
Code for The game of Pong using Photocell Resistors
Dependencies: 4DGL-uLCD-SE SDFileSystem mbed-rtos mbed wave_player
main.cpp
00001 #include "mbed.h" 00002 #include "uLCD_4DGL.h" 00003 #include "SDFileSystem.h" 00004 #include "wave_player.h" 00005 #include <string> 00006 #include "rtos.h" 00007 00008 // ULCD 00009 uLCD_4DGL uLCD(p9,p10,p17); // serial tx, serial rx, reset pin; 00010 00011 // Speaker Output 00012 AnalogOut DACout(p18); 00013 wave_player waver(&DACout); 00014 00015 // SD Cards 00016 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00017 00018 // Paddle Left 00019 AnalogIn photocellleft(p15); 00020 // Paddle Right 00021 AnalogIn photocellright(p16); 00022 00023 //std::string a 00024 void Sound(std::string event){ 00025 FILE *wave_file; 00026 00027 if(event == "hit") 00028 wave_file=fopen("/sd/wavfiles/pac_bonus.wav","r"); 00029 else if(event == "end") 00030 wave_file=fopen("/sd/wavfiles/BUZZER.wav","r"); 00031 else if(event == "start") 00032 wave_file=fopen("/sd/wavfiles/BUZZER.wav","r"); 00033 00034 waver.play(wave_file); 00035 fclose(wave_file); 00036 wait(1); 00037 } 00038 00039 int border = 128; 00040 int paddlesizex = 5; 00041 int paddlesizey = 20; 00042 int paddlecolor = 0x00FF00; 00043 int ballradius = 3; 00044 int ballcolor = 0xFF00FF; 00045 int black = 0x000000; 00046 00047 // Paddle left 00048 00049 int paddlelx = 0; 00050 int paddlely = 0; 00051 int paddlerx = border-paddlesizex; 00052 int paddlery = 50; 00053 00054 int oldpaddlely = paddlely; 00055 int oldpaddlery = paddlery; 00056 00057 int paddlelincr = 1; 00058 int paddlerincr = 1; 00059 00060 // Ball x,y , should be randomized to begin 00061 int ballx = border/2; 00062 int bally = rand() % 100; 00063 00064 int oldballx = ballx; 00065 int oldbally = bally; 00066 // Increments for ball 00067 int ballxincr = -1; 00068 int ballyincr = -1; 00069 00070 int oldphotocell = photocellleft; 00071 00072 int hitvariable = 0; 00073 00074 // Threading 00075 void function_thread3(void const *name){ 00076 FILE *wave_file; 00077 //printf("\n\n\nHello, wave world!\n"); 00078 while(true){ 00079 if(hitvariable == 1){ 00080 wave_file=fopen("/sd/wavfiles/pac_bonus.wav","r"); 00081 waver.play(wave_file); 00082 fclose(wave_file); 00083 hitvariable = 0; 00084 //wait(.2); 00085 } 00086 } 00087 } 00088 00089 // ULCD Functions 00090 void DrawPaddle(int x, int y,int color){ 00091 // Green paddles 00092 uLCD.filled_rectangle(x, y, x+paddlesizex, y+paddlesizey, color); 00093 } 00094 00095 void DrawBall(int x, int y,int color){ 00096 uLCD.filled_circle(x, y, ballradius, color); 00097 } 00098 00099 int main() { 00100 // Begin the game 00101 Sound("start"); 00102 00103 // Thread for Sound 00104 Thread thread3(function_thread3); 00105 00106 // Baudrate 00107 uLCD.baudrate(300000); 00108 int initialphotocell = photocellleft*100/2; 00109 //uLCD.printf("%d",initialphotocell); 00110 //wait(10); 00111 // Rectangle 00112 DrawPaddle(paddlelx,paddlely,paddlecolor); 00113 //wait(5); 00114 DrawPaddle(paddlerx,paddlery,paddlecolor); 00115 00116 DrawBall(ballx,bally,ballcolor); 00117 00118 while(true){ 00119 // Update Positions 00120 oldballx = ballx; 00121 oldbally = bally; 00122 ballx += ballxincr; 00123 bally += ballyincr; 00124 oldpaddlely = paddlely; 00125 oldpaddlery = paddlery; 00126 paddlely += paddlelincr; 00127 paddlery += paddlerincr; 00128 00129 // Ball Collision with right wall 00130 if(ballx+ballradius>=border){ 00131 // Lose 00132 //ballxincr = -2; 00133 Sound("end"); 00134 ballx = border/2; 00135 bally = rand() % 100; 00136 ballxincr = -1; 00137 ballyincr = -ballyincr; 00138 //hitvariable = 1; 00139 //Sound("hit"); 00140 } 00141 // Ball Collision with left wall 00142 else if(ballx<=0){ 00143 // Lose 00144 Sound("end"); 00145 ballx = border/2; 00146 bally = rand() % 100; 00147 ballxincr = 1; 00148 ballyincr = -ballyincr; 00149 //hitvariable = 1; 00150 //Sound("hit"); 00151 } 00152 // Ball Collision with top and Bottom 00153 if(bally+ballradius>=border){ 00154 ballyincr = -1; 00155 hitvariable = 1; 00156 //Sound("hit"); 00157 } 00158 else if(bally<=0){ 00159 ballyincr = 1; 00160 hitvariable = 1; 00161 //Sound("hit"); 00162 } 00163 00164 // Left Paddle Collisions 00165 if(ballx-ballradius<=paddlelx+paddlesizex && bally+ballradius>paddlely && bally-ballradius<paddlely+paddlesizey){ 00166 ballxincr = 1; 00167 } 00168 // Right Paddle Collision 00169 else if(ballx+ballradius>=paddlerx && bally+ballradius>paddlery && bally-ballradius<paddlery+paddlesizey){ 00170 ballxincr = -1; 00171 } 00172 // Paddle not fall off the screen 00173 if(paddlely<0){ 00174 paddlely = 0; 00175 //paddlelincr = 1; 00176 } 00177 else if(paddlely+paddlesizey>=border){ 00178 paddlely = border - paddlesizey; 00179 //paddlelincr = -1; 00180 } 00181 if(paddlery<=0){ 00182 paddlery = 0; 00183 //paddlerincr = 1; 00184 } 00185 else if(paddlery+paddlesizey>=border){ 00186 paddlery = border - paddlesizey; 00187 //paddlerincr = -1; 00188 } 00189 // Get light from Photocells 00190 if(photocellleft*100 >initialphotocell) 00191 { 00192 paddlelincr = -2; 00193 } 00194 else 00195 { 00196 paddlelincr = 2; 00197 } 00198 if(photocellright*100 > initialphotocell) 00199 { 00200 paddlerincr = -2; 00201 } 00202 else 00203 { 00204 paddlerincr = 2; 00205 } 00206 //oldphotocell = photocell; 00207 DrawBall(oldballx,oldbally,black); 00208 DrawBall(ballx,bally,ballcolor); 00209 DrawPaddle(paddlelx,oldpaddlely,black); 00210 DrawPaddle(paddlerx,oldpaddlery,black); 00211 DrawPaddle(paddlelx,paddlely,paddlecolor); 00212 DrawPaddle(paddlerx,paddlery,paddlecolor); 00213 } 00214 } 00215 00216 00217 00218 00219 00220 00221 00222 00223 00224 00225 00226 00227 00228
Generated on Sat Jul 23 2022 08:25:13 by
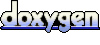