
UDP Sockets use example
Dependencies: EthernetNetIf mbed
UDPSocketExample.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "UDPSocket.h" 00004 00005 EthernetNetIf eth; 00006 UDPSocket udp; 00007 00008 void onUDPSocketEvent(UDPSocketEvent e) 00009 { 00010 switch(e) 00011 { 00012 case UDPSOCKET_READABLE: //The only event for now 00013 char buf[64] = {0}; 00014 Host host; 00015 while( int len = udp.recvfrom( buf, 63, &host ) ) 00016 { 00017 if( len <= 0 ) 00018 break; 00019 printf("From %d.%d.%d.%d: %s\n", host.getIp()[0], host.getIp()[1], host.getIp()[2], host.getIp()[3], buf); 00020 } 00021 break; 00022 } 00023 } 00024 00025 int main() { 00026 printf("Setting up...\n"); 00027 EthernetErr ethErr = eth.setup(); 00028 if(ethErr) 00029 { 00030 printf("Error %d in setup.\n", ethErr); 00031 return -1; 00032 } 00033 printf("Setup OK\n"); 00034 00035 Host multicast(IpAddr(239, 192, 1, 100), 50000, NULL); //Join multicast group on port 50000 00036 00037 udp.setOnEvent(&onUDPSocketEvent); 00038 00039 udp.bind(multicast); 00040 00041 Timer tmr; 00042 tmr.start(); 00043 while(true) 00044 { 00045 Net::poll(); 00046 if(tmr.read() > 5) 00047 { 00048 tmr.reset(); 00049 const char* str = "Hello world!"; 00050 udp.sendto( str, strlen(str), &multicast ); 00051 printf("%s\n", str); 00052 } 00053 } 00054 00055 00056 }
Generated on Wed Jul 13 2022 00:34:10 by
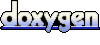