mbed library with additional peripherals for ST F401 board
Fork of mbed-src by
InterruptIn.cpp
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #include "InterruptIn.h" 00017 00018 #if DEVICE_INTERRUPTIN 00019 00020 namespace mbed { 00021 00022 InterruptIn::InterruptIn(PinName pin) { 00023 gpio_irq_init(&gpio_irq, pin, (&InterruptIn::_irq_handler), (uint32_t)this); 00024 gpio_init(&gpio, pin, PIN_INPUT); 00025 } 00026 00027 InterruptIn::~InterruptIn() { 00028 gpio_irq_free(&gpio_irq); 00029 } 00030 00031 int InterruptIn::read() { 00032 return gpio_read(&gpio); 00033 } 00034 00035 void InterruptIn::mode(PinMode pull) { 00036 gpio_mode(&gpio, pull); 00037 } 00038 00039 void InterruptIn::rise(void (*fptr)(void)) { 00040 if (fptr) { 00041 _rise.attach(fptr); 00042 gpio_irq_set(&gpio_irq, IRQ_RISE, 1); 00043 } else { 00044 gpio_irq_set(&gpio_irq, IRQ_RISE, 0); 00045 } 00046 } 00047 00048 void InterruptIn::fall(void (*fptr)(void)) { 00049 if (fptr) { 00050 _fall.attach(fptr); 00051 gpio_irq_set(&gpio_irq, IRQ_FALL, 1); 00052 } else { 00053 gpio_irq_set(&gpio_irq, IRQ_FALL, 0); 00054 } 00055 } 00056 00057 void InterruptIn::_irq_handler(uint32_t id, gpio_irq_event event) { 00058 InterruptIn *handler = (InterruptIn*)id; 00059 switch (event) { 00060 case IRQ_RISE: handler->_rise.call(); break; 00061 case IRQ_FALL: handler->_fall.call(); break; 00062 case IRQ_NONE: break; 00063 } 00064 } 00065 00066 void InterruptIn::enable_irq() { 00067 gpio_irq_enable(&gpio_irq); 00068 } 00069 00070 void InterruptIn::disable_irq() { 00071 gpio_irq_disable(&gpio_irq); 00072 } 00073 00074 #ifdef MBED_OPERATORS 00075 InterruptIn::operator int() { 00076 return read(); 00077 } 00078 #endif 00079 00080 } // namespace mbed 00081 00082 #endif
Generated on Tue Jul 12 2022 18:09:19 by
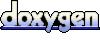