The wait in mci_WaitForEvent will delay all card transactions.
Fork of EALib by
MMA7455.h
00001 00002 #ifndef MMA7455_H 00003 #define MMA7455_H 00004 00005 00006 /** 00007 * Freescale Accelerometer MMA7455. 00008 */ 00009 class MMA7455 { 00010 public: 00011 00012 enum Mode { 00013 ModeStandby = 0, 00014 ModeMeasurement = 1, 00015 }; 00016 00017 /** Acceleration range */ 00018 enum Range { 00019 Range_8g = 0, 00020 Range_2g = 1, 00021 Range_4g = 2 00022 }; 00023 00024 /** 00025 * Create an interface to the MMA7455 accelerometer 00026 * 00027 * @param sda I2C data line pin 00028 * @param scl I2C clock line pin 00029 */ 00030 MMA7455(PinName sda, PinName scl); 00031 00032 bool setMode(Mode mode); 00033 bool setRange(Range range); 00034 00035 bool read(int32_t& x, int32_t& y, int32_t& z); 00036 00037 /** 00038 * Calibrate for 0g, that is, calculate offset to achieve 00039 * 0g values when accelerometer is placed on flat surface. 00040 * 00041 * Please make sure the accelerometer is placed on a flat surface before 00042 * calling this function. 00043 * 00044 * @return true if request was successful; otherwise false 00045 */ 00046 bool calibrate(); 00047 00048 /** 00049 * Get calculated offset values. Offsets will be calculated by the 00050 * calibrate() method. 00051 * 00052 * Use these values and put them in persistent storage to avoid 00053 * having to calibrate the accelerometer after a reset/power cycle. 00054 * 00055 * @param xOff x offset is written to this argument 00056 * @param yOff y offset is written to this argument 00057 * @param zOff z offset is written to this argument 00058 * 00059 * @return true if request was successful; otherwise false 00060 */ 00061 bool getCalibrationOffsets(int32_t& xOff, int32_t& yOff, int32_t& zOff); 00062 00063 /** 00064 * Set calibration offset values. These values should normally 00065 * at one point in time have been retrieved by calling the 00066 * getCalibrationOffsets method. 00067 * 00068 * 00069 * @param xOff x offset 00070 * @param yOff y offset 00071 * @param zOff z offset 00072 * 00073 * @return true if request was successful; otherwise false 00074 */ 00075 bool setCalibrationOffsets(int32_t xOff, int32_t yOff, int32_t zOff); 00076 00077 00078 00079 private: 00080 00081 I2C _i2c; 00082 Mode _mode; 00083 Range _range; 00084 int32_t _xOff; 00085 int32_t _yOff; 00086 int32_t _zOff; 00087 00088 int getStatus(); 00089 int getModeControl(); 00090 int setModeControl(uint8_t mctl); 00091 00092 }; 00093 00094 #endif
Generated on Tue Jul 12 2022 15:13:41 by
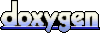