
Custom phone app that controls cup dispenser, drink dispenser, and conveyor belt using the mbed blue tooth capabilities.
Dependencies: Motor Servo mbed
Fork of 4180_WDDDS by
main.cpp
00001 // Demonstrates complete use of the WDDDS systems and functionalities: 00002 // Cup dispenser, dispensing drink 1, dispensing drink 2, and conveyor belt. 00003 // This code: 00004 // - dispenses a cup, moves the cup under the drink dispenser, dispenses drink 1 00005 // then moves cup further along treads when button 1 is pressed 00006 // - dispenses a cup, moves the cup under the drink dispenser, dispenses drink 2 00007 // then moves cup further along treads when button 2 is pressed 00008 // - 4th button is used for unjamming the cup dispenser, as it will extend the 00009 // linear actuator further to push cups out. 00010 00011 #include "mbed.h" 00012 #include "Motor.h" 00013 #include "Servo.h" 00014 00015 Servo poker(p22); 00016 DigitalIn stby(p25); 00017 Motor tread(p24, p12, p11); // pwm, fwd, rev (H-bridge) 00018 Motor pump(p26, p13, p14); // pwm, fwd, rev (H-bridge) 00019 DigitalOut tread2(p21); // MOSFET 00020 BusOut myled(LED1,LED2,LED3,LED4); // check for which button is pressed 00021 Serial blue(p28,p27); // Bluetooth 00022 float cupos = 850; 00023 void cupdispense(); 00024 void drink1(); 00025 void drink2(); 00026 void treads(float time); 00027 00028 int main() 00029 { 00030 poker.Enable(1500,20000); 00031 poker.SetPosition(cupos); 00032 wait(.2); 00033 poker.SetPosition(cupos+10); 00034 stby.mode(PullUp); 00035 char bnum=0; 00036 char bhit=0; 00037 while(1) { 00038 if (blue.getc()=='!') { 00039 if (blue.getc()=='B') { //button data packet 00040 bnum = blue.getc(); //button number 00041 bhit = blue.getc(); //1=hit, 0=release 00042 if (blue.getc()==char(~('!' + 'B' + bnum + bhit))) { //checksum OK? 00043 myled = bnum - '0'; //current button number will appear on LEDs 00044 switch (bnum) { 00045 case '1': //number button 1 00046 if (bhit=='1') { 00047 //add hit code here 00048 drink1(); 00049 00050 } else { 00051 //add release code here 00052 } 00053 break; 00054 case '2': //number button 2 00055 if (bhit=='1') { 00056 //add hit code here 00057 drink2(); 00058 } else { 00059 //add release code here 00060 } 00061 break; 00062 case '3': //number button 3 00063 if (bhit=='1') { 00064 //add hit code here 00065 } else { 00066 //add release code here 00067 } 00068 break; 00069 case '4': //number button 4 00070 if (bhit=='1') { 00071 //add hit code here 00072 cupdispense(); 00073 } else { 00074 //add release code here 00075 } 00076 break; 00077 default: 00078 break; 00079 } 00080 } 00081 } 00082 } 00083 } 00084 } 00085 00086 void cupdispense(){ 00087 if( cupos <2500) { 00088 cupos += 52; 00089 wait_ms(400); 00090 poker.SetPosition(cupos); 00091 cupos += 53; 00092 poker.SetPosition(cupos); 00093 wait_ms(100); 00094 } 00095 } 00096 00097 void drink1(){ 00098 // cupdispense(); 00099 //wait(3.5); 00100 treads(.68); 00101 wait(.4); 00102 pump.speed(1); 00103 wait(2); 00104 pump.speed(0); 00105 wait(5); 00106 treads(1); 00107 } 00108 00109 void drink2(){ 00110 // cupdispense(); 00111 //wait(3.5); 00112 treads(.68); 00113 wait(.4); 00114 pump.speed(-1); 00115 wait(2); 00116 pump.speed(0); 00117 wait(5); 00118 treads(1); 00119 } 00120 00121 void treads(float time) { 00122 tread.speed(.4); 00123 tread2 = 1; 00124 wait(time); 00125 tread.speed(0); 00126 tread2 = 0; 00127 }
Generated on Sun Jul 24 2022 11:23:34 by
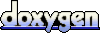