The wait in mci_WaitForEvent will delay all card transactions.
Fork of EALib by
gpdma.h
00001 00002 #ifndef GPDMA_H 00003 #define GPDMA_H 00004 00005 #include "platform.h" 00006 00007 #define GPDMA_CONN_SDC ((1UL)) /*!< SD card */ 00008 00009 typedef enum 00010 { 00011 GPDMA_TRANSFERTYPE_M2M_CONTROLLER_DMA, /* Memory to memory - DMA control */ 00012 GPDMA_TRANSFERTYPE_M2P_CONTROLLER_DMA, /* Memory to peripheral - DMA control */ 00013 GPDMA_TRANSFERTYPE_P2M_CONTROLLER_DMA, /* Peripheral to memory - DMA control */ 00014 GPDMA_TRANSFERTYPE_P2P_CONTROLLER_DMA, /* Source peripheral to destination peripheral - DMA control */ 00015 GPDMA_TRANSFERTYPE_P2P_CONTROLLER_DestPERIPHERAL, /* Source peripheral to destination peripheral - destination peripheral control */ 00016 GPDMA_TRANSFERTYPE_M2P_CONTROLLER_PERIPHERAL, /* Memory to peripheral - peripheral control */ 00017 GPDMA_TRANSFERTYPE_P2M_CONTROLLER_PERIPHERAL, /* Peripheral to memory - peripheral control */ 00018 GPDMA_TRANSFERTYPE_P2P_CONTROLLER_SrcPERIPHERAL, /* Source peripheral to destination peripheral - source peripheral control */ 00019 } gpdma_flowControl_t; 00020 00021 /** 00022 * @brief Initialize the GPDMA 00023 * @param pGPDMA : The base of GPDMA on the chip 00024 * @return Nothing 00025 */ 00026 void gpdma_init(); 00027 00028 /** 00029 * @brief Shutdown the GPDMA 00030 * @param pGPDMA : The base of GPDMA on the chip 00031 * @return Nothing 00032 */ 00033 void gpdma_deinit(); 00034 00035 /** 00036 * @brief Stop a stream DMA transfer 00037 * @param ChannelNum : Channel Number to be closed 00038 * @return Nothing 00039 */ 00040 void gpdma_stop(uint8_t ChannelNum); 00041 00042 /** 00043 * @brief The GPDMA stream interrupt status checking 00044 * @param ChannelNum : Channel Number to be checked on interruption 00045 * @return Status: 00046 * - true : DMA transfer success 00047 * - false : DMA transfer failed 00048 */ 00049 bool gpdma_interrupt(uint8_t ChannelNum); 00050 00051 /** 00052 * @brief Get a free GPDMA channel for one DMA connection 00053 * @param pCh : Assigned channel number (only valid if success) 00054 * @return Status: 00055 * - true : Found a free DMA channel 00056 * - false : No free DMA channels, pCh value is undefined 00057 */ 00058 bool gpdma_getFreeChannel(uint8_t* pCh); 00059 00060 /** 00061 * @brief Do a DMA transfer M2M, M2P,P2M or P2P 00062 * @param ChannelNum : Channel used for transfer 00063 * @param src : Address of Memory or PeripheralConnection_ID which is the source 00064 * @param dst : Address of Memory or PeripheralConnection_ID which is the destination 00065 * @param TransferType: Select the transfer controller and the type of transfer. Should be: 00066 * - GPDMA_TRANSFERTYPE_M2M_CONTROLLER_DMA 00067 * - GPDMA_TRANSFERTYPE_M2P_CONTROLLER_DMA 00068 * - GPDMA_TRANSFERTYPE_P2M_CONTROLLER_DMA 00069 * - GPDMA_TRANSFERTYPE_P2P_CONTROLLER_DMA 00070 * - GPDMA_TRANSFERTYPE_P2P_CONTROLLER_DestPERIPHERAL 00071 * - GPDMA_TRANSFERTYPE_M2P_CONTROLLER_PERIPHERAL 00072 * - GPDMA_TRANSFERTYPE_P2M_CONTROLLER_PERIPHERAL 00073 * - GPDMA_TRANSFERTYPE_P2P_CONTROLLER_SrcPERIPHERAL 00074 * @param Size : The number of DMA transfers 00075 * @return False on error, true on success 00076 */ 00077 //bool gpdma_transfer(uint8_t ChannelNum, 00078 // uint32_t src, 00079 // uint32_t dst, 00080 // gpdma_flowControl_t TransferType, 00081 // uint32_t Size); 00082 00083 bool gpdma_transfer_to_mci(uint8_t ChannelNum, 00084 uint32_t src, 00085 uint32_t Size); 00086 bool gpdma_transfer_from_mci(uint8_t ChannelNum, 00087 uint32_t dst, 00088 uint32_t Size); 00089 #endif 00090 00091
Generated on Tue Jul 12 2022 15:13:41 by
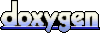