
Final Project for ECE 4180. Morse Code Encoder and Transmission
Dependencies: 4DGL-uLCD-SE EthernetInterface mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "uLCD_4DGL.h" 00003 #include <time.h> 00004 #include <string> 00005 #include "EthernetInterface.h" 00006 00007 // Definitions for networking 00008 const char* ECHO_SERVER_ADDRESS = "10.0.0.32"; 00009 const int ECHO_SERVER_PORT = 7; 00010 00011 // uLCD 00012 uLCD_4DGL uLCD(p9,p10,p11); // serial tx, serial rx, reset pin; 00013 00014 // Mbed Leds 00015 DigitalOut myled1(LED1); 00016 DigitalOut myled2(LED2); 00017 DigitalOut myled3(LED3); 00018 DigitalOut myled4(LED4); 00019 00020 // Push Buttons 00021 DigitalIn pb1(p23); 00022 DigitalIn pb2(p22); 00023 DigitalIn pb3(p21); 00024 00025 // Function declarations 00026 // Screen Declarations 00027 void StartScreen(); 00028 void ClientServerScreen(); 00029 void HelpScreen(); 00030 void InputScreen(); 00031 void EncryptionScreen(); 00032 void DecryptionScreen(); 00033 void CaesarShiftScreen(); 00034 // Actual Functions 00035 string MorseCode(string a); 00036 00037 // UDP Transmission Functions 00038 void Client(string message); 00039 string Server(); 00040 // Cipher declarations 00041 // opt => 0 Encrypt opt => 1 Decrypt 00042 string CaesarCipher(string word,int opt, int shift); 00043 string PolybiusSquareCipher(string a,int opt); 00044 string PolybiusTableTranslation(char letter); 00045 string PolybiusTableLookup(string number); 00046 00047 int main() { 00048 00049 // Create pullups 00050 pb1.mode(PullUp); 00051 pb2.mode(PullUp); 00052 pb3.mode(PullUp); 00053 00054 // Constants 00055 time_t start,end; 00056 double dif; 00057 string morseinput = ""; 00058 string morsetranslation = ""; 00059 string cipheredinput; 00060 string morsereceived = ""; 00061 string cipherreceived = ""; 00062 int clientserver; 00063 int firsttime = 0; 00064 int firsttimeserver = 0; 00065 string chosencipher = ""; 00066 int shiftvalue = 0; 00067 bool inserting = true; 00068 EthernetInterface ethclient; 00069 EthernetInterface ethserver; 00070 const char * IPaddress = "10.0.0.32"; // 10.10.0.65 00071 const char * NetworkMask = "255.255.255.0"; 00072 const char * Gateway = "10.0.0.1"; // 10.10.0.1 00073 00074 // Welcome Screen 00075 StartScreen(); 00076 00077 // pb1 => up 00078 // pb2 => down 00079 // pb3 => right 00080 // 0 - Client 00081 // 1 - Server 00082 while(true) // Infinite Loop. Restart at ClientServerScreen. 00083 { 00084 morseinput = ""; 00085 morsetranslation = ""; 00086 morsereceived = ""; 00087 cipherreceived = ""; 00088 clientserver = 0; 00089 uLCD.cls(); 00090 ClientServerScreen(); 00091 shiftvalue = 0; 00092 while(pb2 && pb1) 00093 { 00094 } 00095 00096 if(!pb1) // pb1 was pressed, do Client Network 00097 { 00098 clientserver = 0; 00099 } 00100 else if(!pb2) 00101 { 00102 clientserver = 1; // pb2 was pressed, do Server Network 00103 } 00104 00105 if(clientserver == 0) 00106 { 00107 uLCD.cls(); 00108 if(firsttime==0) 00109 { 00110 HelpScreen(); 00111 } 00112 uLCD.cls(); 00113 InputScreen(); 00114 // Input Morse Code below. pb1 to input. 00115 // pb2 to move to the next step 00116 start = 0; 00117 end = 0; 00118 while(pb3) 00119 { 00120 00121 if(!pb1) // Means a dot was inserted 00122 { 00123 while(!pb1) // Need to wait until the button is released 00124 { 00125 } 00126 morseinput.append("."); 00127 inserting = true; 00128 time(&start); 00129 } 00130 else if(!pb2) 00131 { 00132 while(!pb2) // Need to wait till release 00133 { 00134 } 00135 morseinput.append("-"); 00136 inserting = true; 00137 time(&start); 00138 } 00139 00140 if(start !=0.0) 00141 { 00142 time(&end); 00143 dif = difftime(end,start); 00144 if(dif>1) 00145 { 00146 string e = MorseCode(morseinput); 00147 if(e!="error") 00148 { 00149 morsetranslation.append(e); 00150 uLCD.printf("%s",e); 00151 } 00152 start = 0; 00153 end = 0; 00154 morseinput = ""; 00155 } 00156 } 00157 } 00158 00159 uLCD.cls(); 00160 EncryptionScreen(); 00161 00162 while(pb2 && pb1 && pb3) 00163 { 00164 } 00165 00166 if(!pb1) // pb1 was pressed, do caesar cipher 00167 { 00168 uLCD.cls(); 00169 CaesarShiftScreen(); 00170 shiftvalue = 0; 00171 uLCD.locate(0,4); 00172 uLCD.printf("%02d",shiftvalue); 00173 while(pb3) 00174 { 00175 if(!pb1) // Means go up one value 00176 { 00177 while(!pb1) // Need to wait until the button is released 00178 { 00179 } 00180 shiftvalue = shiftvalue + 1; 00181 if(shiftvalue>25) 00182 { 00183 shiftvalue = 25; 00184 } 00185 uLCD.locate(0,4); 00186 uLCD.printf("%02d",shiftvalue); 00187 } 00188 if(!pb2) // Means go up one value 00189 { 00190 while(!pb2) // Need to wait until the button is released 00191 { 00192 } 00193 shiftvalue = shiftvalue - 1; 00194 if(shiftvalue<0) 00195 { 00196 shiftvalue = 0; 00197 } 00198 uLCD.locate(0,4); 00199 uLCD.printf("%02d",shiftvalue); 00200 } 00201 } 00202 cipheredinput = CaesarCipher(morsetranslation,0,shiftvalue); 00203 chosencipher = "Caesar Cipher"; 00204 } 00205 else if(!pb2) // pb2 was pressed, do Polybius Square cipher 00206 { 00207 cipheredinput = PolybiusSquareCipher(morsetranslation,0); 00208 chosencipher = "Polybius Sqre"; 00209 } 00210 else if(!pb3) // No Cipher 00211 { 00212 cipheredinput = morsetranslation; 00213 chosencipher = "No Cipher"; 00214 } 00215 wait(2); 00216 00217 // Once the morse input comes in through here. 00218 // Tell the user that we are about to send it all. 00219 uLCD.cls(); 00220 uLCD.printf("%s\n",chosencipher); 00221 uLCD.printf("Sending Value\n\n"); 00222 uLCD.printf("\n%s\n",morsetranslation); 00223 uLCD.printf("\n%s\n",cipheredinput); 00224 wait(3); 00225 00226 if(firsttime == 0) 00227 { 00228 ethclient.init(); 00229 firsttime = 1; 00230 } 00231 00232 ethclient.connect(); 00233 UDPSocket sock; 00234 sock.init(); 00235 Endpoint echo_server; 00236 echo_server.set_address(ECHO_SERVER_ADDRESS, ECHO_SERVER_PORT); 00237 char out_buffer[1024]; 00238 strncpy(out_buffer, cipheredinput.c_str(), sizeof(out_buffer)); 00239 out_buffer[sizeof(out_buffer) - 1] = 0; 00240 //uLCD.printf("\Client IP Address is %s\n", ethclient.getIPAddress()); 00241 //wait(4); 00242 sock.sendTo(echo_server, out_buffer, sizeof(out_buffer)); 00243 sock.close(); 00244 ethclient.disconnect(); 00245 // technically the eth should still be connected 00246 uLCD.cls(); 00247 uLCD.printf("Message has been sent"); 00248 wait(2); 00249 } 00250 else 00251 { 00252 uLCD.cls(); 00253 uLCD.printf("Receiving..."); 00254 00255 if(firsttimeserver == 0) 00256 { 00257 //uLCD.printf("first time server"); 00258 ethserver.init(IPaddress, NetworkMask, Gateway); 00259 firsttimeserver = 1; 00260 } 00261 //uLCD.printf("initialized"); 00262 ethserver.connect(); 00263 UDPSocket server; 00264 server.bind(ECHO_SERVER_PORT); 00265 Endpoint client; 00266 char buffer[256]; 00267 //uLCD.printf("\Server IP Address is %s\n", ethserver.getIPAddress()); 00268 string message; 00269 int n = server.receiveFrom(client, buffer, sizeof(buffer)); 00270 buffer[n] = '\0'; 00271 00272 string message2(buffer); 00273 server.close(); 00274 ethserver.disconnect(); 00275 uLCD.cls(); 00276 uLCD.printf("Message %s",message2); 00277 wait(3); 00278 morsereceived = message2; 00279 // string morsereceived = ""; 00280 // string cipherreceived = ""; 00281 00282 // After Reception, User will have 2 options to decipher 00283 // Engima or Caesar. 00284 uLCD.cls(); 00285 DecryptionScreen(); 00286 while(pb2 && pb1 && pb3) 00287 { 00288 } 00289 00290 if(!pb1) // pb1 was pressed, do caesar cipher 00291 { 00292 uLCD.cls(); 00293 CaesarShiftScreen(); 00294 shiftvalue = 0; 00295 uLCD.locate(0,4); 00296 uLCD.printf("%02d",shiftvalue); 00297 while(pb3) 00298 { 00299 if(!pb1) // Means go up one value 00300 { 00301 while(!pb1) // Need to wait until the button is released 00302 { 00303 } 00304 shiftvalue = shiftvalue + 1; 00305 if(shiftvalue>25) 00306 { 00307 shiftvalue = 25; 00308 } 00309 uLCD.locate(0,4); 00310 uLCD.printf("%02d",shiftvalue); 00311 } 00312 if(!pb2) // Means go up one value 00313 { 00314 while(!pb2) // Need to wait until the button is released 00315 { 00316 } 00317 shiftvalue = shiftvalue - 1; 00318 if(shiftvalue<0) 00319 { 00320 shiftvalue = 0; 00321 } 00322 uLCD.locate(0,4); 00323 uLCD.printf("%02d",shiftvalue); 00324 } 00325 } 00326 cipherreceived = CaesarCipher(morsereceived,1,shiftvalue); // Second input = 1, for decryption 00327 } 00328 else if(!pb2) // pb2 was pressed, do Polybius Square Cipher 00329 { 00330 cipherreceived = PolybiusSquareCipher(morsereceived,1); // Second input = 1, for decryption 00331 } 00332 else if(!pb3) 00333 { 00334 cipherreceived = morsereceived; 00335 } 00336 uLCD.cls(); 00337 uLCD.printf("Decryption\n\n"); 00338 uLCD.printf("%s\n",morsereceived); 00339 uLCD.printf("%s\n",cipherreceived); 00340 wait(5); 00341 } 00342 } 00343 } 00344 00345 00346 // Start Screen to show Welcome Page 00347 void StartScreen() 00348 { 00349 uLCD.printf("Welcome to the Morse Code Enconder and Transmitter.\n\n\n"); 00350 wait(4); 00351 } 00352 00353 // Screen to choose between Client and Servers 00354 void ClientServerScreen() 00355 { 00356 uLCD.printf("Choose an option:\n\n"); 00357 uLCD.printf("1 - Transmit\n"); 00358 uLCD.printf("2 - Receive\n"); 00359 //uLCD.printf("3 - Checkers\n"); 00360 } 00361 00362 // Screen 2 00363 void HelpScreen() 00364 { 00365 uLCD.printf("Up => . \n\n"); 00366 uLCD.printf("Down => - \n\n"); 00367 uLCD.printf("Right => Submit\n"); 00368 wait(2); 00369 } 00370 00371 // Screen 3 00372 void InputScreen() 00373 { 00374 uLCD.printf("Input Morse Code\n"); 00375 } 00376 00377 // Screen 4 00378 void EncryptionScreen() 00379 { 00380 uLCD.printf("Choose encryption:\n\n"); 00381 uLCD.printf("1 - Caesar Cipher\n"); 00382 uLCD.printf("2 - Polybius Sqre\n"); 00383 uLCD.printf("3 - No cipher\n"); 00384 } 00385 00386 void DecryptionScreen() 00387 { 00388 uLCD.printf("Choose decryption:\n\n"); 00389 uLCD.printf("1 - Caesar Cipher\n"); 00390 uLCD.printf("2 - Polybius Sqre\n"); 00391 uLCD.printf("3 - No cipher\n"); 00392 } 00393 00394 void CaesarShiftScreen() { 00395 uLCD.printf("Choose a shift value\n"); 00396 uLCD.printf("UP and DOWN"); 00397 } 00398 00399 // Returns the proper character based on the inputed morse code 00400 string MorseCode(string a) 00401 { 00402 //uLCD.printf("%s",a); 00403 if(a == ".-") { return "A";} 00404 if(a == "-...") { return "B";} 00405 if(a == "-.-.") { return "C";} 00406 if(a == "-..") { return "D";} 00407 if(a == ".") { return "E";} 00408 if(a == "..-.") { return "F";} 00409 if(a == "--.") { return "G";} 00410 if(a == "....") { return "H";} 00411 if(a == "..") { return "I";} 00412 if(a == ".---") { return "J";} 00413 if(a == "-.-") { return "K";} 00414 if(a == ".-..") { return "L";} 00415 if(a == "--") { return "M";} 00416 if(a == "-.") { return "N";} 00417 if(a == "---") { return "O";} 00418 if(a == ".--.") { return "P";} 00419 if(a == "--.-") { return "Q";} 00420 if(a == ".-.") { return "R";} 00421 if(a == "...") { return "S";} 00422 if(a == "-") { return "T";} 00423 if(a == "..-") { return "U";} 00424 if(a == "...-") { return "V";} 00425 if(a == ".--") { return "W";} 00426 if(a == "-..-") { return "X";} 00427 if(a == "-.--") { return "Y";} 00428 if(a == "--..") { return "Z";} 00429 if(a == ".----") { return "1";} 00430 if(a == "..---") { return "2";} 00431 if(a == "...--") { return "3";} 00432 if(a == "....-") { return "4";} 00433 if(a == ".....") { return "5";} 00434 if(a == "-....") { return "6";} 00435 if(a == "--...") { return "7";} 00436 if(a == "---..") { return "8";} 00437 if(a == "----.") { return "9";} 00438 if(a == "-----") { return "0";} 00439 return "error"; 00440 } 00441 00442 // Cipher Codes by Ishita 00443 00444 00445 00446 // Find the length of the string 00447 // Loop through it, and replace each a[i] with its equivalent that is 3 letters later. 00448 // For that will have to loop through Alphabet and find the position of said letter. 00449 // Then increment. So hopefully there is a isin(alphabet,"A) -> that returns the index, position etc. 00450 string CaesarCipher(string word, int opt, int shift) 00451 { 00452 string alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ"; 00453 if(opt == 0) // Encrypt 00454 { 00455 string encrypted = ""; 00456 for (int x = 0; x < word.length(); x++) { 00457 char c = word[x]; 00458 int ind = alphabet.find(c); 00459 ind = (ind + shift) % 26; 00460 encrypted += alphabet[ind]; 00461 } 00462 return encrypted; 00463 } else if (opt == 1) { //Decrypt 00464 string decrypted = ""; 00465 for (int x = 0; x < word.length(); x++) { 00466 char c = word[x]; 00467 int ind = alphabet.find(c); 00468 ind = (ind - shift) % 26; 00469 decrypted += alphabet[ind]; 00470 } 00471 return decrypted; 00472 } else { 00473 return "Error"; 00474 } 00475 } 00476 00477 //Polybius Square cipher 00478 string PolybiusSquareCipher(string word,int opt) 00479 { 00480 string number = ""; 00481 string numbers = ""; 00482 if(opt == 0) // Encrypt 00483 { 00484 string encrypted = ""; 00485 string rows = ""; 00486 string cols = ""; 00487 for (int x = 0; x < word.length(); x++) { 00488 char c = word[x]; 00489 number = PolybiusTableTranslation(c); 00490 rows += number[0]; 00491 cols += number[1]; 00492 } 00493 numbers = rows + cols; 00494 for (int y = 0; y < numbers.length(); y = y + 2) { 00495 encrypted += PolybiusTableLookup(numbers.substr(y,2)); 00496 } 00497 return encrypted; 00498 } else if (opt == 1) { //Decrypt 00499 string decrypted = ""; 00500 for (int x = 0; x < word.length(); x++) { 00501 char c = word[x]; 00502 number = PolybiusTableTranslation(c); 00503 numbers += number; 00504 } 00505 int length = numbers.length()/2; 00506 for (int y = 0; y < length; y++) { 00507 string newNum = ""; 00508 newNum += numbers[y]; 00509 newNum += numbers[y + length]; 00510 decrypted += PolybiusTableLookup(newNum); 00511 } 00512 return decrypted; 00513 } else { 00514 return "Error"; 00515 } 00516 } 00517 00518 00519 00520 string PolybiusTableTranslation(char letter) { 00521 if (letter == 'A') {return "11";} 00522 if (letter == 'B') {return "12";} 00523 if (letter == 'C') {return "13";} 00524 if (letter == 'D') {return "14";} 00525 if (letter == 'E') {return "15";} 00526 if (letter == 'F') {return "21";} 00527 if (letter == 'G') {return "22";} 00528 if (letter == 'H') {return "23";} 00529 if (letter == 'I') {return "24";} 00530 if (letter == 'J') {return "24";} 00531 if (letter == 'K') {return "25";} 00532 if (letter == 'L') {return "31";} 00533 if (letter == 'M') {return "32";} 00534 if (letter == 'N') {return "33";} 00535 if (letter == 'O') {return "34";} 00536 if (letter == 'P') {return "35";} 00537 if (letter == 'Q') {return "41";} 00538 if (letter == 'R') {return "42";} 00539 if (letter == 'S') {return "43";} 00540 if (letter == 'T') {return "44";} 00541 if (letter == 'U') {return "45";} 00542 if (letter == 'V') {return "51";} 00543 if (letter == 'W') {return "52";} 00544 if (letter == 'X') {return "53";} 00545 if (letter == 'Y') {return "54";} 00546 if (letter == 'Z') {return "55";} 00547 00548 return "error"; 00549 } 00550 00551 string PolybiusTableLookup(string number) { 00552 if (number == "11") {return "A";} 00553 if (number == "12") {return "B";} 00554 if (number == "13") {return "C";} 00555 if (number == "14") {return "D";} 00556 if (number == "15") {return "E";} 00557 if (number == "21") {return "F";} 00558 if (number == "22") {return "G";} 00559 if (number == "23") {return "H";} 00560 if (number == "24") {return "I/J";} 00561 if (number == "25") {return "K";} 00562 if (number == "31") {return "L";} 00563 if (number == "32") {return "M";} 00564 if (number == "33") {return "N";} 00565 if (number == "34") {return "O";} 00566 if (number =="35") {return "P";} 00567 if (number == "41") {return "Q";} 00568 if (number == "42") {return "R";} 00569 if (number == "43") {return "S";} 00570 if (number == "44") {return "T";} 00571 if (number == "45") {return "U";} 00572 if (number == "51") {return "V";} 00573 if (number == "52") {return "W";} 00574 if (number == "53") {return "X";} 00575 if (number == "54") {return "Y";} 00576 if (number == "55") {return "Z";} 00577 00578 return "error"; 00579 } 00580 00581 00582 00583
Generated on Wed Jul 13 2022 17:22:24 by
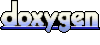