Simple library and program for the SparkFun Ardumoto Shield https://www.sparkfun.com/products/9815 with the ST Nucleo F401RE The SparkFun Ardumoto shield can control two DC motors (up to 2 amps per motor). It is based on the L298 H-bridge. Developped by : Didier Donsez & Jérome Maisonnasse License: CC-SA 3.0, feel free to use this code however you'd like. Please improve upon it! Let me know how you've made it better. This is really simple example code to get you some basic functionality with the Ardumoto Shield.
ArduMotoShield.cpp
00001 /* 00002 Simple library for the SparkFun Ardumoto Shield https://www.sparkfun.com/products/9815 00003 with the ST Nucleo F401RE 00004 00005 The SparkFun Ardumoto shield can control two DC motors (up to 2 amps per motor). It is based on the L298 H-bridge. 00006 00007 Developped by : Didier Donsez & Jérome Maisonnasse 00008 00009 License: CC-SA 3.0, feel free to use this code however you'd like. 00010 Please improve upon it! Let me know how you've made it better. 00011 00012 This is really simple example code to get you some basic functionality with the Ardumoto Shield. 00013 00014 */ 00015 00016 #include "ArduMotoShield.h" 00017 #include "mbed.h" 00018 00019 // depend of the Vin and the max voltage of the motors 00020 float MAXPWM = 0.5f; 00021 00022 #define LOW 0 00023 #define HIGH 1 00024 00025 DigitalOut inApin(D12, LOW); //direction control for motor outputs 1 and 2 is on digital pin 12 00026 DigitalOut inBpin(D13, LOW); //direction control for motor outputs 3 and 4 is on digital pin 13 00027 PwmOut pwmApin(D3); //PWM control for motor outputs 1 and 2 is on digital pin 3 00028 PwmOut pwmBpin(D11); //PWM control for motor outputs 3 and 4 is on digital pin 11 00029 00030 bool isSetup=false; 00031 00032 void ArduMotoShield::setVoltages(float vin, float vmaxmotor) 00033 { 00034 00035 if(vin<vmaxmotor) 00036 MAXPWM=1.0f; 00037 else 00038 MAXPWM=vmaxmotor/vin; 00039 } 00040 00041 00042 void ArduMotoShield::setup() 00043 { 00044 pwmApin.period_ms(10); 00045 pwmApin.pulsewidth_ms(1); 00046 pwmApin.write(0.0f); 00047 00048 pwmBpin.period_ms(10); 00049 pwmBpin.pulsewidth_ms(1); 00050 pwmBpin.write(0.0f); 00051 00052 isSetup=true; 00053 } 00054 00055 // Basic ArduMotoShield operations 00056 00057 void ArduMotoShield::forward() //full speed forward 00058 { 00059 if(!isSetup) setup(); 00060 00061 inApin.write(LOW); 00062 inBpin.write(LOW); 00063 pwmApin.write(MAXPWM); 00064 pwmBpin.write(MAXPWM); 00065 printf("Forward\n"); 00066 } 00067 00068 void ArduMotoShield::backward() //full speed backward 00069 { 00070 if(!isSetup) setup(); 00071 00072 inApin.write(HIGH); 00073 inBpin.write(HIGH); 00074 pwmApin.write(MAXPWM); 00075 pwmBpin.write(MAXPWM); 00076 printf("Backward\n"); 00077 } 00078 00079 void ArduMotoShield::stop() 00080 { 00081 if(!isSetup) setup(); 00082 00083 inApin.write(LOW); 00084 inBpin.write(LOW); 00085 pwmApin.write(0.0f); 00086 pwmBpin.write(0.0f); 00087 printf("Stop\n"); 00088 } 00089 00090 void ArduMotoShield::right() 00091 { 00092 if(!isSetup) setup(); 00093 00094 inApin.write(HIGH); 00095 inBpin.write(LOW); 00096 pwmApin.write(MAXPWM); 00097 pwmBpin.write(MAXPWM); 00098 printf("Right\n"); 00099 } 00100 00101 void ArduMotoShield::left() 00102 { 00103 if(!isSetup) setup(); 00104 00105 inApin.write(LOW); 00106 inBpin.write(HIGH); 00107 pwmApin.write(MAXPWM); 00108 pwmBpin.write(MAXPWM); 00109 printf("Left\n"); 00110 }
Generated on Thu Jul 14 2022 00:15:48 by
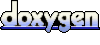