Dependents: SimpleLCDClock readCard2Twitter_http AnalogClock_StepperMotor_NTP ServoCamV1
MySQLClient.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef MYSQL_CLIENT_H 00025 #define MYSQL_CLIENT_H 00026 00027 #include "if/net/net.h" 00028 #include "api/TcpSocket.h" 00029 #include "api/DnsRequest.h" 00030 #include "mbed.h" 00031 00032 #include <string> 00033 using std::string; 00034 00035 #include <map> 00036 using std::map; 00037 00038 #define MYSQL_TIMEOUT_MS 15000 00039 #define MYSQL_PORT 3306 00040 00041 typedef unsigned char byte; 00042 00043 enum MySQLResult 00044 { 00045 MYSQL_OK, 00046 MYSQL_PROCESSING, 00047 MYSQL_PRTCL, 00048 MYSQL_SETUP, //Not properly configured 00049 MYSQL_DNS, //Could not resolve name 00050 MYSQL_AUTHFAILED, //Auth failure 00051 MYSQL_READY, //Ready to send commands 00052 MYSQL_SQL, //SQL Error 00053 MYSQL_TIMEOUT, //Connection timeout 00054 MYSQL_CONN //Connection error 00055 }; 00056 00057 class MySQLClient : protected NetService 00058 { 00059 public: 00060 MySQLClient(); 00061 virtual ~MySQLClient(); 00062 00063 //High Level setup functions 00064 MySQLResult open(Host& host, const string& user, const string& password, const string& db, void (*pMethod)(MySQLResult)); //Non blocking 00065 template<class T> 00066 MySQLResult open(Host& host, const string& user, const string& password, const string& db, T* pItem, void (T::*pMethod)(MySQLResult)) //Non blocking 00067 { 00068 setOnResult(pItem, pMethod); 00069 setup(host, user, password, db); 00070 return MYSQL_PROCESSING; 00071 } 00072 00073 MySQLResult sql(string& sqlCommand); 00074 00075 MySQLResult exit(); 00076 00077 void setOnResult( void (*pMethod)(MySQLResult) ); 00078 class CDummy; 00079 template<class T> 00080 void setOnResult( T* pItem, void (T::*pMethod)(MySQLResult) ) 00081 { 00082 m_pCb = NULL; 00083 m_pCbItem = (CDummy*) pItem; 00084 m_pCbMeth = (void (CDummy::*)(MySQLResult)) pMethod; 00085 } 00086 00087 void setTimeout(int ms); 00088 00089 virtual void poll(); //Called by NetServices 00090 00091 protected: 00092 void resetTimeout(); 00093 00094 void init(); 00095 void close(); 00096 00097 void setup(Host& host, const string& user, const string& password, const string& db); //Setup connection, make DNS Req if necessary 00098 void connect(); //Start Connection 00099 00100 void handleHandshake(); 00101 void sendAuth(); 00102 00103 void handleAuthResult(); 00104 00105 void sendCommand(byte command, byte* arg, int len); 00106 void handleCommandResult(); 00107 00108 void readData(); //Copy to buf 00109 void writeData(); //Copy from buf 00110 00111 void onTcpSocketEvent(TcpSocketEvent e); 00112 void onDnsReply(DnsReply r); 00113 void onResult(MySQLResult r); //Called when exchange completed or on failure 00114 void onTimeout(); //Connection has timed out 00115 00116 private: 00117 CDummy* m_pCbItem; 00118 void (CDummy::*m_pCbMeth)(MySQLResult); 00119 00120 void (*m_pCb)(MySQLResult); 00121 00122 TcpSocket* m_pTcpSocket; 00123 00124 Timer m_watchdog; 00125 int m_timeout; 00126 00127 DnsRequest* m_pDnsReq; 00128 00129 bool m_closed; 00130 00131 enum MySQLStep 00132 { 00133 // MYSQL_INIT, 00134 MYSQL_HANDSHAKE, 00135 MYSQL_AUTH, 00136 MYSQL_COMMANDS, 00137 MYSQL_CLOSED 00138 }; 00139 00140 //Parameters 00141 Host m_host; 00142 00143 string m_user; 00144 string m_password; 00145 string m_db; 00146 00147 //Low-level buffers & state-machine 00148 MySQLStep m_state; 00149 00150 byte* m_buf; 00151 byte* m_pPos; 00152 int m_len; 00153 int m_size; 00154 00155 int m_packetId; 00156 00157 }; 00158 00159 #endif
Generated on Tue Jul 12 2022 22:47:25 by
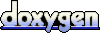