Driver Library for our displays
Dependents: dm_bubbles dm_calc dm_paint dm_sdcard_with_adapter ... more
DmTftRa8875.cpp
00001 /********************************************************************************************** 00002 Copyright (c) 2014 DisplayModule. All rights reserved. 00003 00004 Redistribution and use of this source code, part of this source code or any compiled binary 00005 based on this source code is permitted as long as the above copyright notice and following 00006 disclaimer is retained. 00007 00008 DISCLAIMER: 00009 THIS SOFTWARE IS SUPPLIED "AS IS" WITHOUT ANY WARRANTIES AND SUPPORT. DISPLAYMODULE ASSUMES 00010 NO RESPONSIBILITY OR LIABILITY FOR THE USE OF THE SOFTWARE. 00011 ********************************************************************************************/ 00012 00013 /* Notice: 00014 The panel resolution should be config in DmTftRa8875::init() function. 00015 RA8875Size size = RA8875_480x272 or RA8875Size size = RA8875_800x480; 00016 00017 Tested on NUCLEO-F401RE, LPCXpresso11U68, LPCXpresso824-MAX platform. 00018 */ 00019 00020 #include "DmTftRa8875.h" 00021 #if defined (DM_TOOLCHAIN_ARDUINO) 00022 DmTftRa8875::DmTftRa8875(uint8_t cs, uint8_t sel) 00023 : DmTftBase(480, 272) 00024 #elif defined (DM_TOOLCHAIN_MBED) 00025 DmTftRa8875::DmTftRa8875(PinName cs, PinName sel, PinName mosi, PinName miso, PinName clk) 00026 : DmTftBase(480, 272), spi(mosi, miso, clk) 00027 #endif 00028 { 00029 _cs = cs; 00030 _sel = sel; 00031 } 00032 00033 DmTftRa8875::~DmTftRa8875() 00034 { 00035 #if defined (DM_TOOLCHAIN_MBED) 00036 delete _pinCS; 00037 delete _pinSEL; 00038 00039 _pinCS = NULL; 00040 _pinSEL = NULL; 00041 #endif 00042 } 00043 00044 uint16_t DmTftRa8875::width(void) 00045 { 00046 return _width; 00047 } 00048 00049 uint16_t DmTftRa8875::height(void) 00050 { 00051 return _height; 00052 } 00053 00054 void DmTftRa8875::writeBus(uint8_t data) 00055 { 00056 #if defined (DM_TOOLCHAIN_ARDUINO) 00057 //SPCR = _spiSettings; // SPI Control Register 00058 SPDR = data; // SPI Data Register 00059 while(!(SPSR & _BV(SPIF))); // SPI Status Register Wait for transmission to finish 00060 #elif defined (DM_TOOLCHAIN_MBED) 00061 spi.write(data); 00062 #endif 00063 } 00064 00065 uint8_t DmTftRa8875::readBus(void) 00066 { 00067 #if defined (DM_TOOLCHAIN_ARDUINO) 00068 //SPCR = _spiSettings; // SPI Control Register 00069 SPDR = 0; // SPI Data Register 00070 while(!(SPSR & _BV(SPIF))); // SPI Status Register Wait for transmission to finish 00071 return SPDR; 00072 #elif defined (DM_TOOLCHAIN_MBED) 00073 return spi.write(0x00); // dummy byte to read 00074 #endif 00075 } 00076 00077 void DmTftRa8875::sendCommand(uint8_t index) 00078 { 00079 cbi(_pinCS, _bitmaskCS); 00080 00081 writeBus(0x80); 00082 writeBus(index); 00083 00084 sbi(_pinCS, _bitmaskCS); 00085 } 00086 00087 uint8_t DmTftRa8875::readStatus(void) 00088 { 00089 cbi(_pinCS, _bitmaskCS); 00090 00091 writeBus(0xC0); 00092 uint8_t data = readBus(); 00093 00094 sbi(_pinCS, _bitmaskCS); 00095 00096 return data; 00097 } 00098 00099 void DmTftRa8875::sendData(uint16_t data) 00100 { 00101 00102 uint8_t dh = data>>8; 00103 uint8_t dl = data&0xff; 00104 00105 cbi(_pinCS, _bitmaskCS); 00106 00107 writeBus(0x00); 00108 writeBus(dh); 00109 writeBus(dl); 00110 00111 sbi(_pinCS, _bitmaskCS); 00112 00113 } 00114 00115 void DmTftRa8875::send8BitData(uint8_t data) 00116 { 00117 cbi(_pinCS, _bitmaskCS); 00118 00119 writeBus(0x00); 00120 writeBus(data); 00121 00122 sbi(_pinCS, _bitmaskCS); 00123 } 00124 00125 uint8_t DmTftRa8875::readData(void) 00126 { 00127 cbi(_pinCS, _bitmaskCS); 00128 00129 writeBus(0x40); 00130 uint8_t data = readBus(); 00131 00132 sbi(_pinCS, _bitmaskCS); 00133 00134 return data; 00135 } 00136 00137 void DmTftRa8875::writeReg(uint8_t reg, uint8_t val) 00138 { 00139 sendCommand(reg); 00140 send8BitData(val); 00141 } 00142 00143 uint8_t DmTftRa8875::readReg(uint8_t reg) 00144 { 00145 sendCommand(reg); 00146 return readData(); 00147 } 00148 00149 void DmTftRa8875::setAddress(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1) 00150 { 00151 /* Set active window X */ 00152 writeReg(0x30, x0); // horizontal start point 00153 writeReg(0x31, x0 >> 8); 00154 writeReg(0x34, (uint16_t)(x1) & 0xFF); // horizontal end point 00155 writeReg(0x35, (uint16_t)(x1) >> 8); 00156 00157 /* Set active window Y */ 00158 writeReg(0x32, y0); // vertical start point 00159 writeReg(0x33, y0 >> 8); 00160 writeReg(0x36, (uint16_t)(y1) & 0xFF); // vertical end point 00161 writeReg(0x37, (uint16_t)(y1) >> 8); 00162 00163 writeReg(0x46, x0); 00164 writeReg(0x47, x0 >> 8); 00165 writeReg(0x48, y0); 00166 writeReg(0x49, y0 >> 8); 00167 sendCommand(0x02); 00168 } 00169 00170 void DmTftRa8875::softReset(void) 00171 { 00172 //softreset 00173 sendCommand(0x01); 00174 send8BitData(0x01); 00175 delay(10); 00176 sendCommand(0x01); 00177 send8BitData(0x00); 00178 delay(100); // This much delay needed?? 00179 } 00180 00181 void DmTftRa8875::init(void) 00182 { 00183 // DM_TFT43_108 = RA8875_480x272; DM_TFT50_111 = RA8875_800x480 00184 RA8875Size size = RA8875_480x272; 00185 00186 setTextColor(BLACK, WHITE); 00187 #if defined (DM_TOOLCHAIN_ARDUINO) 00188 _pinCS = portOutputRegister(digitalPinToPort(_cs)); 00189 _bitmaskCS = digitalPinToBitMask(_cs); 00190 _pinSEL = portOutputRegister(digitalPinToPort(_sel)); 00191 _bitmaskSEL = digitalPinToBitMask(_sel); 00192 pinMode(_cs,OUTPUT); 00193 pinMode(_sel,OUTPUT); 00194 digitalWrite(_sel, LOW); // w25 control by MCU 00195 00196 sbi(_pinCS, _bitmaskCS); 00197 00198 SPI.begin(); 00199 SPI.setClockDivider(SPI_CLOCK_DIV2); // 8 MHz (full! speed!) 00200 SPI.setBitOrder(MSBFIRST); 00201 SPI.setDataMode(SPI_MODE0); 00202 _spiSettings = SPCR; 00203 #elif defined (DM_TOOLCHAIN_MBED) 00204 _pinCS = new DigitalOut(_cs); 00205 _pinSEL = new DigitalOut(_sel); 00206 sbi(_pinSEL, _bitmaskSEL); // w25 control by MCU 00207 00208 sbi(_pinCS, _bitmaskCS); 00209 00210 00211 #ifdef TARGET_LPC824 // for LPCXpresso824-MAX 00212 spi.format(8,3); 00213 spi.frequency(8000000); 00214 #elif TARGET_LPC11U6X // for LPCXpresso11U68 00215 spi.format(8,3); 00216 spi.frequency(2000000); 00217 #elif TARGET_NUCLEO_F401RE // for NUCLEO-F401RE 00218 spi.format(8,0); 00219 spi.frequency(8000000); 00220 #else 00221 spi.format(8,3); 00222 spi.frequency(2000000); // Max SPI speed for display is 10 and for 17 for LPC15xx 00223 #endif 00224 softReset(); 00225 #endif 00226 00227 cbi(_pinCS, _bitmaskCS); 00228 00229 /* Timing values */ 00230 uint8_t pixclk; 00231 uint8_t hsync_start; 00232 uint8_t hsync_pw; 00233 uint8_t hsync_finetune; 00234 uint8_t hsync_nondisp; 00235 uint8_t vsync_pw; 00236 uint16_t vsync_nondisp; 00237 uint16_t vsync_start; 00238 00239 if(size == RA8875_480x272) { 00240 _width = 480; 00241 _height = 272; 00242 setWidth(_width); 00243 setHeight(_height); 00244 // PLL init 00245 // 20MHz*(10+1)/((RA8875_PLLC1_PLLDIV1 +1) * 2^RA8875_PLLC2_DIV4)) 00246 writeReg(0x88, 0x00 + 10); 00247 delay(1); 00248 writeReg(0x89, 0x02); 00249 delay(1); 00250 00251 pixclk = 0x80 | 0x02; 00252 hsync_nondisp = 10; 00253 hsync_start = 8; 00254 hsync_pw = 48; 00255 hsync_finetune = 0; 00256 vsync_nondisp = 3; 00257 vsync_start = 8; 00258 vsync_pw = 10; 00259 } 00260 00261 if(size == RA8875_800x480) { 00262 _width = 800; 00263 _height = 480; 00264 setWidth(_width); 00265 setHeight(_height); 00266 // PLL init 00267 // 20MHz*(10+1)/((RA8875_PLLC1_PLLDIV1 +1) * 2^RA8875_PLLC2_DIV4)) 00268 writeReg(0x88, 0x00 + 10); 00269 delay(1); 00270 writeReg(0x89, 0x02); 00271 delay(1); 00272 00273 pixclk = 0x80 | 0x01; 00274 hsync_nondisp = 26; 00275 hsync_start = 32; 00276 hsync_pw = 96; 00277 hsync_finetune = 0; 00278 vsync_nondisp = 32; 00279 vsync_start = 23; 00280 vsync_pw = 2; 00281 } 00282 00283 writeReg(0x10, 0x0C | 0x00); 00284 00285 writeReg(0x04, pixclk); 00286 delay(1); 00287 00288 /* Horizontal settings registers */ 00289 writeReg(0x14, (_width / 8) - 1); // H width: (HDWR + 1) * 8 = 480 00290 writeReg(0x15, 0x00 + hsync_finetune); 00291 writeReg(0x16, (hsync_nondisp - hsync_finetune - 2)/8); // H non-display: HNDR * 8 + HNDFTR + 2 = 10 00292 writeReg(0x17, hsync_start/8 - 1); // Hsync start: (HSTR + 1)*8 00293 writeReg(0x18, 0x00 + (hsync_pw/8 - 1)); // HSync pulse width = (HPWR+1) * 8 00294 00295 /* Vertical settings registers */ 00296 writeReg(0x19, (uint16_t)(_height - 1) & 0xFF); 00297 writeReg(0x1A, (uint16_t)(_height - 1) >> 8); 00298 writeReg(0x1B, vsync_nondisp-1); // V non-display period = VNDR + 1 00299 writeReg(0x1C, vsync_nondisp >> 8); 00300 writeReg(0x1D, vsync_start-1); // Vsync start position = VSTR + 1 00301 writeReg(0x1E, vsync_start >> 8); 00302 writeReg(0x1F, 0x00 + vsync_pw - 1); // Vsync pulse width = VPWR + 1 00303 00304 /* Set active window X */ 00305 writeReg(0x30, 0); // horizontal start point 00306 writeReg(0x31, 0); 00307 writeReg(0x34, (uint16_t)(_width - 1) & 0xFF); // horizontal end point 00308 writeReg(0x35, (uint16_t)(_width - 1) >> 8); 00309 00310 /* Set active window Y */ 00311 writeReg(0x32, 0); // vertical start point 00312 writeReg(0x33, 0); 00313 writeReg(0x36, (uint16_t)(_height - 1) & 0xFF); // vertical end point 00314 writeReg(0x37, (uint16_t)(_height - 1) >> 8); 00315 /* Clear the entire window */ 00316 writeReg(0x8E, 0x80 | 0x00); 00317 /* Wait for the command to finish */ 00318 while (readReg(0x8E) & 0x80); 00319 //delay(100); 00320 // display on 00321 writeReg(0x01, 0x00 | 0x80); 00322 // GPIOX on 00323 writeReg(0xC7, 1); 00324 sbi(_pinCS, _bitmaskCS); 00325 00326 //clearScreen(); 00327 setFontColor(BLACK, WHITE); 00328 //setFontZoom(0, 0); 00329 00330 // spi flash control by MCU 00331 w25CtrlByMCU(); 00332 00333 //backlight on 00334 backlightOn(true); 00335 backlightAdjust(255); // max luminance 00336 00337 } 00338 00339 void DmTftRa8875::eableKeyScan(bool on) 00340 { 00341 if(on) { 00342 writeReg(0xC0, (1 << 7) | (0 << 4 ) | 1 ); // enable key scan 00343 } else { 00344 writeReg(0xC0, (0 << 7)); 00345 } 00346 } 00347 00348 uint8_t DmTftRa8875::getKeyValue(void) 00349 { 00350 uint8_t data = 0xFF; 00351 sendCommand(0xC2); 00352 data = readData(); 00353 00354 // Clear key interrupt status 00355 writeReg(0xF1,readReg(0xF1) | 0x10); 00356 delay(1); 00357 return data; 00358 00359 } 00360 00361 bool DmTftRa8875::isKeyPress(void) 00362 { 00363 uint8_t temp; 00364 temp = readReg(0xF1); 00365 00366 if(temp & 0x10) { 00367 return true; 00368 } else { 00369 return false; 00370 } 00371 } 00372 00373 void DmTftRa8875::backlightOn(bool on) 00374 { 00375 if(on) { 00376 writeReg(0x8A, (1 << 7) | (10 << 0 )); // enable PWM1 00377 } else { 00378 writeReg(0x8A, (0 << 7) | (10 << 0 )); 00379 } 00380 } 00381 00382 void DmTftRa8875::backlightAdjust(uint8_t value) 00383 { 00384 writeReg(0x8B, value); 00385 } 00386 00387 void DmTftRa8875::w25CtrlByMCU(void) 00388 { 00389 #if defined (DM_TOOLCHAIN_ARDUINO) 00390 digitalWrite(_sel, LOW); 00391 #elif defined (DM_TOOLCHAIN_MBED) 00392 cbi(_pinSEL, _bitmaskSEL); 00393 #endif 00394 } 00395 00396 void DmTftRa8875::w25CtrlByRa8875(void) 00397 { 00398 #if defined (DM_TOOLCHAIN_ARDUINO) 00399 digitalWrite(_sel, HIGH); 00400 #elif defined (DM_TOOLCHAIN_MBED) 00401 sbi(_pinSEL, _bitmaskSEL); 00402 #endif 00403 00404 } 00405 00406 void DmTftRa8875::drawImageContinuous(uint32_t startaddress,uint32_t count,uint16_t x0,uint16_t y0,uint16_t x1,uint16_t y1) 00407 { 00408 w25CtrlByRa8875(); 00409 00410 setAddress(x0, y0, x1-1, y1-1); 00411 00412 writeReg(0xE0, 0x00); 00413 00414 writeReg(0x05, (0 << 7) | (0 << 6) | (1 << 5) | (0 << 3) | (1 << 2) | (3 << 1)); 00415 writeReg(0x06, (0 << 0)); // set serial flash frequency 00416 00417 writeReg(0xB0,startaddress & 0xFF); // DMA Source Start Address 00418 writeReg(0xB1,startaddress >> 8); 00419 writeReg(0xB2,startaddress >> 16); 00420 00421 writeReg(0xB4, count & 0xFF); // DMA Transfer Number 00422 writeReg(0xB6, (count & 0xFF00) >> 8 ); 00423 writeReg(0xB8, (count & 0xFF0000) >> 16); 00424 00425 00426 writeReg(0xBF, 0x00); // Continuous mode 00427 writeReg(0xBF, readReg(0xBF) | 0x01); //start DMA 00428 00429 /* Wait for the DMA Transfer to finish */ 00430 while (readReg(0xBF) & 0x01); 00431 00432 w25CtrlByMCU(); 00433 00434 } 00435 00436 void DmTftRa8875::drawImageBlock(uint32_t startaddress, uint32_t count, uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1,uint16_t pic_width, uint16_t block_width, uint16_t block_height) 00437 { 00438 w25CtrlByRa8875(); 00439 00440 setAddress(x0, y0, x1-1, y1-1); 00441 00442 writeReg(0xE0, 0x00); 00443 00444 writeReg(0x05, (0 << 7) | (0 << 6) | (1 << 5) | (0 << 3) | (1 << 2) | (3 << 1)); 00445 writeReg(0x06, (0 << 0)); // set serial flash frequency 00446 00447 writeReg(0xB0,startaddress & 0xFF); // DMA Source Start Address 00448 writeReg(0xB1,startaddress >> 8); 00449 writeReg(0xB2,startaddress >> 16); 00450 00451 writeReg(0xB4, block_width & 0xFF); // DMA block width 00452 writeReg(0xB5, block_width >> 8 ); 00453 writeReg(0xB6, block_height & 0xFF); // DMA block height 00454 writeReg(0xB7, block_height >> 8 ); 00455 00456 writeReg(0xB8, pic_width & 0xFF); // DMA soruce picture width 00457 writeReg(0xB9, pic_width >> 8 ); 00458 00459 writeReg(0xBF, 0x02); // block mode 00460 writeReg(0xBF, readReg(0xBF) | 0x01); //start DMA 00461 00462 /* Wait for the DMA Transfer to finish */ 00463 while (readReg(0xBF) & 0x01); 00464 00465 w25CtrlByMCU(); 00466 00467 } 00468 00469 void DmTftRa8875::drawPoint(uint16_t x, uint16_t y, uint16_t radius) 00470 { 00471 if (radius == 0) { 00472 cbi(_pinCS, _bitmaskCS); 00473 00474 setAddress(x,y,x,y); 00475 sendData(_fgColor); 00476 00477 sbi(_pinCS, _bitmaskCS); 00478 } else { 00479 fillRectangle(x-radius,y-radius,x+radius,y+radius, _fgColor); 00480 } 00481 } 00482 00483 00484 void DmTftRa8875::setFontColor(uint16_t background,uint16_t foreground) 00485 { 00486 /* Set Background Color */ 00487 _bgColor = background; 00488 writeReg(0x60, (background & 0xf800) >> 11); 00489 writeReg(0x61, (background & 0x07e0) >> 5); 00490 writeReg(0x62, (background & 0x001f)); 00491 00492 /* Set Fore Color */ 00493 _fgColor = foreground; 00494 writeReg(0x63, (foreground & 0xf800) >> 11); 00495 writeReg(0x64, (foreground & 0x07e0) >> 5); 00496 writeReg(0x65, (foreground & 0x001f)); 00497 } 00498 00499 00500 void DmTftRa8875::setFontZoom(uint8_t Hsize,uint8_t Vsize) 00501 { 00502 writeReg(0x22, ((Hsize & 0x03) <<2 | (Vsize & 0x03))); 00503 } 00504 00505 void DmTftRa8875::int2str(int n, char *str) 00506 { 00507 char buf[10] = ""; 00508 int i = 0; 00509 int len = 0; 00510 int temp = n < 0 ? -n: n; 00511 00512 if (str == NULL) { 00513 return; 00514 } 00515 while(temp) { 00516 buf[i++] = (temp % 10) + '0'; 00517 temp = temp / 10; 00518 } 00519 00520 len = n < 0 ? ++i: i; 00521 str[i] = 0; 00522 while(1) { 00523 i--; 00524 if (buf[len-i-1] ==0) { 00525 break; 00526 } 00527 str[i] = buf[len-i-1]; 00528 } 00529 if (i == 0 ) { 00530 str[i] = '-'; 00531 } 00532 } 00533 00534 void DmTftRa8875::drawNumber(uint16_t x, uint16_t y, int num, int digitsToShow, bool leadingZeros) 00535 { 00536 char p[10]; 00537 int2str(num, p); 00538 00539 // clear the last number on the screen; default font width is 8. 00540 for(int i=0; i<digitsToShow; i++) 00541 drawString(x+i*8, y," "); 00542 00543 drawString(x, y, p); 00544 } 00545 00546 00547 void DmTftRa8875::drawString(uint16_t x, uint16_t y, const char *p) 00548 { 00549 setAddress(0, 0, _width-1, _height-1); 00550 00551 writeReg(0x40, (1 << 7) | (1 << 0)); // enter text mode 00552 writeReg(0x2A, x); 00553 writeReg(0x2B, x >> 8); 00554 writeReg(0x2C, y); 00555 writeReg(0x2D, y >> 8); 00556 00557 writeReg(0x2F, 0x00); 00558 writeReg(0x21, (0 << 7) | (0 << 5) | (0 << 1) | (0 << 0)); 00559 sendCommand(0x02); 00560 00561 while (*p != '\0') { 00562 send8BitData(*p); 00563 while ((readStatus() & 0x80) == 0x80); // wait finish 00564 p++; 00565 } 00566 writeReg(0x40, (0 << 7)); // enter graphics mode 00567 } 00568 00569 void DmTftRa8875::drawStringCentered(uint16_t x, uint16_t y, uint16_t width, uint16_t height, const char *p) 00570 { 00571 00572 int len = strlen(p); 00573 uint16_t tmp = len * 8; 00574 if (tmp <= width) { 00575 x += (width - tmp)/2; 00576 } 00577 if (16 <= height) { 00578 y += (height - 16)/2; 00579 } 00580 00581 drawString(x, y, p); 00582 } 00583 00584 void DmTftRa8875::clearScreen(uint16_t color) 00585 { 00586 rectangle(0, 0, _width-1, _height-1, color, true); 00587 } 00588 00589 void DmTftRa8875::rectangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t color, bool filled) 00590 { 00591 setAddress(0, 0, _width-1, _height-1); 00592 00593 /* Set x0 */ 00594 writeReg(0x91, x0); 00595 writeReg(0x92, x0 >> 8); 00596 00597 /* Set y0 */ 00598 writeReg(0x93, y0); 00599 writeReg(0x94, y0 >> 8); 00600 00601 /* Set x1 */ 00602 writeReg(0x95, x1); 00603 writeReg(0x96, x1 >> 8); 00604 00605 /* Set y1 */ 00606 writeReg(0x97, y1); 00607 writeReg(0x98, y1 >> 8); 00608 00609 /* Set Color */ 00610 writeReg(0x63, (color & 0xf800) >> 11); 00611 writeReg(0x64, (color & 0x07e0) >> 5); 00612 writeReg(0x65, (color & 0x001f)); 00613 00614 /* Draw! */ 00615 if (filled) { 00616 writeReg(0x90, 0xB0); 00617 } else { 00618 writeReg(0x90, 0x90); 00619 } 00620 00621 /* Wait for the command to finish */ 00622 while (readReg(0x90) & 0x80); 00623 } 00624 00625 void DmTftRa8875::drawRectangle(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1, uint16_t color) 00626 { 00627 rectangle(x0, y0, x1, y1, color, false); 00628 } 00629 void DmTftRa8875::fillRectangle(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1, uint16_t color) 00630 { 00631 rectangle(x0, y0, x1, y1, color, true); 00632 } 00633 00634 void DmTftRa8875::drawLine(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1, uint16_t color) 00635 { 00636 setAddress(0, 0, _width-1, _height-1); 00637 00638 /* Set x0 */ 00639 writeReg(0x91, x0); 00640 writeReg(0x92, x0 >> 8); 00641 00642 /* Set y0 */ 00643 writeReg(0x93, y0); 00644 writeReg(0x94, y0 >> 8); 00645 00646 /* Set x1 */ 00647 writeReg(0x95, x1); 00648 writeReg(0x96, x1 >> 8); 00649 00650 /* Set y1 */ 00651 writeReg(0x97, y1); 00652 writeReg(0x98, y1 >> 8); 00653 00654 /* Set Color */ 00655 writeReg(0x63, (color & 0xf800) >> 11); 00656 writeReg(0x64, (color & 0x07e0) >> 5); 00657 writeReg(0x65, (color & 0x001f)); 00658 00659 /* Draw! */ 00660 writeReg(0x90, 0x80); 00661 00662 /* Wait for the command to finish */ 00663 while (readReg(0x90) & 0x80); 00664 } 00665 00666 void DmTftRa8875::drawVerticalLine(uint16_t x, uint16_t y, uint16_t length, uint16_t color) 00667 { 00668 drawLine(x, y, x, y+length, color); 00669 } 00670 00671 void DmTftRa8875::drawHorizontalLine(uint16_t x, uint16_t y, uint16_t length, uint16_t color) 00672 { 00673 drawLine(x, y, x+length, y, color); 00674 } 00675 00676 void DmTftRa8875::circle(int16_t x, int16_t y, int16_t r, uint16_t color, bool filled) 00677 { 00678 setAddress(0, 0, _width-1, _height-1); 00679 00680 /* Set x */ 00681 writeReg(0x99, x); 00682 writeReg(0x9A, x >> 8); 00683 00684 /* Set y */ 00685 writeReg(0x9B, y); 00686 writeReg(0x9C, y >> 8); 00687 00688 /* Set Radius */ 00689 writeReg(0x9D, r); 00690 00691 /* Set Color */ 00692 writeReg(0x63, (color & 0xf800) >> 11); 00693 writeReg(0x64, (color & 0x07e0) >> 5); 00694 writeReg(0x65, (color & 0x001f)); 00695 00696 /* Draw! */ 00697 if (filled) { 00698 writeReg(0x90, 0x40 | 0x20); 00699 } else { 00700 writeReg(0x90, 0x40 | 0x00); 00701 } 00702 00703 /* Wait for the command to finish */ 00704 while (readReg(0x90) & 0x40); 00705 } 00706 00707 void DmTftRa8875::drawCircle(uint16_t x0, uint16_t y0, uint16_t r, uint16_t color) 00708 { 00709 circle(x0, y0, r, color, false); 00710 } 00711 void DmTftRa8875::fillCircle(uint16_t x0, uint16_t y0, uint16_t r, uint16_t color) 00712 { 00713 circle(x0, y0, r, color, true); 00714 } 00715 00716 void DmTftRa8875::triangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, int16_t x2, int16_t y2, uint16_t color, bool filled) 00717 { 00718 setAddress(0, 0, _width-1, _height-1); 00719 00720 /* Set Point 0 */ 00721 writeReg(0x91, x0); 00722 writeReg(0x92, (x0 >> 8)); 00723 writeReg(0x93, y0); 00724 writeReg(0x94, (y0 >> 8)); 00725 00726 /* Set Point 1 */ 00727 writeReg(0x95, x1); 00728 writeReg(0x96, (x1 >> 8)); 00729 writeReg(0x97, y1); 00730 writeReg(0x98, (y1 >> 8)); 00731 00732 /* Set Point 2 */ 00733 writeReg(0xA9, x2); 00734 writeReg(0xAA, (x2 >> 8)); 00735 writeReg(0xAB, y2); 00736 writeReg(0xAC, (y2 >> 8)); 00737 00738 /* Set Color */ 00739 writeReg(0x63, (color & 0xf800) >> 11); 00740 writeReg(0x64, (color & 0x07e0) >> 5); 00741 writeReg(0x65, (color & 0x001f)); 00742 00743 /* Draw! */ 00744 if (filled) { 00745 writeReg(0x90, 0xA1); 00746 } else { 00747 writeReg(0x90, 0x81); 00748 } 00749 00750 /* Wait for the command to finish */ 00751 while (readReg(0x90) & 0x80); 00752 } 00753 00754 void DmTftRa8875::drawTriangle(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint16_t color) 00755 { 00756 triangle(x0, y0, x1, y1, x2, y2, color, false); 00757 } 00758 00759 void DmTftRa8875::fillTriangle(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2, uint16_t color) 00760 { 00761 triangle(x0, y0, x1, y1, x2, y2, color, true); 00762 } 00763 00764 void DmTftRa8875::ellipse(int16_t x0, int16_t y0, int16_t longAxis, int16_t shortAxis, uint16_t color, bool filled) 00765 { 00766 setAddress(0, 0, _width-1, _height-1); 00767 00768 /* Set Center Point */ 00769 writeReg(0xA5, x0); 00770 writeReg(0xA6, (x0 >> 8)); 00771 writeReg(0xA7, y0); 00772 writeReg(0xA8, (y0 >> 8)); 00773 00774 /* Set Long and Short Axis */ 00775 writeReg(0xA1, longAxis); 00776 writeReg(0xA2, (longAxis >> 8)); 00777 writeReg(0xA3, shortAxis); 00778 writeReg(0xA4, (shortAxis >> 8)); 00779 00780 /* Set Color */ 00781 writeReg(0x63, (color & 0xf800) >> 11); 00782 writeReg(0x64, (color & 0x07e0) >> 5); 00783 writeReg(0x65, (color & 0x001f)); 00784 00785 /* Draw! */ 00786 if (filled) { 00787 writeReg(0xA0, 0xC0); 00788 } else { 00789 writeReg(0xA0, 0x80); 00790 } 00791 00792 /* Wait for the command to finish */ 00793 while (readReg(0xA0) & 0x80); 00794 00795 } 00796 00797 void DmTftRa8875::drawEllipse(int16_t x0, int16_t y0, int16_t longAxis, int16_t shortAxis, uint16_t color) 00798 { 00799 ellipse(x0, y0, longAxis, shortAxis, color, false); 00800 } 00801 00802 void DmTftRa8875::fillEllipse(int16_t x0, int16_t y0, int16_t longAxis, int16_t shortAxis, uint16_t color) 00803 { 00804 ellipse(x0, y0, longAxis, shortAxis, color, true); 00805 } 00806 00807 void DmTftRa8875::curve(int16_t x0, int16_t y0, int16_t longAxis, int16_t shortAxis, uint8_t curvePart, uint16_t color, bool filled) 00808 { 00809 setAddress(0, 0, _width-1, _height-1); 00810 00811 /* Set Center Point */ 00812 writeReg(0xA5, x0); 00813 writeReg(0xA6, (x0 >> 8)); 00814 writeReg(0xA7, y0); 00815 writeReg(0xA8, (y0 >> 8)); 00816 00817 /* Set Long and Short Axis */ 00818 writeReg(0xA1, longAxis); 00819 writeReg(0xA2, (longAxis >> 8)); 00820 writeReg(0xA3, shortAxis); 00821 writeReg(0xA4, (shortAxis >> 8)); 00822 00823 /* Set Color */ 00824 writeReg(0x63, (color & 0xf800) >> 11); 00825 writeReg(0x64, (color & 0x07e0) >> 5); 00826 writeReg(0x65, (color & 0x001f)); 00827 00828 /* Draw! */ 00829 if (filled) { 00830 writeReg(0xA0, 0xD0 | (curvePart & 0x03)); 00831 } else { 00832 writeReg(0xA0, 0x90 | (curvePart & 0x03)); 00833 } 00834 00835 /* Wait for the command to finish */ 00836 while (readReg(0xA0) & 0x80); 00837 00838 } 00839 00840 void DmTftRa8875::drawCurve(int16_t x0, int16_t y0, int16_t longAxis, int16_t shortAxis, uint8_t curvePart, uint16_t color) 00841 { 00842 curve(x0, y0, longAxis, shortAxis, curvePart, color, false); 00843 } 00844 00845 void DmTftRa8875::fillCurve(int16_t x0, int16_t y0, int16_t longAxis, int16_t shortAxis, uint8_t curvePart, uint16_t color) 00846 { 00847 curve(x0, y0, longAxis, shortAxis, curvePart, color, true); 00848 } 00849 00850 void DmTftRa8875::roundrectangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t r1, uint16_t r2, uint16_t color, bool filled) 00851 { 00852 setAddress(0, 0, _width-1, _height-1); 00853 00854 /* Set x0 */ 00855 writeReg(0x91, x0); 00856 writeReg(0x92, x0 >> 8); 00857 00858 /* Set y0 */ 00859 writeReg(0x93, y0); 00860 writeReg(0x94, y0 >> 8); 00861 00862 /* Set x1 */ 00863 writeReg(0x95, x1); 00864 writeReg(0x96, x1 >> 8); 00865 00866 /* Set y1 */ 00867 writeReg(0x97, y1); 00868 writeReg(0x98, y1 >> 8); 00869 00870 /* Set Radius */ 00871 writeReg(0xA1, r1); 00872 writeReg(0xA2, r1 >> 8); 00873 writeReg(0xA3, r2); 00874 writeReg(0xA4, r2 >> 8); 00875 00876 /* Set Color */ 00877 writeReg(0x63, (color & 0xf800) >> 11); 00878 writeReg(0x64, (color & 0x07e0) >> 5); 00879 writeReg(0x65, (color & 0x001f)); 00880 00881 /* Draw! */ 00882 if (filled) { 00883 writeReg(0xA0, 0xE0); 00884 } else { 00885 writeReg(0xA0, 0xA0); 00886 } 00887 00888 /* Wait for the command to finish */ 00889 while (readReg(0xA0) & 0x80); 00890 } 00891 00892 void DmTftRa8875::drawRoundRectangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t r1, uint16_t r2, uint16_t color) 00893 { 00894 roundrectangle(x0, y0, x1, y1, r1, r2, color, false); 00895 } 00896 void DmTftRa8875::fillRoundRectangle(int16_t x0, int16_t y0, int16_t x1, int16_t y1, uint16_t r1, uint16_t r2, uint16_t color) 00897 { 00898 roundrectangle(x0, y0, x1, y1, r1, r2, color, true); 00899 } 00900 /********************************************************************************************************* 00901 END FILE 00902 *********************************************************************************************************/
Generated on Wed Jul 13 2022 04:21:40 by
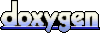