PN532 Driver library This library provides an abstract API to drive the pn532 nfc chip, with I2C/HSU/SPI interface. Its based on the Seeed Studio's Arduino version.
Dependents: PN532_ReadUid Nfctest2
HardwareSerial.h
00001 /* 00002 Copyright (c) 2011 Arduino. All right reserved. 00003 00004 This library is free software; you can redistribute it and/or 00005 modify it under the terms of the GNU Lesser General Public 00006 License as published by the Free Software Foundation; either 00007 version 2.1 of the License, or (at your option) any later version. 00008 00009 This library is distributed in the hope that it will be useful, 00010 but WITHOUT ANY WARRANTY; without even the implied warranty of 00011 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. 00012 See the GNU Lesser General Public License for more details. 00013 00014 You should have received a copy of the GNU Lesser General Public 00015 License along with this library; if not, write to the Free Software 00016 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00017 */ 00018 00019 #ifndef __HARDWARESERIAL_H 00020 #define __HARDWARESERIAL_H 00021 00022 #include "mbed.h" 00023 #include <inttypes.h> 00024 #include "BufferedSerial.h" 00025 00026 00027 class HardwareSerial : public BufferedSerial 00028 { 00029 public: 00030 HardwareSerial (PinName tx, PinName rx) : BufferedSerial (tx, rx) { 00031 00032 } 00033 //virtual void begin(unsigned long) = 0; 00034 //virtual void end() = 0; 00035 //virtual int available(void) = 0; 00036 //virtual int peek(void) = 0; 00037 //virtual int read(void) = 0; 00038 //virtual void flush(void) = 0; 00039 // inline size_t write(uint8_t val) { 00040 // putc (val); 00041 // return 1; 00042 // } 00043 00044 // inline size_t write(const uint8_t *buffer, size_t size) { 00045 // size_t i ; 00046 // for (i = 0; i < size; i ++) 00047 // putc (buffer[i]); 00048 // 00049 // return size; 00050 // } 00051 00052 //using Print::write; // pull in write(str) and write(buf, size) from Print 00053 //virtual operator bool() = 0; 00054 }; 00055 00056 extern void serialEventRun(void) __attribute__((weak)); 00057 00058 #endif // __HARDWARESERIAL_H 00059
Generated on Tue Jul 12 2022 20:44:47 by
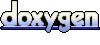