
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
usbhost_lpc17xx.h
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 /* 00025 ************************************************************************************************************** 00026 * NXP USB Host Stack 00027 * 00028 * (c) Copyright 2008, NXP SemiConductors 00029 * (c) Copyright 2008, OnChip Technologies LLC 00030 * All Rights Reserved 00031 * 00032 * www.nxp.com 00033 * www.onchiptech.com 00034 * 00035 * File : usbhost_lpc17xx.h 00036 * Programmer(s) : Ravikanth.P 00037 * Version : 00038 * 00039 ************************************************************************************************************** 00040 */ 00041 00042 #ifndef USBHOST_LPC17xx_H 00043 #define USBHOST_LPC17xx_H 00044 00045 /* 00046 ************************************************************************************************************** 00047 * INCLUDE HEADER FILES 00048 ************************************************************************************************************** 00049 */ 00050 00051 #include "usbhost_inc.h" 00052 00053 /* 00054 ************************************************************************************************************** 00055 * PRINT CONFIGURATION 00056 ************************************************************************************************************** 00057 */ 00058 00059 #define PRINT_ENABLE 1 00060 00061 #if PRINT_ENABLE 00062 #define PRINT_Log(...) printf(__VA_ARGS__) 00063 #define PRINT_Err(rc) printf("ERROR: In %s at Line %u - rc = %d\n", __FUNCTION__, __LINE__, rc) 00064 00065 #else 00066 #define PRINT_Log(...) do {} while(0) 00067 #define PRINT_Err(rc) do {} while(0) 00068 00069 #endif 00070 00071 /* 00072 ************************************************************************************************************** 00073 * GENERAL DEFINITIONS 00074 ************************************************************************************************************** 00075 */ 00076 00077 #define DESC_LENGTH(x) x[0] 00078 #define DESC_TYPE(x) x[1] 00079 00080 00081 #define HOST_GET_DESCRIPTOR(descType, descIndex, data, length) \ 00082 Host_CtrlRecv(USB_DEVICE_TO_HOST | USB_RECIPIENT_DEVICE, GET_DESCRIPTOR, \ 00083 (descType << 8)|(descIndex), 0, length, data) 00084 00085 #define HOST_SET_ADDRESS(new_addr) \ 00086 Host_CtrlSend(USB_HOST_TO_DEVICE | USB_RECIPIENT_DEVICE, SET_ADDRESS, \ 00087 new_addr, 0, 0, NULL) 00088 00089 #define USBH_SET_CONFIGURATION(configNum) \ 00090 Host_CtrlSend(USB_HOST_TO_DEVICE | USB_RECIPIENT_DEVICE, SET_CONFIGURATION, \ 00091 configNum, 0, 0, NULL) 00092 00093 #define USBH_SET_INTERFACE(ifNum, altNum) \ 00094 Host_CtrlSend(USB_HOST_TO_DEVICE | USB_RECIPIENT_INTERFACE, SET_INTERFACE, \ 00095 altNum, ifNum, 0, NULL) 00096 00097 /* 00098 ************************************************************************************************************** 00099 * OHCI OPERATIONAL REGISTER FIELD DEFINITIONS 00100 ************************************************************************************************************** 00101 */ 00102 00103 /* ------------------ HcControl Register --------------------- */ 00104 #define OR_CONTROL_CLE 0x00000010 00105 #define OR_CONTROL_BLE 0x00000020 00106 #define OR_CONTROL_HCFS 0x000000C0 00107 #define OR_CONTROL_HC_OPER 0x00000080 00108 /* ----------------- HcCommandStatus Register ----------------- */ 00109 #define OR_CMD_STATUS_HCR 0x00000001 00110 #define OR_CMD_STATUS_CLF 0x00000002 00111 #define OR_CMD_STATUS_BLF 0x00000004 00112 /* --------------- HcInterruptStatus Register ----------------- */ 00113 #define OR_INTR_STATUS_WDH 0x00000002 00114 #define OR_INTR_STATUS_RHSC 0x00000040 00115 /* --------------- HcInterruptEnable Register ----------------- */ 00116 #define OR_INTR_ENABLE_WDH 0x00000002 00117 #define OR_INTR_ENABLE_RHSC 0x00000040 00118 #define OR_INTR_ENABLE_MIE 0x80000000 00119 /* ---------------- HcRhDescriptorA Register ------------------ */ 00120 #define OR_RH_STATUS_LPSC 0x00010000 00121 #define OR_RH_STATUS_DRWE 0x00008000 00122 /* -------------- HcRhPortStatus[1:NDP] Register -------------- */ 00123 #define OR_RH_PORT_CCS 0x00000001 00124 #define OR_RH_PORT_PRS 0x00000010 00125 #define OR_RH_PORT_CSC 0x00010000 00126 #define OR_RH_PORT_PRSC 0x00100000 00127 00128 00129 /* 00130 ************************************************************************************************************** 00131 * FRAME INTERVAL 00132 ************************************************************************************************************** 00133 */ 00134 00135 #define FI 0x2EDF /* 12000 bits per frame (-1) */ 00136 #define DEFAULT_FMINTERVAL ((((6 * (FI - 210)) / 7) << 16) | FI) 00137 00138 /* 00139 ************************************************************************************************************** 00140 * ENDPOINT DESCRIPTOR CONTROL FIELDS 00141 ************************************************************************************************************** 00142 */ 00143 00144 #define ED_SKIP (USB_INT32U) (0x00001000) /* Skip this ep in queue */ 00145 00146 /* 00147 ************************************************************************************************************** 00148 * TRANSFER DESCRIPTOR CONTROL FIELDS 00149 ************************************************************************************************************** 00150 */ 00151 00152 #define TD_ROUNDING (USB_INT32U) (0x00040000) /* Buffer Rounding */ 00153 #define TD_SETUP (USB_INT32U)(0) /* Direction of Setup Packet */ 00154 #define TD_IN (USB_INT32U)(0x00100000) /* Direction In */ 00155 #define TD_OUT (USB_INT32U)(0x00080000) /* Direction Out */ 00156 #define TD_DELAY_INT(x) (USB_INT32U)((x) << 21) /* Delay Interrupt */ 00157 #define TD_TOGGLE_0 (USB_INT32U)(0x02000000) /* Toggle 0 */ 00158 #define TD_TOGGLE_1 (USB_INT32U)(0x03000000) /* Toggle 1 */ 00159 #define TD_CC (USB_INT32U)(0xF0000000) /* Completion Code */ 00160 00161 /* 00162 ************************************************************************************************************** 00163 * USB STANDARD REQUEST DEFINITIONS 00164 ************************************************************************************************************** 00165 */ 00166 00167 #define USB_DESCRIPTOR_TYPE_DEVICE 1 00168 #define USB_DESCRIPTOR_TYPE_CONFIGURATION 2 00169 #define USB_DESCRIPTOR_TYPE_INTERFACE 4 00170 #define USB_DESCRIPTOR_TYPE_ENDPOINT 5 00171 /* ----------- Control RequestType Fields ----------- */ 00172 #define USB_DEVICE_TO_HOST 0x80 00173 #define USB_HOST_TO_DEVICE 0x00 00174 #define USB_REQUEST_TYPE_CLASS 0x20 00175 #define USB_RECIPIENT_DEVICE 0x00 00176 #define USB_RECIPIENT_INTERFACE 0x01 00177 /* -------------- USB Standard Requests -------------- */ 00178 #define SET_ADDRESS 5 00179 #define GET_DESCRIPTOR 6 00180 #define SET_CONFIGURATION 9 00181 #define SET_INTERFACE 11 00182 00183 /* 00184 ************************************************************************************************************** 00185 * TYPE DEFINITIONS 00186 ************************************************************************************************************** 00187 */ 00188 00189 typedef struct hcEd { /* ----------- HostController EndPoint Descriptor ------------- */ 00190 volatile USB_INT32U Control; /* Endpoint descriptor control */ 00191 volatile USB_INT32U TailTd; /* Physical address of tail in Transfer descriptor list */ 00192 volatile USB_INT32U HeadTd; /* Physcial address of head in Transfer descriptor list */ 00193 volatile USB_INT32U Next; /* Physical address of next Endpoint descriptor */ 00194 } HCED; 00195 00196 typedef struct hcTd { /* ------------ HostController Transfer Descriptor ------------ */ 00197 volatile USB_INT32U Control; /* Transfer descriptor control */ 00198 volatile USB_INT32U CurrBufPtr; /* Physical address of current buffer pointer */ 00199 volatile USB_INT32U Next; /* Physical pointer to next Transfer Descriptor */ 00200 volatile USB_INT32U BufEnd; /* Physical address of end of buffer */ 00201 } HCTD; 00202 00203 typedef struct hcca { /* ----------- Host Controller Communication Area ------------ */ 00204 volatile USB_INT32U IntTable[32]; /* Interrupt Table */ 00205 volatile USB_INT32U FrameNumber; /* Frame Number */ 00206 volatile USB_INT32U DoneHead; /* Done Head */ 00207 volatile USB_INT08U Reserved[116]; /* Reserved for future use */ 00208 volatile USB_INT08U Unknown[4]; /* Unused */ 00209 } HCCA; 00210 00211 /* 00212 ************************************************************************************************************** 00213 * EXTERN DECLARATIONS 00214 ************************************************************************************************************** 00215 */ 00216 #if 0 00217 extern volatile HCED *EDBulkIn; /* BulkIn endpoint descriptor structure */ 00218 extern volatile HCED *EDBulkOut; /* BulkOut endpoint descriptor structure */ 00219 extern volatile HCED *EDBulkHead; 00220 extern volatile HCTD *TDHead; /* Head transfer descriptor structure */ 00221 extern volatile HCTD *TDTail; /* Tail transfer descriptor structure */ 00222 #endif 00223 extern volatile USB_INT08U *TDBuffer; /* Current Buffer Pointer of transfer descriptor */ 00224 00225 /* 00226 ************************************************************************************************************** 00227 * FUNCTION PROTOTYPES 00228 ************************************************************************************************************** 00229 */ 00230 00231 void Host_Init (void); 00232 00233 extern "C" void USB_IRQHandler(void) __irq; 00234 00235 USB_INT32S Host_EnumDev (void); 00236 00237 USB_INT32S Host_TDresult(volatile HCED *ed, 00238 volatile USB_INT32U token); 00239 00240 USB_INT32S Host_ProcessTD(volatile HCED *ed, 00241 volatile USB_INT32U token, 00242 volatile USB_INT08U *buffer, 00243 USB_INT32U buffer_len, 00244 bool block = true); 00245 00246 00247 void Host_DelayUS ( USB_INT32U delay); 00248 void Host_DelayMS ( USB_INT32U delay); 00249 00250 00251 void Host_TDInit (volatile HCTD *td); 00252 void Host_EDInit (volatile HCED *ed); 00253 void Host_HCCAInit (volatile HCCA *hcca); 00254 00255 USB_INT32S Host_CtrlRecv ( USB_INT08U bm_request_type, 00256 USB_INT08U b_request, 00257 USB_INT16U w_value, 00258 USB_INT16U w_index, 00259 USB_INT16U w_length, 00260 volatile USB_INT08U *buffer); 00261 00262 USB_INT32S Host_CtrlSend ( USB_INT08U bm_request_type, 00263 USB_INT08U b_request, 00264 USB_INT16U w_value, 00265 USB_INT16U w_index, 00266 USB_INT16U w_length, 00267 volatile USB_INT08U *buffer); 00268 00269 void Host_FillSetup( USB_INT08U bm_request_type, 00270 USB_INT08U b_request, 00271 USB_INT16U w_value, 00272 USB_INT16U w_index, 00273 USB_INT16U w_length); 00274 00275 00276 void Host_WDHWait (void); 00277 00278 00279 USB_INT32U ReadLE32U (volatile USB_INT08U *pmem); 00280 void WriteLE32U (volatile USB_INT08U *pmem, 00281 USB_INT32U val); 00282 USB_INT16U ReadLE16U (volatile USB_INT08U *pmem); 00283 void WriteLE16U (volatile USB_INT08U *pmem, 00284 USB_INT16U val); 00285 USB_INT32U ReadBE32U (volatile USB_INT08U *pmem); 00286 void WriteBE32U (volatile USB_INT08U *pmem, 00287 USB_INT32U val); 00288 USB_INT16U ReadBE16U (volatile USB_INT08U *pmem); 00289 void WriteBE16U (volatile USB_INT08U *pmem, 00290 USB_INT16U val); 00291 00292 #endif
Generated on Tue Jul 12 2022 18:38:38 by
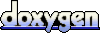