
This performs an ethernet sniff test. Copied from an existing ethernet sniff test and updated to work properly with the LISA, supporting output of content to the USB console. See also: http://mbed.org/users/rolf/code/ethsnif/
Fork of ethsnif by
main.cpp
00001 #include "mbed.h" 00002 #include "C027.h" 00003 #include "Ethernet.h" 00004 #include "hexview.h" 00005 00006 using namespace mbed; 00007 00008 DigitalOut led4(LED); 00009 00010 Ethernet eth; 00011 Serial pc(USBTX, USBRX); 00012 00013 unsigned short htons(unsigned short n) { // Host short to network shor 00014 return ((n & 0xff) << 8) | ((n & 0xff00) >> 8); // Byte swapping 00015 } 00016 00017 void show(char *buf, int size) { 00018 pc.printf("Destination: %02hx:%02hx:%02hx:%02hx:%02hx:%02hx\n\r", 00019 buf[0], buf[1], buf[2], buf[3], buf[4], buf[5]); 00020 pc.printf("Source: %02hx:%02hx:%02hx:%02hx:%02hx:%02hx\n\r", 00021 buf[6], buf[7], buf[8], buf[9], buf[10], buf[11]); 00022 00023 pc.printf("Type %hd\n\r", htons((short)buf[12])); 00024 00025 hexview(buf, size); 00026 } 00027 00028 00029 int main() { 00030 char buffer[0x600]; 00031 int size = 0; 00032 int led_toggle_count = 5; 00033 00034 #if 0 00035 while(1) { 00036 led4 = !led4; 00037 wait(0.2); 00038 } 00039 #else 00040 while( led_toggle_count-- > 0 ) 00041 { 00042 led4 = !led4; 00043 wait(0.2); 00044 } 00045 #endif 00046 00047 // open the PC serial port and (use the same baudrate) 00048 pc.baud(MDMBAUD); 00049 00050 pc.printf( "Start ETHERNET Sniff Testing\n\r"); 00051 00052 while(1) { 00053 if((size = eth.receive()) != 0) { 00054 led4=!led4; 00055 eth.read(buffer, size); 00056 show(buffer, size); 00057 } 00058 } 00059 }
Generated on Thu Jul 21 2022 17:18:25 by
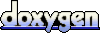