The wait in mci_WaitForEvent will delay all card transactions.
Fork of EALib by
SPIFI.h
00001 00002 #ifndef SPIFI_H 00003 #define SPIFI_H 00004 00005 #include "mbed.h" 00006 #include "spifi_rom_api.h" 00007 00008 /** 00009 * SPIFI Example 00010 * 00011 * @code 00012 * #include "mbed.h" 00013 * #include "SPIFI.h" 00014 * 00015 * int main(void) { 00016 * SPIFI::SpifiError err; 00017 * 00018 * err = SPIFI::instance().init(); 00019 * if (err != SPIFI::Ok) { 00020 * printf("Failed to initialize SPIFI, error %d\n", err); 00021 * } 00022 * 00023 * // Write "Hello World!" into the first bytes of the SPIFI 00024 * char buff[20] = "Hello World!"; 00025 * err = SPIFI::instance().program(0, strlen(buff)+1, buff, SPIFI::EraseAsRequired); 00026 * if (err != SPIFI::Ok) { 00027 * printf("Failed to write to SPIFI, error %d\n", err); 00028 * } 00029 * 00030 * // Now verify that it can be read 00031 * if (memcmp((char*)SPIFI::SpifiMemBase, buff, strlen(buff)+1) == 0) { 00032 * printf("Readback from memory OK: '%s'\n", SPIFI::SpifiMemBase); 00033 * } else { 00034 * printf("Spifi does not contain the correct data!\n"); 00035 * } 00036 * } 00037 * @endcode 00038 */ 00039 class SPIFI { 00040 public: 00041 00042 enum Constants { 00043 SpifiMemBase = 0x28000000 00044 }; 00045 00046 enum Options { 00047 ForceErase = (0<<2), 00048 EraseAsRequired = (1<<2), 00049 CallerErase = (1<<3) 00050 }; 00051 00052 enum SpifiError { 00053 Ok = 0, 00054 Uninitialized = 1, 00055 Verification = 2, 00056 SameAddress = 3, 00057 UnknownError = 4, 00058 InternalError = 0x20002, 00059 Timeout = 0x20003, 00060 OperandError = 0x20004, 00061 Status = 0x20005, 00062 ExtDeviceId = 0x20006, 00063 DeviceId = 0x20007, 00064 DeviceType = 0x20008, 00065 Manufacturer = 0x20009, 00066 InvalidJDECId = 0x2000A, 00067 EraseConflict = 0x2000B, 00068 }; 00069 00070 enum Device { 00071 Spansion_S25FL032, /* Manufacturer: 0x01, devType: 0x02, devID: 0x15 */ 00072 Winbond_W25Q64FV, /* Manufacturer: 0xEF, devType: 0x40, devID: 0x17 */ 00073 UnknownDevice 00074 }; 00075 00076 static SPIFI& instance() 00077 { 00078 static SPIFI singleton; 00079 return singleton; 00080 } 00081 00082 00083 /** Initializes the SPIFI ROM driver making the content of the external serial flash available 00084 * 00085 * @returns 00086 * Ok on success 00087 * An error code on failure 00088 */ 00089 SpifiError init(); 00090 00091 /** Returns the detected external serial flash 00092 * 00093 * @returns 00094 * The detected device or UnknownDevice 00095 */ 00096 Device device() { return _device; } 00097 00098 /** Returns the size (in bytes) of the external serial flash 00099 * 00100 * @returns 00101 * The size in bytes 00102 */ 00103 uint32_t memorySize() { return _memorySize; } 00104 00105 /** Returns the size of an erase block (in bytes) on the external serial flash 00106 * 00107 * @returns 00108 * The erase block size in bytes 00109 */ 00110 uint32_t eraseBlockSize() { return _eraseBlockSize; } 00111 00112 /** Returns the address where the verifcation failed. Use only after a Verification error code 00113 * has been retured from one of the other functions 00114 * 00115 * @returns 00116 * The address to where the program or erase operation failed 00117 */ 00118 uint32_t getVerificationErrorAddr() { return _verError; } 00119 00120 /** Used by the QSPIFileSystem class to get access to all spifi internal data. 00121 * 00122 * @param initData The parameter returned by spifi_init 00123 * @param romPtr The location of the SPIFI ROM driver in memory 00124 */ 00125 void internalData(SPIFIobj** initData, const SPIFI_RTNS** romPtr) { *initData = _romData; *romPtr = _spifi; } 00126 00127 /** Copies len data from src to dest. This function will split len > 256 into writes of 256 bytes each. 00128 * 00129 * Programming means that a bit can either be left at 0, or programmed from 1 to 0. Changing bits from 0 to 1 requires an erase operation. 00130 * While bits can be individually programmed from 1 to 0, erasing bits from 0 to 1 must be done in larger chunks (manufacturer dependant 00131 * but typically 4Kbyte to 64KByte at a time). 00132 * 00133 * Specify how/when erasing is done with the options parameter: 00134 * 00135 * - ForceErase causes len bytes to be erased starting at dest. If a scratch buffer (must be the size of an erase block) is 00136 * provided then only the len bytes will be erased. If no scratch buffer is provided then the erase block surrounding 00137 * dest will be erased. 00138 * 00139 * - EraseAsRequired tests if the destination can be written to without erasing. If it can then no erasing is done. If it 00140 * can't then behaviour is the same as if ForceErase was specified. 00141 * 00142 * - CallerErase tests if the destination can be written to without erasing. If it can then a data is written. If it 00143 * can't then an error code is returned and nothing is written. 00144 * 00145 * Scratch should be NULL or the address of an area of RAM that the SPIFI driver can use 00146 * to save data during erase operations. If provided, the scratch area should be as large 00147 * as the smallest erase size that is available throughout the serial flash device. If scratch 00148 * is NULL (zero) and an erase is necessary, any bytes in the first erase block before dest 00149 * are left in erased state (all ones), as are any bytes in the last erase block after dest + len 00150 * 00151 * @param dest Address to write to, highest byte must be 0x00 or 0x28 as in 0x28000000 00152 * @param len Number of bytes to write 00153 * @param src Data to write 00154 * @param options How to handle content of destination 00155 * @param verify Should all writes be verified or not 00156 * @param scratch Used with ForceErase and EraseAsRequired option to preserve content of flash outside of 00157 * written area. Size of buffer must be at least the size of one erase block. 00158 * 00159 * @returns 00160 * Ok on success 00161 * An error code on failure 00162 */ 00163 SpifiError program(uint32_t dest, unsigned len, char* src, Options options, bool verify=false, char* scratch=NULL); 00164 00165 /** Erases the content of the memory at the specified address 00166 * 00167 * Scratch should be NULL or the address of an area of RAM that the SPIFI driver can use 00168 * to save data during erase operations. If provided, the scratch area should be as large 00169 * as the smallest erase size that is available throughout the serial flash device. If scratch 00170 * is NULL (zero) and an erase is necessary, any bytes in the first erase block before dest 00171 * are left in erased state (all ones), as are any bytes in the last erase block after dest + len 00172 * 00173 * @param dest Address to start erasing at, highest byte must be 0x00 or 0x28 as in 0x28000000 00174 * @param len Number of bytes to erase 00175 * @param verify Should the erased area be verified to make sure it is all 0xff 00176 * @param scratch Used to preserve content of flash outside of erased area. 00177 * Size of buffer must be at least the size of one erase block. 00178 * 00179 * @returns 00180 * Ok on success 00181 * An error code on failure 00182 */ 00183 SpifiError erase(uint32_t dest, unsigned len, char* src, bool verify=false, char* scratch=NULL); 00184 00185 00186 private: 00187 00188 uint32_t _verError; 00189 00190 bool _initialized; 00191 00192 Device _device; 00193 uint32_t _memorySize; 00194 uint32_t _eraseBlockSize; 00195 00196 SPIFIobj* _romData; 00197 const SPIFI_RTNS* _spifi; 00198 00199 char _addrConflictBuff[256]; 00200 00201 explicit SPIFI(); 00202 // hide copy constructor 00203 SPIFI(const SPIFI&); 00204 // hide assign operator 00205 SPIFI& operator=(const SPIFI&); 00206 ~SPIFI(); 00207 00208 SpifiError translateError(int err, bool verify = false); 00209 }; 00210 00211 #endif 00212
Generated on Tue Jul 12 2022 15:13:41 by
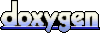