The wait in mci_WaitForEvent will delay all card transactions.
Fork of EALib by
EaLcdBoard.h
00001 00002 #ifndef EALCDBOARD_H 00003 #define EALCDBOARD_H 00004 00005 #include "LcdController.h" 00006 00007 /** An interface to Embedded Artists LCD Boards 00008 * 00009 */ 00010 class EaLcdBoard { 00011 public: 00012 00013 enum Result { 00014 Ok = 0, 00015 InvalidCommandString, 00016 InvalidArgument, 00017 InvalidStorage, 00018 BufferTooSmall, 00019 VersionNotSupported, 00020 LcdAccessError 00021 }; 00022 00023 enum Constants { 00024 NameBufferSize = 30 00025 }; 00026 00027 enum TouchPanelId { 00028 // must be first 00029 TouchPanelInvalidFirst = 0x80, 00030 00031 // Texas Instruments Touch Screen Controller (TSC2046). 00032 TouchPanel_TSC2046, 00033 // Microchip Touch Screen Controller (AR1021). 00034 TouchPanel_AR1021, 00035 00036 // Must be after last panel ID 00037 TouchPanelInvalidLast, 00038 // unknown touch panel 00039 TouchPanelUnknown = 0xFF 00040 00041 }; 00042 00043 typedef struct { 00044 uint8_t swap; // set to 1 if x and y should be swapped 00045 uint32_t xres; // x resistance 00046 uint32_t yres; // y resistance 00047 TouchPanelId panelId; // identifies touch panel 00048 00049 } TouchParams_t; 00050 00051 /** Create an interface to an Embedded Artists LCD Board 00052 * 00053 * @param sda I2C data line pin 00054 * @param scl I2C clock line pin 00055 */ 00056 EaLcdBoard(PinName sda, PinName scl); 00057 00058 /** Open the interface and start initialization. 00059 * 00060 * @param cfg initialize with a given LCD configuration. If this argument is 00061 * NULL the LCD configuration will be retrieved from persistent 00062 * storage on the LCD Board. 00063 * @param initSeq the initialization string. If this argument is NULL the 00064 * initialization string will be retrieved from persistent 00065 * storage on the LCD Board. 00066 * 00067 * @returns the result of the operation 00068 */ 00069 Result open(LcdController::Config* cfg, char* initSeq); 00070 00071 /** Close the interface 00072 * 00073 * @returns the result of the operation 00074 */ 00075 Result close(); 00076 00077 /** Set and activate the address of the frame buffer to use. 00078 * 00079 * It is the content of the frame buffer that is shown on the 00080 * display. All the drawing on the frame buffer can be done 00081 * 'offline' and whenever it should be shown this function 00082 * can be called with the address of the offline frame buffer. 00083 * 00084 * @param address Memory address of the frame buffer 00085 * 00086 * @returns the result of the operation 00087 */ 00088 Result setFrameBuffer(uint32_t address); 00089 00090 /** Get the LCD configuration stored in persistent storage on the LCD Board 00091 * 00092 * @param cfg pointer to a configuration object. Parameters are copied to 00093 * this object. 00094 * 00095 * @returns the result of the operation 00096 */ 00097 Result getLcdConfig(LcdController::Config* cfg); 00098 00099 /** Get the display name stored in persistent storage on the LCD Board 00100 * 00101 * @param buf buffer to which the name will be copied 00102 * @param len size of the buffer in bytes 00103 * 00104 * @returns the result of the operation 00105 */ 00106 Result getDisplayName(char* buf, int len); 00107 00108 /** Get the display manufacturer stored in persistent storage on the 00109 * LCD Board 00110 * 00111 * @param buf buffer to which the name will be copied 00112 * @param len size of the buffer in bytes 00113 * 00114 * @returns the result of the operation 00115 */ 00116 Result getDisplayMfg(char* buf, int len); 00117 00118 /** Get the initialization sequence stored in persistent storage on the 00119 * LCD Board 00120 * 00121 * @param buf buffer to which the string will be copied 00122 * @param len size of the buffer in bytes 00123 * 00124 * @returns the result of the operation 00125 */ 00126 Result getInitSeq(char* buf, int len); 00127 00128 /** Get the power down sequence stored in persistent storage on the 00129 * LCD Board 00130 * 00131 * @param buf buffer to which the string will be copied 00132 * @param len size of the buffer in bytes 00133 * 00134 * @returns the result of the operation 00135 */ 00136 Result getPowerDownSeq(char* buf, int len); 00137 00138 /** 00139 * Get the touch panel parameters stored in persistent storage 00140 * 00141 * @param params pointer to a configuration object. Parameters are copied to 00142 * this object. 00143 * 00144 * @returns the result of the operation 00145 */ 00146 Result getTouchParameters(TouchParams_t* params); 00147 00148 /** 00149 * Write display parameters to the EEPROM. Please use this function with 00150 * care since original parameters will be overwritten and cannot be restored. 00151 * 00152 * @param lcdName the name of the display 00153 * @param lcdMfg the display manufacturer 00154 * @param cfg the display configuration parameters 00155 * @param initSeqStr the initialization sequence string 00156 * @param pdSeqStr the power down sequence string 00157 * @param touch touch panel parameters 00158 */ 00159 Result storeParameters( 00160 const char* lcdName, 00161 const char* lcdMfg, 00162 LcdController::Config* cfg, 00163 const char* initSeqStr, 00164 const char* pdSeqStr, 00165 TouchParams_t* touch, 00166 bool controlWp = false); 00167 00168 00169 private: 00170 00171 typedef struct { 00172 uint32_t magic; // magic number 00173 uint8_t lcd_name[NameBufferSize]; // LCD name 00174 uint8_t lcd_mfg[NameBufferSize]; // manufacturer name 00175 uint16_t lcdParamOff; // offset to LCD parameters 00176 uint16_t initOff; // offset to init sequence string 00177 uint16_t pdOff; // offset to power down sequence string 00178 uint16_t tsOff; // offset to touch parameters 00179 uint16_t end; // end offset 00180 } store_t; 00181 00182 Result getStore(store_t* store); 00183 00184 int eepromRead(uint8_t* buf, uint16_t offset, uint16_t len); 00185 int eepromWrite(uint8_t* buf, uint16_t offset, uint16_t len); 00186 00187 Result parseInitString(char* str, LcdController::Config* cfg); 00188 Result checkVersion(char* v, uint32_t len); 00189 Result execDelay(char* del, uint32_t len); 00190 Result execSeqCtrl(char* cmd, uint32_t len); 00191 Result execPinSet(char* cmd, uint32_t len); 00192 00193 void setLsStates(uint16_t states, uint8_t* ls, uint8_t mode); 00194 void setLeds(void); 00195 void pca9532_setLeds (uint16_t ledOnMask, uint16_t ledOffMask); 00196 void pca9532_setBlink0Period(uint8_t period); 00197 void pca9532_setBlink0Duty(uint8_t duty); 00198 void pca9532_setBlink0Leds(uint16_t ledMask); 00199 00200 void setWriteProtect(bool enable); 00201 void set3V3Signal(bool enabled); 00202 void set5VSignal(bool enabled); 00203 void setDisplayEnableSignal(bool enabled); 00204 void setBacklightContrast(uint32_t value); 00205 00206 I2C _i2c; 00207 LcdController::Config _cfg; 00208 LcdController lcdCtrl; 00209 uint16_t _blink0Shadow; 00210 uint16_t _blink1Shadow; 00211 uint16_t _ledStateShadow; 00212 bool _lcdPwrOn; 00213 }; 00214 00215 #endif
Generated on Tue Jul 12 2022 15:13:41 by
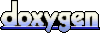