
Test of USSD commands transmission over the Vodafone network with the Vodafone library
Dependencies: VodafoneUSBModem mbed-rtos mbed
Fork of VodafoneK3770USSDTestBeta by
main.cpp
00001 #include "mbed.h" 00002 #include "VodafoneUSBModem.h" 00003 00004 #define USSD_COMMAND "*#134#" 00005 00006 void test(void const*) 00007 { 00008 VodafoneUSBModem modem; 00009 char result[32]; 00010 00011 printf("Sending %s on USSD channel\n", USSD_COMMAND); 00012 00013 int ret = modem.sendUSSD(USSD_COMMAND, result, 32); 00014 if(ret) 00015 { 00016 printf("Send USSD command returned %d\n", ret); 00017 } 00018 00019 printf("Result of command: %s\n", result); 00020 00021 00022 while(1) { 00023 } 00024 } 00025 00026 00027 int main() 00028 { 00029 Thread testTask(test, NULL, osPriorityNormal, 1024 * 4); 00030 DigitalOut led(LED1); 00031 while(1) 00032 { 00033 led=!led; 00034 Thread::wait(1000); 00035 } 00036 00037 return 0; 00038 }
Generated on Tue Jul 19 2022 07:55:58 by
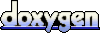