
Example of UART-DMA transfers taken form the npx cmsis driver libary
Embed:
(wiki syntax)
Show/hide line numbers
lpc17xx_pinsel.h
Go to the documentation of this file.
00001 /***********************************************************************//** 00002 * @file lpc17xx_pinsel.h 00003 * @brief Contains all macro definitions and function prototypes 00004 * support for Pin connect block firmware library on LPC17xx 00005 * @version 2.0 00006 * @date 21. May. 2010 00007 * @author NXP MCU SW Application Team 00008 ************************************************************************** 00009 * Software that is described herein is for illustrative purposes only 00010 * which provides customers with programming information regarding the 00011 * products. This software is supplied "AS IS" without any warranties. 00012 * NXP Semiconductors assumes no responsibility or liability for the 00013 * use of the software, conveys no license or title under any patent, 00014 * copyright, or mask work right to the product. NXP Semiconductors 00015 * reserves the right to make changes in the software without 00016 * notification. NXP Semiconductors also make no representation or 00017 * warranty that such application will be suitable for the specified 00018 * use without further testing or modification. 00019 **************************************************************************/ 00020 00021 /* Peripheral group ----------------------------------------------------------- */ 00022 /** @defgroup PINSEL PINSEL 00023 * @ingroup LPC1700CMSIS_FwLib_Drivers 00024 * @{ 00025 */ 00026 00027 #ifndef LPC17XX_PINSEL_H_ 00028 #define LPC17XX_PINSEL_H_ 00029 00030 /* Includes ------------------------------------------------------------------- */ 00031 #include "LPC17xx.h" 00032 #include "lpc_types.h" 00033 00034 #ifdef __cplusplus 00035 extern "C" 00036 { 00037 #endif 00038 00039 /* Public Macros -------------------------------------------------------------- */ 00040 /** @defgroup PINSEL_Public_Macros PINSEL Public Macros 00041 * @{ 00042 */ 00043 00044 /*********************************************************************//** 00045 *!< Macros define for PORT Selection 00046 ***********************************************************************/ 00047 #define PINSEL_PORT_0 ((0)) /**< PORT 0*/ 00048 #define PINSEL_PORT_1 ((1)) /**< PORT 1*/ 00049 #define PINSEL_PORT_2 ((2)) /**< PORT 2*/ 00050 #define PINSEL_PORT_3 ((3)) /**< PORT 3*/ 00051 #define PINSEL_PORT_4 ((4)) /**< PORT 4*/ 00052 00053 /*********************************************************************** 00054 * Macros define for Pin Function selection 00055 **********************************************************************/ 00056 #define PINSEL_FUNC_0 ((0)) /**< default function*/ 00057 #define PINSEL_FUNC_1 ((1)) /**< first alternate function*/ 00058 #define PINSEL_FUNC_2 ((2)) /**< second alternate function*/ 00059 #define PINSEL_FUNC_3 ((3)) /**< third or reserved alternate function*/ 00060 00061 /*********************************************************************** 00062 * Macros define for Pin Number of Port 00063 **********************************************************************/ 00064 #define PINSEL_PIN_0 ((0)) /**< Pin 0 */ 00065 #define PINSEL_PIN_1 ((1)) /**< Pin 1 */ 00066 #define PINSEL_PIN_2 ((2)) /**< Pin 2 */ 00067 #define PINSEL_PIN_3 ((3)) /**< Pin 3 */ 00068 #define PINSEL_PIN_4 ((4)) /**< Pin 4 */ 00069 #define PINSEL_PIN_5 ((5)) /**< Pin 5 */ 00070 #define PINSEL_PIN_6 ((6)) /**< Pin 6 */ 00071 #define PINSEL_PIN_7 ((7)) /**< Pin 7 */ 00072 #define PINSEL_PIN_8 ((8)) /**< Pin 8 */ 00073 #define PINSEL_PIN_9 ((9)) /**< Pin 9 */ 00074 #define PINSEL_PIN_10 ((10)) /**< Pin 10 */ 00075 #define PINSEL_PIN_11 ((11)) /**< Pin 11 */ 00076 #define PINSEL_PIN_12 ((12)) /**< Pin 12 */ 00077 #define PINSEL_PIN_13 ((13)) /**< Pin 13 */ 00078 #define PINSEL_PIN_14 ((14)) /**< Pin 14 */ 00079 #define PINSEL_PIN_15 ((15)) /**< Pin 15 */ 00080 #define PINSEL_PIN_16 ((16)) /**< Pin 16 */ 00081 #define PINSEL_PIN_17 ((17)) /**< Pin 17 */ 00082 #define PINSEL_PIN_18 ((18)) /**< Pin 18 */ 00083 #define PINSEL_PIN_19 ((19)) /**< Pin 19 */ 00084 #define PINSEL_PIN_20 ((20)) /**< Pin 20 */ 00085 #define PINSEL_PIN_21 ((21)) /**< Pin 21 */ 00086 #define PINSEL_PIN_22 ((22)) /**< Pin 22 */ 00087 #define PINSEL_PIN_23 ((23)) /**< Pin 23 */ 00088 #define PINSEL_PIN_24 ((24)) /**< Pin 24 */ 00089 #define PINSEL_PIN_25 ((25)) /**< Pin 25 */ 00090 #define PINSEL_PIN_26 ((26)) /**< Pin 26 */ 00091 #define PINSEL_PIN_27 ((27)) /**< Pin 27 */ 00092 #define PINSEL_PIN_28 ((28)) /**< Pin 28 */ 00093 #define PINSEL_PIN_29 ((29)) /**< Pin 29 */ 00094 #define PINSEL_PIN_30 ((30)) /**< Pin 30 */ 00095 #define PINSEL_PIN_31 ((31)) /**< Pin 31 */ 00096 00097 /*********************************************************************** 00098 * Macros define for Pin mode 00099 **********************************************************************/ 00100 #define PINSEL_PINMODE_PULLUP ((0)) /**< Internal pull-up resistor*/ 00101 #define PINSEL_PINMODE_TRISTATE ((2)) /**< Tri-state */ 00102 #define PINSEL_PINMODE_PULLDOWN ((3)) /**< Internal pull-down resistor */ 00103 00104 /*********************************************************************** 00105 * Macros define for Pin mode (normal/open drain) 00106 **********************************************************************/ 00107 #define PINSEL_PINMODE_NORMAL ((0)) /**< Pin is in the normal (not open drain) mode.*/ 00108 #define PINSEL_PINMODE_OPENDRAIN ((1)) /**< Pin is in the open drain mode */ 00109 00110 /*********************************************************************** 00111 * Macros define for I2C mode 00112 ***********************************************************************/ 00113 #define PINSEL_I2C_Normal_Mode ((0)) /**< The standard drive mode */ 00114 #define PINSEL_I2C_Fast_Mode ((1)) /**< Fast Mode Plus drive mode */ 00115 00116 /** 00117 * @} 00118 */ 00119 00120 /* Private Macros ------------------------------------------------------------- */ 00121 /** @defgroup PINSEL_Private_Macros PINSEL Private Macros 00122 * @{ 00123 */ 00124 00125 /* Pin selection define */ 00126 /* I2C Pin Configuration register bit description */ 00127 #define PINSEL_I2CPADCFG_SDADRV0 _BIT(0) /**< Drive mode control for the SDA0 pin, P0.27 */ 00128 #define PINSEL_I2CPADCFG_SDAI2C0 _BIT(1) /**< I2C mode control for the SDA0 pin, P0.27 */ 00129 #define PINSEL_I2CPADCFG_SCLDRV0 _BIT(2) /**< Drive mode control for the SCL0 pin, P0.28 */ 00130 #define PINSEL_I2CPADCFG_SCLI2C0 _BIT(3) /**< I2C mode control for the SCL0 pin, P0.28 */ 00131 00132 /** 00133 * @} 00134 */ 00135 00136 00137 /* Public Types --------------------------------------------------------------- */ 00138 /** @defgroup PINSEL_Public_Types PINSEL Public Types 00139 * @{ 00140 */ 00141 00142 /** @brief Pin configuration structure */ 00143 typedef struct 00144 { 00145 uint8_t Portnum; /**< Port Number, should be PINSEL_PORT_x, 00146 where x should be in range from 0 to 4 */ 00147 uint8_t Pinnum; /**< Pin Number, should be PINSEL_PIN_x, 00148 where x should be in range from 0 to 31 */ 00149 uint8_t Funcnum; /**< Function Number, should be PINSEL_FUNC_x, 00150 where x should be in range from 0 to 3 */ 00151 uint8_t Pinmode; /**< Pin Mode, should be: 00152 - PINSEL_PINMODE_PULLUP: Internal pull-up resistor 00153 - PINSEL_PINMODE_TRISTATE: Tri-state 00154 - PINSEL_PINMODE_PULLDOWN: Internal pull-down resistor */ 00155 uint8_t OpenDrain; /**< OpenDrain mode, should be: 00156 - PINSEL_PINMODE_NORMAL: Pin is in the normal (not open drain) mode 00157 - PINSEL_PINMODE_OPENDRAIN: Pin is in the open drain mode */ 00158 } PINSEL_CFG_Type; 00159 00160 /** 00161 * @} 00162 */ 00163 00164 00165 /* Public Functions ----------------------------------------------------------- */ 00166 /** @defgroup PINSEL_Public_Functions PINSEL Public Functions 00167 * @{ 00168 */ 00169 00170 void PINSEL_ConfigPin(PINSEL_CFG_Type *PinCfg); 00171 void PINSEL_ConfigTraceFunc (FunctionalState NewState); 00172 void PINSEL_SetI2C0Pins(uint8_t i2cPinMode, FunctionalState filterSlewRateEnable); 00173 00174 00175 /** 00176 * @} 00177 */ 00178 00179 00180 #ifdef __cplusplus 00181 } 00182 #endif 00183 00184 #endif /* LPC17XX_PINSEL_H_ */ 00185 00186 /** 00187 * @} 00188 */ 00189 00190 /* --------------------------------- End Of File ------------------------------ */ 00191
Generated on Tue Jul 12 2022 10:57:55 by
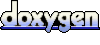