
Example of UART-DMA transfers taken form the npx cmsis driver libary
Embed:
(wiki syntax)
Show/hide line numbers
lpc17xx_uart.h
Go to the documentation of this file.
00001 /***********************************************************************//** 00002 * @file lpc17xx_uart.h 00003 * @brief Contains all macro definitions and function prototypes 00004 * support for UART firmware library on LPC17xx 00005 * @version 3.0 00006 * @date 18. June. 2010 00007 * @author NXP MCU SW Application Team 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup UART UART 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef __LPC17XX_UART_H 00028 #define __LPC17XX_UART_H 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "LPC17xx.h" 00032 #include "lpc_types.h" 00033 00034 00035 #ifdef __cplusplus 00036 extern "C" 00037 { 00038 #endif 00039 00040 /* Public Macros -------------------------------------------------------------- */ 00041 /** @defgroup UART_Public_Macros UART Public Macros 00042 * @{ 00043 */ 00044 00045 /** UART time-out definitions in case of using Read() and Write function 00046 * with Blocking Flag mode 00047 */ 00048 #define UART_BLOCKING_TIMEOUT (0xFFFFFFFFUL) 00049 00050 /** 00051 * @} 00052 */ 00053 00054 /* Private Macros ------------------------------------------------------------- */ 00055 /** @defgroup UART_Private_Macros UART Private Macros 00056 * @{ 00057 */ 00058 00059 /* Accepted Error baud rate value (in percent unit) */ 00060 #define UART_ACCEPTED_BAUDRATE_ERROR (3) /*!< Acceptable UART baudrate error */ 00061 00062 00063 /* --------------------- BIT DEFINITIONS -------------------------------------- */ 00064 /*********************************************************************//** 00065 * Macro defines for Macro defines for UARTn Receiver Buffer Register 00066 **********************************************************************/ 00067 #define UART_RBR_MASKBIT ((uint8_t)0xFF) /*!< UART Received Buffer mask bit (8 bits) */ 00068 00069 /*********************************************************************//** 00070 * Macro defines for Macro defines for UARTn Transmit Holding Register 00071 **********************************************************************/ 00072 #define UART_THR_MASKBIT ((uint8_t)0xFF) /*!< UART Transmit Holding mask bit (8 bits) */ 00073 00074 /*********************************************************************//** 00075 * Macro defines for Macro defines for UARTn Divisor Latch LSB register 00076 **********************************************************************/ 00077 #define UART_LOAD_DLL(div) ((div) & 0xFF) /**< Macro for loading least significant halfs of divisors */ 00078 #define UART_DLL_MASKBIT ((uint8_t)0xFF) /*!< Divisor latch LSB bit mask */ 00079 00080 /*********************************************************************//** 00081 * Macro defines for Macro defines for UARTn Divisor Latch MSB register 00082 **********************************************************************/ 00083 #define UART_DLM_MASKBIT ((uint8_t)0xFF) /*!< Divisor latch MSB bit mask */ 00084 #define UART_LOAD_DLM(div) (((div) >> 8) & 0xFF) /**< Macro for loading most significant halfs of divisors */ 00085 00086 /*********************************************************************//** 00087 * Macro defines for Macro defines for UART interrupt enable register 00088 **********************************************************************/ 00089 #define UART_IER_RBRINT_EN ((uint32_t)(1<<0)) /*!< RBR Interrupt enable*/ 00090 #define UART_IER_THREINT_EN ((uint32_t)(1<<1)) /*!< THR Interrupt enable*/ 00091 #define UART_IER_RLSINT_EN ((uint32_t)(1<<2)) /*!< RX line status interrupt enable*/ 00092 #define UART1_IER_MSINT_EN ((uint32_t)(1<<3)) /*!< Modem status interrupt enable */ 00093 #define UART1_IER_CTSINT_EN ((uint32_t)(1<<7)) /*!< CTS1 signal transition interrupt enable */ 00094 #define UART_IER_ABEOINT_EN ((uint32_t)(1<<8)) /*!< Enables the end of auto-baud interrupt */ 00095 #define UART_IER_ABTOINT_EN ((uint32_t)(1<<9)) /*!< Enables the auto-baud time-out interrupt */ 00096 #define UART_IER_BITMASK ((uint32_t)(0x307)) /*!< UART interrupt enable register bit mask */ 00097 #define UART1_IER_BITMASK ((uint32_t)(0x38F)) /*!< UART1 interrupt enable register bit mask */ 00098 00099 /*********************************************************************//** 00100 * Macro defines for Macro defines for UART interrupt identification register 00101 **********************************************************************/ 00102 #define UART_IIR_INTSTAT_PEND ((uint32_t)(1<<0)) /*!<Interrupt Status - Active low */ 00103 #define UART_IIR_INTID_RLS ((uint32_t)(3<<1)) /*!<Interrupt identification: Receive line status*/ 00104 #define UART_IIR_INTID_RDA ((uint32_t)(2<<1)) /*!<Interrupt identification: Receive data available*/ 00105 #define UART_IIR_INTID_CTI ((uint32_t)(6<<1)) /*!<Interrupt identification: Character time-out indicator*/ 00106 #define UART_IIR_INTID_THRE ((uint32_t)(1<<1)) /*!<Interrupt identification: THRE interrupt*/ 00107 #define UART1_IIR_INTID_MODEM ((uint32_t)(0<<1)) /*!<Interrupt identification: Modem interrupt*/ 00108 #define UART_IIR_INTID_MASK ((uint32_t)(7<<1)) /*!<Interrupt identification: Interrupt ID mask */ 00109 #define UART_IIR_FIFO_EN ((uint32_t)(3<<6)) /*!<These bits are equivalent to UnFCR[0] */ 00110 #define UART_IIR_ABEO_INT ((uint32_t)(1<<8)) /*!< End of auto-baud interrupt */ 00111 #define UART_IIR_ABTO_INT ((uint32_t)(1<<9)) /*!< Auto-baud time-out interrupt */ 00112 #define UART_IIR_BITMASK ((uint32_t)(0x3CF)) /*!< UART interrupt identification register bit mask */ 00113 00114 /*********************************************************************//** 00115 * Macro defines for Macro defines for UART FIFO control register 00116 **********************************************************************/ 00117 #define UART_FCR_FIFO_EN ((uint8_t)(1<<0)) /*!< UART FIFO enable */ 00118 #define UART_FCR_RX_RS ((uint8_t)(1<<1)) /*!< UART FIFO RX reset */ 00119 #define UART_FCR_TX_RS ((uint8_t)(1<<2)) /*!< UART FIFO TX reset */ 00120 #define UART_FCR_DMAMODE_SEL ((uint8_t)(1<<3)) /*!< UART DMA mode selection */ 00121 #define UART_FCR_TRG_LEV0 ((uint8_t)(0)) /*!< UART FIFO trigger level 0: 1 character */ 00122 #define UART_FCR_TRG_LEV1 ((uint8_t)(1<<6)) /*!< UART FIFO trigger level 1: 4 character */ 00123 #define UART_FCR_TRG_LEV2 ((uint8_t)(2<<6)) /*!< UART FIFO trigger level 2: 8 character */ 00124 #define UART_FCR_TRG_LEV3 ((uint8_t)(3<<6)) /*!< UART FIFO trigger level 3: 14 character */ 00125 #define UART_FCR_BITMASK ((uint8_t)(0xCF)) /*!< UART FIFO control bit mask */ 00126 #define UART_TX_FIFO_SIZE (16) 00127 00128 /*********************************************************************//** 00129 * Macro defines for Macro defines for UART line control register 00130 **********************************************************************/ 00131 #define UART_LCR_WLEN5 ((uint8_t)(0)) /*!< UART 5 bit data mode */ 00132 #define UART_LCR_WLEN6 ((uint8_t)(1<<0)) /*!< UART 6 bit data mode */ 00133 #define UART_LCR_WLEN7 ((uint8_t)(2<<0)) /*!< UART 7 bit data mode */ 00134 #define UART_LCR_WLEN8 ((uint8_t)(3<<0)) /*!< UART 8 bit data mode */ 00135 #define UART_LCR_STOPBIT_SEL ((uint8_t)(1<<2)) /*!< UART Two Stop Bits Select */ 00136 #define UART_LCR_PARITY_EN ((uint8_t)(1<<3)) /*!< UART Parity Enable */ 00137 #define UART_LCR_PARITY_ODD ((uint8_t)(0)) /*!< UART Odd Parity Select */ 00138 #define UART_LCR_PARITY_EVEN ((uint8_t)(1<<4)) /*!< UART Even Parity Select */ 00139 #define UART_LCR_PARITY_F_1 ((uint8_t)(2<<4)) /*!< UART force 1 stick parity */ 00140 #define UART_LCR_PARITY_F_0 ((uint8_t)(3<<4)) /*!< UART force 0 stick parity */ 00141 #define UART_LCR_BREAK_EN ((uint8_t)(1<<6)) /*!< UART Transmission Break enable */ 00142 #define UART_LCR_DLAB_EN ((uint8_t)(1<<7)) /*!< UART Divisor Latches Access bit enable */ 00143 #define UART_LCR_BITMASK ((uint8_t)(0xFF)) /*!< UART line control bit mask */ 00144 00145 /*********************************************************************//** 00146 * Macro defines for Macro defines for UART1 Modem Control Register 00147 **********************************************************************/ 00148 #define UART1_MCR_DTR_CTRL ((uint8_t)(1<<0)) /*!< Source for modem output pin DTR */ 00149 #define UART1_MCR_RTS_CTRL ((uint8_t)(1<<1)) /*!< Source for modem output pin RTS */ 00150 #define UART1_MCR_LOOPB_EN ((uint8_t)(1<<4)) /*!< Loop back mode select */ 00151 #define UART1_MCR_AUTO_RTS_EN ((uint8_t)(1<<6)) /*!< Enable Auto RTS flow-control */ 00152 #define UART1_MCR_AUTO_CTS_EN ((uint8_t)(1<<7)) /*!< Enable Auto CTS flow-control */ 00153 #define UART1_MCR_BITMASK ((uint8_t)(0x0F3)) /*!< UART1 bit mask value */ 00154 00155 /*********************************************************************//** 00156 * Macro defines for Macro defines for UART line status register 00157 **********************************************************************/ 00158 #define UART_LSR_RDR ((uint8_t)(1<<0)) /*!<Line status register: Receive data ready*/ 00159 #define UART_LSR_OE ((uint8_t)(1<<1)) /*!<Line status register: Overrun error*/ 00160 #define UART_LSR_PE ((uint8_t)(1<<2)) /*!<Line status register: Parity error*/ 00161 #define UART_LSR_FE ((uint8_t)(1<<3)) /*!<Line status register: Framing error*/ 00162 #define UART_LSR_BI ((uint8_t)(1<<4)) /*!<Line status register: Break interrupt*/ 00163 #define UART_LSR_THRE ((uint8_t)(1<<5)) /*!<Line status register: Transmit holding register empty*/ 00164 #define UART_LSR_TEMT ((uint8_t)(1<<6)) /*!<Line status register: Transmitter empty*/ 00165 #define UART_LSR_RXFE ((uint8_t)(1<<7)) /*!<Error in RX FIFO*/ 00166 #define UART_LSR_BITMASK ((uint8_t)(0xFF)) /*!<UART Line status bit mask */ 00167 00168 /*********************************************************************//** 00169 * Macro defines for Macro defines for UART Modem (UART1 only) status register 00170 **********************************************************************/ 00171 #define UART1_MSR_DELTA_CTS ((uint8_t)(1<<0)) /*!< Set upon state change of input CTS */ 00172 #define UART1_MSR_DELTA_DSR ((uint8_t)(1<<1)) /*!< Set upon state change of input DSR */ 00173 #define UART1_MSR_LO2HI_RI ((uint8_t)(1<<2)) /*!< Set upon low to high transition of input RI */ 00174 #define UART1_MSR_DELTA_DCD ((uint8_t)(1<<3)) /*!< Set upon state change of input DCD */ 00175 #define UART1_MSR_CTS ((uint8_t)(1<<4)) /*!< Clear To Send State */ 00176 #define UART1_MSR_DSR ((uint8_t)(1<<5)) /*!< Data Set Ready State */ 00177 #define UART1_MSR_RI ((uint8_t)(1<<6)) /*!< Ring Indicator State */ 00178 #define UART1_MSR_DCD ((uint8_t)(1<<7)) /*!< Data Carrier Detect State */ 00179 #define UART1_MSR_BITMASK ((uint8_t)(0xFF)) /*!< MSR register bit-mask value */ 00180 00181 /*********************************************************************//** 00182 * Macro defines for Macro defines for UART Scratch Pad Register 00183 **********************************************************************/ 00184 #define UART_SCR_BIMASK ((uint8_t)(0xFF)) /*!< UART Scratch Pad bit mask */ 00185 00186 /*********************************************************************//** 00187 * Macro defines for Macro defines for UART Auto baudrate control register 00188 **********************************************************************/ 00189 #define UART_ACR_START ((uint32_t)(1<<0)) /**< UART Auto-baud start */ 00190 #define UART_ACR_MODE ((uint32_t)(1<<1)) /**< UART Auto baudrate Mode 1 */ 00191 #define UART_ACR_AUTO_RESTART ((uint32_t)(1<<2)) /**< UART Auto baudrate restart */ 00192 #define UART_ACR_ABEOINT_CLR ((uint32_t)(1<<8)) /**< UART End of auto-baud interrupt clear */ 00193 #define UART_ACR_ABTOINT_CLR ((uint32_t)(1<<9)) /**< UART Auto-baud time-out interrupt clear */ 00194 #define UART_ACR_BITMASK ((uint32_t)(0x307)) /**< UART Auto Baudrate register bit mask */ 00195 00196 /*********************************************************************//** 00197 * Macro defines for Macro defines for UART IrDA control register 00198 **********************************************************************/ 00199 #define UART_ICR_IRDAEN ((uint32_t)(1<<0)) /**< IrDA mode enable */ 00200 #define UART_ICR_IRDAINV ((uint32_t)(1<<1)) /**< IrDA serial input inverted */ 00201 #define UART_ICR_FIXPULSE_EN ((uint32_t)(1<<2)) /**< IrDA fixed pulse width mode */ 00202 #define UART_ICR_PULSEDIV(n) ((uint32_t)((n&0x07)<<3)) /**< PulseDiv - Configures the pulse when FixPulseEn = 1 */ 00203 #define UART_ICR_BITMASK ((uint32_t)(0x3F)) /*!< UART IRDA bit mask */ 00204 00205 /*********************************************************************//** 00206 * Macro defines for Macro defines for UART Fractional divider register 00207 **********************************************************************/ 00208 #define UART_FDR_DIVADDVAL(n) ((uint32_t)(n&0x0F)) /**< Baud-rate generation pre-scaler divisor */ 00209 #define UART_FDR_MULVAL(n) ((uint32_t)((n<<4)&0xF0)) /**< Baud-rate pre-scaler multiplier value */ 00210 #define UART_FDR_BITMASK ((uint32_t)(0xFF)) /**< UART Fractional Divider register bit mask */ 00211 00212 /*********************************************************************//** 00213 * Macro defines for Macro defines for UART Tx Enable register 00214 **********************************************************************/ 00215 #define UART_TER_TXEN ((uint8_t)(1<<7)) /*!< Transmit enable bit */ 00216 #define UART_TER_BITMASK ((uint8_t)(0x80)) /**< UART Transmit Enable Register bit mask */ 00217 00218 /*********************************************************************//** 00219 * Macro defines for Macro defines for UART1 RS485 Control register 00220 **********************************************************************/ 00221 #define UART1_RS485CTRL_NMM_EN ((uint32_t)(1<<0)) /*!< RS-485/EIA-485 Normal Multi-drop Mode (NMM) 00222 is disabled */ 00223 #define UART1_RS485CTRL_RX_DIS ((uint32_t)(1<<1)) /*!< The receiver is disabled */ 00224 #define UART1_RS485CTRL_AADEN ((uint32_t)(1<<2)) /*!< Auto Address Detect (AAD) is enabled */ 00225 #define UART1_RS485CTRL_SEL_DTR ((uint32_t)(1<<3)) /*!< If direction control is enabled 00226 (bit DCTRL = 1), pin DTR is used for direction control */ 00227 #define UART1_RS485CTRL_DCTRL_EN ((uint32_t)(1<<4)) /*!< Enable Auto Direction Control */ 00228 #define UART1_RS485CTRL_OINV_1 ((uint32_t)(1<<5)) /*!< This bit reverses the polarity of the direction 00229 control signal on the RTS (or DTR) pin. The direction control pin 00230 will be driven to logic "1" when the transmitter has data to be sent */ 00231 #define UART1_RS485CTRL_BITMASK ((uint32_t)(0x3F)) /**< RS485 control bit-mask value */ 00232 00233 /*********************************************************************//** 00234 * Macro defines for Macro defines for UART1 RS-485 Address Match register 00235 **********************************************************************/ 00236 #define UART1_RS485ADRMATCH_BITMASK ((uint8_t)(0xFF)) /**< Bit mask value */ 00237 00238 /*********************************************************************//** 00239 * Macro defines for Macro defines for UART1 RS-485 Delay value register 00240 **********************************************************************/ 00241 /* Macro defines for UART1 RS-485 Delay value register */ 00242 #define UART1_RS485DLY_BITMASK ((uint8_t)(0xFF)) /** Bit mask value */ 00243 00244 /*********************************************************************//** 00245 * Macro defines for Macro defines for UART FIFO Level register 00246 **********************************************************************/ 00247 #define UART_FIFOLVL_RXFIFOLVL(n) ((uint32_t)(n&0x0F)) /**< Reflects the current level of the UART receiver FIFO */ 00248 #define UART_FIFOLVL_TXFIFOLVL(n) ((uint32_t)((n>>8)&0x0F)) /**< Reflects the current level of the UART transmitter FIFO */ 00249 #define UART_FIFOLVL_BITMASK ((uint32_t)(0x0F0F)) /**< UART FIFO Level Register bit mask */ 00250 00251 00252 /* ---------------- CHECK PARAMETER DEFINITIONS ---------------------------- */ 00253 00254 /** Macro to check the input UART_DATABIT parameters */ 00255 #define PARAM_UART_DATABIT(databit) ((databit==UART_DATABIT_5) || (databit==UART_DATABIT_6)\ 00256 || (databit==UART_DATABIT_7) || (databit==UART_DATABIT_8)) 00257 00258 /** Macro to check the input UART_STOPBIT parameters */ 00259 #define PARAM_UART_STOPBIT(stopbit) ((stopbit==UART_STOPBIT_1) || (stopbit==UART_STOPBIT_2)) 00260 00261 /** Macro to check the input UART_PARITY parameters */ 00262 #define PARAM_UART_PARITY(parity) ((parity==UART_PARITY_NONE) || (parity==UART_PARITY_ODD) \ 00263 || (parity==UART_PARITY_EVEN) || (parity==UART_PARITY_SP_1) \ 00264 || (parity==UART_PARITY_SP_0)) 00265 00266 /** Macro to check the input UART_FIFO parameters */ 00267 #define PARAM_UART_FIFO_LEVEL(fifo) ((fifo==UART_FIFO_TRGLEV0) \ 00268 || (fifo==UART_FIFO_TRGLEV1) || (fifo==UART_FIFO_TRGLEV2) \ 00269 || (fifo==UART_FIFO_TRGLEV3)) 00270 00271 /** Macro to check the input UART_INTCFG parameters */ 00272 #define PARAM_UART_INTCFG(IntCfg) ((IntCfg==UART_INTCFG_RBR) || (IntCfg==UART_INTCFG_THRE) \ 00273 || (IntCfg==UART_INTCFG_RLS) || (IntCfg==UART_INTCFG_ABEO) \ 00274 || (IntCfg==UART_INTCFG_ABTO)) 00275 00276 /** Macro to check the input UART1_INTCFG parameters - expansion input parameter for UART1 */ 00277 #define PARAM_UART1_INTCFG(IntCfg) ((IntCfg==UART1_INTCFG_MS) || (IntCfg==UART1_INTCFG_CTS)) 00278 00279 /** Macro to check the input UART_AUTOBAUD_MODE parameters */ 00280 #define PARAM_UART_AUTOBAUD_MODE(ABmode) ((ABmode==UART_AUTOBAUD_MODE0) || (ABmode==UART_AUTOBAUD_MODE1)) 00281 00282 /** Macro to check the input UART_AUTOBAUD_INTSTAT parameters */ 00283 #define PARAM_UART_AUTOBAUD_INTSTAT(ABIntStat) ((ABIntStat==UART_AUTOBAUD_INTSTAT_ABEO) || \ 00284 (ABIntStat==UART_AUTOBAUD_INTSTAT_ABTO)) 00285 00286 /** Macro to check the input UART_IrDA_PULSEDIV parameters */ 00287 #define PARAM_UART_IrDA_PULSEDIV(PulseDiv) ((PulseDiv==UART_IrDA_PULSEDIV2) || (PulseDiv==UART_IrDA_PULSEDIV4) \ 00288 || (PulseDiv==UART_IrDA_PULSEDIV8) || (PulseDiv==UART_IrDA_PULSEDIV16) \ 00289 || (PulseDiv==UART_IrDA_PULSEDIV32) || (PulseDiv==UART_IrDA_PULSEDIV64) \ 00290 || (PulseDiv==UART_IrDA_PULSEDIV128) || (PulseDiv==UART_IrDA_PULSEDIV256)) 00291 00292 /* Macro to check the input UART1_SignalState parameters */ 00293 #define PARAM_UART1_SIGNALSTATE(x) ((x==INACTIVE) || (x==ACTIVE)) 00294 00295 /** Macro to check the input PARAM_UART1_MODEM_PIN parameters */ 00296 #define PARAM_UART1_MODEM_PIN(x) ((x==UART1_MODEM_PIN_DTR) || (x==UART1_MODEM_PIN_RTS)) 00297 00298 /** Macro to check the input PARAM_UART1_MODEM_MODE parameters */ 00299 #define PARAM_UART1_MODEM_MODE(x) ((x==UART1_MODEM_MODE_LOOPBACK) || (x==UART1_MODEM_MODE_AUTO_RTS) \ 00300 || (x==UART1_MODEM_MODE_AUTO_CTS)) 00301 00302 /** Macro to check the direction control pin type */ 00303 #define PARAM_UART_RS485_DIRCTRL_PIN(x) ((x==UART1_RS485_DIRCTRL_RTS) || (x==UART1_RS485_DIRCTRL_DTR)) 00304 00305 /* Macro to determine if it is valid UART port number */ 00306 #define PARAM_UARTx(x) ((((uint32_t *)x)==((uint32_t *)LPC_UART0)) \ 00307 || (((uint32_t *)x)==((uint32_t *)LPC_UART1)) \ 00308 || (((uint32_t *)x)==((uint32_t *)LPC_UART2)) \ 00309 || (((uint32_t *)x)==((uint32_t *)LPC_UART3))) 00310 #define PARAM_UART_IrDA(x) (((uint32_t *)x)==((uint32_t *)LPC_UART3)) 00311 #define PARAM_UART1_MODEM(x) (((uint32_t *)x)==((uint32_t *)LPC_UART1)) 00312 00313 /** Macro to check the input value for UART1_RS485_CFG_MATCHADDRVALUE parameter */ 00314 #define PARAM_UART1_RS485_CFG_MATCHADDRVALUE(x) ((x<0xFF)) 00315 00316 /** Macro to check the input value for UART1_RS485_CFG_DELAYVALUE parameter */ 00317 #define PARAM_UART1_RS485_CFG_DELAYVALUE(x) ((x<0xFF)) 00318 00319 /** 00320 * @} 00321 */ 00322 00323 00324 /* Public Types --------------------------------------------------------------- */ 00325 /** @defgroup UART_Public_Types UART Public Types 00326 * @{ 00327 */ 00328 00329 /** 00330 * @brief UART Databit type definitions 00331 */ 00332 typedef enum { 00333 UART_DATABIT_5 = 0, /*!< UART 5 bit data mode */ 00334 UART_DATABIT_6 , /*!< UART 6 bit data mode */ 00335 UART_DATABIT_7 , /*!< UART 7 bit data mode */ 00336 UART_DATABIT_8 /*!< UART 8 bit data mode */ 00337 } UART_DATABIT_Type; 00338 00339 /** 00340 * @brief UART Stop bit type definitions 00341 */ 00342 typedef enum { 00343 UART_STOPBIT_1 = (0), /*!< UART 1 Stop Bits Select */ 00344 UART_STOPBIT_2 , /*!< UART Two Stop Bits Select */ 00345 } UART_STOPBIT_Type; 00346 00347 /** 00348 * @brief UART Parity type definitions 00349 */ 00350 typedef enum { 00351 UART_PARITY_NONE = 0, /*!< No parity */ 00352 UART_PARITY_ODD , /*!< Odd parity */ 00353 UART_PARITY_EVEN , /*!< Even parity */ 00354 UART_PARITY_SP_1 , /*!< Forced "1" stick parity */ 00355 UART_PARITY_SP_0 /*!< Forced "0" stick parity */ 00356 } UART_PARITY_Type; 00357 00358 /** 00359 * @brief FIFO Level type definitions 00360 */ 00361 typedef enum { 00362 UART_FIFO_TRGLEV0 = 0, /*!< UART FIFO trigger level 0: 1 character */ 00363 UART_FIFO_TRGLEV1 , /*!< UART FIFO trigger level 1: 4 character */ 00364 UART_FIFO_TRGLEV2 , /*!< UART FIFO trigger level 2: 8 character */ 00365 UART_FIFO_TRGLEV3 /*!< UART FIFO trigger level 3: 14 character */ 00366 } UART_FITO_LEVEL_Type; 00367 00368 /********************************************************************//** 00369 * @brief UART Interrupt Type definitions 00370 **********************************************************************/ 00371 typedef enum { 00372 UART_INTCFG_RBR = 0, /*!< RBR Interrupt enable*/ 00373 UART_INTCFG_THRE , /*!< THR Interrupt enable*/ 00374 UART_INTCFG_RLS , /*!< RX line status interrupt enable*/ 00375 UART1_INTCFG_MS , /*!< Modem status interrupt enable (UART1 only) */ 00376 UART1_INTCFG_CTS , /*!< CTS1 signal transition interrupt enable (UART1 only) */ 00377 UART_INTCFG_ABEO , /*!< Enables the end of auto-baud interrupt */ 00378 UART_INTCFG_ABTO /*!< Enables the auto-baud time-out interrupt */ 00379 } UART_INT_Type; 00380 00381 /** 00382 * @brief UART Line Status Type definition 00383 */ 00384 typedef enum { 00385 UART_LINESTAT_RDR = UART_LSR_RDR, /*!<Line status register: Receive data ready*/ 00386 UART_LINESTAT_OE = UART_LSR_OE, /*!<Line status register: Overrun error*/ 00387 UART_LINESTAT_PE = UART_LSR_PE, /*!<Line status register: Parity error*/ 00388 UART_LINESTAT_FE = UART_LSR_FE, /*!<Line status register: Framing error*/ 00389 UART_LINESTAT_BI = UART_LSR_BI, /*!<Line status register: Break interrupt*/ 00390 UART_LINESTAT_THRE = UART_LSR_THRE, /*!<Line status register: Transmit holding register empty*/ 00391 UART_LINESTAT_TEMT = UART_LSR_TEMT, /*!<Line status register: Transmitter empty*/ 00392 UART_LINESTAT_RXFE = UART_LSR_RXFE /*!<Error in RX FIFO*/ 00393 } UART_LS_Type; 00394 00395 /** 00396 * @brief UART Auto-baudrate mode type definition 00397 */ 00398 typedef enum { 00399 UART_AUTOBAUD_MODE0 = 0, /**< UART Auto baudrate Mode 0 */ 00400 UART_AUTOBAUD_MODE1, /**< UART Auto baudrate Mode 1 */ 00401 } UART_AB_MODE_Type; 00402 00403 /** 00404 * @brief Auto Baudrate mode configuration type definition 00405 */ 00406 typedef struct { 00407 UART_AB_MODE_Type ABMode; /**< Autobaudrate mode */ 00408 FunctionalState AutoRestart; /**< Auto Restart state */ 00409 } UART_AB_CFG_Type; 00410 00411 /** 00412 * @brief UART End of Auto-baudrate type definition 00413 */ 00414 typedef enum { 00415 UART_AUTOBAUD_INTSTAT_ABEO = UART_IIR_ABEO_INT, /**< UART End of auto-baud interrupt */ 00416 UART_AUTOBAUD_INTSTAT_ABTO = UART_IIR_ABTO_INT /**< UART Auto-baud time-out interrupt */ 00417 }UART_ABEO_Type; 00418 00419 /** 00420 * UART IrDA Control type Definition 00421 */ 00422 typedef enum { 00423 UART_IrDA_PULSEDIV2 = 0, /**< Pulse width = 2 * Tpclk 00424 - Configures the pulse when FixPulseEn = 1 */ 00425 UART_IrDA_PULSEDIV4, /**< Pulse width = 4 * Tpclk 00426 - Configures the pulse when FixPulseEn = 1 */ 00427 UART_IrDA_PULSEDIV8, /**< Pulse width = 8 * Tpclk 00428 - Configures the pulse when FixPulseEn = 1 */ 00429 UART_IrDA_PULSEDIV16, /**< Pulse width = 16 * Tpclk 00430 - Configures the pulse when FixPulseEn = 1 */ 00431 UART_IrDA_PULSEDIV32, /**< Pulse width = 32 * Tpclk 00432 - Configures the pulse when FixPulseEn = 1 */ 00433 UART_IrDA_PULSEDIV64, /**< Pulse width = 64 * Tpclk 00434 - Configures the pulse when FixPulseEn = 1 */ 00435 UART_IrDA_PULSEDIV128, /**< Pulse width = 128 * Tpclk 00436 - Configures the pulse when FixPulseEn = 1 */ 00437 UART_IrDA_PULSEDIV256 /**< Pulse width = 256 * Tpclk 00438 - Configures the pulse when FixPulseEn = 1 */ 00439 } UART_IrDA_PULSE_Type; 00440 00441 /********************************************************************//** 00442 * @brief UART1 Full modem - Signal states definition 00443 **********************************************************************/ 00444 typedef enum { 00445 INACTIVE = 0, /* In-active state */ 00446 ACTIVE = !INACTIVE /* Active state */ 00447 }UART1_SignalState; 00448 00449 /** 00450 * @brief UART modem status type definition 00451 */ 00452 typedef enum { 00453 UART1_MODEM_STAT_DELTA_CTS = UART1_MSR_DELTA_CTS, /*!< Set upon state change of input CTS */ 00454 UART1_MODEM_STAT_DELTA_DSR = UART1_MSR_DELTA_DSR, /*!< Set upon state change of input DSR */ 00455 UART1_MODEM_STAT_LO2HI_RI = UART1_MSR_LO2HI_RI, /*!< Set upon low to high transition of input RI */ 00456 UART1_MODEM_STAT_DELTA_DCD = UART1_MSR_DELTA_DCD, /*!< Set upon state change of input DCD */ 00457 UART1_MODEM_STAT_CTS = UART1_MSR_CTS, /*!< Clear To Send State */ 00458 UART1_MODEM_STAT_DSR = UART1_MSR_DSR, /*!< Data Set Ready State */ 00459 UART1_MODEM_STAT_RI = UART1_MSR_RI, /*!< Ring Indicator State */ 00460 UART1_MODEM_STAT_DCD = UART1_MSR_DCD /*!< Data Carrier Detect State */ 00461 } UART_MODEM_STAT_type; 00462 00463 /** 00464 * @brief Modem output pin type definition 00465 */ 00466 typedef enum { 00467 UART1_MODEM_PIN_DTR = 0, /*!< Source for modem output pin DTR */ 00468 UART1_MODEM_PIN_RTS /*!< Source for modem output pin RTS */ 00469 } UART_MODEM_PIN_Type; 00470 00471 /** 00472 * @brief UART Modem mode type definition 00473 */ 00474 typedef enum { 00475 UART1_MODEM_MODE_LOOPBACK = 0, /*!< Loop back mode select */ 00476 UART1_MODEM_MODE_AUTO_RTS , /*!< Enable Auto RTS flow-control */ 00477 UART1_MODEM_MODE_AUTO_CTS /*!< Enable Auto CTS flow-control */ 00478 } UART_MODEM_MODE_Type; 00479 00480 /** 00481 * @brief UART Direction Control Pin type definition 00482 */ 00483 typedef enum { 00484 UART1_RS485_DIRCTRL_RTS = 0, /**< Pin RTS is used for direction control */ 00485 UART1_RS485_DIRCTRL_DTR /**< Pin DTR is used for direction control */ 00486 } UART_RS485_DIRCTRL_PIN_Type; 00487 00488 /********************************************************************//** 00489 * @brief UART Configuration Structure definition 00490 **********************************************************************/ 00491 typedef struct { 00492 uint32_t Baud_rate; /**< UART baud rate */ 00493 UART_PARITY_Type Parity; /**< Parity selection, should be: 00494 - UART_PARITY_NONE: No parity 00495 - UART_PARITY_ODD: Odd parity 00496 - UART_PARITY_EVEN: Even parity 00497 - UART_PARITY_SP_1: Forced "1" stick parity 00498 - UART_PARITY_SP_0: Forced "0" stick parity 00499 */ 00500 UART_DATABIT_Type Databits; /**< Number of data bits, should be: 00501 - UART_DATABIT_5: UART 5 bit data mode 00502 - UART_DATABIT_6: UART 6 bit data mode 00503 - UART_DATABIT_7: UART 7 bit data mode 00504 - UART_DATABIT_8: UART 8 bit data mode 00505 */ 00506 UART_STOPBIT_Type Stopbits; /**< Number of stop bits, should be: 00507 - UART_STOPBIT_1: UART 1 Stop Bits Select 00508 - UART_STOPBIT_2: UART 2 Stop Bits Select 00509 */ 00510 } UART_CFG_Type; 00511 00512 /********************************************************************//** 00513 * @brief UART FIFO Configuration Structure definition 00514 **********************************************************************/ 00515 00516 typedef struct { 00517 FunctionalState FIFO_ResetRxBuf; /**< Reset Rx FIFO command state , should be: 00518 - ENABLE: Reset Rx FIFO in UART 00519 - DISABLE: Do not reset Rx FIFO in UART 00520 */ 00521 FunctionalState FIFO_ResetTxBuf; /**< Reset Tx FIFO command state , should be: 00522 - ENABLE: Reset Tx FIFO in UART 00523 - DISABLE: Do not reset Tx FIFO in UART 00524 */ 00525 FunctionalState FIFO_DMAMode; /**< DMA mode, should be: 00526 - ENABLE: Enable DMA mode in UART 00527 - DISABLE: Disable DMA mode in UART 00528 */ 00529 UART_FITO_LEVEL_Type FIFO_Level; /**< Rx FIFO trigger level, should be: 00530 - UART_FIFO_TRGLEV0: UART FIFO trigger level 0: 1 character 00531 - UART_FIFO_TRGLEV1: UART FIFO trigger level 1: 4 character 00532 - UART_FIFO_TRGLEV2: UART FIFO trigger level 2: 8 character 00533 - UART_FIFO_TRGLEV3: UART FIFO trigger level 3: 14 character 00534 */ 00535 } UART_FIFO_CFG_Type; 00536 00537 /********************************************************************//** 00538 * @brief UART1 Full modem - RS485 Control configuration type 00539 **********************************************************************/ 00540 typedef struct { 00541 FunctionalState NormalMultiDropMode_State; /*!< Normal MultiDrop mode State: 00542 - ENABLE: Enable this function. 00543 - DISABLE: Disable this function. */ 00544 FunctionalState Rx_State; /*!< Receiver State: 00545 - ENABLE: Enable Receiver. 00546 - DISABLE: Disable Receiver. */ 00547 FunctionalState AutoAddrDetect_State; /*!< Auto Address Detect mode state: 00548 - ENABLE: ENABLE this function. 00549 - DISABLE: Disable this function. */ 00550 FunctionalState AutoDirCtrl_State; /*!< Auto Direction Control State: 00551 - ENABLE: Enable this function. 00552 - DISABLE: Disable this function. */ 00553 UART_RS485_DIRCTRL_PIN_Type DirCtrlPin; /*!< If direction control is enabled, state: 00554 - UART1_RS485_DIRCTRL_RTS: 00555 pin RTS is used for direction control. 00556 - UART1_RS485_DIRCTRL_DTR: 00557 pin DTR is used for direction control. */ 00558 SetState DirCtrlPol_Level; /*!< Polarity of the direction control signal on 00559 the RTS (or DTR) pin: 00560 - RESET: The direction control pin will be driven 00561 to logic "0" when the transmitter has data to be sent. 00562 - SET: The direction control pin will be driven 00563 to logic "1" when the transmitter has data to be sent. */ 00564 uint8_t MatchAddrValue ; /*!< address match value for RS-485/EIA-485 mode, 8-bit long */ 00565 uint8_t DelayValue ; /*!< delay time is in periods of the baud clock, 8-bit long */ 00566 } UART1_RS485_CTRLCFG_Type; 00567 00568 /** 00569 * @} 00570 */ 00571 00572 00573 /* Public Functions ----------------------------------------------------------- */ 00574 /** @defgroup UART_Public_Functions UART Public Functions 00575 * @{ 00576 */ 00577 /* UART Init/DeInit functions --------------------------------------------------*/ 00578 void UART_Init(LPC_UART_TypeDef *UARTx, UART_CFG_Type *UART_ConfigStruct); 00579 void UART_DeInit(LPC_UART_TypeDef* UARTx); 00580 void UART_ConfigStructInit(UART_CFG_Type *UART_InitStruct); 00581 00582 /* UART Send/Receive functions -------------------------------------------------*/ 00583 void UART_SendByte(LPC_UART_TypeDef* UARTx, uint8_t Data); 00584 uint8_t UART_ReceiveByte(LPC_UART_TypeDef* UARTx); 00585 uint32_t UART_Send(LPC_UART_TypeDef *UARTx, uint8_t *txbuf, 00586 uint32_t buflen, TRANSFER_BLOCK_Type flag); 00587 uint32_t UART_Receive(LPC_UART_TypeDef *UARTx, uint8_t *rxbuf, \ 00588 uint32_t buflen, TRANSFER_BLOCK_Type flag); 00589 00590 /* UART FIFO functions ----------------------------------------------------------*/ 00591 void UART_FIFOConfig(LPC_UART_TypeDef *UARTx, UART_FIFO_CFG_Type *FIFOCfg); 00592 void UART_FIFOConfigStructInit(UART_FIFO_CFG_Type *UART_FIFOInitStruct); 00593 00594 /* UART get information functions -----------------------------------------------*/ 00595 uint32_t UART_GetIntId(LPC_UART_TypeDef* UARTx); 00596 uint8_t UART_GetLineStatus(LPC_UART_TypeDef* UARTx); 00597 00598 /* UART operate functions -------------------------------------------------------*/ 00599 void UART_IntConfig(LPC_UART_TypeDef *UARTx, UART_INT_Type UARTIntCfg, \ 00600 FunctionalState NewState); 00601 void UART_TxCmd(LPC_UART_TypeDef *UARTx, FunctionalState NewState); 00602 FlagStatus UART_CheckBusy(LPC_UART_TypeDef *UARTx); 00603 void UART_ForceBreak(LPC_UART_TypeDef* UARTx); 00604 00605 /* UART Auto-baud functions -----------------------------------------------------*/ 00606 void UART_ABClearIntPending(LPC_UART_TypeDef *UARTx, UART_ABEO_Type ABIntType); 00607 void UART_ABCmd(LPC_UART_TypeDef *UARTx, UART_AB_CFG_Type *ABConfigStruct, \ 00608 FunctionalState NewState); 00609 00610 /* UART1 FullModem functions ----------------------------------------------------*/ 00611 void UART_FullModemForcePinState(LPC_UART1_TypeDef *UARTx, UART_MODEM_PIN_Type Pin, \ 00612 UART1_SignalState NewState); 00613 void UART_FullModemConfigMode(LPC_UART1_TypeDef *UARTx, UART_MODEM_MODE_Type Mode, \ 00614 FunctionalState NewState); 00615 uint8_t UART_FullModemGetStatus(LPC_UART1_TypeDef *UARTx); 00616 00617 /* UART RS485 functions ----------------------------------------------------------*/ 00618 void UART_RS485Config(LPC_UART1_TypeDef *UARTx, \ 00619 UART1_RS485_CTRLCFG_Type *RS485ConfigStruct); 00620 void UART_RS485ReceiverCmd(LPC_UART1_TypeDef *UARTx, FunctionalState NewState); 00621 void UART_RS485SendSlvAddr(LPC_UART1_TypeDef *UARTx, uint8_t SlvAddr); 00622 uint32_t UART_RS485SendData(LPC_UART1_TypeDef *UARTx, uint8_t *pData, uint32_t size); 00623 00624 /* UART IrDA functions-------------------------------------------------------------*/ 00625 void UART_IrDAInvtInputCmd(LPC_UART_TypeDef* UARTx, FunctionalState NewState); 00626 void UART_IrDACmd(LPC_UART_TypeDef* UARTx, FunctionalState NewState); 00627 void UART_IrDAPulseDivConfig(LPC_UART_TypeDef *UARTx, UART_IrDA_PULSE_Type PulseDiv); 00628 /** 00629 * @} 00630 */ 00631 00632 00633 #ifdef __cplusplus 00634 } 00635 #endif 00636 00637 00638 #endif /* __LPC17XX_UART_H */ 00639 00640 /** 00641 * @} 00642 */ 00643 00644 /* --------------------------------- End Of File ------------------------------ */
Generated on Tue Jul 12 2022 10:57:55 by
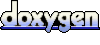