
This tests the CAN interface between two LISA C027 MBED Boards. By connecting the two boards, pins 1,2,3 respectively on the CAN interface, and loading and executing this code on BOTH boards, CAN messages will be received, and printed, on the PC-USB console interface of both devices.
Dependencies: C027-REVB UbloxUSBModem mbed
Fork of C027_ModemTransparentUSBCDC_revb by
main.cpp
00001 #include "mbed.h" 00002 #include "C027.h" 00003 00004 Ticker ticker; 00005 DigitalOut led1(LED); 00006 DigitalOut led2(LED1); 00007 CAN can1(CANRD, CANTD); 00008 Serial pc(USBTX, USBRX); 00009 00010 char counter = 0; 00011 00012 // this function creates a CAN message with a counter in it 00013 // and sends it on the CAN interface 00014 void send() { 00015 if(can1.write(CANMessage(1337, &counter, 1))) { 00016 pc.printf("Message sent: %d\r\n", counter); 00017 counter++; 00018 } 00019 led1 = !led1; 00020 } 00021 00022 int main() { 00023 00024 DigitalOut can_standby(CANS); 00025 00026 // enable the CAN interface 00027 // take it out of standby 00028 can_standby = 0; 00029 00030 // specify the baud rate for communications 00031 // with the serial console to the PC host. 00032 pc.baud(MDMBAUD); 00033 00034 pc.printf( "Starting CAN Testing\r\n"); 00035 00036 CANMessage msg; 00037 while(1) { 00038 00039 if(can1.read(msg)) { 00040 pc.printf("Message received: %d\r\n", msg.data[0]); 00041 led2 = !led2; 00042 } 00043 00044 wait(0.2); 00045 00046 // send a new message. 00047 send(); 00048 } 00049 } 00050 00051
Generated on Mon Aug 8 2022 20:46:36 by
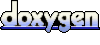