
Dependencies: rtos_net_beta VodafoneK3770LibBeta mbed
main.cpp
00001 /* net_3g_basic_http_test.cpp */ 00002 /* 00003 Copyright (C) 2012 ARM Limited. 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy of 00006 this software and associated documentation files (the "Software"), to deal in 00007 the Software without restriction, including without limitation the rights to 00008 use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies 00009 of the Software, and to permit persons to whom the Software is furnished to do 00010 so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in all 00013 copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00021 SOFTWARE. 00022 */ 00023 00024 #define __DEBUG__ 4 //Maximum verbosity 00025 #ifndef __MODULE__ 00026 #define __MODULE__ "net_3g_basic_http_test.cpp" 00027 #endif 00028 00029 #include "core/fwk.h" 00030 #include "mbed.h" 00031 00032 #include "rtos.h" 00033 00034 #include "if/VodafoneK3770.h" 00035 #include "HTTPClient.h" 00036 00037 DigitalOut led1(LED1); 00038 DigitalOut led2(LED2); 00039 DigitalOut led3(LED3); 00040 DigitalOut led4(LED4); 00041 void notify(bool a, bool b, bool c) 00042 { 00043 led1 = a; 00044 led2 = b; 00045 led3 = c; 00046 } 00047 00048 extern "C" void HardFault_Handler() 00049 { 00050 error("Hard Fault!\n"); 00051 } 00052 00053 void test(void const*) 00054 { 00055 VodafoneK3770 threeg; 00056 HTTPClient http; 00057 char str[512]; 00058 00059 DBG("Hello!"); 00060 00061 int count = 0; 00062 00063 start: 00064 count++; 00065 DBG("iteration #%d", count); 00066 00067 notify(0, 1, 1); 00068 //int ret = threeg.connect("pp.vodafone.co.uk"); 00069 int ret = threeg.connect("SMART"); 00070 notify(0, 1, 0); 00071 if (ret == OK) 00072 { 00073 DBG("Trying to fetch page..."); 00074 ret = http.get("http://mbed.org/media/uploads/donatien/hello.txt", str, 128); 00075 if (ret == OK) 00076 { 00077 DBG("Page fetched successfully - read %d characters", strlen(str)); 00078 DBG("Result: %s", str); 00079 } 00080 else 00081 { 00082 WARN("Error - ret = %d - HTTP return code = %d", ret, http.getHTTPResponseCode()); 00083 } 00084 00085 HTTPMap map; 00086 HTTPText text(str, 512); 00087 map.put("Hello", "World"); 00088 map.put("test", "1234"); 00089 DBG("Trying to post data..."); 00090 ret = http.post("http://httpbin.org/post", map, &text); 00091 if (ret == OK) 00092 { 00093 DBG("Executed POST successfully - read %d characters", strlen(str)); 00094 DBG("Result: %s", str); 00095 } 00096 else 00097 { 00098 WARN("Error - ret = %d - HTTP return code = %d", ret, http.getHTTPResponseCode()); 00099 } 00100 } 00101 threeg.disconnect(); 00102 DBG("Disconnected"); 00103 00104 notify(1, 1, 1); 00105 00106 goto start; 00107 00108 while (1) 00109 { 00110 Thread::wait(100); 00111 } 00112 } 00113 00114 void keepAlive(void const*) 00115 { 00116 while (1) 00117 { 00118 led1 = !led1; 00119 Thread::wait(500); 00120 } 00121 } 00122 00123 void tick() 00124 { 00125 led4 = !led4; 00126 } 00127 00128 int main() 00129 { 00130 Ticker t; 00131 t.attach(tick, 1); 00132 DBG_INIT(); 00133 00134 notify(1, 0, 0); 00135 00136 Thread testTask(test, NULL, osPriorityNormal, 1024 * 4); 00137 keepAlive(NULL); 00138 00139 return 0; 00140 }
Generated on Wed Jul 13 2022 02:33:09 by
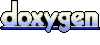