
Example of UART-DMA transfers taken form the npx cmsis driver libary
Embed:
(wiki syntax)
Show/hide line numbers
debug_frmwrk.h
Go to the documentation of this file.
00001 /***********************************************************************//** 00002 * @file debug_frmwrk.h 00003 * @brief Contains some utilities that used for debugging through UART 00004 * @version 2.0 00005 * @date 21. May. 2010 00006 * @author NXP MCU SW Application Team 00007 *---------------------------------------------------------------------------- 00008 * Software that is described herein is for illustrative purposes only 00009 * which provides customers with programming information regarding the 00010 * products. This software is supplied "AS IS" without any warranties. 00011 * NXP Semiconductors assumes no responsibility or liability for the 00012 * use of the software, conveys no license or title under any patent, 00013 * copyright, or mask work right to the product. NXP Semiconductors 00014 * reserves the right to make changes in the software without 00015 * notification. NXP Semiconductors also make no representation or 00016 * warranty that such application will be suitable for the specified 00017 * use without further testing or modification. 00018 **********************************************************************/ 00019 00020 #ifndef DEBUG_FRMWRK_H_ 00021 #define DEBUG_FRMWRK_H_ 00022 00023 //#include <stdarg.h> 00024 #include "lpc17xx_uart.h" 00025 00026 #define USED_UART_DEBUG_PORT 0 00027 00028 #if (USED_UART_DEBUG_PORT==0) 00029 #define DEBUG_UART_PORT LPC_UART0 00030 #elif (USED_UART_DEBUG_PORT==1) 00031 #define DEBUG_UART_PORT LPC_UART1 00032 #endif 00033 00034 #define _DBG(x) _db_msg(DEBUG_UART_PORT, x) 00035 #define _DBG_(x) _db_msg_(DEBUG_UART_PORT, x) 00036 #define _DBC(x) _db_char(DEBUG_UART_PORT, x) 00037 #define _DBD(x) _db_dec(DEBUG_UART_PORT, x) 00038 #define _DBD16(x) _db_dec_16(DEBUG_UART_PORT, x) 00039 #define _DBD32(x) _db_dec_32(DEBUG_UART_PORT, x) 00040 #define _DBH(x) _db_hex(DEBUG_UART_PORT, x) 00041 #define _DBH16(x) _db_hex_16(DEBUG_UART_PORT, x) 00042 #define _DBH32(x) _db_hex_32(DEBUG_UART_PORT, x) 00043 #define _DG _db_get_char(DEBUG_UART_PORT) 00044 //void _printf (const char *format, ...); 00045 00046 extern void (*_db_msg)(LPC_UART_TypeDef *UARTx, const void *s); 00047 extern void (*_db_msg_)(LPC_UART_TypeDef *UARTx, const void *s); 00048 extern void (*_db_char)(LPC_UART_TypeDef *UARTx, uint8_t ch); 00049 extern void (*_db_dec)(LPC_UART_TypeDef *UARTx, uint8_t decn); 00050 extern void (*_db_dec_16)(LPC_UART_TypeDef *UARTx, uint16_t decn); 00051 extern void (*_db_dec_32)(LPC_UART_TypeDef *UARTx, uint32_t decn); 00052 extern void (*_db_hex)(LPC_UART_TypeDef *UARTx, uint8_t hexn); 00053 extern void (*_db_hex_16)(LPC_UART_TypeDef *UARTx, uint16_t hexn); 00054 extern void (*_db_hex_32)(LPC_UART_TypeDef *UARTx, uint32_t hexn); 00055 extern uint8_t (*_db_get_char)(LPC_UART_TypeDef *UARTx); 00056 00057 void UARTPutChar (LPC_UART_TypeDef *UARTx, uint8_t ch); 00058 void UARTPuts(LPC_UART_TypeDef *UARTx, const void *str); 00059 void UARTPuts_(LPC_UART_TypeDef *UARTx, const void *str); 00060 void UARTPutDec(LPC_UART_TypeDef *UARTx, uint8_t decnum); 00061 void UARTPutDec16(LPC_UART_TypeDef *UARTx, uint16_t decnum); 00062 void UARTPutDec32(LPC_UART_TypeDef *UARTx, uint32_t decnum); 00063 void UARTPutHex (LPC_UART_TypeDef *UARTx, uint8_t hexnum); 00064 void UARTPutHex16 (LPC_UART_TypeDef *UARTx, uint16_t hexnum); 00065 void UARTPutHex32 (LPC_UART_TypeDef *UARTx, uint32_t hexnum); 00066 uint8_t UARTGetChar (LPC_UART_TypeDef *UARTx); 00067 void debug_frmwrk_init(void); 00068 00069 #endif /* DEBUG_FRMWRK_H_ */
Generated on Tue Jul 12 2022 10:57:55 by
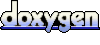