A lib to handle a E-Paper display from Pervasive Displays. There is a interface board from Embedded Artists. The lib can handle graphic and text drawing and is using external fonts.
Dependents: epaper_mbed_130411_KL25Z epaper_mbed_test epaper_KL25Z_2 test_he10 ... more
EPD.h
00001 // Copyright 2013 Pervasive Displays, Inc. 00002 // 00003 // Licensed under the Apache License, Version 2.0 (the "License"); 00004 // you may not use this file except in compliance with the License. 00005 // You may obtain a copy of the License at: 00006 // 00007 // http://www.apache.org/licenses/LICENSE-2.0 00008 // 00009 // Unless required by applicable law or agreed to in writing, 00010 // software distributed under the License is distributed on an 00011 // "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 // express or implied. See the License for the specific language 00013 // governing permissions and limitations under the License. 00014 00015 #ifndef EPD_H 00016 #define EPD_H 00017 00018 #include "mbed.h" 00019 00020 #define PROGMEM 00021 00022 00023 typedef enum { 00024 EPD_1_44, // 128 x 96 00025 EPD_2_0, // 200 x 96 00026 EPD_2_7 // 264 x 176 00027 } EPD_size; 00028 00029 typedef enum { // Image pixel -> Display pixel 00030 EPD_compensate, // B -> W, W -> B (Current Image) 00031 EPD_white, // B -> N, W -> W (Current Image) 00032 EPD_inverse, // B -> N, W -> B (New Image) 00033 EPD_normal // B -> B, W -> W (New Image) 00034 } EPD_stage; 00035 00036 typedef void EPD_reader(void *buffer, uint32_t address, uint16_t length); 00037 00038 class EPD_Class { 00039 private: 00040 DigitalOut EPD_Pin_PANEL_ON; 00041 DigitalOut EPD_Pin_BORDER; 00042 DigitalOut EPD_Pin_DISCHARGE; 00043 PwmOut EPD_Pin_PWM; 00044 DigitalOut EPD_Pin_RESET; 00045 DigitalIn EPD_Pin_BUSY; 00046 DigitalOut EPD_Pin_EPD_CS; 00047 SPI spi_; 00048 00049 EPD_size size; 00050 uint16_t stage_time; 00051 uint16_t factored_stage_time; 00052 uint16_t lines_per_display; 00053 uint16_t dots_per_line; 00054 uint16_t bytes_per_line; 00055 uint16_t bytes_per_scan; 00056 PROGMEM const uint8_t *gate_source; 00057 uint16_t gate_source_length; 00058 PROGMEM const uint8_t *channel_select; 00059 uint16_t channel_select_length; 00060 00061 bool filler; 00062 00063 void SPI_put(uint8_t c); 00064 void SPI_put_wait(uint8_t c, DigitalIn busy_pin); 00065 void SPI_send(DigitalOut cs_pin, const uint8_t *buffer, uint16_t length); 00066 00067 public: 00068 // power up and power down the EPD panel 00069 void begin(); 00070 void end(); 00071 00072 void setFactor(int temperature = 25) { 00073 this->factored_stage_time = this->stage_time * this->temperature_to_factor_10x(temperature) / 10; 00074 } 00075 00076 // clear display (anything -> white) 00077 void clear() { 00078 this->frame_fixed_repeat(0xff, EPD_compensate); 00079 this->frame_fixed_repeat(0xff, EPD_white); 00080 this->frame_fixed_repeat(0xaa, EPD_inverse); 00081 this->frame_fixed_repeat(0xaa, EPD_normal); 00082 } 00083 00084 // assuming a clear (white) screen output an image (PROGMEM data) 00085 void image(const uint8_t *image) { 00086 this->frame_fixed_repeat(0xaa, EPD_compensate); 00087 this->frame_fixed_repeat(0xaa, EPD_white); 00088 this->frame_data_repeat(image, EPD_inverse); 00089 this->frame_data_repeat(image, EPD_normal); 00090 } 00091 00092 // change from old image to new image (PROGMEM data) 00093 void image(const uint8_t *old_image, const uint8_t *new_image) { 00094 this->frame_data_repeat(old_image, EPD_compensate); 00095 this->frame_data_repeat(old_image, EPD_white); 00096 this->frame_data_repeat(new_image, EPD_inverse); 00097 this->frame_data_repeat(new_image, EPD_normal); 00098 } 00099 00100 #if defined(EPD_ENABLE_EXTRA_SRAM) 00101 00102 // change from old image to new image (SRAM version) 00103 void image_sram(const uint8_t *old_image, const uint8_t *new_image) { 00104 this->frame_sram_repeat(old_image, EPD_compensate); 00105 this->frame_sram_repeat(old_image, EPD_white); 00106 this->frame_sram_repeat(new_image, EPD_inverse); 00107 this->frame_sram_repeat(new_image, EPD_normal); 00108 } 00109 #endif 00110 00111 // Low level API calls 00112 // =================== 00113 00114 // single frame refresh 00115 void frame_fixed(uint8_t fixed_value, EPD_stage stage); 00116 void frame_data(const uint8_t *new_image, EPD_stage stage); 00117 #if defined(EPD_ENABLE_EXTRA_SRAM) 00118 void frame_sram(const uint8_t *new_image, EPD_stage stage); 00119 #endif 00120 void frame_cb(uint32_t address, EPD_reader *reader, EPD_stage stage); 00121 00122 // stage_time frame refresh 00123 void frame_fixed_repeat(uint8_t fixed_value, EPD_stage stage); 00124 void frame_data_repeat(const uint8_t *new_image, EPD_stage stage); 00125 #if defined(EPD_ENABLE_EXTRA_SRAM) 00126 void frame_sram_repeat(const uint8_t *new_image, EPD_stage stage); 00127 #endif 00128 void frame_cb_repeat(uint32_t address, EPD_reader *reader, EPD_stage stage); 00129 00130 // convert temperature to compensation factor 00131 int temperature_to_factor_10x(int temperature); 00132 00133 // single line display - very low-level 00134 // also has to handle AVR progmem 00135 void line(uint16_t line, const uint8_t *data, uint8_t fixed_value, bool read_progmem, EPD_stage stage); 00136 00137 // inline static void attachInterrupt(); 00138 // inline static void detachInterrupt(); 00139 00140 EPD_Class(EPD_size size, 00141 PinName panel_on_pin, 00142 PinName border_pin, 00143 PinName discharge_pin, 00144 PinName pwm_pin, 00145 PinName reset_pin, 00146 PinName busy_pin, 00147 PinName chip_select_pin, 00148 PinName mosi, 00149 PinName miso, 00150 PinName sck); 00151 00152 }; 00153 00154 #endif 00155
Generated on Thu Jul 14 2022 09:52:10 by
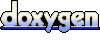