
Example of UART-DMA transfers taken form the npx cmsis driver libary
Embed:
(wiki syntax)
Show/hide line numbers
debug_frmwrk.c
Go to the documentation of this file.
00001 /***********************************************************************//** 00002 * @file debug_frmwrk.c 00003 * @brief Contains some utilities that used for debugging through UART 00004 * @version 2.0 00005 * @date 21. May. 2010 00006 * @author NXP MCU SW Application Team 00007 *---------------------------------------------------------------------------- 00008 * Software that is described herein is for illustrative purposes only 00009 * which provides customers with programming information regarding the 00010 * products. This software is supplied "AS IS" without any warranties. 00011 * NXP Semiconductors assumes no responsibility or liability for the 00012 * use of the software, conveys no license or title under any patent, 00013 * copyright, or mask work right to the product. NXP Semiconductors 00014 * reserves the right to make changes in the software without 00015 * notification. NXP Semiconductors also make no representation or 00016 * warranty that such application will be suitable for the specified 00017 * use without further testing or modification. 00018 **********************************************************************/ 00019 00020 #include "debug_frmwrk.h" 00021 #include "lpc17xx_pinsel.h" 00022 00023 /* If this source file built with example, the LPC17xx FW library configuration 00024 * file in each example directory ("lpc17xx_libcfg.h") must be included, 00025 * otherwise the default FW library configuration file must be included instead 00026 */ 00027 #ifdef __BUILD_WITH_EXAMPLE__ 00028 #include "lpc17xx_libcfg.h" 00029 #else 00030 #include "lpc17xx_libcfg_default.h" 00031 #endif /* __BUILD_WITH_EXAMPLE__ */ 00032 00033 #ifdef _DBGFWK 00034 /* Debug framework */ 00035 00036 void (*_db_msg)(LPC_UART_TypeDef *UARTx, const void *s); 00037 void (*_db_msg_)(LPC_UART_TypeDef *UARTx, const void *s); 00038 void (*_db_char)(LPC_UART_TypeDef *UARTx, uint8_t ch); 00039 void (*_db_dec)(LPC_UART_TypeDef *UARTx, uint8_t decn); 00040 void (*_db_dec_16)(LPC_UART_TypeDef *UARTx, uint16_t decn); 00041 void (*_db_dec_32)(LPC_UART_TypeDef *UARTx, uint32_t decn); 00042 void (*_db_hex)(LPC_UART_TypeDef *UARTx, uint8_t hexn); 00043 void (*_db_hex_16)(LPC_UART_TypeDef *UARTx, uint16_t hexn); 00044 void (*_db_hex_32)(LPC_UART_TypeDef *UARTx, uint32_t hexn); 00045 uint8_t (*_db_get_char)(LPC_UART_TypeDef *UARTx); 00046 00047 00048 /*********************************************************************//** 00049 * @brief Puts a character to UART port 00050 * @param[in] UARTx Pointer to UART peripheral 00051 * @param[in] ch Character to put 00052 * @return None 00053 **********************************************************************/ 00054 void UARTPutChar (LPC_UART_TypeDef *UARTx, uint8_t ch) 00055 { 00056 UART_Send(UARTx, &ch, 1, BLOCKING); 00057 } 00058 00059 00060 /*********************************************************************//** 00061 * @brief Get a character to UART port 00062 * @param[in] UARTx Pointer to UART peripheral 00063 * @return character value that returned 00064 **********************************************************************/ 00065 uint8_t UARTGetChar (LPC_UART_TypeDef *UARTx) 00066 { 00067 uint8_t tmp = 0; 00068 UART_Receive(UARTx, &tmp, 1, BLOCKING); 00069 return(tmp); 00070 } 00071 00072 00073 /*********************************************************************//** 00074 * @brief Puts a string to UART port 00075 * @param[in] UARTx Pointer to UART peripheral 00076 * @param[in] str string to put 00077 * @return None 00078 **********************************************************************/ 00079 void UARTPuts(LPC_UART_TypeDef *UARTx, const void *str) 00080 { 00081 uint8_t *s = (uint8_t *) str; 00082 00083 while (*s) 00084 { 00085 UARTPutChar(UARTx, *s++); 00086 } 00087 } 00088 00089 00090 /*********************************************************************//** 00091 * @brief Puts a string to UART port and print new line 00092 * @param[in] UARTx Pointer to UART peripheral 00093 * @param[in] str String to put 00094 * @return None 00095 **********************************************************************/ 00096 void UARTPuts_(LPC_UART_TypeDef *UARTx, const void *str) 00097 { 00098 UARTPuts (UARTx, str); 00099 UARTPuts (UARTx, "\n\r"); 00100 } 00101 00102 00103 /*********************************************************************//** 00104 * @brief Puts a decimal number to UART port 00105 * @param[in] UARTx Pointer to UART peripheral 00106 * @param[in] decnum Decimal number (8-bit long) 00107 * @return None 00108 **********************************************************************/ 00109 void UARTPutDec(LPC_UART_TypeDef *UARTx, uint8_t decnum) 00110 { 00111 uint8_t c1=decnum%10; 00112 uint8_t c2=(decnum/10)%10; 00113 uint8_t c3=(decnum/100)%10; 00114 UARTPutChar(UARTx, '0'+c3); 00115 UARTPutChar(UARTx, '0'+c2); 00116 UARTPutChar(UARTx, '0'+c1); 00117 } 00118 00119 /*********************************************************************//** 00120 * @brief Puts a decimal number to UART port 00121 * @param[in] UARTx Pointer to UART peripheral 00122 * @param[in] decnum Decimal number (8-bit long) 00123 * @return None 00124 **********************************************************************/ 00125 void UARTPutDec16(LPC_UART_TypeDef *UARTx, uint16_t decnum) 00126 { 00127 uint8_t c1=decnum%10; 00128 uint8_t c2=(decnum/10)%10; 00129 uint8_t c3=(decnum/100)%10; 00130 uint8_t c4=(decnum/1000)%10; 00131 uint8_t c5=(decnum/10000)%10; 00132 UARTPutChar(UARTx, '0'+c5); 00133 UARTPutChar(UARTx, '0'+c4); 00134 UARTPutChar(UARTx, '0'+c3); 00135 UARTPutChar(UARTx, '0'+c2); 00136 UARTPutChar(UARTx, '0'+c1); 00137 } 00138 00139 /*********************************************************************//** 00140 * @brief Puts a decimal number to UART port 00141 * @param[in] UARTx Pointer to UART peripheral 00142 * @param[in] decnum Decimal number (8-bit long) 00143 * @return None 00144 **********************************************************************/ 00145 void UARTPutDec32(LPC_UART_TypeDef *UARTx, uint32_t decnum) 00146 { 00147 uint8_t c1=decnum%10; 00148 uint8_t c2=(decnum/10)%10; 00149 uint8_t c3=(decnum/100)%10; 00150 uint8_t c4=(decnum/1000)%10; 00151 uint8_t c5=(decnum/10000)%10; 00152 uint8_t c6=(decnum/100000)%10; 00153 uint8_t c7=(decnum/1000000)%10; 00154 uint8_t c8=(decnum/10000000)%10; 00155 uint8_t c9=(decnum/100000000)%10; 00156 uint8_t c10=(decnum/100000000)%10; 00157 UARTPutChar(UARTx, '0'+c10); 00158 UARTPutChar(UARTx, '0'+c9); 00159 UARTPutChar(UARTx, '0'+c8); 00160 UARTPutChar(UARTx, '0'+c7); 00161 UARTPutChar(UARTx, '0'+c6); 00162 UARTPutChar(UARTx, '0'+c5); 00163 UARTPutChar(UARTx, '0'+c4); 00164 UARTPutChar(UARTx, '0'+c3); 00165 UARTPutChar(UARTx, '0'+c2); 00166 UARTPutChar(UARTx, '0'+c1); 00167 } 00168 00169 /*********************************************************************//** 00170 * @brief Puts a hex number to UART port 00171 * @param[in] UARTx Pointer to UART peripheral 00172 * @param[in] hexnum Hex number (8-bit long) 00173 * @return None 00174 **********************************************************************/ 00175 void UARTPutHex (LPC_UART_TypeDef *UARTx, uint8_t hexnum) 00176 { 00177 uint8_t nibble, i; 00178 00179 UARTPuts(UARTx, "0x"); 00180 i = 1; 00181 do { 00182 nibble = (hexnum >> (4*i)) & 0x0F; 00183 UARTPutChar(UARTx, (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble)); 00184 } while (i--); 00185 } 00186 00187 00188 /*********************************************************************//** 00189 * @brief Puts a hex number to UART port 00190 * @param[in] UARTx Pointer to UART peripheral 00191 * @param[in] hexnum Hex number (16-bit long) 00192 * @return None 00193 **********************************************************************/ 00194 void UARTPutHex16 (LPC_UART_TypeDef *UARTx, uint16_t hexnum) 00195 { 00196 uint8_t nibble, i; 00197 00198 UARTPuts(UARTx, "0x"); 00199 i = 3; 00200 do { 00201 nibble = (hexnum >> (4*i)) & 0x0F; 00202 UARTPutChar(UARTx, (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble)); 00203 } while (i--); 00204 } 00205 00206 /*********************************************************************//** 00207 * @brief Puts a hex number to UART port 00208 * @param[in] UARTx Pointer to UART peripheral 00209 * @param[in] hexnum Hex number (32-bit long) 00210 * @return None 00211 **********************************************************************/ 00212 void UARTPutHex32 (LPC_UART_TypeDef *UARTx, uint32_t hexnum) 00213 { 00214 uint8_t nibble, i; 00215 00216 UARTPuts(UARTx, "0x"); 00217 i = 7; 00218 do { 00219 nibble = (hexnum >> (4*i)) & 0x0F; 00220 UARTPutChar(UARTx, (nibble > 9) ? ('A' + nibble - 10) : ('0' + nibble)); 00221 } while (i--); 00222 } 00223 00224 ///*********************************************************************//** 00225 // * @brief print function that supports format as same as printf() 00226 // * function of <stdio.h> library 00227 // * @param[in] None 00228 // * @return None 00229 // **********************************************************************/ 00230 //void _printf (const char *format, ...) 00231 //{ 00232 // static char buffer[512 + 1]; 00233 // va_list vArgs; 00234 // char *tmp; 00235 // va_start(vArgs, format); 00236 // vsprintf((char *)buffer, (char const *)format, vArgs); 00237 // va_end(vArgs); 00238 // 00239 // _DBG(buffer); 00240 //} 00241 00242 /*********************************************************************//** 00243 * @brief Initialize Debug frame work through initializing UART port 00244 * @param[in] None 00245 * @return None 00246 **********************************************************************/ 00247 void debug_frmwrk_init(void) 00248 { 00249 UART_CFG_Type UARTConfigStruct; 00250 PINSEL_CFG_Type PinCfg; 00251 00252 #if (USED_UART_DEBUG_PORT==0) 00253 /* 00254 * Initialize UART0 pin connect 00255 */ 00256 PinCfg.Funcnum = 1; 00257 PinCfg.OpenDrain = 0; 00258 PinCfg.Pinmode = 0; 00259 PinCfg.Pinnum = 2; 00260 PinCfg.Portnum = 0; 00261 PINSEL_ConfigPin(&PinCfg); 00262 PinCfg.Pinnum = 3; 00263 PINSEL_ConfigPin(&PinCfg); 00264 #elif #if (USED_UART_DEBUG_PORT==1) 00265 /* 00266 * Initialize UART1 pin connect 00267 */ 00268 PinCfg.Funcnum = 1; 00269 PinCfg.OpenDrain = 0; 00270 PinCfg.Pinmode = 0; 00271 PinCfg.Pinnum = 15; 00272 PinCfg.Portnum = 0; 00273 PINSEL_ConfigPin(&PinCfg); 00274 PinCfg.Pinnum = 16; 00275 PINSEL_ConfigPin(&PinCfg); 00276 #endif 00277 00278 /* Initialize UART Configuration parameter structure to default state: 00279 * Baudrate = 9600bps 00280 * 8 data bit 00281 * 1 Stop bit 00282 * None parity 00283 */ 00284 UART_ConfigStructInit(&UARTConfigStruct); 00285 // Re-configure baudrate to 115200bps 00286 UARTConfigStruct.Baud_rate = 115200; 00287 00288 // Initialize DEBUG_UART_PORT peripheral with given to corresponding parameter 00289 UART_Init((LPC_UART_TypeDef *)DEBUG_UART_PORT, &UARTConfigStruct); 00290 00291 // Enable UART Transmit 00292 UART_TxCmd((LPC_UART_TypeDef *)DEBUG_UART_PORT, ENABLE); 00293 00294 _db_msg = UARTPuts; 00295 _db_msg_ = UARTPuts_; 00296 _db_char = UARTPutChar; 00297 _db_hex = UARTPutHex; 00298 _db_hex_16 = UARTPutHex16; 00299 _db_hex_32 = UARTPutHex32; 00300 _db_dec = UARTPutDec; 00301 _db_dec_16 = UARTPutDec16; 00302 _db_dec_32 = UARTPutDec32; 00303 _db_get_char = UARTGetChar; 00304 } 00305 #endif /*_DBGFWK */
Generated on Tue Jul 12 2022 10:57:55 by
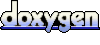