Driver Library for our displays
Dependents: dm_bubbles dm_calc dm_paint dm_sdcard_with_adapter ... more
DmTftIli9325.cpp
00001 /********************************************************************************************** 00002 Copyright (c) 2014 DisplayModule. All rights reserved. 00003 00004 Redistribution and use of this source code, part of this source code or any compiled binary 00005 based on this source code is permitted as long as the above copyright notice and following 00006 disclaimer is retained. 00007 00008 DISCLAIMER: 00009 THIS SOFTWARE IS SUPPLIED "AS IS" WITHOUT ANY WARRANTIES AND SUPPORT. DISPLAYMODULE ASSUMES 00010 NO RESPONSIBILITY OR LIABILITY FOR THE USE OF THE SOFTWARE. 00011 ********************************************************************************************/ 00012 00013 //Tested on NUCLEO-F401RE, LPCXpresso11U68 platform. 00014 00015 #include "DmTftIli9325.h" 00016 00017 #if defined (DM_TOOLCHAIN_ARDUINO) 00018 DmTftIli9325::DmTftIli9325(uint8_t wr, uint8_t cs, uint8_t dc, uint8_t rst) : DmTftBase(240, 320) 00019 #elif defined (DM_TOOLCHAIN_MBED) 00020 DmTftIli9325::DmTftIli9325(PinName wr, PinName cs, PinName dc, PinName rst) : DmTftBase(240, 320) 00021 #endif 00022 { 00023 _wr = wr; 00024 _cs = cs; 00025 _dc = dc; 00026 _rst = rst; 00027 } 00028 00029 DmTftIli9325::~DmTftIli9325() { 00030 #if defined (DM_TOOLCHAIN_MBED) 00031 delete _pinRST; 00032 delete _pinCS; 00033 delete _pinWR; 00034 delete _pinDC; 00035 delete _virtualPortD; 00036 _pinRST = NULL; 00037 _pinCS = NULL; 00038 _pinWR = NULL; 00039 _pinDC = NULL; 00040 _virtualPortD = NULL; 00041 #endif 00042 } 00043 00044 void DmTftIli9325::writeBus(uint8_t data) { 00045 #if defined (DM_TOOLCHAIN_ARDUINO) 00046 PORTD = data; 00047 #elif defined (DM_TOOLCHAIN_MBED) 00048 *_virtualPortD = data; 00049 //if(data & 0x10) 00050 #endif 00051 pulse_low(_pinWR, _bitmaskWR); 00052 } 00053 00054 void DmTftIli9325::sendCommand(uint8_t index) { 00055 cbi(_pinDC, _bitmaskDC); 00056 writeBus(0x00); 00057 writeBus(index); 00058 } 00059 00060 void DmTftIli9325::sendData(uint16_t data) { 00061 sbi(_pinDC, _bitmaskDC); 00062 writeBus(data>>8); 00063 writeBus(data); 00064 } 00065 00066 void DmTftIli9325::setAddress(uint16_t x0, uint16_t y0, uint16_t x1, uint16_t y1) { 00067 sendCommand(0x50); // Set Column 00068 sendData(x0); 00069 sendCommand(0x51); 00070 sendData(x1); 00071 00072 sendCommand(0x52); // Set Page 00073 sendData(y0); 00074 sendCommand(0x53); 00075 sendData(y1); 00076 00077 sendCommand(0x20); 00078 sendData(x0); 00079 sendCommand(0x21); 00080 sendData(y0); 00081 sendCommand(0x22); 00082 } 00083 00084 void DmTftIli9325::init(void) { 00085 setTextColor(BLACK, WHITE); 00086 #if defined (DM_TOOLCHAIN_ARDUINO) 00087 /************************************** 00088 DM-DmTftIli932522-102 Arduino UNO NUM 00089 00090 RST A2 16 00091 CS A3 17 00092 WR A4 18 00093 RS A5 19 00094 00095 DB8 0 0 00096 DB9 1 1 00097 DB10 2 2 00098 DB11 3 3 00099 DB12 4 4 00100 DB13 5 5 00101 DB14 6 6 00102 DB15 7 7 00103 00104 ***************************************/ 00105 DDRD = DDRD | B11111111; // SET PORT D AS OUTPUT 00106 00107 _pinRST = portOutputRegister(digitalPinToPort(_rst)); 00108 _bitmaskRST = digitalPinToBitMask(_rst); 00109 _pinCS = portOutputRegister(digitalPinToPort(_cs)); 00110 _bitmaskCS = digitalPinToBitMask(_cs); 00111 _pinWR = portOutputRegister(digitalPinToPort(_wr)); 00112 _bitmaskWR = digitalPinToBitMask(_wr); 00113 _pinDC = portOutputRegister(digitalPinToPort(_dc)); 00114 _bitmaskDC = digitalPinToBitMask(_dc); 00115 00116 pinMode(_rst, OUTPUT); 00117 pinMode(_cs, OUTPUT); 00118 pinMode(_wr, OUTPUT); 00119 pinMode(_dc,OUTPUT); 00120 #elif defined (DM_TOOLCHAIN_MBED) 00121 _pinRST = new DigitalOut(_rst); 00122 _pinCS = new DigitalOut(_cs); 00123 _pinWR = new DigitalOut(_wr); 00124 _pinDC = new DigitalOut(_dc); 00125 _virtualPortD = new BusOut(D0, D1, D2, D3, D4, SPECIAL_D5, D6, D7); // LPC15XX_H 00126 #endif 00127 00128 sbi(_pinRST, _bitmaskRST); 00129 00130 delay(5); 00131 cbi(_pinRST, _bitmaskRST); 00132 00133 delay(15); 00134 sbi(_pinRST, _bitmaskRST); 00135 sbi(_pinCS, _bitmaskCS); 00136 sbi(_pinWR, _bitmaskWR); 00137 00138 delay(15); 00139 cbi(_pinCS, _bitmaskCS); 00140 00141 00142 00143 sendCommand(0xE5); sendData(0x78F0); 00144 sendCommand(0x01); sendData(0x0100); 00145 sendCommand(0x02); sendData(0x0700); 00146 sendCommand(0x03); sendData(0x1030); 00147 sendCommand(0x04); sendData(0x0000); 00148 sendCommand(0x08); sendData(0x0207); 00149 sendCommand(0x09); sendData(0x0000); 00150 sendCommand(0x0A); sendData(0x0000); 00151 sendCommand(0x0C); sendData(0x0000); 00152 sendCommand(0x0D); sendData(0x0000); 00153 sendCommand(0x0F); sendData(0x0000); 00154 00155 sendCommand(0x10); sendData(0x0000); 00156 sendCommand(0x11); sendData(0x0007); 00157 sendCommand(0x12); sendData(0x0000); 00158 sendCommand(0x13); sendData(0x0000); 00159 00160 sendCommand(0x10); sendData(0x1290); 00161 sendCommand(0x11); sendData(0x0227); 00162 sendCommand(0x12); sendData(0x001D); 00163 sendCommand(0x13); sendData(0x1500); 00164 00165 sendCommand(0x29); sendData(0x0018); 00166 sendCommand(0x2B); sendData(0x000D); 00167 00168 sendCommand(0x30); sendData(0x0004); 00169 sendCommand(0x31); sendData(0x0307); 00170 sendCommand(0x32); sendData(0x0002); 00171 sendCommand(0x35); sendData(0x0206); 00172 sendCommand(0x36); sendData(0x0408); 00173 sendCommand(0x37); sendData(0x0507); 00174 sendCommand(0x38); sendData(0x0204); 00175 sendCommand(0x39); sendData(0x0707); 00176 sendCommand(0x3C); sendData(0x0405); 00177 sendCommand(0x3D); sendData(0x0f02); 00178 00179 sendCommand(0x50); sendData(0x0000); 00180 sendCommand(0x51); sendData(0x00EF); 00181 sendCommand(0x52); sendData(0x0000); 00182 sendCommand(0x53); sendData(0x013F); 00183 sendCommand(0x60); sendData(0xA700); 00184 sendCommand(0x61); sendData(0x0001); 00185 sendCommand(0x6A); sendData(0x0000); 00186 00187 sendCommand(0x80); sendData(0x0000); 00188 sendCommand(0x81); sendData(0x0000); 00189 sendCommand(0x82); sendData(0x0000); 00190 sendCommand(0x83); sendData(0x0000); 00191 sendCommand(0x84); sendData(0x0000); 00192 sendCommand(0x85); sendData(0x0000); 00193 00194 sendCommand(0x90); sendData(0x0010); 00195 sendCommand(0x92); sendData(0x0600); 00196 sendCommand(0x93); sendData(0x0003); 00197 sendCommand(0x95); sendData(0x0110); 00198 sendCommand(0x97); sendData(0x0000); 00199 sendCommand(0x98); sendData(0x0000); 00200 sendCommand(0x07); sendData(0x0133); 00201 sbi(_pinCS, _bitmaskCS); 00202 delay(500); 00203 clearScreen(); 00204 } 00205 00206 /********************************************************************************************************* 00207 END FILE 00208 *********************************************************************************************************/ 00209
Generated on Wed Jul 13 2022 04:21:40 by
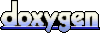