Embed:
(wiki syntax)
Show/hide line numbers
zg_defs.h
Go to the documentation of this file.
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /** 00025 @file 00026 ZG2100 Low-level driver definitions 00027 */ 00028 //Donatien Garnier 2010 00029 00030 #ifndef ZG_DEFS_H 00031 #define ZG_DEFS_H 00032 00033 /* Parameters */ 00034 00035 #define ZG_HEAD_BUF_SIZE 64//16 00036 #define ZG_FIFO_BUF_SIZE 2048 00037 00038 #define ZG_MAX_TX_PENDING 7 00039 00040 #define ZG_SCAN_PROBE_DELAY 20 //In us 00041 #define ZG_SCAN_MIN_CHAN_TIME 400 //In (1024 us) 00042 #define ZG_SCAN_MAX_CHAN_TIME 800 //In (1024 us) 00043 00044 #define ZG_REGION ZG_REGION_EUROPE 00045 00046 /* Interface-specific constants */ 00047 00048 #define ZG_SSID_LEN 32 //Max SSID length 00049 #define ZG_MAX_SUPPORTED_RATES 8 //Max number of supported rates 00050 #define ZG_SCAN_MAX_CHANNELS 14 00051 #define ZG_MACADDR_LEN 6 00052 #define ZG_SNAP_LEN 6 00053 00054 #define ZG_REGION_EUROPE 2 //Channels 1 - 13 00055 #define ZG_REGION_MEA 2 //Channels 1 - 13 00056 #define ZG_REGION_AMERICAS 0 //Channels 1 - 11 00057 #define ZG_REGION_DEFAULT 1 //Channels 1 - 11 00058 #define ZG_REGION_ASIAPAC 2 //Channels 1 - 13 00059 #define ZG_REGION_JAPAN_A 5 //Channel 14 only 00060 00061 #define ZG_WEP_KEYS_COUNT 4 00062 #define ZG_WEP_KEY_LEN 13 00063 #define ZG_WPA_PASS_LEN 64 00064 #define ZG_PMK_LEN 32 00065 00066 /* Commands */ 00067 00068 #define ZG_CMD_REG 0x00 00069 #define ZG_CMD_REG_RD (ZG_CMD_REG | 0x40) 00070 #define ZG_CMD_REG_WR (ZG_CMD_REG | 0x00) 00071 00072 #define ZG_CMD_FIFO 0x80 00073 00074 #define ZG_CMD_FIFO_RD_DATA (ZG_CMD_FIFO | 0x00) 00075 #define ZG_CMD_FIFO_RD_MGMT (ZG_CMD_FIFO | 0x00) 00076 #define ZG_CMD_FIFO_RD_DONE (ZG_CMD_FIFO | 0x50) 00077 00078 #define ZG_CMD_FIFO_WR_DATA (ZG_CMD_FIFO | 0x20) 00079 #define ZG_CMD_FIFO_WR_MGMT (ZG_CMD_FIFO | 0x30) 00080 #define ZG_CMD_FIFO_WR_DONE (ZG_CMD_FIFO | 0x40) 00081 00082 /* Registers */ 00083 00084 #define ZG_REG_INTF 0x01 //8-bit register, interrupt raised flags 00085 #define ZG_REG_INTE 0x02 //8-bit register, interrupt enable flags 00086 00087 #define ZG_REG_INTF2 0x2d //16-bit register, 2nd level interrupt raised flags 00088 #define ZG_REG_INTE2 0x2e //16-bit register, 2nd level interrupt enable flags 00089 00090 #define ZG_REG_SYSINFO 0x21 //8-bit register, read sys info data window 00091 #define ZG_REG_SYSINFO_INDEX 0x2b //16-bit register, sys info data window offset 00092 #define ZG_REG_SYSINFO_STATUS 0x2c //16-bit register, sys info data window status 00093 00094 #define ZG_REG_F0_CTRL0 0x25 //16-bit register, FIFO0 Ctrl reg 00095 #define ZG_REG_F0_CTRL1 0x26 //16-bit register, FIFO0 Ctrl reg 00096 #define ZG_REG_F0_INDEX 0x27 //16-bit register, FIFO0 Index reg 00097 #define ZG_REG_F0_STATUS 0x28 //16-bit register, FIFO0 Status reg 00098 00099 #define ZG_REG_F1_CTRL0 0x29 //16-bit register, FIFO1 Ctrl reg 00100 #define ZG_REG_F1_CTRL1 0x2a //16-bit register, FIFO1 Ctrl reg 00101 #define ZG_REG_F1_INDEX 0x2b //16-bit register, FIFO1 Index reg 00102 #define ZG_REG_F1_STATUS 0x2c //16-bit register, FIFO1 Status reg 00103 00104 #define ZG_REG_F0_ROOM 0x2f //16-bit register, room in FIFO0 for writing 00105 00106 #define ZG_REG_F0_LEN 0x33 //16-bit register, bytes ready to read on FIFO0 00107 #define ZG_REG_F1_LEN 0x35 //16-bit register, bytes ready to read on FIFO1 00108 00109 #define ZG_REG_LOWPWR 0x3d //16-bit register, low power mode cfg 00110 #define ZG_REG_IREG_ADDR 0x3e //16-bit register, set/read the ptr to indexed regs 00111 #define ZG_REG_IREG_DATA 0x3f //16-bit register, index regs data 00112 00113 #define ZG_REG_LEN( reg ) ((reg)>=0x25?2:1) //Return bytes len of a register 00114 00115 /* Indexed Registers */ 00116 00117 #define ZG_IREG_HW_STATUS 0x2a //16-bit register, hardware status 00118 #define ZG_IREG_HW_RST 0x2b //16-bit register, reset reg 00119 00120 #define ZG_HW_STATUS_RESET 0x1000 //ZG_IREG_HW_STATUS Mask to determine wether the chip is in reset 00121 00122 #define ZG_IREG_PWR_STATUS 0x3e //16-bit register, power status (sleep state) 00123 00124 #define ZG_IREG_LEN( reg ) 2 //Bytes len of an indexed register 00125 00126 /* FIFOs */ 00127 00128 /* 00129 When using FIFO requests, frame is formatted like this: 00130 [ZG_CMD_FIFO_WR_****][Type][Subtype] 00131 */ 00132 00133 //Just here for zg_fifo_* cmds 00134 #define ZG_FIFO_DATA 0 00135 #define ZG_FIFO_MGMT 1 00136 #define ZG_FIFO_ANY 0 //A specific FIFO does not have to be specified for reads 00137 00138 /* Types */ 00139 00140 #define ZG_FIFO_WR_TXD_REQ 1 //Req to write TX Data 00141 00142 #define ZG_FIFO_RD_TXD_ACK 1 //TX Data ack'ed 00143 00144 #define ZG_FIFO_RD_RXD_AVL 3 //RX Data available 00145 00146 #define ZG_FIFO_WR_MGMT_REQ 2 //Req to read/write mgmt Data 00147 00148 #define ZG_FIFO_RD_MGMT_AVL 2 //Mgmt Data available/written ok 00149 #define ZG_FIFO_RD_MGMT_EVT 4 //Mgmt Data event 00150 00151 /* Subtypes */ 00152 00153 //TXD, RXD 00154 #define ZG_FIFO_TXD_STD 1 00155 #define ZG_FIFO_RXD_STD 1 00156 00157 //Management 00158 00159 //Events 00160 #define ZG_FIFO_MGMT_DISASSOC 1 //Disassociated 00161 #define ZG_FIFO_MGMT_DEAUTH 2 //Deauthenticated 00162 #define ZG_FIFO_MGMT_CONN 4 //Connection state 00163 00164 //Commands 00165 #define ZG_FIFO_MGMT_SCAN 1 //Network scan 00166 00167 #define ZG_FIFO_MGMT_PSK_CALC 12 //Compute Pre-Shared Key 00168 00169 #define ZG_FIFO_MGMT_PMK_KEY 8 //Set Pairwise Master Key 00170 #define ZG_FIFO_MGMT_WEP_KEY 10 //Set WEP key 00171 00172 #define ZG_FIFO_MGMT_PARM_SET 15 //Set param 00173 #define ZG_FIFO_MGMT_PARM_GET 16 //Get param 00174 00175 #define ZG_FIFO_MGMT_ADHOC 18 //Start an adhoc connection 00176 #define ZG_FIFO_MGMT_CONNECT 19 00177 #define ZG_FIFO_MGMT_DISC 5 00178 00179 #define ZG_FIFO_MGMT_CONN_MGMT 20 //Manage connection 00180 00181 /* Params IDs for Get/Set Params */ 00182 00183 #define ZG_FIFO_MGMT_PARM_MACAD 1 //MAC Addr (6 bytes long) 00184 #define ZG_FIFO_MGMT_PARM_REGION 2 //Region (1 byte long) 00185 #define ZG_FIFO_MGMT_PARM_SYSV 26 //System version (2 bytes long) 00186 00187 /* Masks used by ZG_REG_INT* */ 00188 00189 #define ZG_INT_MASK_F0 0x40 00190 #define ZG_INT_MASK_F1 0x80 00191 00192 /* Internal Error Codes */ 00193 00194 typedef enum __ZG_INT_ERR 00195 { 00196 ZG_INT_OK = 1, 00197 ZG_INT_RESOURCES = 12, //Not enough resources 00198 ZG_INT_TIMEOUT, 00199 ZG_INT_FRAME_ERROR, 00200 ZG_INT_AUTH_REFUSED, 00201 ZG_INT_ASSOC_REFUSED, 00202 ZG_INT_IN_PROGRESS, 00203 ZG_INT_SUPPLICANT_FAILED = 21 00204 00205 } ZG_INT_ERR; 00206 00207 /* F0 / F1 helpers */ 00208 #define ZG_REG_F_CTRL0(n) ((n==0)?ZG_REG_F0_CTRL0:ZG_REG_F1_CTRL0) 00209 #define ZG_REG_F_CTRL1(n) ((n==0)?ZG_REG_F0_CTRL1:ZG_REG_F1_CTRL1) 00210 #define ZG_REG_F_INDEX(n) ((n==0)?ZG_REG_F0_INDEX:ZG_REG_F1_INDEX) 00211 #define ZG_REG_F_STATUS(n) ((n==0)?ZG_REG_F0_STATUS:ZG_REG_F1_STATUS) 00212 00213 #define ZG_REG_F_LEN(n) ((n==0)?ZG_REG_F0_LEN:ZG_REG_F1_LEN) 00214 00215 #define ZG_INT_MASK_F(n) ((n==0)?ZG_INT_MASK_F0:ZG_INT_MASK_F1) 00216 00217 /* Macro helpers (LE Platform, SPI is BE) */ 00218 00219 #define HTODS( x ) ( (((x)<<8) | ((x)>>8)) & 0xFFFF ) //Host to device, short 00220 #define DTOHS( x ) ( HTODS(x) ) //Device to host, short 00221 00222 #define HTODL( x ) ( ( ((x) & 0x000000FF) << 24 ) \ 00223 | ( ((x) & 0x0000FF00) << 8 ) \ 00224 | ( ((x) & 0x00FF0000) >> 8 ) \ 00225 | ( ((x) & 0xFF000000) >> 24 ) ) //Host to device, long 00226 #define DTOHL( x ) ( HTODL(x) ) 00227 00228 /* Platform Specific defs */ 00229 00230 #define ZG_MEM __attribute((section("AHBSRAM1"))) 00231 00232 /* Typedefs */ 00233 00234 typedef unsigned char byte; 00235 typedef unsigned short word; 00236 typedef unsigned int dword; 00237 00238 typedef signed char int8_t; 00239 typedef unsigned char uint8_t; 00240 00241 typedef signed short int16_t; 00242 typedef unsigned short uint16_t; 00243 00244 typedef signed int int32_t; 00245 typedef unsigned int uint32_t; 00246 00247 /* Data structures */ 00248 00249 typedef __packed struct __ZG_SYSV 00250 { 00251 byte rom; 00252 byte revision; 00253 } ZG_SYSV; 00254 00255 ///BSS types 00256 typedef enum __ZG_BSS_TYPE 00257 { 00258 ZG_BSS_INFRA = 1, ///< Infrastructure 00259 ZG_BSS_ADHOC = 2, ///< Ad-Hoc 00260 ZG_BSS_ANY = 3 ///< Either 00261 } ZG_BSS_TYPE; 00262 00263 typedef enum __ZG_PROBE_TYPE 00264 { 00265 ZG_PROBE_ACTIVE = 1, 00266 ZG_PROBE_PASSIVE = 2 00267 } ZG_PROBE_TYPE; 00268 00269 //Scan request 00270 typedef __packed struct __ZG_SCAN_REQ 00271 { 00272 word probe_delay; //In us 00273 word min_chan_time; //Min scan time on each channel in (1024 us) 00274 word max_chan_time; //Max scan time on each channel in (1024 us) 00275 byte bssid_mask[ZG_MACADDR_LEN]; 00276 ZG_BSS_TYPE bss_type; //Infra, adhoc or both 00277 ZG_PROBE_TYPE probe_req; //Send probe request frames 00278 byte ssid_len; 00279 byte channels_count; //Number 00280 char ssid[ZG_SSID_LEN]; //Scan for a specific network, 0 otherwise 00281 byte channels[ZG_SCAN_MAX_CHANNELS]; //Channels to scan 00282 } ZG_SCAN_REQ; 00283 00284 //Scan results header 00285 typedef __packed struct __ZG_SCAN_RES 00286 { 00287 byte result; 00288 byte state; 00289 byte last_channel; //Last channel scanned 00290 byte results_count; //Number of ZG_SCAN_ITEM following this header 00291 } ZG_SCAN_RES; 00292 00293 //Scan result 00294 //See: 00295 // - http://en.wikipedia.org/wiki/Beacon_frame 00296 // - IEEE 802.11-2007 7.2.3.1 Beacon frame format (p. 80 ~ 81) 00297 // - IEEE 802.11-2007 7.3 Management frame body components (p. 87 ~ 139) 00298 typedef __packed struct __ZG_SCAN_ITEM 00299 { 00300 byte bssid[ZG_MACADDR_LEN]; //This is actually a MAC address 00301 char ssid[ZG_SSID_LEN]; //SSID of the network 00302 word capability; 00303 word beacon_period; 00304 word atim_wdw; //http://en.wikipedia.org/wiki/Announcement_Traffic_Indication_Message 00305 byte supported_rates[ZG_MAX_SUPPORTED_RATES];//(0x80 | enc_rate) where enc_rate = (rate / 500Kbps) 00306 byte rssi; 00307 byte supported_rates_count; 00308 byte dtim_period; //http://en.wikipedia.org/wiki/Delivery_Traffic_Indication_Message 00309 ZG_BSS_TYPE bss_type; //Infra or adhoc 00310 byte channel; 00311 byte ssid_len; 00312 } ZG_SCAN_ITEM; 00313 00314 //WEP Key setup 00315 typedef __packed struct __ZG_WEP_REQ 00316 { 00317 byte slot; 00318 byte key_size; 00319 byte default_key; 00320 byte ssid_len; 00321 char ssid[ZG_SSID_LEN]; //SSID of the network 00322 byte keys[ZG_WEP_KEYS_COUNT][ZG_WEP_KEY_LEN]; 00323 } ZG_WEP_REQ; 00324 00325 //PSK Key computation 00326 typedef __packed struct __ZG_PSK_CALC_REQ 00327 { 00328 byte config; 00329 byte phrase_len; 00330 byte ssid_len; 00331 byte _pad; //Padding 00332 char ssid[ZG_SSID_LEN]; //SSID of the network 00333 char pass_phrase[ZG_WPA_PASS_LEN]; 00334 } ZG_PSK_CALC_REQ; 00335 00336 typedef __packed struct __ZG_PSK_CALC_RES 00337 { 00338 byte result; 00339 byte state; 00340 byte key_returned; 00341 byte _pad; //Padding 00342 byte key[ZG_PMK_LEN]; //PSK key returned 00343 } ZG_PSK_CALC_RES; 00344 00345 typedef __packed struct __ZG_PMK_REQ 00346 { 00347 byte slot; 00348 byte ssid_len; 00349 char ssid[ZG_SSID_LEN]; //SSID of the network 00350 byte key[ZG_PMK_LEN]; //PSK key returned 00351 } ZG_PMK_REQ; 00352 00353 ///Security type 00354 typedef enum __ZG_SECURITY 00355 { 00356 ZG_SECURITY_NONE = 0x00, ///< None 00357 ZG_SECURITY_WEP = 0x01, ///< WEP 00358 ZG_SECURITY_WPA = 0x02, ///< WPA 00359 ZG_SECURITY_WPA2 = 0x03, ///< WPA2 00360 ZG_SECURITY_TRY = 0xFF ///< Try all (not recommanded) 00361 } ZG_SECURITY; 00362 00363 typedef __packed struct __ZG_CONNECT_REQ 00364 { 00365 ZG_SECURITY security; 00366 byte ssid_len; 00367 char ssid[ZG_SSID_LEN]; //SSID of the network 00368 word sleep_duration; //Power save sleep duration (/100 ms) 00369 ZG_BSS_TYPE bss_type; 00370 byte _pad; //Padding 00371 } ZG_CONNECT_REQ; 00372 00373 typedef __packed struct __ZG_DISCONNECT_REQ 00374 { 00375 word reason_code; 00376 bool disconnect; 00377 bool deauth_frame; 00378 } ZG_DISCONNECT_REQ; 00379 00380 //Ethernet / ZG Packet headers 00381 typedef __packed struct __ZG_ETH_HDR //Ethernet packet header 00382 { 00383 byte dest[ZG_MACADDR_LEN]; 00384 byte src[ZG_MACADDR_LEN]; 00385 word type; 00386 } ZG_ETH_HDR; 00387 00388 typedef __packed struct __ZG_RX_HDR //ZG packet header on rx 00389 { 00390 word rssi; 00391 byte dest[ZG_MACADDR_LEN]; 00392 byte src[ZG_MACADDR_LEN]; 00393 dword arrival_time; 00394 word data_len; 00395 byte snap[ZG_SNAP_LEN]; //SNAP word, see zg_net 00396 word type; //Ethernet type 00397 } ZG_RX_HDR; 00398 00399 typedef __packed struct __ZG_TX_HDR //ZG packet header on tx 00400 { 00401 word zero; //Must be set to zero 00402 byte dest[ZG_MACADDR_LEN]; 00403 byte snap[ZG_SNAP_LEN]; //SNAP word, see zg_net 00404 word type; //Ethernet type 00405 } ZG_TX_HDR; 00406 00407 #endif 00408
Generated on Tue Jul 12 2022 13:17:38 by
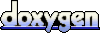