mbed library with additional peripherals for ST F401 board
Fork of mbed-src by
SerialBase.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "SerialBase.h" 00017 #include "wait_api.h" 00018 00019 #if DEVICE_SERIAL 00020 00021 namespace mbed { 00022 00023 SerialBase::SerialBase(PinName tx, PinName rx) { 00024 serial_init(&_serial, tx, rx); 00025 _baud = 9600; 00026 serial_irq_handler(&_serial, SerialBase::_irq_handler, (uint32_t)this); 00027 } 00028 00029 void SerialBase::baud(int baudrate) { 00030 serial_baud(&_serial, baudrate); 00031 _baud = baudrate; 00032 } 00033 00034 void SerialBase::format(int bits, Parity parity, int stop_bits) { 00035 serial_format(&_serial, bits, (SerialParity)parity, stop_bits); 00036 } 00037 00038 int SerialBase::readable() { 00039 return serial_readable(&_serial); 00040 } 00041 00042 00043 int SerialBase::writeable() { 00044 return serial_writable(&_serial); 00045 } 00046 00047 void SerialBase::attach(void (*fptr)(void), IrqType type) { 00048 if (fptr) { 00049 _irq[type].attach(fptr); 00050 serial_irq_set(&_serial, (SerialIrq)type, 1); 00051 } else { 00052 serial_irq_set(&_serial, (SerialIrq)type, 0); 00053 } 00054 } 00055 00056 void SerialBase::_irq_handler(uint32_t id, SerialIrq irq_type) { 00057 SerialBase *handler = (SerialBase*)id; 00058 handler->_irq[irq_type].call(); 00059 } 00060 00061 int SerialBase::_base_getc() { 00062 return serial_getc(&_serial); 00063 } 00064 00065 int SerialBase::_base_putc(int c) { 00066 serial_putc(&_serial, c); 00067 return c; 00068 } 00069 00070 void SerialBase::send_break() { 00071 // Wait for 1.5 frames before clearing the break condition 00072 // This will have different effects on our platforms, but should 00073 // ensure that we keep the break active for at least one frame. 00074 // We consider a full frame (1 start bit + 8 data bits bits + 00075 // 1 parity bit + 2 stop bits = 12 bits) for computation. 00076 // One bit time (in us) = 1000000/_baud 00077 // Twelve bits: 12000000/baud delay 00078 // 1.5 frames: 18000000/baud delay 00079 serial_break_set(&_serial); 00080 wait_us(18000000/_baud); 00081 serial_break_clear(&_serial); 00082 } 00083 00084 #ifdef DEVICE_SERIAL_FC 00085 void SerialBase::set_flow_control(Flow type, PinName flow1, PinName flow2) { 00086 FlowControl flow_type = (FlowControl)type; 00087 switch(type) { 00088 case RTS: 00089 serial_set_flow_control(&_serial, flow_type, flow1, NC); 00090 break; 00091 00092 case CTS: 00093 serial_set_flow_control(&_serial, flow_type, NC, flow1); 00094 break; 00095 00096 case RTSCTS: 00097 case Disabled: 00098 serial_set_flow_control(&_serial, flow_type, flow1, flow2); 00099 break; 00100 00101 default: 00102 break; 00103 } 00104 } 00105 #endif 00106 00107 } // namespace mbed 00108 00109 #endif
Generated on Tue Jul 12 2022 18:09:20 by
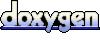