cmd, "AOK",NULL,2000); 00268 00269 sendCommand("set ip dhcp 0", "AOK",NULL,2000); 00270 00271 state.dhcp = false; 00272 retries = 3; 00273 00274 sprintf(cmd, "join %s\r", ssid); //join the SSID network 00275 while(retries) 00276 { 00277 if( sendCommand(cmd, "Associated",NULL,10000,true) ) 00278 { 00279 exitCmdMode(); //leave command mode 00280 return true; 00281 } 00282 else{ 00283 retries--; 00284 myDebug.printf("Trying to JOIN Static...\n"); 00285 } 00286 }; 00287 } 00288 00289 exitCmdMode(); //leave command mode 00290 00291 return false; //could not connect 00292 } 00293 ///////////////////////////////////////// 00294 //::join() 00295 // 00296 //The SSID and pass pharse are saved 00297 //when the constructor is called 00298 //////////////////////////////////////// 00299 bool Wifly::join () 00300 { 00301 char cmd[200]; 00302 unsigned char retries = 3; 00303 00304 ////////////////////////////////////// 00305 ///auth set wlan auth <value> 00306 sprintf(cmd, "set w a %d\r", state.sec); 00307 sendCommand(cmd, "AOK"); 00308 wait_ms(10); 00309 00310 sprintf(cmd, "set w p %s\r", this->phrase); //Returns 450ms 00311 myDebug.printf(cmd); 00312 sendCommand(cmd, "AOK"); 00313 wait_ms(10); 00314 sendCommand("set ip dhcp 1", "AOK"); 00315 wait_ms(10); 00316 //key step 00317 /* if (state.sec != NONE) 00318 { 00319 myDebug.printf("state.sec != NONE\n"); 00320 //Create command string 00321 if (state.sec == WPA) 00322 { 00323 /////////////////////////////////////////// 00324 ///set wlan phrase <string> 00325 myDebug.printf("set WPA\n"); 00326 sprintf(cmd, "set w p %s\r", phrase); 00327 } 00328 else if (state.sec == WEP_128) 00329 { 00330 myDebug.printf("set WEP\n"); 00331 sprintf(cmd, "set w k %s\r", phrase); 00332 } 00333 00334 if (!sendCommand(cmd, "AOK")) //returns 500ms 00335 { 00336 } 00337 } 00338 */ 00339 00340 wait_ms(10); 00341 00342 if(state.dhcp) 00343 { 00344 myDebug.printf("Connect DHCP...\n"); 00345 sprintf(cmd, "join %s\r", this->ssid); 00346 00347 state.dhcp = true; 00348 while(retries) 00349 { 00350 if( sendCommand(cmd, "DHCP=ON",NULL,10000,true) ) 00351 { 00352 exitCmdMode(); //leave command mode 00353 return true; 00354 } 00355 else{ 00356 retries--; 00357 myDebug.printf("Trying to JOIN...\n"); 00358 } 00359 00360 }; 00361 } 00362 else //setup for static IP 00363 { 00364 //myDebug.printf("Connect Static IP\n"); 00365 sprintf(cmd, "set ip a %s\r", this->staticIP); 00366 sendCommand(cmd, "AOK",NULL,2000); 00367 00368 sendCommand("set ip dhcp 0", "AOK",NULL,2000); 00369 00370 state.dhcp = false; 00371 retries = 3; 00372 00373 sprintf(cmd, "join %s\r", ssid); //join the SSID network 00374 while(retries) 00375 { 00376 if( sendCommand(cmd, "Associated",NULL,10000,true) ) 00377 { 00378 exitCmdMode(); //leave command mode 00379 return true; 00380 } 00381 else{ 00382 retries--; 00383 myDebug.printf("Trying to JOIN Static...\n"); 00384 } 00385 }; 00386 } 00387 00388 exitCmdMode(); //leave command mode 00389 00390 return false; 00391 } 00392 00393 void Wifly::enableDHCP(bool DHCP_STATIC) 00394 { 00395 state.dhcp = DHCP_STATIC; 00396 } 00397 00398 void Wifly::setStaticIP(const char *staticIP) 00399 { 00400 char buffer[100]; 00401 strcpy(this->staticIP,staticIP); 00402 sprintf(buffer,"IPset---: %s\n",this->staticIP); 00403 myDebug.printf(buffer); 00404 00405 } 00406 00407 ///////////////////////////////////////// 00408 //::setBaudRate() 00409 // 00410 //Baudrate can be change on the fly 00411 //Once the command is sent the RN-171 00412 //will exit the command mode 00413 bool Wifly::setBaudRate(int baud) 00414 { 00415 char cmd[60]; 00416 sprintf(cmd, "set u b %i\r", baud); 00417 if ( !sendCommand(cmd, "AOK") ) 00418 { 00419 return false; 00420 } 00421 00422 //Automatically exits command mode 00423 state.cmd_mode = false; 00424 return true; 00425 } 00426 00427 bool Wifly::setProtocol(Protocol p) 00428 { 00429 // use udp auto pairing 00430 char cmd[20]; 00431 sprintf(cmd, "set i p %d\r", p); 00432 if (!sendCommand(cmd, "AOK")) 00433 return false; 00434 00435 switch(p) { 00436 case TCP: 00437 // set ip flags: tcp retry enabled 00438 if (!sendCommand("set i f 0x07\r", "AOK")) 00439 return false; 00440 break; 00441 case UDP: 00442 // set ip flags: udp auto pairing enabled 00443 if (!sendCommand("set i h 0.0.0.0\r", "AOK")) 00444 return false; 00445 if (!sendCommand("set i f 0x40\r", "AOK")) 00446 return false; 00447 break; 00448 } 00449 state.proto = p; 00450 return true; 00451 } 00452 00453 char * Wifly::getStringSecurity() 00454 { 00455 switch(state.sec) { 00456 case NONE: 00457 return "NONE"; 00458 case WEP_128: 00459 return "WEP_128"; 00460 case WPA: 00461 return "WPA"; 00462 } 00463 return "UNKNOWN"; 00464 } 00465 00466 bool Wifly::connect(const char * host, int port) 00467 { 00468 char rcv[20]; 00469 char cmd[20]; 00470 00471 // try to open 00472 sprintf(cmd, "open %s %d\r", host, port); 00473 if (sendCommand(cmd, "OPEN", NULL, 10000)) { 00474 state.tcp = true; 00475 state.cmd_mode = false; 00476 return true; 00477 } 00478 00479 // if failed, retry and parse the response 00480 if (sendCommand(cmd, NULL, rcv, 5000)) { 00481 if (strstr(rcv, "OPEN") == NULL) { 00482 if (strstr(rcv, "Connected") != NULL) { 00483 wait(0.25); 00484 if (!sendCommand("close\r", "CLOS")) 00485 return false; 00486 wait(0.25); 00487 if (!sendCommand(cmd, "OPEN", NULL, 10000)) 00488 return false; 00489 } else { 00490 return false; 00491 } 00492 } 00493 } else { 00494 return false; 00495 } 00496 00497 state.tcp = true; 00498 state.cmd_mode = false; 00499 00500 return true; 00501 } 00502 00503 00504 bool Wifly::gethostbyname(const char * host, char * ip) 00505 { 00506 string h = host; 00507 char cmd[30], rcv[100]; 00508 int l = 0; 00509 char * point; 00510 int nb_digits = 0; 00511 00512 // no dns needed 00513 int pos = h.find("."); 00514 if (pos != string::npos) { 00515 string sub = h.substr(0, h.find(".")); 00516 nb_digits = atoi(sub.c_str()); 00517 } 00518 //printf("substrL %s\r\n", sub.c_str()); 00519 if (count(h.begin(), h.end(), '.') == 3 && nb_digits > 0) { 00520 strcpy(ip, host); 00521 } 00522 // dns needed 00523 else { 00524 nb_digits = 0; 00525 sprintf(cmd, "lookup %s\r", host); 00526 if (!sendCommand(cmd, NULL, rcv)) 00527 return false; 00528 00529 // look for the ip address 00530 char * begin = strstr(rcv, "=") + 1; 00531 for (int i = 0; i < 3; i++) { 00532 point = strstr(begin + l, "."); 00533 DBG("str: %s", begin + l); 00534 l += point - (begin + l) + 1; 00535 } 00536 DBG("str: %s", begin + l); 00537 while(*(begin + l + nb_digits) >= '0' && *(begin + l + nb_digits) <= '9') { 00538 DBG("digit: %c", *(begin + l + nb_digits)); 00539 nb_digits++; 00540 } 00541 memcpy(ip, begin, l + nb_digits); 00542 ip[l+nb_digits] = 0; 00543 DBG("ip from dns: %s", ip); 00544 } 00545 return true; 00546 } 00547 00548 00549 void Wifly::flush() 00550 { 00551 while (wifiSer.readable()) 00552 wifiSer.getc(); 00553 } 00554 00555 //////////////////////////////////////////////////////////// 00556 //sendCommand() 00557 // 00558 // 00559 // 00560 // 00561 // 00562 // 00563 //////////////////////////////////////////////////////////// 00564 bool Wifly::sendCommand(const char * cmd, const char * ack, char * res, int timeout, bool DHCPconn) 00565 { 00566 if (!state.cmd_mode) { 00567 cmdMode(); 00568 } 00569 // Expected res strRet 00570 switch( this->sendString(cmd, strlen(cmd), ack, res, timeout, DHCPconn) ) 00571 { 00572 case 0: 00573 //myDebug.printf("...OK\n"); 00574 return true; 00575 00576 case 1: 00577 // myDebug.printf("Time out\n"); //do a retry 00578 // exitCmdMode(); 00579 return false; 00580 case 2: //DHCP connected 00581 state.associated = true; 00582 myDebug.printf("DHCP CONNECTED\n"); 00583 exitCmdMode(); 00584 return true; 00585 00586 case 3: 00587 myDebug.printf("DHCP Failed\n"); //do a retry 00588 // exitCmdMode(); 00589 return false; 00590 00591 default: 00592 exitCmdMode(); 00593 return false; 00594 00595 00596 }; 00597 return false; 00598 } 00599 00600 bool Wifly::cmdMode() 00601 { 00602 // if already in cmd mode, return 00603 if (state.cmd_mode) 00604 return true; 00605 00606 if ( this->sendString("$$$", 3, "CMD") == -1) 00607 { 00608 ERR("cannot enter in cmd mode\r\n"); 00609 exitCmdMode(); 00610 return false; 00611 } 00612 state.cmd_mode = true; 00613 return true; 00614 } 00615 00616 bool Wifly::disconnect() 00617 { 00618 // if already disconnected, return 00619 if (!state.associated) 00620 return true; 00621 00622 if (!sendCommand("leave\r", "DeAuth")) 00623 return false; 00624 exitCmdMode(); 00625 00626 state.associated = false; 00627 return true; 00628 00629 } 00630 00631 bool Wifly::is_connected() 00632 { 00633 return (tcp_status.read() == 1) ? true : false; 00634 } 00635 00636 00637 bool Wifly::reset(bool howIreset) 00638 { 00639 if(howIreset) 00640 { 00641 reset_pin = 0; 00642 wait(0.2); 00643 reset_pin = 1; 00644 wait(0.2); 00645 } 00646 else 00647 { 00648 // sendToDisplay(RS485Cmd,0,"software Reset\n"); 00649 sendCommand("factory R\r", "Defaults"); 00650 wait_ms(10); 00651 sendCommand("save\r", "Stor"); 00652 00653 return true; 00654 } 00655 00656 return true; 00657 00658 } 00659 00660 bool Wifly::reboot() 00661 { 00662 // if already in cmd mode, return 00663 if ( !sendCommand("reboot\r", "Reboot",NULL,5000,false) ) 00664 return false; 00665 00666 wait(0.3); 00667 00668 state.cmd_mode = false; 00669 return true; 00670 } 00671 00672 00673 ///////////////////////////////////////// 00674 //::close() 00675 // 00676 //disconnect a TCP connection. 00677 //////////////////////////////////////// 00678 bool Wifly::close() 00679 { 00680 // if not connected, return 00681 if (!state.tcp) 00682 return true; 00683 00684 wait(0.25); 00685 if (!sendCommand("close\r", "CLOS")) 00686 return false; 00687 exitCmdMode(); 00688 00689 state.tcp = false; 00690 return true; 00691 } 00692 00693 ///////////////////////////////////////// 00694 //::putc() 00695 // 00696 //Wrapper for wiFiSerial putc() 00697 int Wifly::putc(char c) 00698 { 00699 return wifiSer.putc(c); 00700 } 00701 00702 ///////////////////////////////////////// 00703 //exitCmdMode() 00704 // 00705 //Tell WiFi module to exit command mode 00706 bool Wifly::exitCmdMode() 00707 { 00708 flush(); 00709 ////////////////////////// 00710 ///We flush the buffer 00711 while (wifiSer.readable()) 00712 wifiSer.getc(); 00713 00714 if (!state.cmd_mode) 00715 return true; 00716 00717 if (!sendCommand("exit\r", "EXIT")) 00718 return false; 00719 00720 state.cmd_mode = false; 00721 ////////////////////////// 00722 ///We flush the buffer 00723 while (wifiSer.readable()) 00724 wifiSer.getc(); 00725 00726 return true; 00727 } 00728 00729 ///////////////////////////////////////// 00730 //readable() 00731 // 00732 //BufferedSerial buffer has data? 00733 int Wifly::readable() 00734 { 00735 return wifiSer.readable(); //// note: look if things are in the buffer; 00736 } 00737 00738 00739 ///////////////////////////////////////// 00740 //writeable() 00741 // 00742 //BufferedSerial This call will always 00743 //return true. the cicular queue will 00744 //always allow data 00745 int Wifly::writeable() 00746 { 00747 return wifiSer.writeable(); 00748 } 00749 00750 char Wifly::getc() 00751 { 00752 char c; 00753 00754 c = wifiSer.getc(); 00755 00756 // while (!buf_wifly.available()); 00757 // buf_wifly.dequeue(&c); 00758 return c; 00759 } 00760 00761 ///////////////////////////////////////////////////// 00762 ///These two functions are no longer needed since 00763 ///The BufferedSerial class takes care of this 00764 void Wifly::handler_rx(void) 00765 { 00766 //read characters 00767 // while (wifiSer.readable()) 00768 // buf_wifly.queue(wifiSer.getc()); 00769 } 00770 00771 void Wifly::attach_rx(bool callback) 00772 { 00773 /* 00774 if (!callback) 00775 wifiSer.attach(NULL); 00776 else 00777 wifiSer.attach(this, &Wifly::handler_rx);*/ 00778 } 00779 00780 //////////////////////////////////////////////////////////// 00781 //sendString() 00782 // 00783 // 00784 // 00785 // 00786 // 00787 // 00788 //////////////////////////////////////////////////////////` 00789 int Wifly::sendString(const char * str, int len, const char * ACK, char * res, int timeout,bool DHCPconn) 00790 { 00791 char read; 00792 size_t found = string::npos; //same as -1 substring not found else position of string 00793 size_t DHCPnotCONN = string::npos; 00794 string AUTH_ERR = "AUTH-ERR"; //Error string on DHCP invalid SSID or PAss Phrase 00795 string FAILED = "NONE FAILED"; 00796 string DHCP_CONN = "DHCP=ON"; 00797 string checking; 00798 Timer tmr; 00799 int result = 0; 00800 char buffer[280]; 00801 int pos = 0; 00802 int DHCPconnect = 0; 00803 00804 // DBG("will send: %s\r\n","checka: %s\r\n",); 00805 00806 // attach_rx(false); 00807 //sprintf(buffer,"will send: %s\r\n", str); 00808 //myDebug.printf(buffer); 00809 00810 //We flush the buffer 00811 while (wifiSer.readable()) 00812 wifiSer.getc(); 00813 00814 00815 ////////////////////////////////////////// 00816 ///This is probably a get command 00817 if (!ACK || !strcmp(ACK, "NO")) 00818 { 00819 for (int i = 0; i < len; i++) 00820 { 00821 result = (putc(str[i]) == str[i]) ? result + 1 : result; 00822 } 00823 } 00824 else 00825 { 00826 // myDebug.printf("here1\n"); 00827 //We flush the buffer 00828 while (wifiSer.readable()) 00829 wifiSer.getc(); 00830 00831 tmr.start(); 00832 //////////////////////////////////////// 00833 ///Send command to wiFly transciver 00834 for (int i = 0; i < len; i++) 00835 { 00836 result = (putc(str[i]) == str[i]) ? result + 1 : result; 00837 } 00838 00839 //////////////////////////////////////// 00840 ///Now get expected results 00841 while (1) 00842 { 00843 ////////////////////////////// 00844 ///Time has expired 00845 if (tmr.read_ms() > timeout) 00846 { 00847 // myDebug.printf("timeout!\n"); 00848 //We flush the buffer 00849 while (wifiSer.readable()) 00850 wifiSer.getc(); 00851 00852 00853 sprintf(buffer,"timeout: %s\r\n", checking.c_str()); 00854 myDebug.printf(buffer); 00855 //DBG("check: %s\r\n", checking.c_str()); 00856 00857 // attach_rx(true); 00858 return 1; 00859 } 00860 else if (wifiSer.readable()) 00861 { 00862 read = wifiSer.getc(); 00863 if ( read != '\r' && read != '\n') 00864 { 00865 //////////////////////////////////////// 00866 ///This method is ineficient!!! 00867 checking += read; 00868 found = checking.find(ACK); 00869 00870 00871 //////////////////////////////////////// 00872 ///if a + value string found 00873 if (found != string::npos) 00874 { 00875 wait(0.01); 00876 // sprintf(buffer,"found: %s\n",checking.c_str() ); 00877 // myDebug.printf(buffer); 00878 00879 ////////////////////////// 00880 ///We flush the buffer 00881 while (wifiSer.readable()) 00882 wifiSer.getc(); 00883 00884 if( DHCP_CONN.find(ACK) != string::npos) 00885 { 00886 return 2; //successful DHCP 00887 } 00888 return 0; //found string 0 good 00889 // break; 00890 } 00891 00892 /////////////////////////////////////////////////// 00893 ///Special case check for DHCP attempt connection 00894 if(DHCPconn) 00895 { 00896 DHCPnotCONN = checking.find(AUTH_ERR); 00897 if (DHCPnotCONN != string::npos) 00898 { 00899 wait(0.01); 00900 sprintf(buffer,"AUTH-ERR: %s\n",checking.c_str() ); 00901 myDebug.printf(buffer); 00902 00903 ////////////////////////////// 00904 ///We flush the buffer 00905 while (wifiSer.readable()) 00906 wifiSer.getc(); 00907 00908 return 3; //DHCP Error 00909 // break; 00910 } 00911 00912 DHCPnotCONN = checking.find(FAILED); 00913 if (DHCPnotCONN != string::npos) 00914 { 00915 wait(0.01); 00916 sprintf(buffer,"NONE FAILED: %s\n",checking.c_str() ); 00917 myDebug.printf(buffer); 00918 00919 //We flush the buffer 00920 while (wifiSer.readable()) 00921 wifiSer.getc(); 00922 00923 return 3; 00924 // break; 00925 } 00926 }//if(DHCPconn} 00927 00928 }//if ( read != '\r' && read != '\n') 00929 }//else if (wifiSer.readable()) 00930 } //while (1) 00931 // sprintf(buffer,"check2: %s\r\n", checking.c_str()); 00932 // myDebug.printf(buffer); 00933 // DBG("check: %s\r\n", checking.c_str()); 00934 00935 // attach_rx(true); 00936 return result; 00937 } 00938 00939 //the user wants the result from the command (ACK == NULL, res != NULL) 00940 if ( res != NULL) 00941 { 00942 int i = 0; 00943 Timer timeout; 00944 timeout.start(); 00945 tmr.reset(); 00946 while (1) { 00947 if (timeout.read() > 2) { 00948 if (i == 0) { 00949 res = NULL; 00950 break; 00951 } 00952 res[i] = '\0'; 00953 // DBG("user str 1: %s\r\n", res); 00954 00955 break; 00956 } else { 00957 if (tmr.read_ms() > 300) { 00958 res[i] = '\0'; 00959 // DBG("user str: %s\r\n", res); 00960 00961 break; 00962 } 00963 if (wifiSer.readable()) { 00964 tmr.start(); 00965 read = wifiSer.getc(); 00966 00967 // we drop \r and \n 00968 if ( read != '\r' && read != '\n') { 00969 res[i++] = read; 00970 } 00971 } 00972 } 00973 } 00974 // DBG("user str: %s\r\n", res); 00975 return 0; 00976 } 00977 00978 // //We flush the buffer 00979 // while (wifiSer.readable()) 00980 // wifiSer.getc(); 00981 00982 // attach_rx(true); 00983 //DBG("result: %d\r\n", result) 00984 return 1; 00985 } 00986 00987 /* if( read == ACK[pos] ) 00988 { 00989 pos++; 00990 sprintf(buffer,"cmp: %d\n",pos); 00991 myDebug.printf(buffer); 00992 00993 if(pos == len) 00994 { 00995 myDebug.printf("myCompare-Break\n"); 00996 /////////////////////////// 00997 ///We have a compare 00998 //We flush the buffer 00999 while (wifiSer.readable()) 01000 wifiSer.getc(); 01001 01002 break; 01003 } 01004 }//if( read == ACK[pos] ) 01005 else 01006 { 01007 if(pos == len) 01008 { 01009 myDebug.printf("myCompare2\n"); 01010 } 01011 else 01012 pos = 0; 01013 } 01014 */
Generated on Wed Jul 20 2022 20:24:18 by
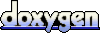