
Websocket demo using the Sprint/Sierra Wireless 598U dongle
Dependencies: SprintUSBModem WebSocketClient mbed-rtos mbed
main.cpp
00001 #include "mbed.h" 00002 #include "SprintUSBModem.h" 00003 #include "Websocket.h" 00004 00005 void test(void const*) 00006 { 00007 SprintUSBModem modem(p18); 00008 Websocket ws("ws://sockets.mbed.org:443/ws/demo/rw"); 00009 char recv[128]; 00010 00011 Thread::wait(5000); 00012 printf("Switching power on\r\n"); 00013 00014 modem.power(true); 00015 00016 int ret = modem.connect(); 00017 if(ret) 00018 { 00019 printf("Could not connect\r\n"); 00020 return; 00021 } 00022 00023 bool c = ws.connect(); 00024 printf("Connect result: %s\r\n", c?"OK":"Failed"); 00025 00026 for(int i = 0; i < 10000; i++) 00027 { 00028 if(!(i%100)) 00029 { 00030 int ret = ws.send("WebSocket Hello World!"); 00031 if(ret<0) 00032 { 00033 printf("Timeout\r\n"); 00034 ws.close(); 00035 c = ws.connect(); 00036 printf("Connect result: %s\r\n", c?"OK":"Failed"); 00037 } 00038 } 00039 00040 if (ws.read(recv)) { 00041 printf("rcv: %s\r\n", recv); 00042 } 00043 00044 } 00045 00046 modem.disconnect(); 00047 00048 printf("Disconnected\r\n"); 00049 00050 modem.power(false); 00051 00052 printf("Powered off\r\n"); 00053 00054 while(1) { 00055 } 00056 } 00057 00058 00059 int main() 00060 { 00061 DBG_INIT(); 00062 DBG_SET_SPEED(115200); 00063 DBG_SET_NEWLINE("\r\n"); 00064 Thread testTask(test, NULL, osPriorityNormal, 1024 * 5); 00065 DigitalOut led(LED1); 00066 while(1) 00067 { 00068 led=!led; 00069 Thread::wait(1000); 00070 } 00071 00072 return 0; 00073 }
Generated on Thu Jul 14 2022 16:12:20 by
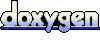