The wait in mci_WaitForEvent will delay all card transactions.
Fork of EALib by
GFXFb.h
00001 00002 #ifndef GFXFB_H 00003 #define GFXFB_H 00004 00005 #include "Adafruit_GFX.h" 00006 00007 #define BLACK 0x0000 00008 /* Light gray color, 565 mode */ 00009 #define LIGHTGRAY 0X7BEF 00010 /* Dark gray color, 565 mode */ 00011 #define DARKGRAY 0x39E7 00012 /* White color, 565 mode */ 00013 #define WHITE 0xffff 00014 /* Red color, 565 mode */ 00015 #define RED 0xF800 00016 /* Green color, 565 mode */ 00017 #define GREEN 0x07E0 00018 /* Blue color, 565 mode */ 00019 #define BLUE 0x001F 00020 /* Magenta color, 565 mode */ 00021 #define MAGENTA (RED | BLUE) 00022 /* Cyan color, 565 mode */ 00023 #define CYAN (GREEN | BLUE) 00024 /* Yellow color, 565 mode */ 00025 #define YELLOW (RED | GREEN) 00026 /* Light red color, 565 mode */ 00027 #define LIGHTRED 0x7800 00028 /* Light green color, 565 mode */ 00029 #define LIGHTGREEN 0x03E0 00030 /* Light blue color, 565 mode */ 00031 #define LIGHTBLUE 0x000F 00032 /* Light magenta color, 565 mode */ 00033 #define LIGHTMAGENTA (LIGHTRED | LIGHTBLUE) 00034 /* Light cyan color, 565 mode */ 00035 #define LIGHTCYAN (LIGHTGREEN | LIGHTBLUE) 00036 /* Light yellow color, 565 mode */ 00037 #define LIGHTYELLOW (LIGHTRED | LIGHTGREEN) 00038 00039 /** 00040 * Graphical library based on Adafruit's GFX using a Frame buffer. 00041 */ 00042 class GFXFb : public Adafruit_GFX { 00043 public: 00044 00045 /** Create an interface to the GFX graphical library 00046 * 00047 * @param w width of the display 00048 * @param h height of the display 00049 * @param fb frame buffer that will be used by the graphical library. Can 00050 * be set by calling setFb instead. 00051 */ 00052 GFXFb(uint16_t w, uint16_t h, uint16_t* fb = 0); 00053 00054 virtual void drawPixel(int16_t x, int16_t y, uint16_t color); 00055 virtual void drawFastVLine(int16_t x, int16_t y, int16_t h, uint16_t color); 00056 virtual void drawFastHLine(int16_t x, int16_t y, int16_t w, uint16_t color); 00057 virtual void fillScreen(uint16_t color); 00058 00059 /** Associate a frame buffer with the graphical library. 00060 * All drawing requests will be performed on the frame buffer. 00061 * 00062 * @param fb frame buffer that will be used by the graphical library 00063 */ 00064 void setFb(uint16_t* fb) {_fb = fb;} 00065 00066 /** Get the frame buffer associated with the graphical library. 00067 * 00068 * @returns the frame buffer associated with the graphical library. 00069 */ 00070 uint16_t* getFb() {return _fb;} 00071 00072 /** Write a null-terminated string 00073 * 00074 * @param s the string to write on the display 00075 */ 00076 void writeString(const char* s); 00077 00078 /** Get the width in pixels of the given string. 00079 * 00080 * @param s width will be calculated on this string 00081 * @returns the width in pixels of the string 00082 */ 00083 int16_t getStringWidth(const char* s); 00084 00085 /** Get the height in pixels of the given string. 00086 * 00087 * @param s height will be calculated on this string 00088 * @returns the height in pixels of the string 00089 */ 00090 int16_t getStringHeight(const char* s); 00091 00092 00093 private: 00094 00095 uint16_t* _fb; 00096 }; 00097 00098 #endif 00099 00100
Generated on Tue Jul 12 2022 15:13:41 by
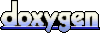