Simple BLE Radio tester and signal strength (RSSI) meter, using microbit radio lib.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 00027 /* 00028 * This is a simple example that uses the micro:bit radio to show if another 00029 * micro:bit is nearby broadcasting with the same group number. 00030 * The display will show the received signal strength in -dBm. 00031 * 00032 */ 00033 00034 #include "config.h" 00035 #include "MicroBit.h" 00036 00037 #if MICROBIT_BLE_ENABLED 00038 #ifdef YOTTA_CFG 00039 #error "This example needs BLE to be disabled. Use the yotta config.json in the proximit-heart directory to do this" 00040 #else 00041 #error "This example needs BLE to be disabled in the microbit config file in the microbit-dal: MicroBitConfig.h" 00042 #endif 00043 #endif 00044 00045 #ifdef USE_MICROBIT_ELEMENTS 00046 00047 // Used microbit components. 00048 00049 MicroBitDisplay display; 00050 MicroBitRadio radio; 00051 MicroBitMessageBus messageBus; 00052 00053 #else 00054 00055 // Complete microbit object. 00056 00057 MicroBit uBit; 00058 00059 #endif 00060 00061 static int display = 0, receiving = 0, direction = 1; 00062 static uint8_t radioRSSI = 98, radioGroup = radioGroupNumber; 00063 ManagedString rxdata; 00064 void onData(MicroBitEvent) 00065 { 00066 rxdata = uBit.radio.datagram.recv(); 00067 receiving = 3; 00068 radioRSSI = uBit.radio.getRSSI(); 00069 display = 1; 00070 } 00071 00072 void onButtonA(MicroBitEvent) 00073 { 00074 if ( radioGroup != radioGroupNumber ) 00075 { 00076 radioGroup = radioGroupNumber; 00077 uBit.radio.setGroup(radioGroup); 00078 } 00079 direction = -direction; 00080 display = 2; 00081 } 00082 00083 void onButtonAlong(MicroBitEvent) 00084 { 00085 display = 3; 00086 } 00087 00088 void onButtonAhold(MicroBitEvent) 00089 { 00090 display = 3; 00091 } 00092 00093 void onButtonAdouble(MicroBitEvent) 00094 { 00095 display = 3; 00096 } 00097 00098 void onButtonBlong(MicroBitEvent) 00099 { 00100 display = 4; 00101 } 00102 00103 void onButtonB(MicroBitEvent) 00104 { 00105 radioGroup += direction; 00106 uBit.radio.setGroup(radioGroup); 00107 display = 2; 00108 } 00109 00110 int main() 00111 { 00112 int sleeping; 00113 00114 // Initialise the micro:bit runtime. 00115 uBit.init(); 00116 uBit.radio.setGroup(radioGroupNumber); 00117 //Setup a handler to run when data is received. 00118 uBit.messageBus.listen(MICROBIT_ID_RADIO, MICROBIT_RADIO_EVT_DATAGRAM, onData); 00119 // Setup some button handlers to allow extra control with buttons. 00120 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_CLICK, onButtonA); 00121 uBit.messageBus.listen(MICROBIT_ID_BUTTON_B, MICROBIT_BUTTON_EVT_CLICK, onButtonB); 00122 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_LONG_CLICK, onButtonAlong); 00123 uBit.messageBus.listen(MICROBIT_ID_BUTTON_B, MICROBIT_BUTTON_EVT_LONG_CLICK, onButtonBlong); 00124 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_HOLD, onButtonAhold); 00125 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_DOUBLE_CLICK, onButtonAdouble); 00126 uBit.radio.enable(); 00127 00128 while ( true ) 00129 { 00130 switch ( display ) 00131 { 00132 default: 00133 case 0: // nothing to display? 00134 uBit.display.print("?"); 00135 sleeping = 1000; 00136 break; 00137 00138 case 1: // display signal strength. 00139 uBit.display.scroll(radioRSSI); 00140 sleeping = 500; 00141 break; 00142 00143 case 2: // display group number. 00144 uBit.display.print("#", 300); 00145 uBit.display.scroll(radioGroup); 00146 sleeping = 500; 00147 break; 00148 00149 case 3: // display received data. 00150 uBit.display.scroll(rxdata); 00151 sleeping = 500; 00152 break; 00153 } 00154 00155 if ( receiving ) 00156 { 00157 receiving -= 1; 00158 display = receiving && display == 1 ? 1 : 0; 00159 } 00160 uBit.sleep(sleeping); 00161 } 00162 }
Generated on Tue Jul 12 2022 21:03:26 by
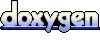