
Bonjour/Zerconf library
Embed:
(wiki syntax)
Show/hide line numbers
net.cpp
00001 00002 /* 00003 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "net.h" 00025 00026 //#define __DEBUG 00027 #include "dbg/dbg.h" 00028 00029 Net::Net() : m_defaultIf(NULL), m_lpIf(), m_lpNetTcpSocket(), m_lpNetUdpSocket() 00030 { 00031 00032 } 00033 00034 Net::~Net() 00035 { 00036 00037 } 00038 00039 00040 void Net::poll() 00041 { 00042 //DBG("\r\nNet : Services polling\r\n"); 00043 00044 //Poll Services 00045 NetService::servicesPoll(); 00046 00047 //DBG("\r\nNet : Interfaces polling\r\n"); 00048 00049 //Poll Interfaces 00050 list<NetIf*>::iterator pIfIt; 00051 00052 for ( pIfIt = net().m_lpIf.begin() ; pIfIt != net().m_lpIf.end(); pIfIt++ ) 00053 { 00054 (*pIfIt)->poll(); 00055 } 00056 00057 //DBG("\r\nNet : Sockets polling\r\n"); 00058 00059 //Poll Tcp Sockets 00060 list<NetTcpSocket*>::iterator pNetTcpSocketIt; 00061 00062 for ( pNetTcpSocketIt = net().m_lpNetTcpSocket.begin() ; pNetTcpSocketIt != net().m_lpNetTcpSocket.end(); ) 00063 { 00064 (*pNetTcpSocketIt)->poll(); 00065 00066 if( (*pNetTcpSocketIt)->m_closed && !((*pNetTcpSocketIt)->m_refs) ) 00067 { 00068 (*pNetTcpSocketIt)->m_removed = true; 00069 delete (*pNetTcpSocketIt); 00070 (*pNetTcpSocketIt) = NULL; 00071 pNetTcpSocketIt = net().m_lpNetTcpSocket.erase(pNetTcpSocketIt); 00072 } 00073 else 00074 { 00075 pNetTcpSocketIt++; 00076 } 00077 } 00078 00079 //Poll Udp Sockets 00080 list<NetUdpSocket*>::iterator pNetUdpSocketIt; 00081 00082 for ( pNetUdpSocketIt = net().m_lpNetUdpSocket.begin() ; pNetUdpSocketIt != net().m_lpNetUdpSocket.end(); ) 00083 { 00084 (*pNetUdpSocketIt)->poll(); 00085 00086 if( (*pNetUdpSocketIt)->m_closed && !((*pNetUdpSocketIt)->m_refs) ) 00087 { 00088 (*pNetUdpSocketIt)->m_removed = true; 00089 delete (*pNetUdpSocketIt); 00090 (*pNetUdpSocketIt) = NULL; 00091 pNetUdpSocketIt = net().m_lpNetUdpSocket.erase(pNetUdpSocketIt); 00092 } 00093 else 00094 { 00095 pNetUdpSocketIt++; 00096 } 00097 } 00098 00099 00100 } 00101 00102 NetTcpSocket* Net::tcpSocket(NetIf& netif) { 00103 NetTcpSocket* pNetTcpSocket = netif.tcpSocket(); 00104 pNetTcpSocket->m_refs++; 00105 return pNetTcpSocket; 00106 } 00107 00108 NetTcpSocket* Net::tcpSocket() { //NetTcpSocket on default if 00109 if ( net().m_defaultIf == NULL ) 00110 return NULL; 00111 NetTcpSocket* pNetTcpSocket = net().m_defaultIf->tcpSocket(); 00112 pNetTcpSocket->m_refs++; 00113 return pNetTcpSocket; 00114 } 00115 00116 void Net::releaseTcpSocket(NetTcpSocket* pNetTcpSocket) 00117 { 00118 pNetTcpSocket->m_refs--; 00119 if(!pNetTcpSocket->m_closed && !pNetTcpSocket->m_refs) 00120 pNetTcpSocket->close(); 00121 } 00122 00123 NetUdpSocket* Net::udpSocket(NetIf& netif) { 00124 NetUdpSocket* pNetUdpSocket = netif.udpSocket(); 00125 pNetUdpSocket->m_refs++; 00126 return pNetUdpSocket; 00127 } 00128 00129 NetUdpSocket* Net::udpSocket() { //NetTcpSocket on default if 00130 if ( net().m_defaultIf == NULL ) 00131 return NULL; 00132 NetUdpSocket* pNetUdpSocket = net().m_defaultIf->udpSocket(); 00133 pNetUdpSocket->m_refs++; 00134 return pNetUdpSocket; 00135 } 00136 00137 void Net::releaseUdpSocket(NetUdpSocket* pNetUdpSocket) 00138 { 00139 pNetUdpSocket->m_refs--; 00140 if(!pNetUdpSocket->m_closed && !pNetUdpSocket->m_refs) 00141 pNetUdpSocket->close(); 00142 } 00143 00144 NetDnsRequest* Net::dnsRequest(const char* hostname, NetIf& netif) { 00145 return netif.dnsRequest(hostname); 00146 } 00147 00148 NetDnsRequest* Net::dnsRequest(const char* hostname) { //Create a new NetDnsRequest object from default if 00149 if ( net().m_defaultIf == NULL ) 00150 return NULL; 00151 return net().m_defaultIf->dnsRequest(hostname); 00152 } 00153 00154 NetDnsRequest* Net::dnsRequest(Host* pHost, NetIf& netif) 00155 { 00156 return netif.dnsRequest(pHost); 00157 } 00158 00159 NetDnsRequest* Net::dnsRequest(Host* pHost) //Creats a new NetDnsRequest object from default if 00160 { 00161 if ( net().m_defaultIf == NULL ) 00162 return NULL; 00163 return net().m_defaultIf->dnsRequest(pHost); 00164 } 00165 00166 void Net::setDefaultIf(NetIf& netif) { 00167 net().m_defaultIf = &netif; 00168 } 00169 00170 void Net::setDefaultIf(NetIf* pIf) 00171 { 00172 net().m_defaultIf = pIf; 00173 } 00174 00175 NetIf* Net::getDefaultIf() { 00176 return net().m_defaultIf; 00177 } 00178 00179 void Net::registerIf(NetIf* pIf) 00180 { 00181 net().m_lpIf.push_back(pIf); 00182 } 00183 00184 void Net::unregisterIf(NetIf* pIf) 00185 { 00186 if( net().m_defaultIf == pIf ) 00187 net().m_defaultIf = NULL; 00188 net().m_lpIf.remove(pIf); 00189 } 00190 00191 void Net::registerNetTcpSocket(NetTcpSocket* pNetTcpSocket) 00192 { 00193 net().m_lpNetTcpSocket.push_back(pNetTcpSocket); 00194 DBG("\r\nNetTcpSocket [ + %p ] %d\r\n", (void*)pNetTcpSocket, net().m_lpNetTcpSocket.size()); 00195 } 00196 00197 void Net::unregisterNetTcpSocket(NetTcpSocket* pNetTcpSocket) 00198 { 00199 DBG("\r\nNetTcpSocket [ - %p ] %d\r\n", (void*)pNetTcpSocket, net().m_lpNetTcpSocket.size() - 1); 00200 if(!pNetTcpSocket->m_removed) 00201 net().m_lpNetTcpSocket.remove(pNetTcpSocket); 00202 } 00203 00204 void Net::registerNetUdpSocket(NetUdpSocket* pNetUdpSocket) 00205 { 00206 net().m_lpNetUdpSocket.push_back(pNetUdpSocket); 00207 DBG("\r\nNetUdpSocket [ + %p ] %d\r\n", (void*)pNetUdpSocket, net().m_lpNetUdpSocket.size()); 00208 } 00209 00210 void Net::unregisterNetUdpSocket(NetUdpSocket* pNetUdpSocket) 00211 { 00212 DBG("\r\nNetUdpSocket [ - %p ] %d\r\n", (void*)pNetUdpSocket, net().m_lpNetUdpSocket.size() - 1); 00213 if(!pNetUdpSocket->m_removed) 00214 net().m_lpNetUdpSocket.remove(pNetUdpSocket); 00215 } 00216 00217 Net& Net::net() 00218 { 00219 static Net* pInst = new Net(); //Called only once 00220 return *pInst; 00221 }
Generated on Tue Jul 12 2022 18:38:37 by
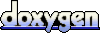