The wait in mci_WaitForEvent will delay all card transactions.
Fork of EALib by
TSC2046.h
00001 00002 #ifndef TSC2046_H 00003 #define TSC2046_H 00004 00005 #include "TouchPanel.h" 00006 00007 #define TSC2046_NUM_CALIB_POINTS (3) 00008 00009 /** 00010 * Texas Instruments Touch Screen Controller (TSC2046). 00011 */ 00012 class TSC2046 : public TouchPanel { 00013 public: 00014 00015 00016 /** 00017 * Constructor 00018 * 00019 * @param mosi SPI MOSI pin 00020 * @param miso SPI MISO pin 00021 * @param sck SPI SCK pin 00022 * @param cs chip-select pin 00023 */ 00024 TSC2046(PinName mosi, PinName miso, PinName sck, PinName cs); 00025 00026 virtual bool init(uint16_t width, uint16_t height); 00027 00028 virtual bool read(touchCoordinate_t &coord); 00029 virtual bool calibrateStart(); 00030 virtual bool getNextCalibratePoint(uint16_t* x, uint16_t* y); 00031 virtual bool waitForCalibratePoint(bool* morePoints, uint32_t timeout); 00032 00033 /** 00034 * Calibrate the touch panel with already gathered calibration values. 00035 * The list with calibration points must at one point have been retrieved 00036 * by calling getCalibrationValues; 00037 * 00038 * @param values list with calibration values 00039 * @param numValues the size of the list must match the number of 00040 * calibration points needed for this touch panel (TSC2046_NUM_CALIB_POINTS) 00041 * 00042 * @return true if the request was successful; otherwise false 00043 */ 00044 bool calibrate(touchCoordinate_t* values, int numValues); 00045 00046 /** 00047 * Get calibration values for the calibration points used by this touch 00048 * panel. This method may only be called after a successful calibration 00049 * has been performed. 00050 * 00051 * The list with values can be written to persistent storage and used 00052 * to calibrate the display without involving the user. 00053 * 00054 * @param values calibration values will be written to this list 00055 * @param numValues the size of the list must match the number of 00056 * calibration points needed for this touch panel (TSC2046_NUM_CALIB_POINTS) 00057 * 00058 * @return true if the request was successful; otherwise false 00059 */ 00060 bool getCalibrationValues(touchCoordinate_t* values, int numValues); 00061 00062 00063 private: 00064 00065 typedef struct { 00066 int64_t x; 00067 int64_t y; 00068 } calibPoint_t; 00069 00070 typedef struct { 00071 int64_t An; 00072 int64_t Bn; 00073 int64_t Cn; 00074 int64_t Dn; 00075 int64_t En; 00076 int64_t Fn; 00077 int64_t Divider; 00078 } calibMatrix_t; 00079 00080 SPI _spi; 00081 DigitalOut _cs; 00082 bool _calibrated; 00083 bool _initialized; 00084 calibMatrix_t _calibMatrix; 00085 00086 uint16_t _width; 00087 uint16_t _height; 00088 int _calibPoint; 00089 int _insetPx; 00090 00091 touchCoordinate_t _calibrateValues[TSC2046_NUM_CALIB_POINTS][2]; 00092 00093 void readAndFilter(touchCoordinate_t &coord); 00094 int32_t getFilteredValue(int cmd); 00095 uint16_t spiTransfer(uint8_t cmd); 00096 00097 void calibrate(touchCoordinate_t &ref1, 00098 touchCoordinate_t &ref2, 00099 touchCoordinate_t &ref3, 00100 touchCoordinate_t &scr1, 00101 touchCoordinate_t &scr2, 00102 touchCoordinate_t &scr3); 00103 00104 void getCalibratePoint(int pointNum, int32_t* x, int32_t *y); 00105 int waitForTouch(int32_t* x, int32_t* y, uint32_t timeout); 00106 00107 00108 00109 int setCalibrationMatrix( calibPoint_t * displayPtr, 00110 calibPoint_t * screenPtr, 00111 calibMatrix_t * matrixPtr); 00112 int getDisplayPoint( calibPoint_t * displayPtr, 00113 calibPoint_t * screenPtr, 00114 calibMatrix_t * matrixPtr ); 00115 00116 00117 00118 }; 00119 00120 #endif
Generated on Tue Jul 12 2022 15:13:41 by
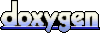