
A sprinkler controller that takes HTTP command for zone and duration.
Dependencies: EthernetNetIf mbed HTTPServer
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "HTTPServer.h" 00004 #include "sprinkler_handler.h" 00005 #include "share.h" 00006 #include "NTPClient.h" 00007 00008 NTPClient ntp; 00009 00010 DigitalOut led1(LED1, "led1"); 00011 DigitalOut led2(LED2, "led2"); 00012 DigitalOut led3(LED3, "led3"); 00013 DigitalOut led4(LED4, "led4"); 00014 DigitalOut ledgreen(p29); 00015 DigitalOut ledyellow(p30); 00016 00017 LocalFileSystem fs("webfs"); 00018 00019 EthernetNetIf eth; 00020 HTTPServer svr; 00021 Timer activity_timer; 00022 00023 DigitalIn bypass(p16); 00024 DigitalIn zone1in(p17); 00025 DigitalIn zone2in(p18); 00026 DigitalIn zone3in(p19); 00027 DigitalIn zone4in(p20); 00028 00029 DigitalOut zone1out(p24); 00030 DigitalOut zone2out(p23); 00031 DigitalOut zone3out(p22); 00032 DigitalOut zone4out(p21); 00033 00034 int compute1out = 0; 00035 int compute2out = 0; 00036 int compute3out = 0; 00037 int compute4out = 0; 00038 00039 void lanActivity( void ) { 00040 activity_timer.start(); 00041 ledgreen = 0; 00042 } 00043 00044 int main() { 00045 //Base::add_rpc_class<DigitalOut>(); 00046 ledyellow = 0; 00047 ledgreen = 0; 00048 activity_timer.reset(); 00049 00050 printf("Setting up...\n"); 00051 EthernetErr ethErr = eth.setup(); 00052 if(ethErr) { 00053 printf("Error %d in setup.\n", ethErr); 00054 return -1; 00055 } else { 00056 ledgreen = 1; 00057 } 00058 00059 printf("Setup OK\n"); 00060 00061 FSHandler::mount("/webfs", "/files"); //Mount /webfs path on /files web path 00062 FSHandler::mount("/webfs", "/"); //Mount /webfs path on web root path 00063 00064 svr.addHandler<SimpleHandler>("/check"); 00065 // the main url with be http://a.b.c.d/sprinkler 00066 // a GET request will return current status 00067 // a POST with parameters of zone<n> and duration in seconds will set it 00068 svr.addHandler<SprinklerHandler>("/sprinkler"); 00069 svr.addHandler<RPCHandler>("/rpc"); 00070 svr.addHandler<FSHandler>("/files"); 00071 svr.addHandler<FSHandler>("/"); //Default handler 00072 //Example : Access to mbed.htm : http://a.b.c.d/mbed.htm or http://a.b.c.d/files/mbed.htm 00073 00074 svr.bind(80); 00075 00076 printf("Listening...\n"); 00077 00078 Timer tm; 00079 int timesync = 0; 00080 tm.start(); 00081 time_t initialtime = time(NULL); 00082 printf("Time as a basic string = %s\r", ctime(&initialtime)); 00083 //Listen indefinitely 00084 while(true) { 00085 //wait(0.01); 00086 Net::poll(); 00087 if(tm.read()>0.5) { 00088 ledyellow = !ledyellow; //Show that we are alive 00089 tm.start(); 00090 timesync++; 00091 } 00092 if(activity_timer > 0.2) { 00093 ledgreen = 1; 00094 } 00095 00096 if (timesync > 3600 * 2) { // every hour 00097 printf( "Setting time...\r\n" ); 00098 Host server2(IpAddr(), 123, "pool.ntp.org"); 00099 ntp.setTime(server2); 00100 time_t seconds = time(NULL); 00101 printf("Time as a basic string = %s\r", ctime(&seconds)); 00102 timesync = 0; 00103 } 00104 00105 if (bypass) { // ignore all other choices other than inputs 00106 led1 = zone1out = zone1in; 00107 led2 = zone2out = zone2in; 00108 led3 = zone3out = zone3in; 00109 led4 = zone4out = zone4in; 00110 } else { // take computed outputs 00111 led1 = zone1out = compute1out; 00112 led2 = zone2out = compute2out; 00113 led3 = zone3out = compute3out; 00114 led4 = zone4out = compute4out; 00115 } 00116 } 00117 } 00118
Generated on Fri Jul 15 2022 01:20:29 by
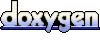